step/stepcore
#include <collisionsolver.h>
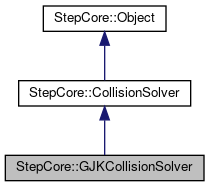
Public Member Functions | |
GJKCollisionSolver () | |
GJKCollisionSolver (const GJKCollisionSolver &solver) | |
void | bodyAdded (BodyList &bodies, Body *body) |
void | bodyRemoved (BodyList &bodies, Body *body) |
int | checkContacts (BodyList &bodies, bool collisions=false, int *count=NULL) |
void | getContactsInfo (ConstraintsInfo &info, bool collisions=false) |
GJKCollisionSolver & | operator= (const GJKCollisionSolver &) |
void | resetCaches () |
int | solveCollisions (BodyList &bodies) |
![]() | |
CollisionSolver () | |
virtual | ~CollisionSolver () |
double | localError () const |
virtual void | setToleranceAbs (double toleranceAbs) |
double | toleranceAbs () const |
![]() | |
Object (const QString &name=QString()) | |
virtual | ~Object () |
const QString & | name () const |
void | setName (const QString &name) |
Protected Member Functions | |
void | addContact (Body *body0, Body *body1) |
void | checkCache (BodyList &bodies) |
int | checkContact (Contact *contact) |
int | checkDiskDisk (Contact *contact) |
int | checkDiskParticle (Contact *contact) |
int | checkPolygonDisk (Contact *contact) |
int | checkPolygonParticle (Contact *contact) |
int | checkPolygonPolygon (Contact *contact) |
int | solveDiskDisk (Contact *contact) |
int | solveDiskParticle (Contact *contact) |
int | solvePolygonDisk (Contact *contact) |
int | solvePolygonParticle (Contact *contact) |
int | solvePolygonPolygon (Contact *contact) |
Protected Attributes | |
ContactValueList | _contacts |
bool | _contactsIsValid |
![]() | |
double | _localError |
double | _toleranceAbs |
![]() | |
QString | _name |
Additional Inherited Members | |
![]() | |
enum | { InternalError = Solver::CollisionError } |
Detailed Description
Discrete collision solver using Gilbert-Johnson-Keerthi distance algorithm.
Objects are treated as colliding if distance between them is greater than zero but smaller than certain small value. If distance is less than zero objects are always treated as interpenetrating - this signals World::doEvolve to invalidate current time step and try with smaller stepSize until objects are colliding but not interpenetrating.
Definition at line 154 of file collisionsolver.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 159 of file collisionsolver.h.
|
inline |
Definition at line 162 of file collisionsolver.h.
Member Function Documentation
Definition at line 1248 of file collisionsolver.cc.
Reimplemented from StepCore::CollisionSolver.
Definition at line 1225 of file collisionsolver.cc.
Reimplemented from StepCore::CollisionSolver.
Definition at line 1234 of file collisionsolver.cc.
|
protected |
Definition at line 1209 of file collisionsolver.cc.
|
inlineprotected |
Definition at line 828 of file collisionsolver.cc.
|
virtual |
Check (and update) state of the contact.
- Parameters
-
contact contact to check (only body0 and body1 fields must be set)
- Returns
- state of the contact (equals to contact->state)Check and count contacts between several bodies
- Parameters
-
bodies list of bodies to check count number of contacts
- Returns
- maximum contact state (i.e. maximum value of Contact::state)
Implements StepCore::CollisionSolver.
Definition at line 839 of file collisionsolver.cc.
|
protected |
Definition at line 732 of file collisionsolver.cc.
|
protected |
Definition at line 769 of file collisionsolver.cc.
|
protected |
Definition at line 347 of file collisionsolver.cc.
|
protected |
Definition at line 541 of file collisionsolver.cc.
|
protected |
Definition at line 39 of file collisionsolver.cc.
|
virtual |
Fill the constraint info structure with the contacts computed by checkContacts()
- Parameters
-
info ConstraintsInfo structure to fill
Implements StepCore::CollisionSolver.
Definition at line 862 of file collisionsolver.cc.
|
inline |
Definition at line 164 of file collisionsolver.h.
|
virtual |
Reset internal caches of collision information.
- Todo:
- do it automatically by checking the cache
Reimplemented from StepCore::CollisionSolver.
Definition at line 1285 of file collisionsolver.cc.
|
virtual |
Solve the collisions between bodies.
Implements StepCore::CollisionSolver.
Definition at line 1173 of file collisionsolver.cc.
|
protected |
Definition at line 1131 of file collisionsolver.cc.
|
protected |
Definition at line 1152 of file collisionsolver.cc.
|
protected |
Definition at line 1035 of file collisionsolver.cc.
|
protected |
Definition at line 1083 of file collisionsolver.cc.
|
protected |
Definition at line 974 of file collisionsolver.cc.
Member Data Documentation
|
protected |
Definition at line 207 of file collisionsolver.h.
|
protected |
Definition at line 208 of file collisionsolver.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:43 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.