libkdegames
#include <KGameRenderedItem>
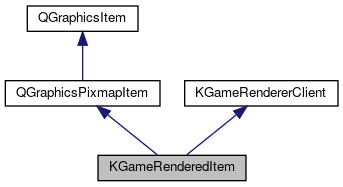
Public Member Functions | |
KGameRenderedItem (KGameRenderer *renderer, const QString &spriteKey, QGraphicsItem *parent=0) | |
virtual | ~KGameRenderedItem () |
![]() | |
QGraphicsPixmapItem (QGraphicsItem *parent) | |
QGraphicsPixmapItem (const QPixmap &pixmap, QGraphicsItem *parent) | |
~QGraphicsPixmapItem () | |
virtual QRectF | boundingRect () const |
virtual bool | contains (const QPointF &point) const |
virtual bool | isObscuredBy (const QGraphicsItem *item) const |
QPointF | offset () const |
virtual QPainterPath | opaqueArea () const |
virtual void | paint (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) |
QPixmap | pixmap () const |
void | setOffset (const QPointF &offset) |
void | setOffset (qreal x, qreal y) |
void | setPixmap (const QPixmap &pixmap) |
void | setShapeMode (ShapeMode mode) |
void | setTransformationMode (Qt::TransformationMode mode) |
virtual QPainterPath | shape () const |
ShapeMode | shapeMode () const |
Qt::TransformationMode | transformationMode () const |
virtual int | type () const |
![]() | |
QGraphicsItem (QGraphicsItem *parent) | |
virtual | ~QGraphicsItem () |
bool | acceptDrops () const |
Qt::MouseButtons | acceptedMouseButtons () const |
bool | acceptHoverEvents () const |
bool | acceptsHoverEvents () const |
bool | acceptTouchEvents () const |
virtual void | advance (int phase) |
virtual QRectF | boundingRect () const =0 |
QRegion | boundingRegion (const QTransform &itemToDeviceTransform) const |
qreal | boundingRegionGranularity () const |
CacheMode | cacheMode () const |
QList< QGraphicsItem * > | childItems () const |
QList< QGraphicsItem * > | children () const |
QRectF | childrenBoundingRect () const |
void | clearFocus () |
QPainterPath | clipPath () const |
virtual bool | collidesWithItem (const QGraphicsItem *other, Qt::ItemSelectionMode mode) const |
virtual bool | collidesWithPath (const QPainterPath &path, Qt::ItemSelectionMode mode) const |
QList< QGraphicsItem * > | collidingItems (Qt::ItemSelectionMode mode) const |
QGraphicsItem * | commonAncestorItem (const QGraphicsItem *other) const |
QCursor | cursor () const |
QVariant | data (int key) const |
QTransform | deviceTransform (const QTransform &viewportTransform) const |
qreal | effectiveOpacity () const |
void | ensureVisible (const QRectF &rect, int xmargin, int ymargin) |
void | ensureVisible (qreal x, qreal y, qreal w, qreal h, int xmargin, int ymargin) |
bool | filtersChildEvents () const |
GraphicsItemFlags | flags () const |
QGraphicsItem * | focusItem () const |
QGraphicsItem * | focusProxy () const |
void | grabKeyboard () |
void | grabMouse () |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsItemGroup * | group () const |
bool | handlesChildEvents () const |
bool | hasCursor () const |
bool | hasFocus () const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const |
void | installSceneEventFilter (QGraphicsItem *filterItem) |
bool | isActive () const |
bool | isAncestorOf (const QGraphicsItem *child) const |
bool | isBlockedByModalPanel (QGraphicsItem **blockingPanel) const |
bool | isClipped () const |
bool | isEnabled () const |
bool | isObscured () const |
bool | isObscured (const QRectF &rect) const |
bool | isObscured (qreal x, qreal y, qreal w, qreal h) const |
bool | isPanel () const |
bool | isSelected () const |
bool | isUnderMouse () const |
bool | isVisible () const |
bool | isVisibleTo (const QGraphicsItem *parent) const |
bool | isWidget () const |
bool | isWindow () const |
QTransform | itemTransform (const QGraphicsItem *other, bool *ok) const |
QPointF | mapFromItem (const QGraphicsItem *item, const QPointF &point) const |
QPolygonF | mapFromItem (const QGraphicsItem *item, const QRectF &rect) const |
QPolygonF | mapFromItem (const QGraphicsItem *item, const QPolygonF &polygon) const |
QPainterPath | mapFromItem (const QGraphicsItem *item, const QPainterPath &path) const |
