marble
#include <MarbleMap.h>
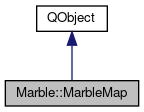
Public Slots | |
void | centerOn (const qreal lon, const qreal lat) |
void | clearVolatileTileCache () |
void | downloadRegion (QVector< TileCoordsPyramid > const &) |
void | notifyMouseClick (int x, int y) |
void | paint (GeoPainter &painter, const QRect &dirtyRect) |
void | reload () |
void | rotateBy (const qreal &deltaLon, const qreal &deltaLat) |
void | setCenterLatitude (qreal lat) |
void | setCenterLongitude (qreal lon) |
void | setDefaultAngleUnit (AngleUnit angleUnit) |
void | setDefaultFont (const QFont &font) |
void | setLockToSubSolarPoint (bool visible) |
void | setMapThemeId (const QString &maptheme) |
void | setProjection (Projection projection) |
void | setPropertyValue (const QString &name, bool value) |
void | setRadius (int radius) |
void | setShowAtmosphere (bool visible) |
void | setShowBackground (bool visible) |
void | setShowBorders (bool visible) |
void | setShowCities (bool visible) |
void | setShowCityLights (bool visible) |
void | setShowClouds (bool visible) |
void | setShowCompass (bool visible) |
void | setShowCrosshairs (bool visible) |
void | setShowFrameRate (bool visible) |
void | setShowGrid (bool visible) |
void | setShowIceLayer (bool visible) |
void | setShowLakes (bool visible) |
void | setShowOtherPlaces (bool visible) |
void | setShowOverviewMap (bool visible) |
void | setShowPlaces (bool visible) |
void | setShowRelief (bool visible) |
void | setShowRivers (bool visible) |
void | setShowRuntimeTrace (bool visible) |
void | setShowScaleBar (bool visible) |
void | setShowSunShading (bool visible) |
void | setShowTerrain (bool visible) |
void | setShowTileId (bool visible) |
void | setSubSolarPointIconVisible (bool visible) |
void | setVolatileTileCacheLimit (quint64 kiloBytes) |
Signals | |
void | framesPerSecond (qreal fps) |
void | mouseClickGeoPosition (qreal lon, qreal lat, GeoDataCoordinates::Unit) |
void | mouseMoveGeoPosition (const QString &) |
void | pluginSettingsChanged () |
void | projectionChanged (Projection) |
void | radiusChanged (int radius) |
void | renderPluginInitialized (RenderPlugin *renderPlugin) |
void | renderStateChanged (const RenderState &state) |
void | renderStatusChanged (RenderStatus status) |
void | repaintNeeded (const QRegion &dirtyRegion=QRegion()) |
void | themeChanged (const QString &theme) |
void | tileLevelChanged (int level) |
void | visibleLatLonAltBoxChanged (const GeoDataLatLonAltBox &visibleLatLonAltBox) |
Public Member Functions | |
MarbleMap () | |
MarbleMap (MarbleModel *model) | |
virtual | ~MarbleMap () |
void | addLayer (LayerInterface *layer) |
qreal | centerLatitude () const |
qreal | centerLongitude () const |
QList< AbstractDataPlugin * > | dataPlugins () const |
AngleUnit | defaultAngleUnit () const |
QFont | defaultFont () const |
AbstractFloatItem * | floatItem (const QString &nameId) const |
QList< AbstractFloatItem * > | floatItems () const |
bool | geoCoordinates (int x, int y, qreal &lon, qreal &lat, GeoDataCoordinates::Unit=GeoDataCoordinates::Degree) const |
int | height () const |
bool | isLockedToSubSolarPoint () const |
bool | isSubSolarPointIconVisible () const |
MapQuality | mapQuality (ViewContext viewContext) const |
MapQuality | mapQuality () const |
QString | mapThemeId () const |
int | maximumZoom () const |
int | minimumZoom () const |
MarbleModel * | model () const |
int | preferredRadiusCeil (int radius) |
int | preferredRadiusFloor (int radius) |
Projection | projection () const |
bool | propertyValue (const QString &name) const |
int | radius () const |
void | removeLayer (LayerInterface *layer) |
QList< RenderPlugin * > | renderPlugins () const |
RenderState | renderState () const |
RenderStatus | renderStatus () const |
bool | screenCoordinates (qreal lon, qreal lat, qreal &x, qreal &y) const |
