marble
#include <GeoDataPolygon.h>
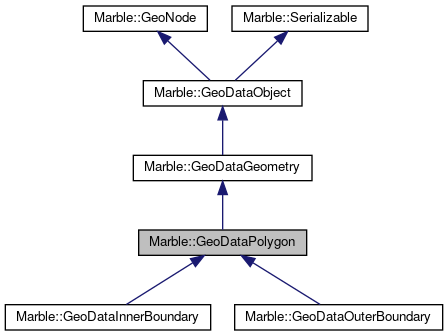
Additional Inherited Members | |
![]() | |
bool | equals (const GeoDataGeometry &other) const |
![]() | |
virtual bool | equals (const GeoDataObject &other) const |
Detailed Description
A polygon that can have "holes".
GeoDataPolygon is a tool class that implements the Polygon tag/class of the Open Geospatial Consortium standard KML 2.2.
GeoDataPolygon extends GeoDataGeometry to store and edit Polygons.
In the QPainter API "pure" Polygons would represent polygons with "holes" inside. However QPolygon doesn't provide this feature directly.
Whenever a Polygon is painted GeoDataLineStyle should be used to assign a color and line width.
The polygon consists of
- a single outer boundary and
- optionally a set of inner boundaries.
All boundaries are LinearRings.
The boundaries of a GeoDataPolygon consist of several (geodetic) nodes which are each connected through line segments. The nodes are stored as GeoDataCoordinates objects.
The API which provides access to the nodes is similar to the API of QVector.
GeoDataPolygon allows Polygons to be tessellated in order to make them follow the terrain and the curvature of the earth. The tessellation options allow for different ways of visualization:
- Not tessellated: A Polygon that connects each two nodes directly and straight in screen coordinate space.
- A tessellated line: Each line segment is bent so that the Polygon follows the curvature of the earth and its terrain. A tessellated line segment connects two nodes at the shortest possible distance ("along great circles").
- A tessellated line that follows latitude circles whenever possible: In this case Latitude circles are followed as soon as two subsequent nodes have exactly the same amount of latitude. In all other places the line segments follow great circles.
Some convenience methods have been added that allow to calculate the geodesic bounding box or the length of a Polygon.
- See also
- GeoDataLinearRing
Definition at line 81 of file GeoDataPolygon.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new Polygon.
Definition at line 22 of file GeoDataPolygon.cpp.
|
explicit |
Creates a Polygon from an existing geometry object.
Definition at line 27 of file GeoDataPolygon.cpp.
|
virtual |
Destroys a Polygon.
Definition at line 32 of file GeoDataPolygon.cpp.
Member Function Documentation
void Marble::GeoDataPolygon::appendInnerBoundary | ( | const GeoDataLinearRing & | boundary | ) |
Appends a given LinearRing as an inner boundary of the Polygon.
- See also
- GeoDataLinearRing
Definition at line 149 of file GeoDataPolygon.cpp.
|
virtual |
Returns whether the given coordinates lie within the polygon.
- Returns
true
if the coordinates lie within the polygon (and not in its holes), false otherwise.
Definition at line 196 of file GeoDataPolygon.cpp.
QVector< GeoDataLinearRing > & Marble::GeoDataPolygon::innerBoundaries | ( | ) |
Returns a set of inner boundaries which are represented as LinearRings.
- See also
- GeoDataLinearRing
Definition at line 139 of file GeoDataPolygon.cpp.
const QVector< GeoDataLinearRing > & Marble::GeoDataPolygon::innerBoundaries | ( | ) | const |
Returns a set of inner boundaries which are represented as LinearRings.
- See also
- GeoDataLinearRing
Definition at line 144 of file GeoDataPolygon.cpp.
|
virtual |
Returns whether a Polygon is a closed polygon.
- Returns
true
for a Polygon.
Definition at line 82 of file GeoDataPolygon.cpp.
|
virtual |
Returns the smallest latLonAltBox that contains the Polygon.
- See also
- GeoDataLatLonAltBox
Reimplemented from Marble::GeoDataGeometry.
Definition at line 118 of file GeoDataPolygon.cpp.
bool Marble::GeoDataPolygon::operator!= | ( | const GeoDataPolygon & | other | ) | const |
Definition at line 77 of file GeoDataPolygon.cpp.
bool Marble::GeoDataPolygon::operator== | ( | const GeoDataPolygon & | other | ) | const |
Returns true/false depending on whether this and other are/are not equal.
Definition at line 49 of file GeoDataPolygon.cpp.
GeoDataLinearRing & Marble::GeoDataPolygon::outerBoundary | ( | ) |
Returns the outer boundary that is represented as a LinearRing.
- See also
- GeoDataLinearRing
Definition at line 123 of file GeoDataPolygon.cpp.
const GeoDataLinearRing & Marble::GeoDataPolygon::outerBoundary | ( | ) | const |
Returns the outer boundary that is represented as a LinearRing.
- See also
- GeoDataLinearRing
Definition at line 128 of file GeoDataPolygon.cpp.
|
virtual |
Serialize the Polygon to a stream.
- Parameters
-
stream the stream.
Reimplemented from Marble::GeoDataGeometry.
Definition at line 155 of file GeoDataPolygon.cpp.
void Marble::GeoDataPolygon::setOuterBoundary | ( | const GeoDataLinearRing & | boundary | ) |
Sets the given LinearRing as an outer boundary of the Polygon.
- See also
- GeoDataLinearRing
Definition at line 133 of file GeoDataPolygon.cpp.
void Marble::GeoDataPolygon::setTessellate | ( | bool | tessellate | ) |
Sets the tessellation property for the Polygon.
If tessellate is true
then the Polygon's line segments are bent and follow the earth's surface and terrain along great circles. If tessellate is false
then the Polygon's line segments are rendered as straight lines in screen coordinate space.
Definition at line 92 of file GeoDataPolygon.cpp.
void Marble::GeoDataPolygon::setTessellationFlags | ( | TessellationFlags | f | ) |
Sets the given tessellation flags for a Polygon.
Definition at line 112 of file GeoDataPolygon.cpp.
bool Marble::GeoDataPolygon::tessellate | ( | ) | const |
Returns whether the Polygon follows the earth's surface.
- Returns
true
if the Polygon's line segments follow the earth's surface and terrain along great circles.
Definition at line 87 of file GeoDataPolygon.cpp.
TessellationFlags Marble::GeoDataPolygon::tessellationFlags | ( | ) | const |
Returns the tessellation flags for a Polygon.
Definition at line 107 of file GeoDataPolygon.cpp.
|
virtual |
Unserialize the Polygon from a stream.
- Parameters
-
stream the stream.
Reimplemented from Marble::GeoDataGeometry.
Definition at line 174 of file GeoDataPolygon.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:13:45 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.