libkdegames/libkdegamesprivate
#include <KGameSvgDocument>
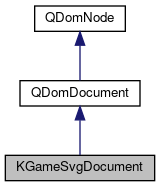
Public Types | |
enum | MatrixOption { ApplyToCurrentMatrix = 0x01, ReplaceCurrentMatrix = 0x02 } |
typedef QFlags< MatrixOption > | MatrixOptions |
enum | StylePropertySortOption { Unsorted = 0x01, UseInkscapeOrder = 0x02 } |
typedef QFlags < StylePropertySortOption > | StylePropertySortOptions |
Detailed Description
A class for manipulating an SVG file using DOM.
This class is a wrapper around QDomDocument for SVG files. It:
- implements elementById();
- manipulates a node's style properties; and,
- manipulates a node's transform properties.
- Note
- The DOM standard requires all changes to be "live", so we cannot cache any values from the file; instead, we always have to query the DOM for the current value. This also means that style & matrix changes we make happen to the DOM immediately.
A typical use is to read in an SVG file, edit the style or transform attributes in DOM as desired, and then output a QByteArray suitable for being loaded with QSvgRenderer::load().
To read an SVG file into DOM:
To find a node with a specific value in its id attribute, for example where id="playerOneGamepiece":
Most methods operate on the last node found by elementById()
or elementByUniqueAttributeValue()
. If the methods are working on the wrong node, then you are mistaken about which node was the last node (or you found a bug). Try calling setCurrentNode()
with the node you are wanting to modify to see if this is the issue. Consider the following code for example:
To skew the currentNode()
:
- Warning
- Be careful when using the KGameSvgDocument::ApplyToCurrentMatrix flag. It multiplies the matrices, so if you repeatedly apply the same matrix to a node, you have a polynomial series
x^2
, and you will very quickly run into overflow issues.
To output currentNode()
to be rendered:
To output the whole document to be rendered (See QDomDocument::toByteArray()):
- See also
- QDomDocument, QSvgRenderer
- Version
- 0.1
- Todo:
- Add convenience functions for getting/setting individual style properties. I haven't completely convinced myself of the utility of this, so don't hold your breathe. ;-)
Definition at line 115 of file kgamesvgdocument.h.
Member Typedef Documentation
Q_DECLARE_FLAGS macro confuses doxygen, so create typedef's manually.
Definition at line 152 of file kgamesvgdocument.h.
Q_DECLARE_FLAGS macro confuses doxygen, so create typedef's manually.
Definition at line 168 of file kgamesvgdocument.h.
Member Enumeration Documentation
Options for applying (multiplying) or replacing the current matrix.
Enumerator | |
---|---|
ApplyToCurrentMatrix |
Apply to current matrix. |
ReplaceCurrentMatrix |
Replace the current matrix. |
Definition at line 141 of file kgamesvgdocument.h.
Options for sorting style properties when building a style attribute.
Enumerator | |
---|---|
Unsorted |
When building a style attribute, do not sort. |
UseInkscapeOrder |
When building a style attribute, sort properties the same way Inkscape does. |
Definition at line 157 of file kgamesvgdocument.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
Definition at line 144 of file kgamesvgdocument.cpp.
KGameSvgDocument::KGameSvgDocument | ( | const KGameSvgDocument & | doc | ) |
Copy Constructor.
Definition at line 148 of file kgamesvgdocument.cpp.
|
virtual |
Destructor.
Definition at line 153 of file kgamesvgdocument.cpp.
Member Function Documentation
QDomNode KGameSvgDocument::currentNode | ( | ) | const |
Returns the last node found by elementById, or null if node not found.
- Returns
- The current node
- See also
- setCurrentNode()
Definition at line 304 of file kgamesvgdocument.cpp.
QDomNode KGameSvgDocument::def | ( | ) | const |
Returns the first def in the document.
- Returns
- The first def in the document
Definition at line 426 of file kgamesvgdocument.cpp.
QDomNodeList KGameSvgDocument::defs | ( | ) | const |
Returns the defs in the document.
- Returns
- The defs in the document
Definition at line 421 of file kgamesvgdocument.cpp.
Returns a node with the given id.
This is a convenience function. We call elementByUniqueAttributeValue(), but we assume that the name of the attribute is "id". This assumption will be correct for valid SVG files.
Returns the element whose ID is equal to elementId. If no element with the ID was found, this function returns a null element.
- Parameters
-
attributeValue The value of the id attribute to find
- Returns
- the matching node, or a null node if no matching node found
- See also
- elementByUniqueAttributeValue()
Definition at line 178 of file kgamesvgdocument.cpp.
QDomNode KGameSvgDocument::elementByUniqueAttributeValue | ( | const QString & | attributeName, |
const QString & | attributeValue | ||
) |
Returns the node with the given value for the given attribute.
Returns the element whose attribute given in attributeName
is equal to the value given in attributeValue
.
QDomDocument::elementById() always returns a null node because TT says they can't know which attribute is the id attribute. Here, we allow the id attribute to be specified.
