marble
#include <MarbleGraphicsItem.h>
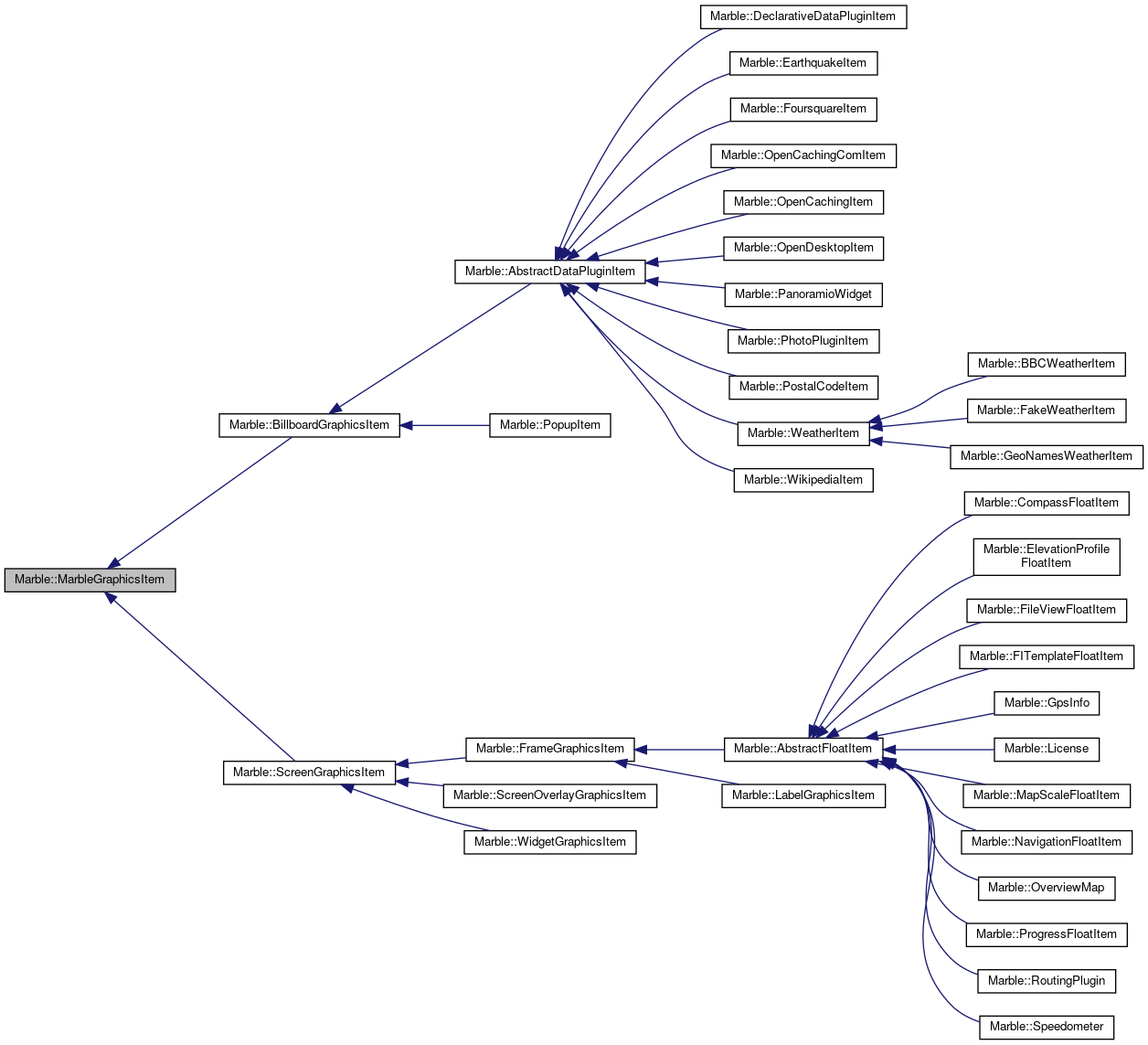
Public Types | |
enum | CacheMode { NoCache, ItemCoordinateCache, DeviceCoordinateCache } |
Public Member Functions | |
virtual | ~MarbleGraphicsItem () |
CacheMode | cacheMode () const |
bool | contains (const QPointF &point) const |
virtual QRectF | contentRect () const |
virtual QSizeF | contentSize () const |
void | hide () |
AbstractMarbleGraphicsLayout * | layout () const |
bool | paintEvent (QPainter *painter, const ViewportParams *viewport) |
void | setCacheMode (CacheMode mode) |
virtual void | setContentSize (const QSizeF &size) |
void | setLayout (AbstractMarbleGraphicsLayout *layout) |
virtual void | setProjection (const ViewportParams *viewport) |
void | setSize (const QSizeF &size) |
void | setVisible (bool visible) |
void | show () |
QSizeF | size () const |
bool | visible () const |
Protected Member Functions | |
MarbleGraphicsItem (MarbleGraphicsItemPrivate *d_ptr) | |
virtual bool | eventFilter (QObject *object, QEvent *e) |
virtual void | paint (QPainter *painter) |
void | update () |
Protected Attributes | |
MarbleGraphicsItemPrivate *const | d |
Detailed Description
Definition at line 34 of file MarbleGraphicsItem.h.
