KDE3Support
#include <k3textedit.h>
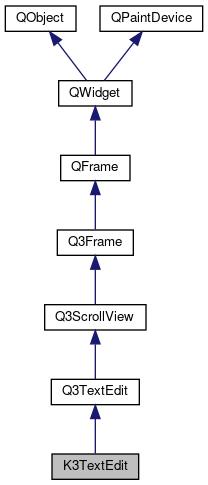
Public Slots | |
void | checkSpelling () |
Public Member Functions | |
K3TextEdit (const QString &text, const QString &context=QString(), QWidget *parent=0, const char *name=0) | |
K3TextEdit (QWidget *parent=0L, const char *name=0) | |
~K3TextEdit () | |
bool | checkSpellingEnabled () const |
void | highLightWord (unsigned int length, unsigned int pos) |
void | setCheckSpellingEnabled (bool check) |
virtual void | setPalette (const QPalette &palette) |
virtual void | setReadOnly (bool readOnly) |
![]() | |
Q3TextEdit (const QString &text, const QString &context, QWidget *parent, const char *name) | |
Q3TextEdit (QWidget *parent, const char *name) | |
virtual | ~Q3TextEdit () |
int | alignment () const |
QString | anchorAt (const QPoint &pos, Qt::AnchorAttribute attr) |
virtual void | append (const QString &text) |
AutoFormatting | autoFormatting () const |
bool | bold () const |
int | charAt (const QPoint &pos, int *para) const |
virtual void | clear () |
virtual void | clearParagraphBackground (int para) |
void | clicked (int para, int pos) |
QColor | color () const |
QString | context () const |
virtual void | copy () |
void | copyAvailable (bool yes) |
void | currentAlignmentChanged (int a) |
void | currentColorChanged (const QColor &c) |
QFont | currentFont () const |
void | currentFontChanged (const QFont &f) |
void | currentVerticalAlignmentChanged (Q3TextEdit::VerticalAlignment a) |
void | cursorPositionChanged (int para, int pos) |
virtual void | cut () |
virtual void | del () |
QString | documentTitle () const |
virtual void | doKeyboardAction (Q3TextEdit::KeyboardAction action) |
void | doubleClicked (int para, int pos) |
virtual void | ensureCursorVisible () |
virtual bool | eventFilter (QObject *o, QEvent *e) |
QString | family () const |
virtual bool | find (const QString &expr, bool cs, bool wo, bool forward, int *para, int *index) |
QFont | font () const |
void | getCursorPosition (int *para, int *index) const |
void | getSelection (int *paraFrom, int *indexFrom, int *paraTo, int *indexTo, int selNum) const |
bool | hasSelectedText () const |
virtual int | heightForWidth (int w) const |
virtual void | insert (const QString &text, bool indent, bool checkNewLine, bool removeSelected) |
virtual void | insert (const QString &text, uint insertionFlags) |
virtual void | insertAt (const QString &text, int para, int index) |
virtual void | insertParagraph (const QString &text, int para) |
bool | isModified () const |
bool | isOverwriteMode () const |
bool | isReadOnly () const |
bool | isRedoAvailable () const |
bool | isUndoAvailable () const |
bool | isUndoRedoEnabled () const |
bool | italic () const |
int | length () const |
int | lineOfChar (int para, int index) |
int | lines () const |
int | linesOfParagraph (int para) const |
bool | linkUnderline () const |
Q3MimeSourceFactory * | mimeSourceFactory () const |
void | modificationChanged (bool m) |
virtual void | moveCursor (Q3TextEdit::CursorAction action, bool select) |
QBrush | paper () const |
int | paragraphAt (const QPoint &pos) const |
QColor | paragraphBackgroundColor (int para) const |
int | paragraphLength (int para) const |
QRect | paragraphRect (int para) const |
int | paragraphs () const |
virtual void | paste () |
virtual void | pasteSubType (const QByteArray &subtype) |
virtual void | placeCursor (const QPoint &pos, Q3TextCursor *c) |
int | pointSize () const |
virtual void | redo () |
void | redoAvailable (bool yes) |
virtual void | removeParagraph (int para) |
virtual void | removeSelectedText (int selNum) |
virtual void | removeSelection (int selNum) |
void | returnPressed () |
virtual void | scrollToAnchor (const QString &name) |
virtual void | scrollToBottom () |
virtual void | selectAll (bool select) |
QString | selectedText () const |
void | selectionChanged () |
virtual void | setAlignment (int a) |
void | setAutoFormatting (QFlags< Q3TextEdit::AutoFormattingFlag >) |
virtual void | setBold (bool b) |
