KDECore
#include <kautostart.h>
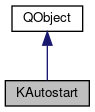
Public Types | |
enum | Condition { NoConditions = 0x0, CheckCommand = 0x1, CheckCondition = 0x2, CheckAll = 0xff } |
enum | StartPhase { BaseDesktop = 0, DesktopServices = 1, Applications = 2 } |
Public Member Functions | |
KAutostart (const QString &entryName=QString(), QObject *parent=0) | |
~KAutostart () | |
void | addToAllowedEnvironments (const QString &environment) |
void | addToExcludedEnvironments (const QString &environment) |
QStringList | allowedEnvironments () const |
bool | autostarts (const QString &environment=QString(), Conditions check=NoConditions) const |
bool | checkAllowedEnvironment (const QString &environment) const |
QString | command () const |
QString | commandToCheck () const |
QStringList | excludedEnvironments () const |
void | removeFromAllowedEnvironments (const QString &environment) |
void | removeFromExcludedEnvironments (const QString &environment) |
void | setAllowedEnvironments (const QStringList &environments) |
void | setAutostarts (bool autostart) |
void | setCommand (const QString &command) |
void | setCommandToCheck (const QString &exec) |
void | setExcludedEnvironments (const QStringList &environments) |
void | setStartPhase (StartPhase phase) |
void | setVisibleName (const QString &entryName) |
QString | startAfter () const |
StartPhase | startPhase () const |
QString | visibleName () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static bool | isServiceRegistered (const QString &entryName) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
KAutostart provides a programmatic means to control the state of autostart services on a per-user basis.
This is useful for applications that wish to offer a configurable means to allow the application to be autostarted.
By using this class you future-proof your applications against potential future or platform-specific changes to the autostart mechanism(s).
Typical usage might look like:
Definition at line 49 of file kautostart.h.
Member Enumeration Documentation
Flags for each of the conditions that may affect whether or not a service actually autostarted on login.
Definition at line 80 of file kautostart.h.
Enumerates the various autostart phases that occur during start-up.
Definition at line 103 of file kautostart.h.
Constructor & Destructor Documentation
Creates a new KAutostart object that represents the autostart service "entryName".
If the service already exists in the system then the values associated with that service, such as the executable command, will be loaded as well.
Note that unless this service is explicitly set to autostart, simply creating a KAutostart object will not result in the service being autostarted on next log in.
If no such service is already registered and the command to be executed on startup is not the same as entryName, then you will want to set the associated command with setExec(const QString&)
- See also
- setExec
- Parameters
-
entryName the name used to identify the service. If none is provided then it uses the name registered with KAboutData. parent QObject
Definition at line 75 of file kautostart.cpp.
KAutostart::~KAutostart | ( | ) |
Definition at line 99 of file kautostart.cpp.
Member Function Documentation
void KAutostart::addToAllowedEnvironments | ( | const QString & | environment | ) |
Adds an environment to the list of environments this service may start in.
Definition at line 289 of file kautostart.cpp.
void KAutostart::addToExcludedEnvironments | ( | const QString & | environment | ) |
Adds an environment to the list of environments this service may not be autostarted in.
- See also
- removeFromExcludedEnvironments()
Definition at line 329 of file kautostart.cpp.
QStringList KAutostart::allowedEnvironments | ( | ) | const |
Returns the list of environments (e.g.
"KDE") this service is allowed to start in. Use checkAllowedEnvironment() or autostarts() for actual checks.
This does not take other autostart conditions into account. If any environment is added to the allowed environments list, then only those environments will be allowed to autoload the service. It is not allowed to specify both allowed and excluded environments at the same time.
- See also
- setAllowedEnvironments()
Definition at line 274 of file kautostart.cpp.
bool KAutostart::autostarts | ( | const QString & | environment = QString() , |
Conditions | check = NoConditions |
||
) | const |
Returns whether or not the service represented by entryName in the autostart system is set to autostart at login or not.
