rocs/RocsCore
#include <Pointer.h>
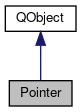
Public Slots | |
void | addDynamicProperty (const QString &property, const QVariant &value) |
QList< QString > | properties () const |
void | removeDynamicProperty (const QString &property) |
void | renameDynamicProperty (const QString &oldName, const QString &newName) |
void | setColor (const QColor &color) |
void | setPointerType (int pointerType) |
void | setVisible (bool visible) |
void | setWidth (qreal width) |
Qt::PenStyle | style () const |
QScriptValue | type () const |
void | updateDynamicProperty (const QString &property) |
void | updateRelativeIndex () |
Signals | |
void | changed () |
void | directionChanged (PointerType::Direction direction) |
void | pointerTypeChanged (int pointerType) |
void | posChanged () |
void | propertyAdded (const QString &name) |
void | propertyChanged (const QString &name) |
void | propertyRemoved (const QString &name) |
void | removed () |
Public Member Functions | |
virtual | ~Pointer () |
Q_INVOKABLE void | add_property (const QString &name, const QString &value) |
QColor | color () const |
DataStructurePtr | dataStructure () const |
PointerType::Direction | direction () const |
Q_INVOKABLE QScriptValue | end () const |
DataPtr | from () const |
PointerPtr | getPointer () const |
bool | isDirected () const |
bool | isVisible () const |
int | pointerType () const |
int | relativeIndex () const |
Q_INVOKABLE void | remove () |
Q_INVOKABLE void | remove_property (const QString &name) |
QScriptValue | scriptValue () const |
Q_INVOKABLE void | self_remove () |
Q_INVOKABLE QScriptValue | set_type (int type) |
void | setEngine (QScriptEngine *engine) |
Q_INVOKABLE QScriptValue | start () const |
DataPtr | to () const |
qreal | width () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static PointerPtr | create (DataStructurePtr dataStructure, DataPtr from, DataPtr to, int pointerType) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
Pointer (DataStructurePtr parent, DataPtr from, DataPtr to, int pointerType) | |
bool | eventFilter (QObject *obj, QEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Static Protected Member Functions | |
template<typename T > | |
static PointerPtr | create (DataStructurePtr parent, DataPtr from, DataPtr to, int pointerType) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Properties | |
QColor | color |
bool | directed |
QScriptValue | from |
QScriptValue | to |
int | type |
qreal | width |
![]() | |
objectName | |
Detailed Description
Pointers are connections between two nodes in the data structure.
They are usually unidirectional and hold several properties. Properties can accessed directly by the getter methods or (also from the script engine) be created by 'add_property(name, value)' and access by 'pointer.propertyName'.
Constructor & Destructor Documentation
|
virtual |
Default destructor.
DO NOT CALL IT, let the shared pointer take care for deletion.
Definition at line 117 of file Pointer.cpp.
|
protected |
Protected constructor.
Use static
- See also
- create() method.
- Parameters
-
parent the data structure the pointer belongs to from the first data element to the second data element pointerType the type of this pointer (default is 0)
Definition at line 99 of file Pointer.cpp.
Member Function Documentation
Add new property to pointer.
- Parameters
-
name is identifier for new property value is the initial value of the property
Definition at line 317 of file Pointer.cpp.
Add new dynamic property with identifier property
to this data element and sets it to value
.
- Parameters
-
property is the identifier for the new property value is the value of this new property
Definition at line 260 of file Pointer.cpp.
|
signal |
Emitted when a connected data element or the pointer is changed.
QColor Pointer::color | ( | ) | const |
- Returns
- the color of this pointer
|
static |
Create pointer objects.
- Parameters
-
dataStructure is data structure to that the new data element belongs from is source of pointer to is target of pointer pointerType is the type of the created pointer, it must be existing and registered
- Returns
- the created pointer element
Definition at line 53 of file Pointer.cpp.
|
inlinestaticprotected |
DataStructurePtr Pointer::dataStructure | ( | ) | const |
- Returns
- data structure to that this pointer belongs
Definition at line 140 of file Pointer.cpp.
PointerType::Direction Pointer::direction | ( | ) | const |
- Returns
- the direction of the pointer.
Definition at line 210 of file Pointer.cpp.
|
signal |
Emitted when direction was changed.