void | setMapQualityForViewContext (MapQuality qualityForViewContext, ViewContext viewContext) |
void | setSize (int width, int height) |
void | setSize (const QSize &size) |
void | setViewContext (ViewContext viewContext) |
bool | showAtmosphere () const |
bool | showBackground () const |
bool | showBorders () const |
bool | showCities () const |
bool | showCityLights () const |
bool | showClouds () const |
bool | showCompass () const |
bool | showCrosshairs () const |
bool | showFrameRate () const |
bool | showGrid () const |
bool | showIceLayer () const |
bool | showLakes () const |
bool | showOtherPlaces () const |
bool | showOverviewMap () const |
bool | showPlaces () const |
bool | showRelief () const |
bool | showRivers () const |
bool | showScaleBar () const |
bool | showSunShading () const |
bool | showTerrain () const |
QSize | size () const |
TextureLayer * | textureLayer () const |
int | tileZoomLevel () const |
ViewContext | viewContext () const |
ViewportParams * | viewport () |
const ViewportParams * | viewport () const |
quint64 | volatileTileCacheLimit () const |
QVector< const GeoDataPlacemark * > | whichFeatureAt (const QPoint &) const |
QList< AbstractDataPluginItem * > | whichItemAt (const QPoint &curpos) const |
int | width () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
virtual void | customPaint (GeoPainter *painter) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
A class that can paint a view of the earth.
FIXME: Change this description when we are done.
This class can paint a view of the earth or any other globe, depending on which dataset is used. It can be used to show the globe in a widget like MarbleWidget does, or on any other QPaintDevice.
The projection and other view parameters that control how MarbleMap paints the map is given through the class ViewParams. If the programmer wants to allow the user to control the map, he/she has to provide a way for the user to interact with it. An example of this can be seen in the class MarbleWidgetInputHandler, that lets the user control a MarbleWidget that uses MarbleMap internally.
The MarbleMap needs to be provided with a data model to work. This model is contained in the MarbleModel class. The widget can also construct its own model if none is given to the constructor. This data model contains 3 separate datatypes: tiles which provide the background, vectors which provide things like country borders and coastlines and placemarks which can show points of interest, such as cities, mountain tops or the poles.
- See also
- MarbleWidget
- MarbleControlBox
- MarbleModel
Definition at line 91 of file MarbleMap.h.
Constructor & Destructor Documentation
Marble::MarbleMap::MarbleMap | ( | ) |
Construct a new MarbleMap.
This constructor should be used when you will only use one MarbleMap. The widget will create its own MarbleModel when created.
Definition at line 244 of file MarbleMap.cpp.
|
explicit |
Construct a new MarbleMap.
- Parameters
-
model the data model for the widget.
This constructor should be used when you plan to use more than one MarbleMap for the same MarbleModel (not yet supported, but will be soon).
Definition at line 255 of file MarbleMap.cpp.
|
virtual |
Definition at line 268 of file MarbleMap.cpp.
Member Function Documentation
void Marble::MarbleMap::addLayer | ( | LayerInterface * | layer | ) |
Add a layer to be included in rendering.
Definition at line 1237 of file MarbleMap.cpp.
qreal Marble::MarbleMap::centerLatitude | ( | ) | const |
Return the latitude of the center point.
- Returns
- The latitude of the center point in degree.
Definition at line 406 of file MarbleMap.cpp.
qreal Marble::MarbleMap::centerLongitude | ( | ) | const |
Return the longitude of the center point.
- Returns
- The longitude of the center point in degree.