QPointF | mapFromItem (const QGraphicsItem *item, qreal x, qreal y) const |
QPolygonF | mapFromItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const |
QPointF | mapFromParent (const QPointF &point) const |
QPolygonF | mapFromParent (const QRectF &rect) const |
QPolygonF | mapFromParent (const QPolygonF &polygon) const |
QPainterPath | mapFromParent (const QPainterPath &path) const |
QPointF | mapFromParent (qreal x, qreal y) const |
QPolygonF | mapFromParent (qreal x, qreal y, qreal w, qreal h) const |
QPolygonF | mapFromScene (const QPolygonF &polygon) const |
QPointF | mapFromScene (const QPointF &point) const |
QPolygonF | mapFromScene (const QRectF &rect) const |
QPainterPath | mapFromScene (const QPainterPath &path) const |
QPointF | mapFromScene (qreal x, qreal y) const |
QPolygonF | mapFromScene (qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectFromItem (const QGraphicsItem *item, const QRectF &rect) const |
QRectF | mapRectFromItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectFromParent (const QRectF &rect) const |
QRectF | mapRectFromParent (qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectFromScene (qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectFromScene (const QRectF &rect) const |
QRectF | mapRectToItem (const QGraphicsItem *item, const QRectF &rect) const |
QRectF | mapRectToItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectToParent (const QRectF &rect) const |
QRectF | mapRectToParent (qreal x, qreal y, qreal w, qreal h) const |
QRectF | mapRectToScene (const QRectF &rect) const |
QRectF | mapRectToScene (qreal x, qreal y, qreal w, qreal h) const |
QPointF | mapToItem (const QGraphicsItem *item, const QPointF &point) const |
QPolygonF | mapToItem (const QGraphicsItem *item, const QRectF &rect) const |
QPolygonF | mapToItem (const QGraphicsItem *item, const QPolygonF &polygon) const |
QPainterPath | mapToItem (const QGraphicsItem *item, const QPainterPath &path) const |
QPointF | mapToItem (const QGraphicsItem *item, qreal x, qreal y) const |
QPolygonF | mapToItem (const QGraphicsItem *item, qreal x, qreal y, qreal w, qreal h) const |
QPointF | mapToParent (const QPointF &point) const |
QPolygonF | mapToParent (const QRectF &rect) const |
QPainterPath | mapToParent (const QPainterPath &path) const |
QPointF | mapToParent (qreal x, qreal y) const |
QPolygonF | mapToParent (qreal x, qreal y, qreal w, qreal h) const |
QPolygonF | mapToParent (const QPolygonF &polygon) const |
QPolygonF | mapToScene (const QRectF &rect) const |
QPainterPath | mapToScene (const QPainterPath &path) const |
QPointF | mapToScene (qreal x, qreal y) const |
QPolygonF | mapToScene (qreal x, qreal y, qreal w, qreal h) const |
QPolygonF | mapToScene (const QPolygonF &polygon) const |
QPointF | mapToScene (const QPointF &point) const |
QMatrix | matrix () const |
void | moveBy (qreal dx, qreal dy) |
qreal | opacity () const |
virtual void | paint (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget)=0 |
QGraphicsItem * | panel () const |
PanelModality | panelModality () const |
QGraphicsItem * | parentItem () const |
QGraphicsObject * | parentObject () const |
QGraphicsWidget * | parentWidget () const |
QPointF | pos () const |
void | removeSceneEventFilter (QGraphicsItem *filterItem) |
void | resetMatrix () |
void | resetTransform () |
void | rotate (qreal angle) |
qreal | rotation () const |
void | scale (qreal sx, qreal sy) |
qreal | scale () const |
QGraphicsScene * | scene () const |
QRectF | sceneBoundingRect () const |
QMatrix | sceneMatrix () const |
QPointF | scenePos () const |
QTransform | sceneTransform () const |
void | scroll (qreal dx, qreal dy, const QRectF &rect) |
void | setAcceptDrops (bool on) |
void | setAcceptedMouseButtons (QFlags< Qt::MouseButton > buttons) |
void | setAcceptHoverEvents (bool enabled) |
void | setAcceptsHoverEvents (bool enabled) |
void | setAcceptTouchEvents (bool enabled) |
void | setActive (bool active) |
