rocs/RocsCore
#include <Document.h>
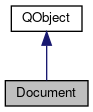
Public Slots | |
DataStructurePtr | activeDataStructure () const |
DataStructurePtr | addDataStructure (const QString &name=QString()) |
DataStructurePtr | addDataStructure (DataStructurePtr dataStructure) |
void | setActiveDataStructure (int index) |
void | setActiveDataStructure (DataStructurePtr g) |
void | setModified (const bool mod=true) |
void | updateGraphics (DataTypePtr dataType) |
void | updateGraphics (PointerTypePtr pointerType) |
void | updateSceneRect (const QPointF &position) |
Signals | |
void | activeDataStructureChanged (DataStructurePtr g) |
void | dataStructureCreated (DataStructurePtr g) |
void | dataStructureListChanged () |
void | dataTypeCreated (int identifier) |
void | dataTypeRemoved (int identifier) |
void | nameChanged (QString name) |
void | pointerTypeCreated (int identifier) |
void | pointerTypeRemoved (int identifier) |
void | sceneRectChanged (const QRectF &) |
Public Member Functions | |
Document (const QString &name, QObject *parent=0) | |
~Document () | |
DataStructureBackendInterface * | backend () const |
void | changeBackend () |
virtual void | cleanUpBeforeConvert () |
void | clear () |
QList< DataStructurePtr > & | dataStructures () const |
DataTypePtr | dataType (int dataType) const |
QList< int > | dataTypeList () const |
QtScriptBackend * | engineBackend () const |
QString | fileUrl () const |
int | groupType () |
QString | iconPackage () const |
bool | isModified () const |
QString | name () const |
PointerTypePtr | pointerType (int pointerType) const |
QList< int > | pointerTypeList () const |
int | registerDataType (const QString &name, int identifier=0) |
int | registerPointerType (const QString &name, int identifier=0) |
void | remove (DataStructurePtr dataStructure) |
bool | removeDataType (int dataType) |
bool | removePointerType (int pointerType) |
void | save () |
void | saveAs (const QString &fileUrl) |
QRectF | sceneRect () const |
void | setBackend (const QString &pluginIdentifier) |
void | setFileUrl (const KUrl &fileUrl) |
void | setName (const QString &name) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static bool | isValidIdentifier (const QString &identifier) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Definition at line 41 of file Document.h.
Constructor & Destructor Documentation
Definition at line 75 of file Document.cpp.
Document::~Document | ( | ) |
Definition at line 118 of file Document.cpp.
Member Function Documentation
|
slot |
- Returns
- the currently selected data structure, if none exists the shared pointer is invalid
Definition at line 431 of file Document.cpp.
|
signal |
|
slot |
Add data structure to graph document with name name
.
If no name is given, the name of the used data structure is used.
- Parameters
-
name is the optional name of the to be created data structure
- Returns
- the added data structure
Definition at line 333 of file Document.cpp.
|
slot |
Add data structure dataStructure
to the document.
- Parameters
-
dataStructure the data structure to be added to the document
- Returns
- the added data structure for argument chaining
Definition at line 342 of file Document.cpp.
DataStructureBackendInterface * Document::backend | ( | ) | const |
- Returns
- backend of this document
Definition at line 436 of file Document.cpp.
void Document::changeBackend | ( | ) |
Change data structure backend of this document to currently active backend.
- See also
- DataStructureBackend::activeBackend(). Changing the backend of a data structure is a LOSSY operation (converting between backends and converting back will result in data loss!)
Definition at line 101 of file Document.cpp.
|
virtual |
clear data that only is useful for a type of data structure and that cannot be converted to others from all data structeres of this document.
TODO this method should be protected
Definition at line 308 of file Document.cpp.
void Document::clear | ( | ) |
Remove everything contained at this document.
Definition at line 408 of file Document.cpp.
|
signal |
|
signal |
QList< DataStructurePtr > & Document::dataStructures | ( | ) | const |
Definition at line 227 of file Document.cpp.
DataTypePtr Document::dataType | ( | int | dataType | ) | const |
Definition at line 197 of file Document.cpp.
|
signal |
QList< int > Document::dataTypeList | ( | ) | const |
Getter for all registered data types.
- Returns
- list of all data type ids
Definition at line 160 of file Document.cpp.
|
signal |
QtScriptBackend * Document::engineBackend | ( | ) | const |
Definition at line 239 of file Document.cpp.