Definition at line 414 of file MarbleMap.cpp.
|
slot |
Center the view on a geographical point.
- Parameters
-
lat an angle parallel to the latitude lines +90(N) - -90(S) lon an angle parallel to the longitude lines +180(W) - -180(E)
Definition at line 643 of file MarbleMap.cpp.
|
slot |
Definition at line 1158 of file MarbleMap.cpp.
|
protectedvirtual |
Enables custom drawing onto the MarbleMap straight after.
the globe and before all other layers have been rendered.
- Parameters
-
painter
- Deprecated:
- implement LayerInterface and add it using
addLayer()
Definition at line 772 of file MarbleMap.cpp.
QList< AbstractDataPlugin * > Marble::MarbleMap::dataPlugins | ( | ) | const |
Returns a list of all DataPlugins on the layer.
- Returns
- the list of DataPlugins
Definition at line 1227 of file MarbleMap.cpp.
AngleUnit Marble::MarbleMap::defaultAngleUnit | ( | ) | const |
Definition at line 1171 of file MarbleMap.cpp.
QFont Marble::MarbleMap::defaultFont | ( | ) | const |
Definition at line 1195 of file MarbleMap.cpp.
|
slot |
Definition at line 448 of file MarbleMap.cpp.
AbstractFloatItem * Marble::MarbleMap::floatItem | ( | const QString & | nameId | ) | const |
Returns a list of all FloatItems in the model.
- Returns
- the list of the floatItems
Definition at line 1216 of file MarbleMap.cpp.
QList< AbstractFloatItem * > Marble::MarbleMap::floatItems | ( | ) | const |
Definition at line 1211 of file MarbleMap.cpp.
|
signal |
bool Marble::MarbleMap::geoCoordinates | ( | int | x, |
int | y, | ||
qreal & | lon, | ||
qreal & | lat, | ||
GeoDataCoordinates::Unit | unit = GeoDataCoordinates::Degree |
||
) | const |
Get the earth coordinates corresponding to a pixel in the map.
- Parameters
-
x the x coordinate of the pixel y the y coordinate of the pixel lon the longitude angle is returned through this parameter lat the latitude angle is returned through this parameter
- Returns
true
if the pixel (x, y) is within the globefalse
if the pixel (x, y) is outside the globe, i.e. in space.
Definition at line 686 of file MarbleMap.cpp.
int Marble::MarbleMap::height | ( | ) | const |
Definition at line 359 of file MarbleMap.cpp.
bool Marble::MarbleMap::isLockedToSubSolarPoint | ( | ) | const |
Return whether the globe is locked to the sub solar point.
- Returns
- if globe is locked to sub solar point
Definition at line 544 of file MarbleMap.cpp.
bool Marble::MarbleMap::isSubSolarPointIconVisible | ( | ) | const |
Return whether the sun icon is shown in the sub solar point.
- Returns
- visibility of the sun icon in the sub solar point
Definition at line 549 of file MarbleMap.cpp.
MapQuality Marble::MarbleMap::mapQuality | ( | ViewContext | viewContext | ) | const |
Definition at line 307 of file MarbleMap.cpp.
MapQuality Marble::MarbleMap::mapQuality | ( | ) | const |
Return the current map quality.
Definition at line 312 of file MarbleMap.cpp.
QString Marble::MarbleMap::mapThemeId | ( | ) | const |
Get the ID of the current map theme To ensure that a unique identifier is being used the theme does NOT get represented by its name but the by relative location of the file that specifies the theme:
Example: maptheme = "earth/bluemarble/bluemarble.dgml"
Definition at line 777 of file MarbleMap.cpp.
int Marble::MarbleMap::maximumZoom | ( | ) | const |
return the minimum zoom value for the current map theme.
Definition at line 430 of file MarbleMap.cpp.
int Marble::MarbleMap::minimumZoom | ( | ) | const |
return the minimum zoom value for the current map theme.