virtual void | setColor (const QColor &c) |
virtual void | setCurrentFont (const QFont &f) |
virtual void | setCursorPosition (int para, int index) |
virtual void | setFamily (const QString &fontFamily) |
virtual void | setItalic (bool b) |
virtual void | setLinkUnderline (bool) |
virtual void | setMimeSourceFactory (Q3MimeSourceFactory *factory) |
virtual void | setModified (bool m) |
virtual void | setOverwriteMode (bool b) |
virtual void | setPaper (const QBrush &pap) |
virtual void | setParagraphBackgroundColor (int para, const QColor &bg) |
virtual void | setPointSize (int s) |
virtual void | setSelection (int paraFrom, int indexFrom, int paraTo, int indexTo, int selNum) |
virtual void | setSelectionAttributes (int selNum, const QColor &back, bool invertText) |
virtual void | setStyleSheet (Q3StyleSheet *styleSheet) |
virtual void | setTabChangesFocus (bool b) |
virtual void | setTabStopWidth (int ts) |
void | setText (const QString &txt) |
virtual void | setText (const QString &text, const QString &context) |
virtual void | setTextFormat (Qt::TextFormat f) |
virtual void | setUnderline (bool b) |
virtual void | setUndoDepth (int d) |
virtual void | setUndoRedoEnabled (bool b) |
virtual void | setVerticalAlignment (Q3TextEdit::VerticalAlignment a) |
virtual void | setWordWrap (Q3TextEdit::WordWrap mode) |
virtual void | setWrapColumnOrWidth (int) |
virtual void | setWrapPolicy (Q3TextEdit::WrapPolicy policy) |
virtual QSize | sizeHint () const |
Q3StyleSheet * | styleSheet () const |
virtual void | sync () |
Q3SyntaxHighlighter * | syntaxHighlighter () const |
bool | tabChangesFocus () const |
int | tabStopWidth () const |
QString | text (int para) const |
QString | text () const |
void | textChanged () |
Qt::TextFormat | textFormat () const |
bool | underline () const |
virtual void | undo () |
void | undoAvailable (bool yes) |
int | undoDepth () const |
VerticalAlignment | verticalAlignment () const |
WordWrap | wordWrap () const |
int | wrapColumnOrWidth () const |
WrapPolicy | wrapPolicy () const |
virtual void | zoomIn (int range) |
virtual void | zoomIn () |
virtual void | zoomOut () |
virtual void | zoomOut (int range) |
virtual void | zoomTo (int size) |
![]() | |
Q3ScrollView (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~Q3ScrollView () | |
virtual void | addChild (QWidget *child, int x, int y) |
int | bottomMargin () const |
void | center (int x, int y) |
void | center (int x, int y, float xmargin, float ymargin) |
bool | childIsVisible (QWidget *child) |
int | childX (QWidget *child) |
int | childY (QWidget *child) |
QWidget * | clipper () const |
int | contentsHeight () const |
void | contentsMoving (int x, int y) |
void | contentsToViewport (int x, int y, int &vx, int &vy) const |
QPoint | contentsToViewport (const QPoint &p) const |
int | contentsWidth () const |
int | contentsX () const |
int | contentsY () const |
QWidget * | cornerWidget () const |
bool | dragAutoScroll () const |
void | enableClipper (bool y) |
void | ensureVisible (int x, int y) |
void | ensureVisible (int x, int y, int xmargin, int ymargin) |
bool | hasStaticBackground () const |
QScrollBar * | horizontalScrollBar () const |
void | horizontalSliderPressed () |
void | horizontalSliderReleased () |
ScrollBarMode | hScrollBarMode () const |
bool | isHorizontalSliderPressed () |
bool | isVerticalSliderPressed () |
int | leftMargin () const |
virtual QSize | minimumSizeHint () const |
virtual void | moveChild (QWidget *child, int x, int y) |
void | removeChild (QWidget *child) |
void | repaintContents (const QRect &r, bool erase) |
void | repaintContents (int x, int y, int w, int h, bool erase) |
void | repaintContents (bool erase) |
virtual void | resizeContents (int w, int h) |
ResizePolicy | resizePolicy () const |
int | rightMargin () const |
void | scrollBy (int dx, int dy) |
virtual void | setContentsPos (int x, int y) |
virtual void | setCornerWidget (QWidget *corner) |
virtual void | setDragAutoScroll (bool b) |
virtual void | setHScrollBarMode (ScrollBarMode) |
