step/stepcore
#include <particle.h>
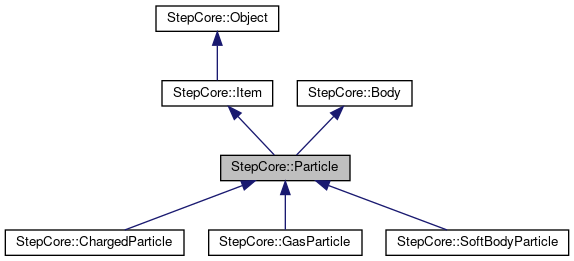
Public Types | |
enum | { PositionOffset = 0 } |
Public Member Functions | |
Particle (Vector2d position=Vector2d::Zero(), Vector2d velocity=Vector2d::Zero(), double mass=1) | |
Vector2d | acceleration () const |
void | addForce (const double *force, const double *forceVariance) |
void | applyForce (const Vector2d &force) |
const Vector2d & | force () const |
void | getAccelerations (double *acceleration, double *accelerationVariance) |
void | getInverseMass (VectorXd *inverseMass, DynSparseRowMatrix *variance, int offset) |
void | getVariables (double *position, double *velocity, double *positionVariance, double *velocityVariance) |
double | kineticEnergy () const |
double | mass () const |
Vector2d | momentum () const |
ParticleErrors * | particleErrors () |
const Vector2d & | position () const |
void | resetForce (bool resetVariance) |
void | setForce (const Vector2d &force) |
void | setKineticEnergy (double kineticEnergy) |
void | setMass (double mass) |
void | setMomentum (const Vector2d &momentum) |
void | setPosition (const Vector2d &position) |
void | setVariables (const double *position, const double *velocity, const double *positionVariance, const double *velocityVariance) |
void | setVelocity (const Vector2d &velocity) |
int | variablesCount () |
const Vector2d & | velocity () const |
![]() | |
Item (const QString &name=QString()) | |
Item (const Item &item) | |
virtual | ~Item () |
Color | color () const |
void | deleteObjectErrors () |
ItemGroup * | group () const |
ObjectErrors * | objectErrors () |
Item & | operator= (const Item &item) |
void | setColor (Color color) |
virtual void | setGroup (ItemGroup *group) |
virtual void | setWorld (World *world) |
ObjectErrors * | tryGetObjectErrors () const |
World * | world () const |
virtual void | worldItemRemoved (Item *item STEPCORE_UNUSED) |
![]() | |
Object (const QString &name=QString()) | |
virtual | ~Object () |
const QString & | name () const |
void | setName (const QString &name) |
![]() | |
Body () | |
virtual | ~Body () |
int | variablesOffset () const |
Protected Member Functions | |
ObjectErrors * | createObjectErrors () |
Protected Attributes | |
Vector2d | _force |
double | _mass |
Vector2d | _position |
Vector2d | _velocity |
![]() | |
QString | _name |
Detailed Description
Particle with mass.
Definition at line 103 of file particle.h.
Member Enumeration Documentation
anonymous enum |
Enumerator | |
---|---|
PositionOffset |
Offset of particle position in variables array. |
Definition at line 108 of file particle.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a particle.
Definition at line 110 of file particle.cc.
Member Function Documentation
|
inline |
Get acceleration of the particle.
Definition at line 127 of file particle.h.
|
virtual |
Add force and (possibly) its variance to force accomulator.
- Note
- This function is used only by generic constraints handling code, force objects should use body-specific functions.
Implements StepCore::Body.
Definition at line 154 of file particle.cc.
|
inline |
Apply force to the body.
Definition at line 135 of file particle.h.
|
inlineprotectedvirtual |
Creates specific ObjectErrors-derived class (to be reimplemented in derived classes)
Reimplemented from StepCore::Item.
Reimplemented in StepCore::ChargedParticle.
Definition at line 168 of file particle.h.
|
inline |
Get force that acts upon particle.
Definition at line 130 of file particle.h.
|
virtual |
Copy acceleration (forces left-multiplied by inverse mass) and (possibly) its variances to arrays.
Variances should only be copied if accelerationVariance != NULL.
Implements StepCore::Body.
Definition at line 141 of file particle.cc.
|
virtual |
Get inverse mass and (possibly) its variance matrixes.
Variance should only be copied of variance != NULL.
Implements StepCore::Body.
Definition at line 171 of file particle.cc.
|
virtual |
Copy positions, velocities and (possibly) its variances to arrays.
Variances should only be copied if positionVariance != NULL.
Implements StepCore::Body.
Definition at line 115 of file particle.cc.
|
inline |
Get kinetic energy of the particle.
Definition at line 148 of file particle.h.
|
inline |
Get mass of the particle.
Definition at line 138 of file particle.h.
|
inline |
Get momentum of the particle.
Definition at line 143 of file particle.h.
|
inline |
Get (and possibly create) ParticleErrors object.
Definition at line 164 of file particle.h.
|
inline |
Get position of the particle.
Definition at line 117 of file particle.h.
|
virtual |
Reset force accomulator and (possibly) its variance to zero.
Variance should only be reseted if resetVariance == true.
Implements StepCore::Body.
Definition at line 165 of file particle.cc.
|
inline |
Set force that acts upon particle.
Definition at line 132 of file particle.h.
void StepCore::Particle::setKineticEnergy | ( | double | kineticEnergy | ) |
Set kinetic energy of the particle (will modify only velocity)
Definition at line 183 of file particle.cc.
|
inline |
Set mass of the particle.
Definition at line 140 of file particle.h.
|
inline |
Set momentum of the particle (will modify only velocity)
Definition at line 145 of file particle.h.
|
inline |
Set position of the particle.
Definition at line 119 of file particle.h.
|
virtual |
Set positions, velocities and (possibly) its variances using values in arrays and also reset accelerations and its variances.
Variances should only be copied and reseted if positionVariance != NULL.
Implements StepCore::Body.
Definition at line 127 of file particle.cc.
|
inline |
Set velocity of the particle.
Definition at line 124 of file particle.h.
|
inlinevirtual |
Get count of dynamic variables (not including velocities)
Implements StepCore::Body.
Definition at line 152 of file particle.h.
|
inline |
Get velocity of the particle.
Definition at line 122 of file particle.h.
Member Data Documentation
|
protected |
Definition at line 172 of file particle.h.
|
protected |
Definition at line 173 of file particle.h.
|
protected |
Definition at line 170 of file particle.h.
|
protected |
Definition at line 171 of file particle.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:43 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.