Definition at line 422 of file MarbleMap.cpp.
MarbleModel * Marble::MarbleMap::model | ( | ) | const |
Return the model that this view shows.
Definition at line 283 of file MarbleMap.cpp.
|
signal |
|
signal |
|
slot |
used to notify about the position of the mouse click
Definition at line 1146 of file MarbleMap.cpp.
|
slot |
Paint the map using a give painter.
- Parameters
-
painter The painter to use. dirtyRect the rectangle that actually needs repainting.
Definition at line 739 of file MarbleMap.cpp.
|
signal |
This signal is emit when the settings of a plugin changed.
int Marble::MarbleMap::preferredRadiusCeil | ( | int | radius | ) |
Definition at line 382 of file MarbleMap.cpp.
int Marble::MarbleMap::preferredRadiusFloor | ( | int | radius | ) |
Definition at line 391 of file MarbleMap.cpp.
Projection Marble::MarbleMap::projection | ( | ) | const |
Get the Projection used for the map.
- Returns
Spherical
a Globe-
Equirectangular
a flat map -
Mercator
another flat map
Definition at line 660 of file MarbleMap.cpp.
|
signal |
bool Marble::MarbleMap::propertyValue | ( | const QString & | name | ) | const |
Return the property value by name.
- Returns
- The property value (usually: visibility).
Definition at line 496 of file MarbleMap.cpp.
int Marble::MarbleMap::radius | ( | ) | const |
Return the radius of the globe in pixels.
Definition at line 364 of file MarbleMap.cpp.
|
signal |
|
slot |
Reload the currently displayed map by reloading texture tiles from the Internet.
In the future this should be extended to all kinds of data which is used in the map.
Definition at line 443 of file MarbleMap.cpp.
void Marble::MarbleMap::removeLayer | ( | LayerInterface * | layer | ) |
Remove a layer from being included in rendering.
Definition at line 1242 of file MarbleMap.cpp.
|
signal |
Signal that a render item has been initialized.
QList< RenderPlugin * > Marble::MarbleMap::renderPlugins | ( | ) | const |
Returns a list of all RenderPlugins in the model, this includes float items.
- Returns
- the list of RenderPlugins
Definition at line 1206 of file MarbleMap.cpp.
RenderState Marble::MarbleMap::renderState | ( | ) | const |
Definition at line 1252 of file MarbleMap.cpp.
|
signal |
RenderStatus Marble::MarbleMap::renderStatus | ( | ) | const |
Definition at line 1247 of file MarbleMap.cpp.
|
signal |
Emitted when the layer rendering status has changed.
- Parameters
-
status New render status
This signal is emitted when the repaint of the view was requested.
If available with the dirtyRegion
which is the region the view will change in. If dirtyRegion.isEmpty() returns true, the whole viewport has to be repainted.
|
slot |
Rotate the view by the two angles phi and theta.
- Parameters
-
deltaLon an angle that specifies the change in terms of longitude deltaLat an angle that specifies the change in terms of latitude
This function rotates the view by two angles, deltaLon ("theta") and deltaLat ("phi"). If we start at (0, 0), the result will be the exact equivalent of (lon, lat), otherwise the resulting angle will be the sum of the previous position and the two offsets.
Definition at line 636 of file MarbleMap.cpp.
bool Marble::MarbleMap::screenCoordinates | ( | qreal | lon, |
qreal | lat, | ||
qreal & | x, | ||
qreal & | y | ||
) | const |
Get the screen coordinates corresponding to geographical coordinates in the map.
- Parameters
-
lon the lon coordinate of the requested pixel position lat the lat coordinate of the requested pixel position x the x coordinate of the pixel is returned through this parameter y the y coordinate of the pixel is returned through this parameter
- Returns
true
if the geographical coordinates are visible on the screenfalse
if the geographical coordinates are not visible on the screen
Definition at line 680 of file MarbleMap.cpp.
|
slot |
Set the latitude for the center point.
