marble
#include <RenderPluginModel.h>
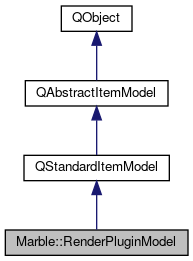
Public Types | |
enum | ItemDataRole { Name = Qt::DisplayRole, Icon = Qt::DecorationRole, Description = Qt::ToolTipRole, NameId = Qt::UserRole + 2, ConfigurationDialogAvailable, BackendTypes, Version, AboutDataText, CopyrightYears } |
Public Slots | |
void | applyPluginState () |
void | retrievePluginState () |
Public Member Functions | |
RenderPluginModel (QObject *parent=0) | |
~RenderPluginModel () | |
QList< PluginAuthor > | pluginAuthors (const QModelIndex &index) const |
DialogConfigurationInterface * | pluginDialogConfigurationInterface (const QModelIndex &index) |
void | setRenderPlugins (const QList< RenderPlugin * > &renderPlugins) |
![]() | |
QStandardItemModel (QObject *parent) | |
QStandardItemModel (int rows, int columns, QObject *parent) | |
~QStandardItemModel () | |
void | appendColumn (const QList< QStandardItem * > &items) |
void | appendRow (const QList< QStandardItem * > &items) |
void | appendRow (QStandardItem *item) |
void | clear () |
virtual int | columnCount (const QModelIndex &parent) const |
virtual QVariant | data (const QModelIndex &index, int role) const |
virtual bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) |
QList< QStandardItem * > | findItems (const QString &text, QFlags< Qt::MatchFlag > flags, int column) const |
virtual Qt::ItemFlags | flags (const QModelIndex &index) const |
virtual bool | hasChildren (const QModelIndex &parent) const |
virtual QVariant | headerData (int section, Qt::Orientation orientation, int role) const |
QStandardItem * | horizontalHeaderItem (int column) const |
virtual QModelIndex | index (int row, int column, const QModelIndex &parent) const |
QModelIndex | indexFromItem (const QStandardItem *item) const |
void | insertColumn (int column, const QList< QStandardItem * > &items) |
bool | insertColumn (int column, const QModelIndex &parent) |
virtual bool | insertColumns (int column, int count, const QModelIndex &parent) |
void | insertRow (int row, const QList< QStandardItem * > &items) |
void | insertRow (int row, QStandardItem *item) |
bool | insertRow (int row, const QModelIndex &parent) |
virtual bool | insertRows (int row, int count, const QModelIndex &parent) |
QStandardItem * | invisibleRootItem () const |
QStandardItem * | item (int row, int column) const |
void | itemChanged (QStandardItem *item) |
virtual QMap< int, QVariant > | itemData (const QModelIndex &index) const |
QStandardItem * | itemFromIndex (const QModelIndex &index) const |
const QStandardItem * | itemPrototype () const |
virtual QMimeData * | mimeData (const QModelIndexList &indexes) const |
virtual QStringList | mimeTypes () const |
virtual QModelIndex | parent (const QModelIndex &child) const |
virtual bool | removeColumns (int column, int count, const QModelIndex &parent) |
virtual bool | removeRows (int row, int count, const QModelIndex &parent) |
virtual int | rowCount (const QModelIndex &parent) const |
void | setColumnCount (int columns) |
virtual bool | setData (const QModelIndex &index, const QVariant &value, int role) |
virtual bool | setHeaderData (int section, Qt::Orientation orientation, const QVariant &value, int role) |
void | setHorizontalHeaderItem (int column, QStandardItem *item) |
void | setHorizontalHeaderLabels (const QStringList &labels) |
void | setItem (int row, QStandardItem *item) |
void | setItem (int row, int column, QStandardItem *item) |
virtual bool | setItemData (const QModelIndex &index, const QMap< int, QVariant > &roles) |
void | setItemPrototype (const QStandardItem *item) |
void | setRowCount (int rows) |
void | setSortRole (int role) |
void | setVerticalHeaderItem (int row, QStandardItem *item) |
void | setVerticalHeaderLabels (const QStringList &labels) |
virtual void | sort (int column, Qt::SortOrder order) |
int | sortRole () const |
virtual Qt::DropActions | supportedDropActions () const |
QList< QStandardItem * > | takeColumn (int column) |
QStandardItem * | takeHorizontalHeaderItem (int column) |
QStandardItem * | takeItem (int row, int column) |
QList< QStandardItem * > | takeRow (int row) |
QStandardItem * | takeVerticalHeaderItem (int row) |
QStandardItem * | verticalHeaderItem (int row) const |
![]() | |
QAbstractItemModel (QObject *parent) | |
virtual | ~QAbstractItemModel () |
virtual QModelIndex | buddy (const QModelIndex &index) const |
virtual bool | canFetchMore (const QModelIndex &parent) const |
virtual int | columnCount (const QModelIndex &parent) const =0 |
void | columnsAboutToBeInserted (const QModelIndex &parent, int start, int end) |
void | columnsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsAboutToBeRemoved (const QModelIndex &parent, int start, int end) |
void | columnsInserted (const QModelIndex &parent, int start, int end) |
void | columnsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsRemoved (const QModelIndex &parent, int start, int end) |
