parley
#include <document.h>
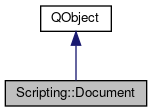
Public Types | |
enum | ErrorCode { NoError = 0, Unknown, InvalidXml, FileTypeUnknown, FileCannotWrite, FileWriterFailed, FileCannotRead, FileReaderFailed, FileDoesNotExist } |
enum | FileDialogMode { Reading, Writing } |
enum | FileType { KvdNone, Automatic, Kvtml, Wql, Pauker, Vokabeln, Xdxf, Csv, Kvtml1 } |
enum | LessonDeletion { DeleteEmptyLesson, DeleteEntriesAndLesson } |
Public Slots | |
QVariantList | allLessons () |
int | appendIdentifier (Identifier *identifier) |
void | appendLesson (QObject *lesson) |
void | appendNewIdentifier (const QString &name, const QString &locale) |
QObject * | appendNewLesson (const QString &name) |
QObject * | appendNewLesson (const QString &name, Lesson *parent) |
static KEduVocDocument::FileType | detectFileType (const QString &fileName) |
static QString | errorDescription (int errorCode) |
QObject * | findLesson (const QString &name) |
QObject * | identifier (int index) |
int | identifierCount () const |
QVariantList | identifiers () |
void | merge (Document *docToMerge, bool matchIdentifiers) |
QObject * | newIdentifier () |
QObject * | newLesson (const QString &name) |
static QString | pattern (KEduVocDocument::FileDialogMode mode) |
void | removeIdentifier (int index) |
int | saveAs (const QString &url, KEduVocDocument::FileType ft=KEduVocDocument::Automatic, const QString &generator=QString("Parley")) |
void | setWordType (QObject *tr, const QString &wordtype) |
QStringList | wordTypes () |
Public Member Functions | |
Document (QObject *parent=0) | |
Document (KEduVocDocument *doc) | |
~Document () | |
QString | author () const |
QString | authorContact () const |
QString | category () const |
QString | csvDelimiter () const |
QString | documentComment () const |
QString | generator () const |
KEduVocDocument * | kEduVocDocument () |
QString | license () const |
QObject * | rootLesson () |
void | setAuthor (const QString &author) |
void | setAuthorContact (const QString &authorContact) |
void | setCategory (const QString &category) |
void | setCsvDelimiter (const QString &delimiter) |
void | setDocumentComment (const QString &comment) |
void | setGenerator (const QString &generator) |
void | setLicense (const QString &license) |
void | setTitle (const QString &title) |
void | setUrl (const QString &url) |
void | setVersion (const QString &ver) |
QString | title () const |
QString | url () const |
QString | version () const |
KEduVocWordType * | wordTypeFromString (const QString &name) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Properties | |
QString | author |
QString | authorContact |
QString | category |
QString | csvDelimiter |
QString | documentComment |
QString | generator |
QString | license |
QObject | rootLesson |
QString | title |
QString | url |
QString | version |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
KEduVocDocument wrapping class for Kross scripts.
The Document class provides methods and properties for accessing the document lessons, entries, languages, word types and general document parameters. The easiest way of accessing this class is through the Parley.document (Parley.doc) property like in the example.
You can access document lessons with the following ways:
- Document::rootLesson property
- allLessons() function
- findLesson() function
and add a new lesson with
- newLesson() and appendLesson() function
- Document::appendNewLesson(const QString &) function
- Document::appendNewLesson(const QString &,Lesson*) function
The document identifiers (see Identifier class) can be set by these functions:
- newIdentifier() and appendIdentifier() functions
- appendNewIdentifier() function
- removeIdentifier() function (for removing a language)
and be accessed by:
- as a list with identifiers() function
- individually by identifier() function
- and get how many identifiers exist with identifierCount() function
The saveAs() function can be used to save into a file a newly created document, or the active document. See Parley::newDocument().
Definition at line 66 of file document.h.
Member Enumeration Documentation
the return code when opening/saving
Enumerator | |
---|---|
NoError | |
Unknown | |
InvalidXml | |
FileTypeUnknown | |
FileCannotWrite | |
FileWriterFailed | |
FileCannotRead | |
FileReaderFailed | |
FileDoesNotExist |
Definition at line 109 of file document.h.
known vocabulary file types
Enumerator | |
---|---|
KvdNone | |
Automatic | |
Kvtml | |
Wql | |
Pauker | |
Vokabeln | |
Xdxf | |
Csv | |
Kvtml1 |
Definition at line 96 of file document.h.
delete only empty lessons or also if they have entries
Enumerator | |
---|---|
DeleteEmptyLesson | |
DeleteEntriesAndLesson |
Definition at line 128 of file document.h.
Constructor & Destructor Documentation
Scripting::Document::Document | ( | QObject * | parent = 0 | ) |
Definition at line 25 of file document.cpp.
Scripting::Document::Document | ( | KEduVocDocument * | doc | ) |
Definition at line 30 of file document.cpp.
Scripting::Document::~Document | ( | ) |
Definition at line 35 of file document.cpp.
Member Function Documentation
|
slot |
Returns all the lessons in the document (including sublessons)
- Returns
- A list of all the lessons in the document
Definition at line 39 of file document.cpp.
|
inlineslot |
Appends a new identifier (usually a language)
- Parameters
-
identifier the identifier to append. If empty default names are used.
- Returns
- the identifier number
Definition at line 449 of file document.h.
|
slot |
Appends a lesson to the document.