virtual void | setMargins (int left, int top, int right, int bottom) |
virtual void | setResizePolicy (ResizePolicy) |
void | setStaticBackground (bool y) |
virtual void | setVisible (bool visible) |
virtual void | setVScrollBarMode (ScrollBarMode) |
void | showChild (QWidget *child, bool y) |
int | topMargin () const |
void | updateContents (const QRect &r) |
void | updateContents (int x, int y, int w, int h) |
void | updateContents () |
void | updateScrollBars () |
QScrollBar * | verticalScrollBar () const |
void | verticalSliderPressed () |
void | verticalSliderReleased () |
QWidget * | viewport () const |
QSize | viewportSize (int x, int y) const |
QPoint | viewportToContents (const QPoint &vp) const |
void | viewportToContents (int vx, int vy, int &x, int &y) const |
int | visibleHeight () const |
int | visibleWidth () const |
ScrollBarMode | vScrollBarMode () const |
![]() | |
Q3Frame (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~Q3Frame () | |
QRect | contentsRect () const |
int | frameWidth () const |
int | margin () const |
void | setMargin (int) |
![]() | |
QFrame (QWidget *parent, QFlags< Qt::WindowType > f) | |
QFrame (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QFrame () | |
QRect | frameRect () const |
Shadow | frameShadow () const |
Shape | frameShape () const |
int | frameStyle () const |
int | frameWidth () const |
int | lineWidth () const |
int | midLineWidth () const |
void | setFrameRect (const QRect &) |
void | setFrameShadow (Shadow) |
void | setFrameShape (Shape) |
void | setFrameStyle (int style) |
void | setLineWidth (int) |
void | setMidLineWidth (int) |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Protected Member Functions | |
virtual void | contentsWheelEvent (QWheelEvent *) |
virtual Q3PopupMenu * | createPopupMenu (const QPoint &pos) |
virtual Q3PopupMenu * | createPopupMenu () |
virtual void | deleteWordBack () |
virtual void | deleteWordForward () |
virtual void | focusInEvent (QFocusEvent *) |
virtual void | keyPressEvent (QKeyEvent *) |
virtual void | virtual_hook (int id, void *data) |
![]() | |
virtual void | changeEvent (QEvent *ev) |
virtual void | contentsContextMenuEvent (QContextMenuEvent *e) |
virtual void | contentsDragEnterEvent (QDragEnterEvent *e) |
virtual void | contentsDragLeaveEvent (QDragLeaveEvent *e) |
virtual void | contentsDragMoveEvent (QDragMoveEvent *e) |
virtual void | contentsDropEvent (QDropEvent *e) |
virtual void | contentsMouseDoubleClickEvent (QMouseEvent *e) |
virtual void | contentsMouseMoveEvent (QMouseEvent *e) |
virtual void | contentsMousePressEvent (QMouseEvent *e) |
virtual void | contentsMouseReleaseEvent (QMouseEvent *e) |
virtual void | drawContents (QPainter *p, int cx, int cy, int cw, int ch) |
virtual bool | event (QEvent *e) |
virtual bool | focusNextPrevChild (bool n) |
virtual void | inputMethodEvent (QInputMethodEvent *e) |
void | repaintChanged () |
virtual void | resizeEvent (QResizeEvent *e) |
Q3TextCursor * | textCursor () const |
virtual void | viewportResizeEvent (QResizeEvent *e) |
![]() | |
virtual void | contextMenuEvent (QContextMenuEvent *e) |
virtual void | drawContentsOffset (QPainter *p, int offsetx, int offsety, int clipx, int clipy, int clipw, int cliph) |
virtual void | frameChanged () |
virtual void | mouseDoubleClickEvent (QMouseEvent *e) |
virtual void | mouseMoveEvent (QMouseEvent *e) |
virtual void | mousePressEvent (QMouseEvent *e) |
virtual void | mouseReleaseEvent (QMouseEvent *e) |
virtual void | setHBarGeometry (QScrollBar &hbar, int x, int y, int w, int h) |
virtual void | setVBarGeometry (QScrollBar &vbar, int x, int y, int w, int h) |
virtual void | viewportPaintEvent (QPaintEvent *pe) |
virtual void | wheelEvent (QWheelEvent *e) |
![]() | |
virtual void | drawContents (QPainter *painter) |
virtual void | drawFrame (QPainter *p) |
virtual void | paintEvent (QPaintEvent *event) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEvent *event) |
bool | focusNextChild () |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
Detailed Description
A KDE'ified Q3TextEdit.