- Parameters
-
lat the new value for the latitude in degree
Definition at line 650 of file MarbleMap.cpp.
|
slot |
Set the longitude for the center point.
- Parameters
-
lon the new value for the longitude in degree
Definition at line 655 of file MarbleMap.cpp.
|
slot |
Definition at line 1182 of file MarbleMap.cpp.
|
slot |
Definition at line 1200 of file MarbleMap.cpp.
|
slot |
Set the globe locked to the sub solar point.
- Parameters
-
vsible if globe is locked to the sub solar point
Definition at line 1050 of file MarbleMap.cpp.
void Marble::MarbleMap::setMapQualityForViewContext | ( | MapQuality | qualityForViewContext, |
ViewContext | viewContext | ||
) |
Definition at line 299 of file MarbleMap.cpp.
|
slot |
Set a new map theme.
- Parameters
-
maptheme The ID of the new maptheme. To ensure that a unique identifier is being used the theme does NOT get represented by its name but the by relative location of the file that specifies the theme:
Example: maptheme = "earth/bluemarble/bluemarble.dgml"
Definition at line 782 of file MarbleMap.cpp.
|
slot |
Set the Projection used for the map.
- Parameters
-
projection projection type (e.g. Spherical, Equirectangular, Mercator)
Definition at line 665 of file MarbleMap.cpp.
|
slot |
Sets the value of a map theme property.
- Parameters
-
value value of the property (usually: visibility)
Later on we might add a "setPropertyType and a QVariant if needed.
Definition at line 982 of file MarbleMap.cpp.
|
slot |
Set the radius of the globe in pixels.
- Parameters
-
radius The new globe radius value in pixels.
Definition at line 369 of file MarbleMap.cpp.
|
slot |
Set whether the atmospheric glow is visible.
- Parameters
-
visible visibility of the atmospheric glow
Definition at line 1009 of file MarbleMap.cpp.
|
slot |
Definition at line 1141 of file MarbleMap.cpp.
|
slot |
Set whether the borders visible.
- Parameters
-
visible visibility of the borders
Definition at line 1116 of file MarbleMap.cpp.
|
slot |
Set whether the city place mark overlay is visible.
- Parameters
-
visible visibility of the city place marks
Definition at line 1091 of file MarbleMap.cpp.
|
slot |
Set whether city lights instead of night shadow are visible.
- Parameters
-
visible visibility of city lights
Definition at line 1044 of file MarbleMap.cpp.
|
slot |
Set whether the cloud cover is visible.
- Parameters
-
visible visibility of the cloud cover
Definition at line 1032 of file MarbleMap.cpp.
|
slot |
Set whether the compass overlay is visible.
- Parameters
-
visible visibility of the compass
Definition at line 1004 of file MarbleMap.cpp.
|
slot |
Set whether the crosshairs are visible.
- Parameters
-
visible visibility of the crosshairs
Definition at line 1020 of file MarbleMap.cpp.
|
slot |
Set whether the frame rate gets shown.
- Parameters
-
visible visibility of the frame rate
Definition at line 1131 of file MarbleMap.cpp.
|
slot |
Set whether the coordinate grid overlay is visible.
- Parameters
-
visible visibility of the coordinate grid
Definition at line 1081 of file MarbleMap.cpp.
|
slot |
Set whether the ice layer is visible.
- Parameters
-
visible visibility of the ice layer
Definition at line 1111 of file MarbleMap.cpp.
|
slot |
Set whether the lakes are visible.
- Parameters
-
visible visibility of the lakes
Definition at line 1126 of file MarbleMap.cpp.
|
slot |
Set whether the other places overlay is visible.
- Parameters
-
visible visibility of other places
Definition at line 1101 of file MarbleMap.cpp.
|
slot |
Set whether the overview map overlay is visible.
- Parameters
-
visible visibility of the overview map
Definition at line 994 of file MarbleMap.cpp.
|
slot |
Set whether the place mark overlay is visible.