- Parameters
-
lesson Lesson object (the lesson to be added)
Definition at line 104 of file document.cpp.
Append a new identifier by giving the name
and locale
.
- Parameters
-
name Language description ex. "American English" locale Language locale ex. "en_US"
Definition at line 96 of file document.cpp.
Creates a new lesson and appends it to the root lesson.
- Parameters
-
name Lesson name
- Returns
- A reference to the new lesson
Definition at line 111 of file document.cpp.
Creates a new lesson and appends it to the parent
lesson.
- Parameters
-
name Lesson name parent Parent lesson
- Returns
- A reference to the new lesson
Definition at line 118 of file document.cpp.
|
inline |
Definition at line 170 of file document.h.
|
inline |
Definition at line 181 of file document.h.
|
inline |
Definition at line 214 of file document.h.
|
inline |
Definition at line 247 of file document.h.
|
inlinestaticslot |
Definition at line 527 of file document.h.
|
inline |
Definition at line 203 of file document.h.
|
inlinestaticslot |
Returns a more detailed description of the errorCode
given.
Definition at line 544 of file document.h.
Searches through all the lessons (recursively) and returns the first lesson the specified name
.
- Parameters
-
name Name of the lesson to look for
- Returns
- A reference to a lesson if found. 0 otherwise
Definition at line 125 of file document.cpp.
|
inline |
Definition at line 226 of file document.h.
|
inlineslot |
Returns the identifier of translation index
.
- Parameters
-
index number of translation 0..x
- Returns
- the language identifier: en=english, de=german, ...
Definition at line 467 of file document.h.
|
inlineslot |
- Returns
- the number of different identifiers (usually languages)
Definition at line 424 of file document.h.
|
slot |
Returns a list of all the identifiers of this document.
Definition at line 86 of file document.cpp.
|
inline |
Definition at line 139 of file document.h.
|
inline |
Definition at line 192 of file document.h.
|
inlineslot |
Merges data from another document.
- Parameters
-
docToMerge document containing the data to be merged matchIdentifiers if true only entries having identifiers present in the current document will be mergedurl is empty (or NULL) actual name is preserved
Definition at line 404 of file document.h.
|
inlineslot |
Creates a new identifier and returns a reference to it.
- Returns
Definition at line 432 of file document.h.
Creates and returns a new lesson (doesn't add it as a sublesson to any lesson)
- Parameters
-
name Name of the lesson
- Returns
- Lesson object (the new lesson)
Definition at line 287 of file document.h.
|
inlinestaticslot |
Create a string with the supported document types, that can be used as filter in KFileDialog.
It includes also an entry to match all the supported types.
- Parameters
-
mode the mode for the supported document types. See FileDialogMode enum
- Returns
- the filter string
Definition at line 539 of file document.h.
|
inlineslot |
Removes identifier and the according translations in all entries.
- Parameters
-
index number of translation 0..x
Definition at line 476 of file document.h.
|
inline |
Definition at line 144 of file document.h.
|
inlineslot |
Saves the data under the given name.
- Parameters
-
url if url is empty (or NULL) actual name is preserved ft the filetype to be used when saving the document (default value: Automatic). See enum FileType. generator the name of the application saving the document (default value: "Parley")
- Returns
- ErrorCode
Definition at line 391 of file document.h.
|
inline |
Definition at line 165 of file document.h.
|
inline |
Definition at line 176 of file document.h.
|
inline |
Definition at line 209 of file document.h.
|
inline |
Definition at line 256 of file document.h.
|
inline |
Definition at line 198 of file document.h.
|
inline |
Definition at line 221 of file document.h.
|
inline |
Definition at line 187 of file document.h.
|
inline |
Definition at line 154 of file document.h.
|
inline |
Definition at line 263 of file document.h.
|
inline |
Definition at line 232 of file document.h.
Sets the word type (wordtype
) of the given tr
translation object.
If the wordtype
is not valid, no changes are made to the translation object
- Parameters
-
tr Translation object to set it's word type wordtype Word type name
Definition at line 61 of file document.cpp.
|
inline |
Definition at line 159 of file document.h.
|
inline |
Definition at line 268 of file document.h.
|
inline |
Definition at line 237 of file document.h.
KEduVocWordType * Scripting::Document::wordTypeFromString | ( | const QString & | name | ) |
Definition at line 45 of file document.cpp.
|
slot |
Returns a string list with all the available word type's names.
Definition at line 75 of file document.cpp.
Property Documentation
|
readwrite |
Document author's name.
Definition at line 74 of file document.h.
|
readwrite |
Author contact info (email)
Definition at line 76 of file document.h.
|
readwrite |
Category that the lesson belongs.
Definition at line 82 of file document.h.
|
readwrite |
Delimiter (separator) used for csv import and export.
Definition at line 88 of file document.h.
|
readwrite |
Comment about the document.
Definition at line 80 of file document.h.
|
readwrite |
Generator program (which program generates this file)
Definition at line 84 of file document.h.
|
readwrite |
Document license.
Definition at line 78 of file document.h.
|
read |
Document's root lesson.
Definition at line 70 of file document.h.
|
readwrite |
Document's title.
Definition at line 72 of file document.h.
|
readwrite |
URL of the XML file.
Definition at line 90 of file document.h.
|
readwrite |
Document version.
Definition at line 86 of file document.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:15:57 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.