This is just a little subclass of Q3TextEdit, implementing some standard KDE features, like Cursor auto-hiding, configurable wheelscrolling (fast-scroll or zoom) and deleting of entire words with Ctrl-Backspace or Ctrl-Delete.
Basic rule: whenever you want to use Q3TextEdit, use K3TextEdit!
- See also
- Q3TextEdit
Definition at line 42 of file k3textedit.h.
Constructor & Destructor Documentation
K3TextEdit::K3TextEdit | ( | const QString & | text, |
const QString & | context = QString() , |
||
QWidget * | parent = 0 , |
||
const char * | name = 0 |
||
) |
Constructs a K3TextEdit object.
See Q3TextEdit::Q3TextEdit for details.
Definition at line 55 of file k3textedit.cpp.
K3TextEdit::K3TextEdit | ( | QWidget * | parent = 0L , |
const char * | name = 0 |
||
) |
Constructs a K3TextEdit object.
See Q3TextEdit::Q3TextEdit for details.
Definition at line 63 of file k3textedit.cpp.
K3TextEdit::~K3TextEdit | ( | ) |
Destroys the K3TextEdit object.
Definition at line 70 of file k3textedit.cpp.
Member Function Documentation
|
slot |
Create a modal dialog to check the spelling.
This slot will not return until spell checking has been completed.
Definition at line 345 of file k3textedit.cpp.
bool K3TextEdit::checkSpellingEnabled | ( | ) | const |
Returns true if spell checking is enabled for this text edit.
- See also
- setCheckSpellingEnabled()
Definition at line 305 of file k3textedit.cpp.
|
protectedvirtual |
Reimplemented to allow fast-wheelscrolling with Ctrl-Wheel or zoom.
Reimplemented from Q3TextEdit.
Definition at line 254 of file k3textedit.cpp.
|
protectedvirtual |
Reimplemented from Q3TextEdit to add spelling related items when appropriate.
Reimplemented from Q3TextEdit.
Definition at line 214 of file k3textedit.cpp.
|
protectedvirtual |
This is just a reimplementation of a deprecated method from Q3TextEdit and is just here to keep source compatibility.
This should not be used in new code. Specifically reimplementing this method will probably not do what you expect. See the method above.
Reimplemented from Q3TextEdit.
Definition at line 249 of file k3textedit.cpp.
|
protectedvirtual |
Deletes a word backwards from the current cursor position, if available.
Definition at line 195 of file k3textedit.cpp.
|
protectedvirtual |
Deletes a word forwards from the current cursor position, if available.
Definition at line 202 of file k3textedit.cpp.
|
protectedvirtual |
Reimplemented to instantiate a KDictSpellingHighlighter, if spellchecking is enabled.
Reimplemented from QWidget.
Definition at line 297 of file k3textedit.cpp.
void K3TextEdit::highLightWord | ( | unsigned int | length, |
unsigned int | pos | ||
) |
Definition at line 406 of file k3textedit.cpp.
|
protectedvirtual |
Reimplemented to catch "delete word" key events.
Reimplemented from Q3TextEdit.
Definition at line 75 of file k3textedit.cpp.
void K3TextEdit::setCheckSpellingEnabled | ( | bool | check | ) |
Turns spell checking for this text edit on or off.
- See also
- checkSpellingEnabled()
Definition at line 275 of file k3textedit.cpp.
|
virtual |
Reimplemented for tracking custom palettes.
Definition at line 262 of file k3textedit.cpp.
|
virtual |
Reimplemented to set a proper "deactivated" background color.
Reimplemented from Q3TextEdit.
Definition at line 310 of file k3textedit.cpp.
|
protectedvirtual |
Definition at line 342 of file k3textedit.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:48 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.