QString Document::fileUrl | ( | ) | const |
- Returns
- path used for saving
Definition at line 386 of file Document.cpp.
int Document::groupType | ( | ) |
Returns data type for element groups.
If type is not registered yet, it will be created.
Definition at line 217 of file Document.cpp.
QString Document::iconPackage | ( | ) | const |
Definition at line 170 of file Document.cpp.
bool Document::isModified | ( | ) | const |
Definition at line 303 of file Document.cpp.
|
static |
Evaluates given string and returns true if identifier is valid, otherwise returns false.
- Parameters
-
identifier the string to be tested if it is valid property identifier
Definition at line 232 of file Document.cpp.
QString Document::name | ( | ) | const |
Definition at line 253 of file Document.cpp.
|
signal |
PointerTypePtr Document::pointerType | ( | int | pointerType | ) | const |
Definition at line 207 of file Document.cpp.
|
signal |
QList< int > Document::pointerTypeList | ( | ) | const |
Getter for all registered pointer types.
- Returns
- list of all pointer type ids
Definition at line 165 of file Document.cpp.
|
signal |
int Document::registerDataType | ( | const QString & | name, |
int | identifier = 0 |
||
) |
Register new type for data elements.
If identifier is alreade in use or if no identifier is provided, a new identifier is created.
- Parameters
-
name of the dataType identifier is optional identifier for data type
- Returns
- positive integer >0 if successfully registered, else <=0
Definition at line 124 of file Document.cpp.
int Document::registerPointerType | ( | const QString & | name, |
int | identifier = 0 |
||
) |
Register new type for pointers.
If identifier is already in use or if no identifier is provided, a new identifier is created.
- Parameters
-
name of the pointerType
- Returns
- positive integer >0 if successfully registered, else <=0
Definition at line 142 of file Document.cpp.
void Document::remove | ( | DataStructurePtr | dataStructure | ) |
Definition at line 396 of file Document.cpp.
bool Document::removeDataType | ( | int | dataType | ) |
removes this data type and all data elements of this type
- Parameters
-
dataType is positive id>0
- Returns
- true if a dataType was removed
Definition at line 175 of file Document.cpp.
bool Document::removePointerType | ( | int | pointerType | ) |
Removes this pointer type and all data elements of this type.
Aborts and returns "false" if pointer type is "0" or if the pointertype does not exists.
- Parameters
-
pointerType is positive id>0
- Returns
- true if a dataType was removed
Definition at line 186 of file Document.cpp.
void Document::save | ( | ) |
Save graph document in former file.
It is expected that
- See also
- fileUrl() is not empty.
Definition at line 375 of file Document.cpp.
void Document::saveAs | ( | const QString & | fileUrl | ) |
Save graph document under the given fileUrl
.
Old file is not changed.
- Parameters
-
fileUrl path to local file to that document shall be saved
Definition at line 381 of file Document.cpp.
QRectF Document::sceneRect | ( | ) | const |
- Returns
- the size of document' (visual) area
Definition at line 272 of file Document.cpp.
|
signal |
|
slot |
Sets the active data structure of graph document with index index
in the data structure list.
To get list of indices
- See also
- dataStructures();
- Parameters
-
index is the index of the data structure in internal data structure list
Definition at line 315 of file Document.cpp.
|
slot |
Definition at line 324 of file Document.cpp.
void Document::setBackend | ( | const QString & | pluginIdentifier | ) |
Set data structure plugin for this document.
This function is only safe if the document is empty, otherwise behavior is undefined.
Definition at line 441 of file Document.cpp.
void Document::setFileUrl | ( | const KUrl & | fileUrl | ) |
Set file path used for saving.
- Parameters
-
fileUrl path to local file for saving the document
Definition at line 391 of file Document.cpp.
|
slot |
Definition at line 96 of file Document.cpp.
void Document::setName | ( | const QString & | name | ) |
Definition at line 245 of file Document.cpp.
|
slot |
Definition at line 258 of file Document.cpp.
|
slot |
Definition at line 265 of file Document.cpp.
|
slot |
Updates scene rect according to given position.
If position
lies outside of rect, the rect is increased. Otherwise, (currently) nothing is done.
- Parameters
-
position is the new position of the changed data element
Definition at line 277 of file Document.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.