marble
#include <EclipsesItem.h>
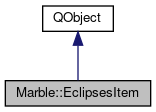
Public Types | |
enum | EclipsePhase { TotalMoon = -4, PartialMoon = -3, PenumbralMoon = -1, PartialSun = 1, NonCentralAnnularSun = 2, NonCentralTotalSun = 3, AnnularSun = 4, TotalSun = 5, AnnularTotalSun = 6 } |
Public Member Functions | |
EclipsesItem (EclSolar *ecl, int index, QObject *parent=0) | |
~EclipsesItem () | |
const GeoDataLineString & | centralLine () |
const QDateTime & | dateMaximum () const |
const QDateTime & | endDatePartial () const |
const QDateTime & | endDateTotal () const |
QIcon | icon () const |
int | index () const |
double | magnitude () const |
const GeoDataCoordinates & | maxLocation () |
const GeoDataLineString & | northernPenumbra () |
int | partialDurationHours () const |
EclipsesItem::EclipsePhase | phase () const |
QString | phaseText () const |
GeoDataLinearRing | shadowCone60MagPenumbra () |
GeoDataLinearRing | shadowConePenumbra () |
GeoDataLinearRing | shadowConeUmbra () |
const GeoDataLineString & | southernPenumbra () |
const QDateTime & | startDatePartial () const |
const QDateTime & | startDateTotal () const |
const QList< GeoDataLinearRing > & | sunBoundaries () |
bool | takesPlaceAt (const QDateTime &dateTime) const |
const GeoDataLinearRing & | umbra () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
The representation of an eclipse event.
This class represents an eclipse event on earth. It calculates all basic information like date and visibility upon initialization. Expensive calculations like boundary polygons are done the first time they are requested.
The calculations are done using the eclsolar backend that has to be passed to the constructor.
Definition at line 37 of file EclipsesItem.h.
Member Enumeration Documentation
A type of an eclipse event.
Enumerator | |
---|---|
TotalMoon | |
PartialMoon | |
PenumbralMoon | |
PartialSun | |
NonCentralAnnularSun | |
NonCentralTotalSun | |
AnnularSun | |
TotalSun | |
AnnularTotalSun |
Definition at line 46 of file EclipsesItem.h.
Constructor & Destructor Documentation
Construct the EclipseItem object and trigger basic calculations.
- Parameters
-
ecl The EclSolar backend parent The parent object
Definition at line 20 of file EclipsesItem.cpp.
Marble::EclipsesItem::~EclipsesItem | ( | ) |
Definition at line 39 of file EclipsesItem.cpp.
Member Function Documentation
const GeoDataLineString & Marble::EclipsesItem::centralLine | ( | ) |
The eclipse's central line.
- Returns
- The central line of the eclipse
Definition at line 144 of file EclipsesItem.cpp.
const QDateTime & Marble::EclipsesItem::dateMaximum | ( | ) | const |
Returns the date of the eclipse event's maximum.
- Returns
- The DateTime of the eclipse's maximum
- See also
- maxLocation
Definition at line 104 of file EclipsesItem.cpp.
const QDateTime & Marble::EclipsesItem::endDatePartial | ( | ) | const |
Returns the end date of the eclipse's partial phase.
- Returns
- The end date of the partial phase
- See also
- startDatePartial
Definition at line 114 of file EclipsesItem.cpp.
const QDateTime & Marble::EclipsesItem::endDateTotal | ( | ) | const |
Returns the end date of the eclipse's total phase.
If the eclipse has a total phase, the date and time of its ending is returned. If this is no total eclipse, an invalid datetime object will be returned.
- Returns
- The end date of the total phase or an invalid date
- See also
- startDateTotal
Definition at line 130 of file EclipsesItem.cpp.
QIcon Marble::EclipsesItem::icon | ( | ) | const |
Returns an icon of the eclipse type.
- Returns
- an icon representing the eclipse's type
Definition at line 59 of file EclipsesItem.cpp.
int Marble::EclipsesItem::index | ( | ) | const |
The index of the eclipse event.
Returns the index of the eclipse event.
- Returns
- The eclipse events index.