- Parameters
-
environment if provided the check will be performed as if being loaded in that environment check autostart conditions to check for (see commandToCheck())
- See also
- setAutostarts()
Definition at line 115 of file kautostart.cpp.
Checks whether autostart is allowed in the given environment, depending on allowedEnvironments() and excludedEnvironments().
- Since
- 4.3
Definition at line 160 of file kautostart.cpp.
QString KAutostart::command | ( | ) | const |
Returns the associated command for this autostart service.
- See also
- setCommand()
Definition at line 175 of file kautostart.cpp.
QString KAutostart::commandToCheck | ( | ) | const |
Returns the executable to check for when attempting to autostart this service.
If the executable is not found in the user's environment, it will not autostart.
- See also
- setCommandToCheck()
Definition at line 210 of file kautostart.cpp.
QStringList KAutostart::excludedEnvironments | ( | ) | const |
Returns the list of environments this service is explicitly not allowed to start in.
Use checkAllowedEnvironment() or autostarts() for actual checks.
This does not take other autostart conditions such as into account. It is not allowed to specify both allowed and excluded environments at the same time.
- See also
- setExcludedEnvironments()
Definition at line 314 of file kautostart.cpp.
Checks whether or not a service by the given name entryName
is registered with the autostart system.
Does not check whether or not it is set to actually autostart or not.
- Parameters
-
entryName the name of the service to check for
Definition at line 205 of file kautostart.cpp.
void KAutostart::removeFromAllowedEnvironments | ( | const QString & | environment | ) |
Removes an environment to the list of environments this service may start in.
- See also
- addToAllowedEnvironments()
Definition at line 301 of file kautostart.cpp.
void KAutostart::removeFromExcludedEnvironments | ( | const QString & | environment | ) |
Removes an environment to the list of environments this service may not be autostarted in.
- See also
- addToExcludedEnvironments()
Definition at line 341 of file kautostart.cpp.
void KAutostart::setAllowedEnvironments | ( | const QStringList & | environments | ) |
Sets the environments this service is allowed to start in.
Definition at line 279 of file kautostart.cpp.
void KAutostart::setAutostarts | ( | bool | autostart | ) |
Sets the given exec to start automatically at login.
- Parameters
-
autostart will register with the autostart facility when true and deregister when false
- See also
- autostarts()
Definition at line 104 of file kautostart.cpp.
void KAutostart::setCommand | ( | const QString & | command | ) |
Set the associated command for this autostart service.
- See also
- command()
Definition at line 180 of file kautostart.cpp.
void KAutostart::setCommandToCheck | ( | const QString & | exec | ) |
Sets the executable to check for the existence of when autostarting this service.
- See also
- commandToCheck()
Definition at line 215 of file kautostart.cpp.
void KAutostart::setExcludedEnvironments | ( | const QStringList & | environments | ) |
Sets the environments this service is not allowed to start in.
Definition at line 319 of file kautostart.cpp.
void KAutostart::setStartPhase | ( | KAutostart::StartPhase | phase | ) |
Sets the service (by name) this service should be started after.
Note that this is KDE specific and may not work in other environments.
- See also
- StartPhase, startPhase()
Definition at line 251 of file kautostart.cpp.
void KAutostart::setVisibleName | ( | const QString & | entryName | ) |
Sets the user-visible name for this autostart service.
- See also
- visibleName()
Definition at line 195 of file kautostart.cpp.
QString KAutostart::startAfter | ( | ) | const |
Returns the name of another service that should be autostarted before this one (if that service would be autostarted).
- Since
- 4.3
Definition at line 354 of file kautostart.cpp.
KAutostart::StartPhase KAutostart::startPhase | ( | ) | const |
Returns the autostart phase this service is started in.
Note that this is KDE specific and may not work in other environments.
- See also
- StartPhase, setStartPhase()
Definition at line 246 of file kautostart.cpp.
QString KAutostart::visibleName | ( | ) | const |
Returns the user-visible name this autostart service is registered as.
- See also
- setVisibleName(), setEntryName()
Definition at line 190 of file kautostart.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.