This function also sets m_currentNode
to this node.
- Parameters
-
attributeName The name of the identifing attribute, such as "id" to find. attributeValue The value to look for in the attribute attributeName
The values held in this attribute must be unique in the document, or the consequences may be unpredictably incorrect. You've been warned. ;-)
- Returns
- the matching node, or a null node if no matching node found
Definition at line 165 of file kgamesvgdocument.cpp.
QDomNodeList KGameSvgDocument::linearGradients | ( | ) | const |
Returns the linearGradients in the document.
- Returns
- The linearGradients in the document
Definition at line 411 of file kgamesvgdocument.cpp.
void KGameSvgDocument::load | ( | ) |
Reads the SVG file svgFilename() into DOM.
- Returns
- nothing
Definition at line 183 of file kgamesvgdocument.cpp.
void KGameSvgDocument::load | ( | const QString & | svgFilename | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This function permits specifying the svg file and loading it at once.
- Parameters
-
svgFilename The filename of the SVG file to open.
- Returns
- nothing
Definition at line 222 of file kgamesvgdocument.cpp.
QByteArray KGameSvgDocument::nodeToByteArray | ( | ) | const |
Builds a new svg document and returns a QByteArray suitable for passing to QSvgRenderer::load().
Internally, we call nodeToSvg()
and then convert to a QByteArray, so this method should be called instead of nodeToSvg()
.
- Returns
- the QByteArray
Definition at line 391 of file kgamesvgdocument.cpp.
QString KGameSvgDocument::nodeToSvg | ( | ) | const |
Returns the current node and it's children as a new xml svg document.
- Returns
- The xml for the new svg document
Definition at line 340 of file kgamesvgdocument.cpp.
KGameSvgDocument & KGameSvgDocument::operator= | ( | const KGameSvgDocument & | doc | ) |
Assignment Operator.
Definition at line 158 of file kgamesvgdocument.cpp.
QDomNodeList KGameSvgDocument::patterns | ( | ) | const |
Returns the patterns in the document.
- Returns
- The patterns in the document
Definition at line 406 of file kgamesvgdocument.cpp.
QDomNodeList KGameSvgDocument::radialGradients | ( | ) | const |
Returns the radialGradients in the document.
- Returns
- The radialGradients in the document
Definition at line 416 of file kgamesvgdocument.cpp.
void KGameSvgDocument::rotate | ( | double | degrees, |
const MatrixOptions & | options = ApplyToCurrentMatrix |
||
) |
Rotates the origin of the current node counterclockwise.
- Parameters
-
degrees The amount in degrees to rotate by. options Apply to current matrix or replace current matrix.
- Returns
- nothing
- See also
- QMatrix::rotate()
Definition at line 228 of file kgamesvgdocument.cpp.
void KGameSvgDocument::scale | ( | double | xFactor, |
double | yFactor, | ||
const MatrixOptions & | options = ApplyToCurrentMatrix |
||
) |
Scales the origin of the current node.
- Note
- Neither
xFactor
noryFactor
may be zero, otherwise you scale the element into nonexistence.
- Parameters
-
xFactor The factor to scale the x-axis by. yFactor The factor to scale the y-axis by. options Apply to current matrix or replace current matrix.
- Returns
- nothing
- See also
- QMatrix::scale()
Definition at line 284 of file kgamesvgdocument.cpp.
void KGameSvgDocument::setCurrentNode | ( | const QDomNode & | node | ) |
Sets the current node.
- Parameters
-
node The node to set currentNode to.
- Returns
- nothing
- See also
- currentNode()
Definition at line 309 of file kgamesvgdocument.cpp.
void KGameSvgDocument::setStyle | ( | const QString & | styleAttribute | ) |
Sets the style attribute of the current node.
Unless you are parsing your own style attribute for some reason, you probably want to use setStyleProperty() or setStyleProperties().
- Parameters
-
styleAttribute The style attribute to apply.
- Returns
- nothing
Definition at line 401 of file kgamesvgdocument.cpp.
void KGameSvgDocument::setStyleProperties | ( | const QHash< QString, QString > & | _styleProperties, |
const StylePropertySortOptions & | options = Unsorted |
||
) |
Sets the style properties of the current node.
The only(?) reason to set useInkscapeOrder to true is if you are saving the svg xml to a file that may be human-edited later, for consistency. There is a performance hit, since hashes store their data unsorted.
- Parameters
-
_styleProperties The hash of style properties to apply. options Apply the hash so the properties are in the same order as Inkscape writes them.
- Returns
- nothing
- See also
- styleProperties()
Definition at line 468 of file kgamesvgdocument.cpp.
void KGameSvgDocument::setStyleProperty | ( | const QString & | propertyName, |
const QString & | propertyValue | ||
) |
Sets the value of the style property given for the current node.
- Note
- Internally, we create a hash with
styleProperties
, then update thepropertyName
topropertyValue
, before finally applying the hash to DOM viasetStyleProperties()
. Because of this, if you need to set multiple properties per node, it will be more efficient to callstyleProperties()
, modify the hash it returns, and then apply the hash withsetStyleProperties()
.
