marble
#include <AbstractProjection.h>
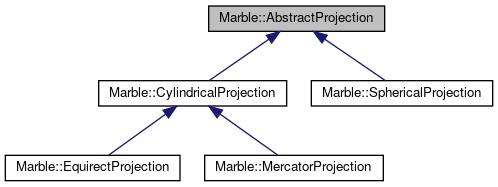
Public Types | |
enum | PreservationType { NoPreservation, Conformal, EqualArea } |
enum | SurfaceType { Cylindrical, Pseudocylindrical, Hybrid, Conical, Pseudoconical, Azimuthal } |
Public Member Functions | |
AbstractProjection () | |
virtual | ~AbstractProjection () |
virtual bool | geoCoordinates (const int x, const int y, const ViewportParams *viewport, qreal &lon, qreal &lat, GeoDataCoordinates::Unit unit=GeoDataCoordinates::Degree) const =0 |
virtual bool | isOrientedNormal () const |
virtual GeoDataLatLonAltBox | latLonAltBox (const QRect &screenRect, const ViewportParams *viewport) const |
virtual bool | mapCoversViewport (const ViewportParams *viewport) const =0 |
QRegion | mapRegion (const ViewportParams *viewport) const |
virtual QPainterPath | mapShape (const ViewportParams *viewport) const =0 |
qreal | maxLat () const |
virtual qreal | maxValidLat () const |
qreal | minLat () const |
virtual qreal | minValidLat () const |
virtual PreservationType | preservationType () const |
virtual bool | repeatableX () const |
bool | screenCoordinates (const qreal lon, const qreal lat, const ViewportParams *viewport, qreal &x, qreal &y) const |
virtual bool | screenCoordinates (const GeoDataCoordinates &geopoint, const ViewportParams *viewport, qreal &x, qreal &y, bool &globeHidesPoint) const =0 |
bool | screenCoordinates (const GeoDataCoordinates &geopoint, const ViewportParams *viewport, qreal &x, qreal &y) const |
virtual bool | screenCoordinates (const GeoDataCoordinates &coordinates, const ViewportParams *viewport, qreal *x, qreal &y, int &pointRepeatNum, const QSizeF &size, bool &globeHidesPoint) const =0 |
virtual bool | screenCoordinates (const GeoDataLineString &lineString, const ViewportParams *viewport, QVector< QPolygonF * > &polygons) const =0 |
void | setMaxLat (qreal maxLat) |
void | setMinLat (qreal minLat) |
virtual SurfaceType | surfaceType () const =0 |
virtual bool | traversableDateLine () const |
virtual bool | traversablePoles () const |
Protected Member Functions | |
AbstractProjection (AbstractProjectionPrivate *dd) | |
Protected Attributes | |
const QScopedPointer < AbstractProjectionPrivate > | d_ptr |
Detailed Description
A base class for all projections in Marble.
Definition at line 49 of file AbstractProjection.h.
Member Enumeration Documentation
Enumerator | |
---|---|
NoPreservation | |
Conformal | |
EqualArea |
Definition at line 62 of file AbstractProjection.h.
Enumerator | |
---|---|
Cylindrical | |
Pseudocylindrical | |
Hybrid | |
Conical | |
Pseudoconical | |
Azimuthal |
Definition at line 53 of file AbstractProjection.h.
Constructor & Destructor Documentation
AbstractProjection::AbstractProjection | ( | ) |
Construct a new AbstractProjection.
Definition at line 28 of file AbstractProjection.cpp.
|
virtual |
Definition at line 38 of file AbstractProjection.cpp.
|
protected |
Definition at line 33 of file AbstractProjection.cpp.
Member Function Documentation
|
pure virtual |
Get the earth coordinates corresponding to a pixel in the map.
- Parameters
-
x the x coordinate of the pixel y the y coordinate of the pixel viewport the viewport parameters lon the longitude angle is returned through this parameter lat the latitude angle is returned through this parameter unit the unit of the angles for lon and lat.
- Returns
true
if the pixel (x, y) is within the globefalse
if the pixel (x, y) is outside the globe, i.e. in space.
Implemented in Marble::SphericalProjection, Marble::EquirectProjection, and Marble::MercatorProjection.
|
virtual |
Definition at line 113 of file AbstractProjection.cpp.
|
virtual |
Reimplemented in Marble::SphericalProjection, Marble::EquirectProjection, and Marble::MercatorProjection.
