step/stepcore
object.h File Reference
Include dependency graph for object.h:
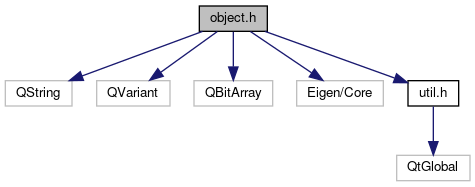
This graph shows which files directly or indirectly include this file:
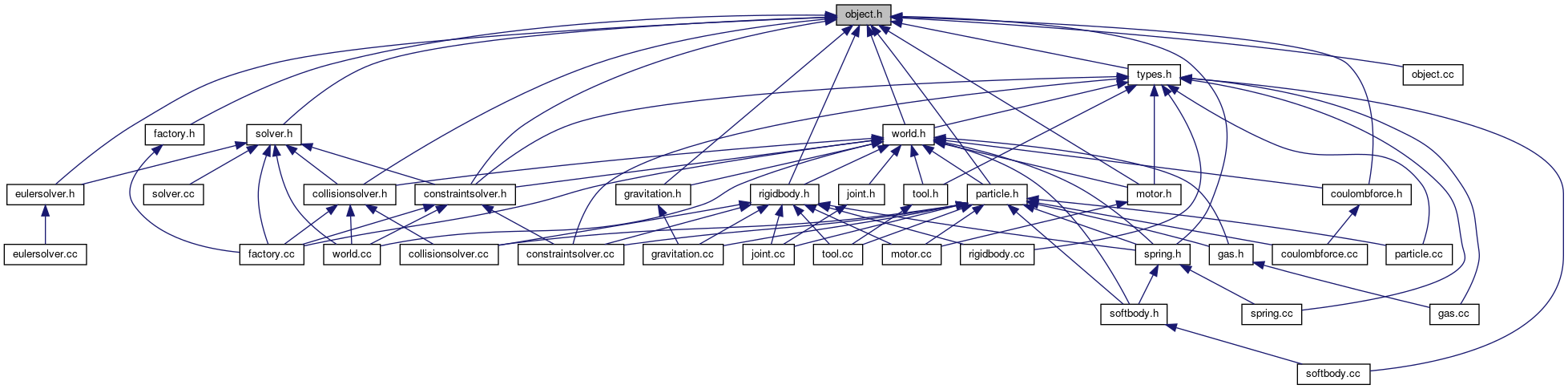
Go to the source code of this file.
Classes | |
class | StepCore::MetaObject |
struct | StepCore::MetaObjectHelper< Class, N > |
struct | StepCore::MetaObjectHelper< Class, MetaObject::ABSTRACT > |
class | StepCore::MetaProperty |
struct | StepCore::MetaPropertyHelper< C, T > |
class | StepCore::Object |
Namespaces | |
StepCore | |
Macros | |
#define | _STEPCORE_PROPERTY_NULL StepCore::MetaProperty() |
#define | STEPCORE_FROM_UTF8(str) QString::fromUtf8(str) |
#define | STEPCORE_META_OBJECT(_className, _classNameNoop, _description, _flags, __superClasses, __properties) |
#define | STEPCORE_OBJECT(_className) |
#define | STEPCORE_OBJECT_NA(_className) |
#define | STEPCORE_PROPERTY_R(_type, _name, _nameNoop, _units, _description, _read) STEPCORE_PROPERTY_RF(_type, _name, _nameNoop, _units, _description, 0, _read) |
#define | STEPCORE_PROPERTY_R_D(_type, _name, _nameNoop, _units, _description, _read) STEPCORE_PROPERTY_RF(_type, _name, _nameNoop, _units, _description, StepCore::MetaProperty::DYNAMIC, _read) |
#define | STEPCORE_PROPERTY_RF(_type, _name, _nameNoop, _units, _description, _flags, _read) |
#define | STEPCORE_PROPERTY_RW(_type, _name, _nameNoop, _units, _description, _read, _write) |
#define | STEPCORE_PROPERTY_RW_D(_type, _name, _nameNoop, _units, _description, _read, _write) |
#define | STEPCORE_PROPERTY_RWF(_type, _name, _nameNoop, _units, _description, _flags, _read, _write) |
#define | STEPCORE_SUPER_CLASS(_className) _className::staticMetaObject(), |
#define | STEPCORE_UNITS_1 QString("") |
#define | STEPCORE_UNITS_NULL QString() |
Functions | |
template<class _Dst , class _Src > | |
_Dst | StepCore::stepcore_cast (_Src src) |
template<typename T > | |
T | StepCore::stringToType (const QString &s, bool *ok) |
template<typename T > | |
QString | StepCore::typeToString (const T &v) |
template<typename T > | |
QVariant | StepCore::typeToVariant (const T &v) |
template<typename T > | |
T | StepCore::variantToType (const QVariant &v, bool *ok) |
Detailed Description
Object, MetaObject and MetaProperty classes.