- Parameters
-
propertyName The name of the property to set. propertyValue The value of the property to set.
- Returns
- nothing
Definition at line 330 of file kgamesvgdocument.cpp.
void KGameSvgDocument::setSvgFilename | ( | const QString & | svgFilename | ) |
Sets the current SVG filename.
- Parameters
-
svgFilename The filename of the SVG file to open.
- Returns
- nothing
- See also
- svgFilename()
Definition at line 320 of file kgamesvgdocument.cpp.
void KGameSvgDocument::setTransform | ( | const QString & | transformAttribute | ) |
Sets the transform attribute of the current node.
As this function works on QStrings, it replaces the existing transform attribute. If you need to multiply, use setTransformMatrix() instead.
- Parameters
-
transformAttribute The transform attribute to apply.
- Returns
- nothing
- See also
- transform(), transformMatrix(), setTransformMatrix()
Definition at line 436 of file kgamesvgdocument.cpp.
void KGameSvgDocument::setTransformMatrix | ( | QMatrix & | matrix, |
const MatrixOptions & | options = ApplyToCurrentMatrix |
||
) |
Sets the transform attribute of the current node.
- Parameters
-
matrix The matrix to apply. options Should we apply matrix to the current matrix? We modify matrix internally if options
includes ApplyToCurrentMatrix, so it can't be passed as const. Normally we want to apply the existing matrix. If we apply the matrix, we potentially end up squaring with each call, e.g. x^2.
- Returns
- nothing
- See also
- transformMatrix()
Definition at line 622 of file kgamesvgdocument.cpp.
void KGameSvgDocument::shear | ( | double | xRadians, |
double | yRadians, | ||
const MatrixOptions & | options = ApplyToCurrentMatrix |
||
) |
Shears the origin of the current node.
- Parameters
-
xRadians The amount in radians to shear (skew) the x-axis by. yRadians The amount in radians to shear (skew) the y-axis by. options Apply to current matrix or replace current matrix.
- Returns
- nothing
- See also
- QMatrix::shear()
Definition at line 260 of file kgamesvgdocument.cpp.
void KGameSvgDocument::skew | ( | double | xDegrees, |
double | yDegrees, | ||
const MatrixOptions & | options = ApplyToCurrentMatrix |
||
) |
Skews the origin of the current node.
This is a convenience function. It simply coverts it's arguments to radians, then calls shear().
- Parameters
-
xDegrees The amount in degrees to shear (skew) the x-axis by. yDegrees The amount in degrees to shear (skew) the y-axis by. options Apply to current matrix or replace current matrix.
- Returns
- nothing
- See also
- shear()
Definition at line 276 of file kgamesvgdocument.cpp.
QString KGameSvgDocument::style | ( | ) | const |
Returns the style attribute of the current node.
Unless you are parsing your own style attribute for some reason, you probably want to use styleProperty() or styleProperties().
- Returns
- The style atttibute.
- See also
- styleProperty() styleProperties()
Definition at line 396 of file kgamesvgdocument.cpp.
Returns a hash of the style properties of the current node.
- Returns
- The style properties.
- See also
- setStyleProperties()
Definition at line 441 of file kgamesvgdocument.cpp.
Returns the value of the style property given for the current node.
- Note
- Internally, we create a hash with
styleProperties
, then return the value of thepropertyName
property. As such, if you need the values of multiple properties, it will be more efficient to callstyleProperties()
and then use the hash directly.
See KGameSvgDocumentPrivate::m_inkscapeOrder for a list of common SVG style properties
- Parameters
-
propertyName the name of the property to return
- Returns
- The value style property given, or null if no such property for this node.
Definition at line 325 of file kgamesvgdocument.cpp.
QString KGameSvgDocument::svgFilename | ( | ) | const |
Returns the name of the SVG file this DOM represents.
- Returns
- The current filename.
- See also
- setSvgFilename()
Definition at line 315 of file kgamesvgdocument.cpp.
QString KGameSvgDocument::transform | ( | ) | const |
Returns the transform attribute of the current node.
- Returns
- The transform atttibute.
Definition at line 431 of file kgamesvgdocument.cpp.
QMatrix KGameSvgDocument::transformMatrix | ( | ) | const |
Returns the transform attribute of the current node as a matrix.
- Returns
- The matrix for the transform atttibute.
- See also
- setTransformMatrix()
Definition at line 508 of file kgamesvgdocument.cpp.
void KGameSvgDocument::translate | ( | int | xPixels, |
int | yPixels, | ||
const MatrixOptions & | options = ApplyToCurrentMatrix |
||
) |
Moves the origin of the current node.
- Parameters
-
xPixels The number of pixels to move the x-axis by. yPixels The number of pixels to move the y-axis by. options Apply to current matrix or replace current matrix.
- Returns
- nothing
- See also
- QMatrix::translate()
Definition at line 244 of file kgamesvgdocument.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:50 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.