Definition at line 43 of file EclipsesItem.cpp.
double Marble::EclipsesItem::magnitude | ( | ) | const |
Return the eclipse's magnitude.
- Returns
- The magnitude of the eclipse
Definition at line 99 of file EclipsesItem.cpp.
const GeoDataCoordinates & Marble::EclipsesItem::maxLocation | ( | ) |
Return the coordinates of the eclipse's maximum.
- Returns
- GeoDataCoordinates of the eclipse's maximum
- See also
- dateMaximum
Definition at line 135 of file EclipsesItem.cpp.
const GeoDataLineString & Marble::EclipsesItem::northernPenumbra | ( | ) |
Return the eclipse's northern penumbra.
- Returns
- The eclipse's northern umbra
Definition at line 171 of file EclipsesItem.cpp.
int Marble::EclipsesItem::partialDurationHours | ( | ) | const |
Returns the number of hours the partial phase takes place.
- Returns
- The number of hours of the partial phase
- See also
- startDatePartial, endDatePartial
Definition at line 119 of file EclipsesItem.cpp.
EclipsesItem::EclipsePhase Marble::EclipsesItem::phase | ( | ) | const |
Returns the phase of this eclipse event.
- Returns
- the phase or type of this eclipse event
- See also
- phaseText
Definition at line 54 of file EclipsesItem.cpp.
QString Marble::EclipsesItem::phaseText | ( | ) | const |
Returns a human readable representation of the eclipse type.
- Returns
- A string representing the eclipse's type
- See also
- phase
Definition at line 82 of file EclipsesItem.cpp.
GeoDataLinearRing Marble::EclipsesItem::shadowCone60MagPenumbra | ( | ) |
Return the shadow cone of the penumbra at 60 percent magnitude.
- Returns
- The shadow cone of the penumbra at 60 percent magnitude
Definition at line 198 of file EclipsesItem.cpp.
GeoDataLinearRing Marble::EclipsesItem::shadowConePenumbra | ( | ) |
Return the shadow cone of the penumbra.
- Returns
- The shadow cone of the penumbra
Definition at line 189 of file EclipsesItem.cpp.
GeoDataLinearRing Marble::EclipsesItem::shadowConeUmbra | ( | ) |
Return the shadow cone of the umbra.
- Returns
- The shadow cone of the umbra
Definition at line 180 of file EclipsesItem.cpp.
const GeoDataLineString & Marble::EclipsesItem::southernPenumbra | ( | ) |
Return the eclipse's southern penumbra.
- Returns
- The eclipse's southern penumbra
Definition at line 162 of file EclipsesItem.cpp.
const QDateTime & Marble::EclipsesItem::startDatePartial | ( | ) | const |
Returns the start date of the eclipse's partial phase.
- Returns
- The start date of the partial phase
- See also
- endDatePartial
Definition at line 109 of file EclipsesItem.cpp.
const QDateTime & Marble::EclipsesItem::startDateTotal | ( | ) | const |
Returns the start date of the eclipse's total phase.
If the eclipse has a total phase, the date and time of its beginning is returned. If this is no total eclipse, an invalid datetime object will be returned.
- Returns
- The start date of the total phase or an invalid date
- See also
- endDateTotal
Definition at line 125 of file EclipsesItem.cpp.
const QList< GeoDataLinearRing > & Marble::EclipsesItem::sunBoundaries | ( | ) |
Return the eclipse's sun boundaries.
- Returns
- The eclipse's sun boundaries
Definition at line 207 of file EclipsesItem.cpp.
bool Marble::EclipsesItem::takesPlaceAt | ( | const QDateTime & | dateTime | ) | const |
Check if the event takes place at a given datetime.
- Parameters
-
dateTime The date time to check
Checks whether or not this eclipse event takes place at the given dateTime
. This is true if dateTime
is between the global start and end dates of the event.
- Returns
- True if the event takes place at
dateTime
or false otherwise.
Definition at line 48 of file EclipsesItem.cpp.
const GeoDataLinearRing & Marble::EclipsesItem::umbra | ( | ) |
Return the eclipse's umbra.
- Returns
- The eclipse's umbra
Definition at line 153 of file EclipsesItem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:13:44 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.