virtual QVariant | data (const QModelIndex &index, int role) const =0 |
void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight) |
virtual void | fetchMore (const QModelIndex &parent) |
bool | hasIndex (int row, int column, const QModelIndex &parent) const |
void | headerDataChanged (Qt::Orientation orientation, int first, int last) |
virtual QModelIndex | index (int row, int column, const QModelIndex &parent) const =0 |
bool | insertColumn (int column, const QModelIndex &parent) |
bool | insertRow (int row, const QModelIndex &parent) |
void | layoutAboutToBeChanged () |
void | layoutChanged () |
virtual QModelIndexList | match (const QModelIndex &start, int role, const QVariant &value, int hits, QFlags< Qt::MatchFlag > flags) const |
void | modelAboutToBeReset () |
void | modelReset () |
virtual QModelIndex | parent (const QModelIndex &index) const =0 |
bool | removeColumn (int column, const QModelIndex &parent) |
bool | removeRow (int row, const QModelIndex &parent) |
virtual void | revert () |
const QHash< int, QByteArray > & | roleNames () const |
virtual int | rowCount (const QModelIndex &parent) const =0 |
void | rowsAboutToBeInserted (const QModelIndex &parent, int start, int end) |
void | rowsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsAboutToBeRemoved (const QModelIndex &parent, int start, int end) |
void | rowsInserted (const QModelIndex &parent, int start, int end) |
void | rowsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsRemoved (const QModelIndex &parent, int start, int end) |
void | setSupportedDragActions (QFlags< Qt::DropAction > actions) |
QModelIndex | sibling (int row, int column, const QModelIndex &index) const |
virtual QSize | span (const QModelIndex &index) const |
virtual bool | submit () |
Qt::DropActions | supportedDragActions () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
void | beginInsertColumns (const QModelIndex &parent, int first, int last) |
void | beginInsertRows (const QModelIndex &parent, int first, int last) |
bool | beginMoveColumns (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
bool | beginMoveRows (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
void | beginRemoveColumns (const QModelIndex &parent, int first, int last) |
void | beginRemoveRows (const QModelIndex &parent, int first, int last) |
void | beginResetModel () |
void | changePersistentIndex (const QModelIndex &from, const QModelIndex &to) |
void | changePersistentIndexList (const QModelIndexList &from, const QModelIndexList &to) |
QModelIndex | createIndex (int row, int column, void *ptr) const |
QModelIndex | createIndex (int row, int column, int id) const |
QModelIndex | createIndex (int row, int column, quint32 id) const |
void | endInsertColumns () |
void | endInsertRows () |
void | endMoveColumns () |
void | endMoveRows () |
void | endRemoveColumns () |
void | endRemoveRows () |
void | endResetModel () |
QModelIndexList | persistentIndexList () const |
void | reset () |
void | resetInternalData () |
void | setRoleNames (const QHash< int, QByteArray > &roleNames) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
sortRole | |
![]() | |
objectName | |
Detailed Description
Provides common access to various kinds of plugins without having to know about their details.
Definition at line 30 of file RenderPluginModel.h.
Member Enumeration Documentation
This enum contains the data roles for the QStandardItems.
Enumerator | |
---|---|
Name | |
Icon | |
Description | |
NameId | |
ConfigurationDialogAvailable | |
BackendTypes | |
Version | |
AboutDataText | |
CopyrightYears |
Definition at line 38 of file RenderPluginModel.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 38 of file RenderPluginModel.cpp.
Marble::RenderPluginModel::~RenderPluginModel | ( | ) |
Definition at line 44 of file RenderPluginModel.cpp.
Member Function Documentation
|
slot |
Apply the plugin state from the user interface.
Definition at line 100 of file RenderPluginModel.cpp.
QList< PluginAuthor > Marble::RenderPluginModel::pluginAuthors | ( | const QModelIndex & | index | ) | const |
Definition at line 70 of file RenderPluginModel.cpp.
DialogConfigurationInterface * Marble::RenderPluginModel::pluginDialogConfigurationInterface | ( | const QModelIndex & | index | ) |
Definition at line 81 of file RenderPluginModel.cpp.
|
slot |
Retrieve the current plugin state for the user interface.
Definition at line 93 of file RenderPluginModel.cpp.
void Marble::RenderPluginModel::setRenderPlugins | ( | const QList< RenderPlugin * > & | renderPlugins | ) |
Set the RenderPlugins the model should manage.
The given plugins must not be deleted as long as the model has a hold on them, i.e. until the model is deleted or a different set of plugins is assigned.
- Parameters
-
renderPlugins the RenderPlugins to be managed
Definition at line 54 of file RenderPluginModel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:13:46 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.