Member Enumeration Documentation
Enumerator | |
---|---|
NoCache | |
ItemCoordinateCache | |
DeviceCoordinateCache |
Definition at line 39 of file MarbleGraphicsItem.h.
Constructor & Destructor Documentation
|
virtual |
Definition at line 33 of file MarbleGraphicsItem.cpp.
|
explicitprotected |
Definition at line 28 of file MarbleGraphicsItem.cpp.
Member Function Documentation
MarbleGraphicsItem::CacheMode MarbleGraphicsItem::cacheMode | ( | ) | const |
Returns the cache mode of the item.
Definition at line 154 of file MarbleGraphicsItem.cpp.
bool MarbleGraphicsItem::contains | ( | const QPointF & | point | ) | const |
Returns true if the Item contains point
in parent coordinates.
Definition at line 110 of file MarbleGraphicsItem.cpp.
|
virtual |
Returns the rect of the content in item coordinates.
Reimplemented in Marble::FrameGraphicsItem.
Definition at line 215 of file MarbleGraphicsItem.cpp.
|
virtual |
Returns the size of the content of the MarbleGraphicsItem.
This is identical to size() for default MarbleGraphicsItems.
Reimplemented in Marble::FrameGraphicsItem.
Definition at line 205 of file MarbleGraphicsItem.cpp.
Reimplemented in Marble::AbstractFloatItem, Marble::PopupItem, Marble::ScreenGraphicsItem, Marble::OverviewMap, Marble::ElevationProfileFloatItem, Marble::NavigationFloatItem, Marble::FileViewFloatItem, Marble::RoutingPlugin, Marble::License, and Marble::WidgetGraphicsItem.
Definition at line 225 of file MarbleGraphicsItem.cpp.
void MarbleGraphicsItem::hide | ( | ) |
Hides the item.
Equivalent to setVisible( false )
Definition at line 187 of file MarbleGraphicsItem.cpp.
AbstractMarbleGraphicsLayout * MarbleGraphicsItem::layout | ( | ) | const |
Returns the layout of the MarbleGraphicsItem.
Definition at line 141 of file MarbleGraphicsItem.cpp.
|
protectedvirtual |
Paints the item in item coordinates.
This has to be reimplemented by the subclass This function will be called by paintEvent().
Reimplemented in Marble::FrameGraphicsItem, Marble::PopupItem, Marble::WikipediaItem, Marble::OpenCachingComItem, Marble::WidgetGraphicsItem, Marble::FoursquareItem, Marble::OpenDesktopItem, Marble::PanoramioWidget, Marble::EarthquakeItem, Marble::ScreenOverlayGraphicsItem, and Marble::PostalCodeItem.
Definition at line 220 of file MarbleGraphicsItem.cpp.
bool MarbleGraphicsItem::paintEvent | ( | QPainter * | painter, |
const ViewportParams * | viewport | ||
) |
Paints the item on the screen in view coordinates.
It is not safe to call this function from a thread other than the gui thread.
Definition at line 38 of file MarbleGraphicsItem.cpp.
void MarbleGraphicsItem::setCacheMode | ( | CacheMode | mode | ) |
Set the cache mode of the item.
Definition at line 159 of file MarbleGraphicsItem.cpp.
|
virtual |
Set the size of the content of the item.
Reimplemented in Marble::FrameGraphicsItem, and Marble::LabelGraphicsItem.
Definition at line 210 of file MarbleGraphicsItem.cpp.
void MarbleGraphicsItem::setLayout | ( | AbstractMarbleGraphicsLayout * | layout | ) |
Set the layout of the graphics item.
The layout will now handle positions of added child items. The MarbleGraphicsItem takes ownership of the layout.
Definition at line 146 of file MarbleGraphicsItem.cpp.
|
virtual |
Reimplemented in Marble::ElevationProfileFloatItem, Marble::OverviewMap, Marble::NavigationFloatItem, Marble::CompassFloatItem, Marble::MapScaleFloatItem, and Marble::ScreenOverlayGraphicsItem.
Definition at line 272 of file MarbleGraphicsItem.cpp.
void MarbleGraphicsItem::setSize | ( | const QSizeF & | size | ) |
Set the size of the item.
Definition at line 197 of file MarbleGraphicsItem.cpp.
void MarbleGraphicsItem::setVisible | ( | bool | visible | ) |
Makes the item visible or invisible, depending on visible
.
Definition at line 182 of file MarbleGraphicsItem.cpp.
void MarbleGraphicsItem::show | ( | ) |
Shows the item.
Equivalent to setVisible( true )
Definition at line 192 of file MarbleGraphicsItem.cpp.
QSizeF MarbleGraphicsItem::size | ( | ) | const |
Returns the size of the item.
Definition at line 136 of file MarbleGraphicsItem.cpp.
|
protected |
Marks the item and all parent items as invalid.
If caching is enabled, the next paintEvent() will cause the cache to be recreated, such that the paintEvent()s after will be optimized.
Definition at line 167 of file MarbleGraphicsItem.cpp.
bool MarbleGraphicsItem::visible | ( | ) | const |
Returns if the item is visible.
Definition at line 177 of file MarbleGraphicsItem.cpp.
Member Data Documentation
|
protected |
Definition at line 144 of file MarbleGraphicsItem.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:13:45 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.