marble
#include <GeoDataStyleMap.h>
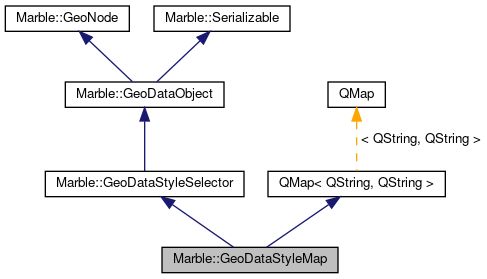
Public Member Functions | |
GeoDataStyleMap () | |
GeoDataStyleMap (const GeoDataStyleMap &other) | |
~GeoDataStyleMap () | |
QString | lastKey () const |
virtual const char * | nodeType () const |
bool | operator!= (const GeoDataStyleMap &other) const |
GeoDataStyleMap & | operator= (const GeoDataStyleMap &other) |
bool | operator== (const GeoDataStyleMap &other) const |
virtual void | pack (QDataStream &stream) const |
void | setLastKey (QString key) |
virtual void | unpack (QDataStream &stream) |
![]() | |
GeoDataStyleSelector () | |
GeoDataStyleSelector (const GeoDataStyleSelector &other) | |
~GeoDataStyleSelector () | |
bool | operator!= (const GeoDataStyleSelector &other) const |
GeoDataStyleSelector & | operator= (const GeoDataStyleSelector &other) |
bool | operator== (const GeoDataStyleSelector &other) const |
![]() | |
GeoDataObject () | |
GeoDataObject (const GeoDataObject &) | |
virtual | ~GeoDataObject () |
QString | id () const |
GeoDataObject & | operator= (const GeoDataObject &) |
virtual GeoDataObject * | parent () const |
QString | resolvePath (const QString &relativePath) const |
void | setId (const QString &value) |
virtual void | setParent (GeoDataObject *parent) |
void | setTargetId (const QString &value) |
QString | targetId () const |
![]() | |
GeoNode () | |
virtual | ~GeoNode () |
![]() | |
virtual | ~Serializable () |
![]() | |
QMap () | |
QMap (const QMap< Key, T > &other) | |
QMap (const std::map< Key, T > &other) | |
~QMap () | |
iterator | begin () |
const_iterator | begin () const |
void | clear () |
const_iterator | constBegin () const |
const_iterator | constEnd () const |
const_iterator | constFind (const Key &key) const |
bool | contains (const Key &key) const |
int | count () const |
int | count (const Key &key) const |
bool | empty () const |
iterator | end () |
const_iterator | end () const |
iterator | erase (iterator pos) |
void | erase (const Key &key) |
iterator | find (const Key &key) |
const_iterator | find (const Key &key) const |
iterator | insert (const Key &key, const T &value) |
iterator | insert (const Key &key, const T &value, bool overwrite) |
iterator | insertMulti (const Key &key, const T &value) |
bool | isEmpty () const |
const Key | key (const T &value) const |
const Key | key (const T &value, const Key &defaultKey) const |
QList< Key > | keys (const T &value) const |
QList< Key > | keys () const |
iterator | lowerBound (const Key &key) |
const_iterator | lowerBound (const Key &key) const |
bool | operator!= (const QMap< Key, T > &other) const |
QMap< Key, T > & | operator= (const QMap< Key, T > &other) |
bool | operator== (const QMap< Key, T > &other) const |
T & | operator[] (const Key &key) |
const T | operator[] (const Key &key) const |
int | remove (const Key &key) |
iterator | remove (iterator it) |
iterator | replace (const Key &key, const T &value) |
int | size () const |
void | swap (QMap< Key, T > &other) |
T | take (const Key &key) |
std::map< Key, T > | toStdMap () const |
QList< Key > | uniqueKeys () const |
QMap< Key, T > & | unite (const QMap< Key, T > &other) |
const_iterator | upperBound (const Key &key) const |
iterator | upperBound (const Key &key) |
const T | value (const Key &key, const T &defaultValue) const |
const T | value (const Key &key) const |
QList< T > | values () const |
QList< T > | values (const Key &key) const |
Additional Inherited Members | |
![]() | |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | key_type |
typedef | mapped_type |
typedef | size_type |
![]() | |
virtual bool | equals (const GeoDataObject &other) const |
Detailed Description
a class to map different styles to one style
A GeoDataStyleMap connects styles for highlighted and normal context. Besides GeoDataStyleSelector it derives from QMap<QString, QString>.
GeoDataStyle GeoDataStyleSelector
Definition at line 38 of file GeoDataStyleMap.h.
Constructor & Destructor Documentation
Marble::GeoDataStyleMap::GeoDataStyleMap | ( | ) |
Definition at line 32 of file GeoDataStyleMap.cpp.
Marble::GeoDataStyleMap::GeoDataStyleMap | ( | const GeoDataStyleMap & | other | ) |
Definition at line 37 of file GeoDataStyleMap.cpp.
Marble::GeoDataStyleMap::~GeoDataStyleMap | ( | ) |
Definition at line 43 of file GeoDataStyleMap.cpp.
Member Function Documentation
QString Marble::GeoDataStyleMap::lastKey | ( | ) | const |
return the last key
Definition at line 53 of file GeoDataStyleMap.cpp.
|
virtual |
Provides type information for downcasting a GeoNode.
Reimplemented from Marble::GeoDataStyleSelector.
Definition at line 48 of file GeoDataStyleMap.cpp.
bool Marble::GeoDataStyleMap::operator!= | ( | const GeoDataStyleMap & | other | ) | const |
Definition at line 82 of file GeoDataStyleMap.cpp.
GeoDataStyleMap & Marble::GeoDataStyleMap::operator= | ( | const GeoDataStyleMap & | other | ) |
assignment operator
- Parameters
-
other the styleMap which gets duplicated.
Definition at line 63 of file GeoDataStyleMap.cpp.
bool Marble::GeoDataStyleMap::operator== | ( | const GeoDataStyleMap & | other | ) | const |
Definition at line 71 of file GeoDataStyleMap.cpp.
|
virtual |
Serialize the stylemap to a stream.
- Parameters
-
stream the stream
Reimplemented from Marble::GeoDataStyleSelector.
Definition at line 87 of file GeoDataStyleMap.cpp.
void Marble::GeoDataStyleMap::setLastKey | ( | QString | key | ) |
Set the last key this property is needed to set an entry in the kml parser after the parser has set the last key, it will read the value and add both to this map.
- Parameters
-
key the last key
Definition at line 58 of file GeoDataStyleMap.cpp.
|
virtual |
Unserialize the stylemap from a stream.
- Parameters
-
stream the stream
Reimplemented from Marble::GeoDataStyleSelector.
Definition at line 94 of file GeoDataStyleMap.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:13:45 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.