kigo
#include <themerenderer.h>
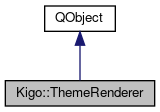
Public Types | |
enum | Element { Background = 1, Board, HandicapMark, WhiteStone, WhiteStoneTransparent, WhiteTerritory, BlackStone, BlackStoneTransparent, BlackTerritory, PlacementMarker } |
Signals | |
void | themeChanged (const QString &) |
Public Member Functions | |
QSize | elementSize (Element element) const |
bool | loadTheme (const QString &themeName) |
void | renderElement (Element element, QPainter *painter, const QRectF &rect) const |
QPixmap | renderElement (Element element, const QSize &size) const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static ThemeRenderer * | self () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
The class ThemeRenderer loads shapes from a SVG theme file and converts them into pixmaps which can be used by QGraphicsView classes to display the game scene.
The class is implemented as a singleton because it is needed only once per application and uses a pixamp cache to efficiently store the pixmaps.
ThemeRenderer is implemented as a singleton.
- Since
- 0.1
Definition at line 47 of file themerenderer.h.
Member Enumeration Documentation
Enumeration of all possible renderable scene element types.
Enumerator | |
---|---|
Background | |
Board | |
HandicapMark | |
WhiteStone | |
WhiteStoneTransparent | |
WhiteTerritory | |
BlackStone | |
BlackStoneTransparent | |
BlackTerritory | |
PlacementMarker |
Definition at line 59 of file themerenderer.h.
Member Function Documentation
Retrieves the default size of a given theme element from the current SVG theme.
- Parameters
-
element The theme element to return the size of
- Returns
- The rounded size of the elements rectangle
Definition at line 182 of file themerenderer.cpp.
bool Kigo::ThemeRenderer::loadTheme | ( | const QString & | themeName | ) |
Load the game theme specified by 'name'.
See the correspondig KConfigXT settings file and available themes for details.
- Parameters
-
themeName The name of the theme to load
Definition at line 52 of file themerenderer.cpp.
void Kigo::ThemeRenderer::renderElement | ( | Element | element, |
QPainter * | painter, | ||
const QRectF & | rect | ||
) | const |
Renders a specific element of the current SVG theme.
- Parameters
-
element Determines which part of the theme to render painter The QPainter to paint the element with rect The desired size of the element to render
Definition at line 86 of file themerenderer.cpp.
Renders and returns a specific element of the current SVG theme.
- Parameters
-
element Determines which part of the theme to render size The desired size of the element to render
Definition at line 97 of file themerenderer.cpp.
|
inlinestatic |
Only one ThemeRenderer is needed per application, this method returns the singleton self.
- Returns
- ThemeRenderer self
Definition at line 78 of file themerenderer.h.
|
signal |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:29 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.