void | setBoundingRegionGranularity (qreal granularity) |
void | setCacheMode (CacheMode mode, const QSize &logicalCacheSize) |
void | setCursor (const QCursor &cursor) |
void | setData (int key, const QVariant &value) |
void | setEnabled (bool enabled) |
void | setFiltersChildEvents (bool enabled) |
void | setFlag (GraphicsItemFlag flag, bool enabled) |
void | setFlags (QFlags< QGraphicsItem::GraphicsItemFlag > flags) |
void | setFocus (Qt::FocusReason focusReason) |
void | setFocusProxy (QGraphicsItem *item) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setGroup (QGraphicsItemGroup *group) |
void | setHandlesChildEvents (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setMatrix (const QMatrix &matrix, bool combine) |
void | setOpacity (qreal opacity) |
void | setPanelModality (PanelModality panelModality) |
void | setParentItem (QGraphicsItem *newParent) |
void | setPos (const QPointF &pos) |
void | setPos (qreal x, qreal y) |
void | setRotation (qreal angle) |
void | setScale (qreal factor) |
void | setSelected (bool selected) |
void | setToolTip (const QString &toolTip) |
void | setTransform (const QTransform &matrix, bool combine) |
void | setTransformations (const QList< QGraphicsTransform * > &transformations) |
void | setTransformOriginPoint (qreal x, qreal y) |
void | setTransformOriginPoint (const QPointF &origin) |
void | setVisible (bool visible) |
void | setX (qreal x) |
void | setY (qreal y) |
void | setZValue (qreal z) |
void | shear (qreal sh, qreal sv) |
void | show () |
void | stackBefore (const QGraphicsItem *sibling) |
QGraphicsObject * | toGraphicsObject () |
const QGraphicsObject * | toGraphicsObject () const |
QString | toolTip () const |
QGraphicsItem * | topLevelItem () const |
QGraphicsWidget * | topLevelWidget () const |
QTransform | transform () const |
QList< QGraphicsTransform * > | transformations () const |
QPointF | transformOriginPoint () const |
void | translate (qreal dx, qreal dy) |
void | ungrabKeyboard () |
void | ungrabMouse () |
void | unsetCursor () |
void | update (qreal x, qreal y, qreal width, qreal height) |
void | update (const QRectF &rect) |
QGraphicsWidget * | window () const |
qreal | x () const |
qreal | y () const |
qreal | zValue () const |
![]() | |
KGameRendererClient (KGameRenderer *renderer, const QString &spriteKey) | |
virtual | ~KGameRendererClient () |
QHash< QColor, QColor > | customColors () const |
int | frame () const |
int | frameCount () const |
KGameRenderer * | renderer () const |
QSize | renderSize () const |
void | setCustomColors (const QHash< QColor, QColor > &customColors) |
void | setFrame (int frame) |
void | setRenderSize (const QSize &renderSize) |
void | setSpriteKey (const QString &spriteKey) |
QString | spriteKey () const |
Additional Inherited Members | |
![]() | |
typedef | GraphicsItemFlags |
Detailed Description
A QGraphicsPixmapItem which reacts to theme changes automatically.
- Since
- 4.6 This class is a QGraphicsPixmapItem which retrieves its pixmap from a KGameRenderer, and updates it automatically when the KGameRenderer changes the theme.
Definition at line 39 of file kgamerendereditem.h.
Constructor & Destructor Documentation
KGameRenderedItem::KGameRenderedItem | ( | KGameRenderer * | renderer, |
const QString & | spriteKey, | ||
QGraphicsItem * | parent = 0 |
||
) |
Creates a new KGameRenderedItem which renders the sprite with the given spriteKey as provided by the given renderer.
Definition at line 26 of file kgamerendereditem.cpp.
|
virtual |
Definition at line 34 of file kgamerendereditem.cpp.
Member Function Documentation
|
protectedvirtual |
This method is called when the KGameRenderer has provided a new pixmap for this client (esp.
after theme changes and after calls to setFrame(), setRenderSize() and setSpriteKey()).
Implements KGameRendererClient.
Definition at line 39 of file kgamerendereditem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:42 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.