QScriptValue Pointer::end | ( | ) | const |
Reimplemented from QObject.
Definition at line 121 of file Pointer.cpp.
DataPtr Pointer::from | ( | ) | const |
- Returns
- source data element of this pointer
PointerPtr Pointer::getPointer | ( | ) | const |
- Returns
- shared pointer to this pointer element
Definition at line 93 of file Pointer.cpp.
bool Pointer::isDirected | ( | ) | const |
- Returns
- true if the pointer is directed, otherwise false
Definition at line 215 of file Pointer.cpp.
bool Pointer::isVisible | ( | ) | const |
- Returns
- true if the edge is visible, otherwise false.
Definition at line 226 of file Pointer.cpp.
int Pointer::pointerType | ( | ) | const |
- Returns
- pointer type identifier,
- See also
- class PointerType
Definition at line 155 of file Pointer.cpp.
|
signal |
Emitted when the pointer type of this pointer changes.
|
signal |
Emitted when the position of a connected data element changes.
Definition at line 179 of file Pointer.cpp.
|
signal |
|
signal |
|
signal |
int Pointer::relativeIndex | ( | ) | const |
The relative index is the index that this pointer has relative to the pair of data elements to which it is bound.
I.e., if its end points have have two or more pointers connecteds between them, the relative index gives a unique identifier for the pointer. Note that the relative index can change whenever pointers between its endpoints are created or removed.
- Returns
- the relative index identifier
Definition at line 131 of file Pointer.cpp.
void Pointer::remove | ( | ) |
Remove pointer.
Definition at line 184 of file Pointer.cpp.
void Pointer::remove_property | ( | const QString & | name | ) |
Remove a property named name
from this pointer.
- Parameters
-
name identifier of the property to remove.
Definition at line 312 of file Pointer.cpp.
|
signal |
Emitted when this pointer is removed.
|
slot |
Remove dynamic property with identifier property
from data element.
- Parameters
-
property is identifier of the property
Definition at line 270 of file Pointer.cpp.
Definition at line 286 of file Pointer.cpp.
QScriptValue Pointer::scriptValue | ( | ) | const |
if the qtscript is enabled for this rocs, this method returns the self-referenced script value for this pointer.
- Returns
- QScriptValue self reference for this datum.
Definition at line 346 of file Pointer.cpp.
void Pointer::self_remove | ( | ) |
- Deprecated:
- Will be removed in Rocs 2.0
Definition at line 203 of file Pointer.cpp.
QScriptValue Pointer::set_type | ( | int | type | ) |
Set pointer type from script engine.
If pointer type type
does not exist, the method returns false. Otherwise it returns true.
- Parameters
-
type is pointer type identifier.
- Returns
- if change of type was successful
Definition at line 296 of file Pointer.cpp.
|
slot |
Set color attribute of this pointer.
The new color must be set either in format "#000000" or by it's english name ("red" for example).
- Parameters
-
color is the new color of this pointer
Definition at line 231 of file Pointer.cpp.
void Pointer::setEngine | ( | QScriptEngine * | engine | ) |
if qtscript is enabled for this rocs, this method will set the engine for this single object engine
the QScriptEngine that will work on the object
Definition at line 340 of file Pointer.cpp.
|
slot |
Change pointer type of the pointer.
The specified pointer type must exist.
- Parameters
-
pointerType is the new pointer type
Definition at line 161 of file Pointer.cpp.
|
slot |
Definition at line 220 of file Pointer.cpp.
|
slot |
Set the width of this pointer.
- Parameters
-
width of the pointer line
Definition at line 249 of file Pointer.cpp.
QScriptValue Pointer::start | ( | ) | const |
- Returns
- the
- See also
- from() data element for script engine
Definition at line 322 of file Pointer.cpp.
|
slot |
- Returns
- line style of the pointer line
Definition at line 255 of file Pointer.cpp.
DataPtr Pointer::to | ( | ) | const |
- Returns
- target data element of this pointer
|
slot |
- Returns
- pointer type identifier for script engine
|
slot |
Definition at line 276 of file Pointer.cpp.
|
slot |
qreal Pointer::width | ( | ) | const |
- Returns
- width of the pointer
Property Documentation
|
read |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.