- Parameters
-
visible visibility of the place marks
Definition at line 1086 of file MarbleMap.cpp.
|
slot |
Set whether the relief is visible.
- Parameters
-
visible visibility of the relief
Definition at line 1106 of file MarbleMap.cpp.
|
slot |
Set whether the rivers are visible.
- Parameters
-
visible visibility of the rivers
Definition at line 1121 of file MarbleMap.cpp.
|
slot |
Definition at line 1136 of file MarbleMap.cpp.
|
slot |
Set whether the scale bar overlay is visible.
- Parameters
-
visible visibility of the scale bar
Definition at line 999 of file MarbleMap.cpp.
|
slot |
Set whether the night shadow is visible.
- Parameters
-
visibile visibility of shadow
Definition at line 1039 of file MarbleMap.cpp.
|
slot |
Set whether the terrain place mark overlay is visible.
- Parameters
-
visible visibility of the terrain place marks
Definition at line 1096 of file MarbleMap.cpp.
|
slot |
Set whether the is tile is visible NOTE: This is part of the transitional debug API and might be subject to changes until Marble 0.8.
- Parameters
-
visible visibility of the tile
Definition at line 1076 of file MarbleMap.cpp.
void Marble::MarbleMap::setSize | ( | int | width, |
int | height | ||
) |
Definition at line 337 of file MarbleMap.cpp.
void Marble::MarbleMap::setSize | ( | const QSize & | size | ) |
Definition at line 342 of file MarbleMap.cpp.
|
slot |
Set whether the sun icon is shown in the sub solar point.
- Parameters
-
visible if the sun icon is shown in the sub solar point
Definition at line 1069 of file MarbleMap.cpp.
void Marble::MarbleMap::setViewContext | ( | ViewContext | viewContext | ) |
Definition at line 317 of file MarbleMap.cpp.
|
slot |
Set the limit of the volatile (in RAM) tile cache.
- Parameters
-
bytes The limit in kilobytes.
Definition at line 1165 of file MarbleMap.cpp.
bool Marble::MarbleMap::showAtmosphere | ( | ) | const |
Return whether the atmospheric glow is visible.
- Returns
- The cloud cover visibility.
Definition at line 554 of file MarbleMap.cpp.
bool Marble::MarbleMap::showBackground | ( | ) | const |
Definition at line 625 of file MarbleMap.cpp.
bool Marble::MarbleMap::showBorders | ( | ) | const |
Return whether the borders are visible.
- Returns
- The border visibility.
Definition at line 605 of file MarbleMap.cpp.
bool Marble::MarbleMap::showCities | ( | ) | const |
Return whether the city place marks are visible.
- Returns
- The city place mark visibility.
Definition at line 580 of file MarbleMap.cpp.
bool Marble::MarbleMap::showCityLights | ( | ) | const |
Return whether the city lights are shown instead of the night shadow.
- Returns
- visibility of city lights
Definition at line 539 of file MarbleMap.cpp.
bool Marble::MarbleMap::showClouds | ( | ) | const |
Return whether the cloud cover is visible.
- Returns
- The cloud cover visibility.
Definition at line 529 of file MarbleMap.cpp.
bool Marble::MarbleMap::showCompass | ( | ) | const |
Return whether the compass bar is visible.
- Returns
- The compass visibility.
Definition at line 519 of file MarbleMap.cpp.
bool Marble::MarbleMap::showCrosshairs | ( | ) | const |
Return whether the crosshairs are visible.
- Returns
- The crosshairs' visibility.
Definition at line 559 of file MarbleMap.cpp.
bool Marble::MarbleMap::showFrameRate | ( | ) | const |
Return whether the frame rate gets displayed.
- Returns
- the frame rates visibility
Definition at line 620 of file MarbleMap.cpp.
bool Marble::MarbleMap::showGrid | ( | ) | const |
Return whether the coordinate grid is visible.
- Returns
- The coordinate grid visibility.