Definition in file object.h.
Macro Definition Documentation
#define _STEPCORE_PROPERTY_NULL StepCore::MetaProperty() |
#define STEPCORE_FROM_UTF8 | ( | str | ) | QString::fromUtf8(str) |
#define STEPCORE_META_OBJECT | ( | _className, | |
_classNameNoop, | |||
_description, | |||
_flags, | |||
__superClasses, | |||
__properties | |||
) |
Value:
const StepCore::MetaProperty _className::_classProperties[] = { _STEPCORE_PROPERTY_NULL, __properties }; \
const StepCore::MetaObject* _className::_superClasses[] = { 0, __superClasses }; \
const StepCore::MetaObject _className::_metaObject = { \
QString(STEPCORE_STRINGIFY(_className)), QString(_description), _flags, \
StepCore::MetaObjectHelper<_className, _flags & StepCore::MetaObject::ABSTRACT>::newObjectHelper, \
StepCore::MetaObjectHelper<_className, _flags & StepCore::MetaObject::ABSTRACT>::cloneObjectHelper, \
_superClasses+1, sizeof(_superClasses)/sizeof(*_superClasses)-1, \
_classProperties+1, sizeof(_classProperties)/sizeof(*_classProperties)-1, false, 0, 0, 0, QBitArray(), "", "" };
#define STEPCORE_OBJECT | ( | _className | ) |
Value:
public: \
STEPCORE_OBJECT_NA(_className)
#define STEPCORE_OBJECT_NA | ( | _className | ) |
Value:
private: \
typedef _className _thisType; \
static const StepCore::MetaObject _metaObject; \
static const StepCore::MetaObject* _superClasses[]; \
static const StepCore::MetaProperty _classProperties[]; \
public: \
private:
#define STEPCORE_PROPERTY_R | ( | _type, | |
_name, | |||
_nameNoop, | |||
_units, | |||
_description, | |||
_read | |||
) | STEPCORE_PROPERTY_RF(_type, _name, _nameNoop, _units, _description, 0, _read) |
#define STEPCORE_PROPERTY_R_D | ( | _type, | |
_name, | |||
_nameNoop, | |||
_units, | |||
_description, | |||
_read | |||
) | STEPCORE_PROPERTY_RF(_type, _name, _nameNoop, _units, _description, StepCore::MetaProperty::DYNAMIC, _read) |
#define STEPCORE_PROPERTY_RF | ( | _type, | |
_name, | |||
_nameNoop, | |||
_units, | |||
_description, | |||
_flags, | |||
_read | |||
) |
Value:
StepCore::MetaProperty( STEPCORE_STRINGIFY(_name), _units, _description, \
StepCore::MetaProperty::READABLE | _flags, qMetaTypeId<_type>(), \
Definition: object.h:289
#define STEPCORE_PROPERTY_RW | ( | _type, | |
_name, | |||
_nameNoop, | |||
_units, | |||
_description, | |||
_read, | |||
_write | |||
) |
Value:
STEPCORE_PROPERTY_RWF(_type, _name, _nameNoop, _units, _description, \
StepCore::MetaProperty::STORED, _read, _write)
#define STEPCORE_PROPERTY_RWF(_type, _name, _nameNoop, _units, _description, _flags, _read, _write)
Definition: object.h:390
#define STEPCORE_PROPERTY_RW_D | ( | _type, | |
_name, | |||
_nameNoop, | |||
_units, | |||
_description, | |||
_read, | |||
_write | |||
) |
Value:
STEPCORE_PROPERTY_RWF(_type, _name, _nameNoop, _units, _description, \
StepCore::MetaProperty::STORED | StepCore::MetaProperty::DYNAMIC, _read, _write)
#define STEPCORE_PROPERTY_RWF(_type, _name, _nameNoop, _units, _description, _flags, _read, _write)
Definition: object.h:390
Variable changes during simulation or changes of other properties.
Definition: object.h:84
#define STEPCORE_PROPERTY_RWF | ( | _type, | |
_name, | |||
_nameNoop, | |||
_units, | |||
_description, | |||
_flags, | |||
_read, | |||
_write | |||
) |
Value:
StepCore::MetaProperty( STEPCORE_STRINGIFY(_name), _units, _description, \
qMetaTypeId<_type>(), \
Definition: object.h:289
#define STEPCORE_SUPER_CLASS | ( | _className | ) | _className::staticMetaObject(), |
This file is part of the KDE documentation.
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:43 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:43 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.