kblackbox
#include <kbbballsonboard.h>
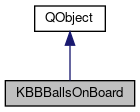
Signals | |
void | changes () |
Public Member Functions | |
KBBBallsOnBoard (KBBGameDoc *parent, const int columns, const int rows) | |
int | absolutePositionToBorderPosition (int position[DIM_MAX]) |
int | absolutePositionToBoxPosition (int position[DIM_MAX]) |
void | add (int boxPosition) |
void | borderPositionToAbsolutePosition (int borderPosition, int position[DIM_MAX]) |
int | columns () |
bool | contains (int boxPosition) |
int | count () |
void | newBoard (const int columns, const int rows) |
int | numberOfBallsNotIn (KBBBallsOnBoard *otherBoard) |
int | oppositeBorderPosition (int borderPosition) |
int | oppositeBorderPositionWithPoints (const int borderPosition, QList< int > &points) |
void | ray (const int borderPosition, QList< int > &points) |
void | remove (int boxPosition) |
int | rows () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Set of balls (or various objects) with positions on the board.
The set of balls manages the position and the number of the balls. The balls can be placed of any kind (placed by the player or the hiden balls to find for instance).
It computes also the trajectory of the laser ray with the given balls.
There are 3 different kinds of coordinates for object positions.
- The 1st one is the (absolute) position in 2 dimensions between (0,0) and (2 + m_columns + 2, 2 + m_rows + 2). It is used to manage the positions of the graphic elements but also to calculate the laser ray trajectory.
- The 2nd one is the border position in 1 dimension between 0 and (2 * m_rows + 2 * m_columns -1). Only borders can be managed with this coordinate.
- The 3rd one is the box position in 1 dimension between 0 and (m_columns*m_rows - 1). It is used to manage the postion of the balls in the black box.
Definition at line 62 of file kbbballsonboard.h.
Constructor & Destructor Documentation
KBBBallsOnBoard::KBBBallsOnBoard | ( | KBBGameDoc * | parent, |
const int | columns, | ||
const int | rows | ||
) |
Constructor.
Definition at line 44 of file kbbballsonboard.cpp.
Member Function Documentation
int KBBBallsOnBoard::absolutePositionToBorderPosition | ( | int | position[DIM_MAX] | ) |
Convert (absolute) position to border position.
- Parameters
-
position The (absolute) position to convert.
- Returns
- The result of the conversion: border position.
- See also
- borderPositionToAbsolutePosition(int borderPosition, int position[DIM_MAX])
- absolutePositionToBorderPosition(int position[DIM_MAX])
Definition at line 56 of file kbbballsonboard.cpp.
int KBBBallsOnBoard::absolutePositionToBoxPosition | ( | int | position[DIM_MAX] | ) |
Convert (absolute) position to box position.
- Parameters
-
position The (absolute) position to convert.
- Returns
- The result of the conversion: box position.
Definition at line 72 of file kbbballsonboard.cpp.
void KBBBallsOnBoard::add | ( | int | boxPosition | ) |
Add a ball on this board.
- Parameters
-
boxPosition The box position of the ball to add
- See also
- remove(int boxPosition)
Definition at line 78 of file kbbballsonboard.cpp.
void KBBBallsOnBoard::borderPositionToAbsolutePosition | ( | int | borderPosition, |
int | position[DIM_MAX] | ||
) |
Convert border position to (abosulte) position.
- Parameters
-
borderPosition The border position to convert. position The result of the conversion: (absolute) position.
- See also
- borderPositionToAbsolutePosition(int position[DIM_MAX])
Definition at line 85 of file kbbballsonboard.cpp.
|
signal |
int KBBBallsOnBoard::columns | ( | ) |
Definition at line 106 of file kbbballsonboard.cpp.
bool KBBBallsOnBoard::contains | ( | int | boxPosition | ) |
Check if there is a ball at the given position in the black box.
- Parameters
-
boxPosition Box position to check
Definition at line 112 of file kbbballsonboard.cpp.
int KBBBallsOnBoard::count | ( | ) |
Number of balls on this board.
Definition at line 118 of file kbbballsonboard.cpp.
void KBBBallsOnBoard::newBoard | ( | const int | columns, |
const int | rows | ||
) |
Define a new board and remove all balls.
- Parameters
-
columns Number of columns rows Number of rows
Definition at line 124 of file kbbballsonboard.cpp.
int KBBBallsOnBoard::numberOfBallsNotIn | ( | KBBBallsOnBoard * | otherBoard | ) |
Compares 2 boards and return the number of differences.
- Parameters
-
otherBoard Other set of balls in a board
- Returns
- Number of balls in the set that are not in the other given set
Definition at line 133 of file kbbballsonboard.cpp.
int KBBBallsOnBoard::oppositeBorderPosition | ( | int | borderPosition | ) |
Compute the opposite border position of the given position.
- Parameters
-
borderPosition The border position
Definition at line 145 of file kbbballsonboard.cpp.
int KBBBallsOnBoard::oppositeBorderPositionWithPoints | ( | const int | borderPosition, |
QList< int > & | points | ||
) |
Definition at line 151 of file kbbballsonboard.cpp.
void KBBBallsOnBoard::ray | ( | const int | borderPosition, |
QList< int > & | points | ||
) |
Compute the trajectory of a ray with the balls of the set.
- Parameters
-
borderPosition The border position
Definition at line 180 of file kbbballsonboard.cpp.
void KBBBallsOnBoard::remove | ( | int | boxPosition | ) |
Remove a ball on this board.
- Parameters
-
boxPosition The box position of the ball to be removed
- See also
- add(int boxPosition);
Definition at line 186 of file kbbballsonboard.cpp.
int KBBBallsOnBoard::rows | ( | ) |
Definition at line 193 of file kbbballsonboard.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.