Definition at line 524 of file MarbleMap.cpp.
bool Marble::MarbleMap::showIceLayer | ( | ) | const |
Return whether the ice layer is visible.
- Returns
- The ice layer visibility.
Definition at line 600 of file MarbleMap.cpp.
bool Marble::MarbleMap::showLakes | ( | ) | const |
Return whether the lakes are visible.
- Returns
- The lakes' visibility.
Definition at line 615 of file MarbleMap.cpp.
bool Marble::MarbleMap::showOtherPlaces | ( | ) | const |
Return whether other places are visible.
- Returns
- The visibility of other places.
Definition at line 590 of file MarbleMap.cpp.
bool Marble::MarbleMap::showOverviewMap | ( | ) | const |
Return whether the overview map is visible.
- Returns
- The overview map visibility.
Definition at line 509 of file MarbleMap.cpp.
bool Marble::MarbleMap::showPlaces | ( | ) | const |
Return whether the place marks are visible.
- Returns
- The place mark visibility.
Definition at line 575 of file MarbleMap.cpp.
bool Marble::MarbleMap::showRelief | ( | ) | const |
Return whether the relief is visible.
- Returns
- The relief visibility.
Definition at line 595 of file MarbleMap.cpp.
bool Marble::MarbleMap::showRivers | ( | ) | const |
Return whether the rivers are visible.
- Returns
- The rivers' visibility.
Definition at line 610 of file MarbleMap.cpp.
bool Marble::MarbleMap::showScaleBar | ( | ) | const |
Return whether the scale bar is visible.
- Returns
- The scale bar visibility.
Definition at line 514 of file MarbleMap.cpp.
bool Marble::MarbleMap::showSunShading | ( | ) | const |
Return whether the night shadow is visible.
- Returns
- visibility of night shadow
Definition at line 534 of file MarbleMap.cpp.
bool Marble::MarbleMap::showTerrain | ( | ) | const |
Return whether the terrain place marks are visible.
- Returns
- The terrain place mark visibility.
Definition at line 585 of file MarbleMap.cpp.
QSize Marble::MarbleMap::size | ( | ) | const |
Definition at line 349 of file MarbleMap.cpp.
TextureLayer * Marble::MarbleMap::textureLayer | ( | ) | const |
Definition at line 1258 of file MarbleMap.cpp.
|
signal |
Signal that the theme has changed.
- Parameters
-
theme Name of the new theme.
|
signal |
int Marble::MarbleMap::tileZoomLevel | ( | ) | const |
Definition at line 400 of file MarbleMap.cpp.
ViewContext Marble::MarbleMap::viewContext | ( | ) | const |
Definition at line 331 of file MarbleMap.cpp.
ViewportParams * Marble::MarbleMap::viewport | ( | ) |
Definition at line 288 of file MarbleMap.cpp.
const ViewportParams * Marble::MarbleMap::viewport | ( | ) | const |
Definition at line 293 of file MarbleMap.cpp.
|
signal |
This signal is emitted when the visible region of the map changes.
This typically happens when the user moves the map around or zooms.
quint64 Marble::MarbleMap::volatileTileCacheLimit | ( | ) | const |
Returns the limit in kilobytes of the volatile (in RAM) tile cache.
- Returns
- the limit of volatile tile cache in kilobytes.
Definition at line 630 of file MarbleMap.cpp.
QVector< const GeoDataPlacemark * > Marble::MarbleMap::whichFeatureAt | ( | const QPoint & | curpos | ) | const |
Definition at line 438 of file MarbleMap.cpp.
QList< AbstractDataPluginItem * > Marble::MarbleMap::whichItemAt | ( | const QPoint & | curpos | ) | const |
Returns all widgets of dataPlugins on the position curpos.
Definition at line 1232 of file MarbleMap.cpp.
int Marble::MarbleMap::width | ( | ) | const |
Definition at line 354 of file MarbleMap.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:13:45 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.