step/stepcore
#include <solver.h>
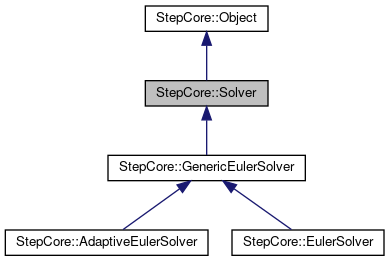
Public Types | |
enum | { OK = 0, ToleranceError = 2048, InternalError = 2049, CollisionDetected = 4096, IntersectionDetected = 4097, Aborted = 8192, CollisionError = 16384, ConstraintError = 32768 } |
typedef int(* | Function )(double t, const double *y, const double *yvar, double *f, double *fvar, void *params) |
Public Member Functions | |
Solver (int dimension=0, Function function=NULL, void *params=NULL, double stepSize=0.001) | |
Solver (double stepSize) | |
virtual | ~Solver () |
int | dimension () const |
virtual int | doCalcFn (double *t, const VectorXd *y, const VectorXd *yvar=0, VectorXd *f=0, VectorXd *fvar=0)=0 |
virtual int | doEvolve (double *t, double t1, VectorXd *y, VectorXd *yvar)=0 |
Function | function () const |
double | localError () const |
double | localErrorRatio () const |
void * | params () const |
virtual void | setDimension (int dimension) |
virtual void | setFunction (Function function) |
virtual void | setParams (void *params) |
virtual void | setStepSize (double stepSize) |
virtual void | setToleranceAbs (double toleranceAbs) |
virtual void | setToleranceRel (double toleranceRel) |
QString | solverType () const |
double | stepSize () const |
double | toleranceAbs () const |
double | toleranceRel () const |
![]() | |
Object (const QString &name=QString()) | |
virtual | ~Object () |
const QString & | name () const |
void | setName (const QString &name) |
Protected Attributes | |
int | _dimension |
Function | _function |
double | _localError |
double | _localErrorRatio |
void * | _params |
double | _stepSize |
double | _toleranceAbs |
double | _toleranceRel |
![]() | |
QString | _name |
Detailed Description
Generic Solver interface.
Provides generic interface suitable for large variety of ordinaty differential equations integration algorithms.
It solves system of the first order differential equations:
Dimension of system is provided via setDimension(), function is provided via setFunction().
It also provides interface for setting allowed local tolerance. It can be defined using two properties: toleranceAbs and toleranceRel.
On each step solver calculates local error estimation (which depends on used integration method) and local error ratio for each variable using the following formula:
Non-adaptive solvers cancel current step if maximal local error ratio is bigger than 1.1 (exact value depends on solver) and doEvolve() function returns false.
Adaptive solvers use maximal local error ratio to change time step until the ratio becomes almost equal 1. If it can't be made smaller than 1.1 (exact value depends on solver), solvers cancel current step and doEvolve() function returns false.
Maximal (over all variables) error estimation and error ratio from last time step can be obtained using localError() and localErrorRatio() methods.
Member Typedef Documentation
typedef int(* StepCore::Solver::Function)(double t, const double *y, const double *yvar, double *f, double *fvar, void *params) |
Member Enumeration Documentation
anonymous enum |
Constructor & Destructor Documentation
|
inlineexplicit |
|
inline |
Member Function Documentation
|
inline |
|
pure virtual |
Calculate function value.
Implemented in StepCore::GenericEulerSolver.
|
pure virtual |
Integrate.
- Parameters
-
t Current time (will be updated by the new value) t1 Target time y Function value yvar Function variance
- Returns
- Solver::OK on success, error status on failure
- Todo:
- Provide error message
Implemented in StepCore::GenericEulerSolver.
|
inline |
|
inline |
|
inline |
|
inline |
|
inlinevirtual |
Set ODE dimension.
Reimplemented in StepCore::GenericEulerSolver.
|
inlinevirtual |
|
inlinevirtual |
|
inlinevirtual |
|
inlinevirtual |
|
inlinevirtual |
|
inline |
|
inline |
|
inline |
|
inline |
Member Data Documentation
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:43 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.