Definition at line 136 of file AbstractProjection.cpp.
|
pure virtual |
Implemented in Marble::SphericalProjection, Marble::EquirectProjection, and Marble::MercatorProjection.
QRegion AbstractProjection::mapRegion | ( | const ViewportParams * | viewport | ) | const |
Definition at line 216 of file AbstractProjection.cpp.
|
pure virtual |
Implemented in Marble::SphericalProjection, and Marble::CylindricalProjection.
qreal AbstractProjection::maxLat | ( | ) | const |
Definition at line 54 of file AbstractProjection.cpp.
|
virtual |
Reimplemented in Marble::SphericalProjection, Marble::EquirectProjection, and Marble::MercatorProjection.
Definition at line 49 of file AbstractProjection.cpp.
qreal AbstractProjection::minLat | ( | ) | const |
Definition at line 76 of file AbstractProjection.cpp.
|
virtual |
Reimplemented in Marble::SphericalProjection, Marble::EquirectProjection, and Marble::MercatorProjection.
Definition at line 71 of file AbstractProjection.cpp.
|
virtual |
Reimplemented in Marble::SphericalProjection, Marble::EquirectProjection, and Marble::MercatorProjection.
Definition at line 108 of file AbstractProjection.cpp.
|
virtual |
Reimplemented in Marble::SphericalProjection, and Marble::CylindricalProjection.
Definition at line 93 of file AbstractProjection.cpp.
bool AbstractProjection::screenCoordinates | ( | const qreal | lon, |
const qreal | lat, | ||
const ViewportParams * | viewport, | ||
qreal & | x, | ||
qreal & | y | ||
) | const |
Get the screen coordinates corresponding to geographical coordinates in the map.
- Parameters
-
lon the lon coordinate of the requested pixel position in radians lat the lat coordinate of the requested pixel position in radians viewport the viewport parameters x the x coordinate of the pixel is returned through this parameter y the y coordinate of the pixel is returned through this parameter
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
- See also
- ViewportParams
Definition at line 118 of file AbstractProjection.cpp.
|
pure virtual |
Get the screen coordinates corresponding to geographical coordinates in the map.
- Parameters
-
geopoint the point on earth, including altitude, that we want the coordinates for. viewport the viewport parameters x the x coordinate of the pixel is returned through this parameter y the y coordinate of the pixel is returned through this parameter globeHidesPoint whether the point gets hidden on the far side of the earth
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
- See also
- ViewportParams
Implemented in Marble::SphericalProjection, Marble::EquirectProjection, and Marble::MercatorProjection.
bool AbstractProjection::screenCoordinates | ( | const GeoDataCoordinates & | geopoint, |
const ViewportParams * | viewport, | ||
qreal & | x, | ||
qreal & | y | ||
) | const |
Definition at line 127 of file AbstractProjection.cpp.
|
pure virtual |
Get the coordinates of screen points for geographical coordinates in the map.
- Parameters
-
coordinates the point on earth, including altitude, that we want the coordinates for. viewport the viewport parameters x the x coordinates of the pixels are returned through this parameter y the y coordinate of the pixel is returned through this parameter pointRepeatNum the amount of times that a single geographical point gets represented on the map globeHidesPoint whether the point gets hidden on the far side of the earth
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
- See also
- ViewportParams
Implemented in Marble::SphericalProjection, Marble::EquirectProjection, and Marble::MercatorProjection.
|
pure virtual |
Implemented in Marble::SphericalProjection, and Marble::CylindricalProjection.
void AbstractProjection::setMaxLat | ( | qreal | maxLat | ) |
Definition at line 60 of file AbstractProjection.cpp.
void AbstractProjection::setMinLat | ( | qreal | minLat | ) |
Definition at line 82 of file AbstractProjection.cpp.
|
pure virtual |
Implemented in Marble::SphericalProjection, and Marble::CylindricalProjection.
|
virtual |
Reimplemented in Marble::SphericalProjection, and Marble::CylindricalProjection.
Definition at line 103 of file AbstractProjection.cpp.
|
virtual |
Reimplemented in Marble::SphericalProjection, and Marble::CylindricalProjection.
Definition at line 98 of file AbstractProjection.cpp.
Member Data Documentation
|
protected |
Definition at line 193 of file AbstractProjection.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:13:44 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.