libkdegames/highscore
#include <KScoreDialog>
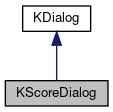
Public Types | |
enum | AddScoreFlag { AskName = 0x1, LessIsMore = 0x2 } |
typedef QMap< int, QString > | FieldInfo |
enum | Fields { Name = 1 << 0, Level = 1 << 1, Date = 1 << 2, Time = 1 << 3, Score = 1 << 4, Custom1 = 1 << 10, Custom2 = 1 << 11, Custom3 = 1 << 12, Custom4 = 1 << 13, Custom5 = 1 << 14, Max = 1 << 30 } |
Public Member Functions | |
KScoreDialog (int fields=Name, QWidget *parent=0) | |
~KScoreDialog () | |
void | addField (int field, const QString &header, const QString &key) |
void | addLocalizedConfigGroupName (const QPair< QByteArray, QString > &group) |
void | addLocalizedConfigGroupNames (const QMap< QByteArray, QString > &groups) |
int | addScore (const FieldInfo &newInfo=FieldInfo(), const AddScoreFlags &flags=0) |
int | addScore (int newScore, const AddScoreFlags &flags=0) |
virtual void | exec () |
void | hideField (int field) |
int | highScore () |
void | initFromDifficulty (const KgDifficulty *difficulty, bool setConfigGroup=true) |
void | setComment (const QString &comment) |
void KDE_DEPRECATED | setConfigGroup (const QString &group=QString()) |
void | setConfigGroup (const QPair< QByteArray, QString > &group) |
void | setConfigGroupWeights (const QMap< int, QByteArray > &weights) |
void | setHiddenConfigGroups (const QList< QByteArray > &hiddenGroups) |
virtual void | show () |
Detailed Description
A simple high score implementation.
This class can be used both for displaying the current high scores and also for adding new highscores. It is the recommended way of implementing a simple highscore table.
To display the current highscores it is simply a case of creating a KScoreDialog object and calling exec(). This example code will display the Name and Score (the score is always added automatically unless hidden hideField since it is used for sorting) of the top 10 players:
To add a new highscore, e.g. at the end of a game you simply create an object with the Fields you want to write (i.e. KScoreDialog::Name | KScoreDialog::Score), call addScore and then (optionally) display the dialog. This code will allow you to add a highscore with a Name and Score field. If it's the first time a player has a score on the table, they will be prompted for their name but subsequent times they will have their name filled in automatically.
Or if you want to fill the name in from the code you can pass a default name by doing
If you want to add an extra field (e.g. the number of moves taken) then do
You can define up to 5 Custom fields.
Definition at line 96 of file kscoredialog.h.
Member Typedef Documentation
Definition at line 126 of file kscoredialog.h.
Member Enumeration Documentation
Flags for setting preferences for adding scores.
Enumerator | |
---|---|
AskName |
Promt the player for their name. |
LessIsMore |
A lower numerical score means higher placing on the table. |
Definition at line 119 of file kscoredialog.h.
enum KScoreDialog::Fields |
Highscore fields.
Enumerator | |
---|---|
Name | |
Level | |
Date | |
Time | |
Score | |
Custom1 |
Field for custom information. |
Custom2 | |
Custom3 | |
Custom4 | |
Custom5 | |
Max |
Only for setting a maximum. |
Definition at line 102 of file kscoredialog.h.
Constructor & Destructor Documentation
- Parameters
-
fields Bitwise OR of the Fields that should be listed (Score is always present) parent passed to parent QWidget constructor.
Definition at line 101 of file kscoredialog.cpp.
KScoreDialog::~KScoreDialog | ( | ) |
Definition at line 144 of file kscoredialog.cpp.
Member Function Documentation
Define an extra FieldInfo entry.
- Parameters
-
field id of this field Fields e.g. KScoreDialog::Custom1 header text shown in the header in the dialog for this field. e.g. "Number of Moves" key unique key used to store this field. e.g. "moves"
Definition at line 222 of file kscoredialog.cpp.
void KScoreDialog::addLocalizedConfigGroupName | ( | const QPair< QByteArray, QString > & | group | ) |
You must add the translations of all group names to the dialog.
This is best done by passing the name through ki18n(). The group set through setConfigGroup(const KLocalizedString& group) will be added automatically
- Parameters
-
group the translated group name
Definition at line 164 of file kscoredialog.cpp.
void KScoreDialog::addLocalizedConfigGroupNames | ( | const QMap< QByteArray, QString > & | groups | ) |
You must add the translations of all group names to the dialog.
This is best done by passing the name through ki18n(). The group set through setConfigGroup(const KLocalizedString& group) will be added automatically.
This function can be used directly with KGameDifficulty::localizedLevelStrings().
- Parameters
-
group the list of translated group names
Definition at line 173 of file kscoredialog.cpp.
int KScoreDialog::addScore | ( | const FieldInfo & | newInfo = FieldInfo() , |
const AddScoreFlags & | flags = 0 |
||
) |
Adds a new score to the list.
- Parameters
-
newInfo info about the score. flags set whether the user should be prompted for their name and how the scores should be sorted
- Returns
- The highscore position if the score was good enough to make it into the list (1 being topscore) or 0 otherwise.
Definition at line 515 of file kscoredialog.cpp.
int KScoreDialog::addScore | ( | int | newScore, |
const AddScoreFlags & | flags = 0 |
||
) |
Convenience function for ease of use.
- Parameters
-
newScore the score of the player. flags set whether the user should be prompted for their name and how the scores should be sorted
- Returns
- The highscore position if the score was good enough to make it into the list (1 being topscore) or 0 otherwise.
Definition at line 594 of file kscoredialog.cpp.
|
virtual |
Display the dialog as modal.
Definition at line 607 of file kscoredialog.cpp.
void KScoreDialog::hideField | ( | int | field | ) |
Hide a field so that it is not shown on the table (but is still stored in the configuration file).
- Parameters
-
field id of this field Fields e.g. KScoreDialog::Score
Definition at line 229 of file kscoredialog.cpp.
int KScoreDialog::highScore | ( | ) |
- Returns
- the current best score in the group
Definition at line 663 of file kscoredialog.cpp.
void KScoreDialog::initFromDifficulty | ( | const KgDifficulty * | difficulty, |
bool | setConfigGroup = true |
||
) |
Assume that config groups (incl.
current selection) are equal to difficulty levels, and initialize them. This is usually equal to the following code using KGameDifficulty:
Definition at line 182 of file kscoredialog.cpp.
void KScoreDialog::setComment | ( | const QString & | comment | ) |
- Parameters
-
comment to add when showing high-scores. The comment is only used once.
Definition at line 217 of file kscoredialog.cpp.
The group name must be passed though I18N_NOOP() in order for the group name to be translated.
i.e.
If you set a group, it will be prefixed in the config file by 'KHighscore_' otherwise the group will simply be 'KHighscore'.
- Parameters
-
group to use for reading/writing highscores from/to.
- Deprecated:
- since 4.1
Definition at line 151 of file kscoredialog.cpp.
void KScoreDialog::setConfigGroup | ( | const QPair< QByteArray, QString > & | group | ) |
The group name must be passed though ki18n() in order for the group name to be translated.
i.e.
If you set a group, it will be prefixed in the config file by 'KHighscore_' otherwise the group will simply be 'KHighscore'.
- Parameters
-
group to use for reading/writing highscores from/to.
Definition at line 157 of file kscoredialog.cpp.
void KScoreDialog::setConfigGroupWeights | ( | const QMap< int, QByteArray > & | weights | ) |
It is a good idea giving config group weigths, otherwise tabs get ordered by their tab name that is not probably what you want.
This function can be used directly with KGameDifficulty::levelWeights().
- Parameters
-
group the list of untranslated group names and their weights
- Since
- KDE 4.2
Definition at line 205 of file kscoredialog.cpp.
void KScoreDialog::setHiddenConfigGroups | ( | const QList< QByteArray > & | hiddenGroups | ) |
Hide some config groups so that they are not shown on the dialog (but are still stored in the configuration file).
- Parameters
-
hiddenGroups the list of group names you want to hide
- Since
- KDE 4.6
Definition at line 200 of file kscoredialog.cpp.
|
virtual |
Display the dialog as non-modal.
Definition at line 601 of file kscoredialog.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:46 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.