KDECore
#include <KConfigGroup>
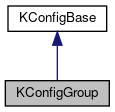
Public Member Functions | |
KConfigGroup () | |
KConfigGroup (KConfigBase *master, const QString &group) | |
KConfigGroup (KConfigBase *master, const char *group) | |
KConfigGroup (const KConfigBase *master, const QString &group) | |
KConfigGroup (const KConfigBase *master, const char *group) | |
KConfigGroup (const KSharedConfigPtr &master, const QString &group) | |
KConfigGroup (const KSharedConfigPtr &master, const char *group) | |
KConfigGroup (const KConfigGroup &) | |
~KConfigGroup () | |
AccessMode | accessMode () const |
void | changeGroup (const QString &group) |
void | changeGroup (const char *group) |
KConfig * | config () |
const KConfig * | config () const |
void | copyTo (KConfigBase *other, WriteConfigFlags pFlags=Normal) const |
void | deleteEntry (const QString &pKey, WriteConfigFlags pFlags=Normal) |
void | deleteEntry (const char *pKey, WriteConfigFlags pFlags=Normal) |
void | deleteGroup (WriteConfigFlags pFlags=Normal) |
QMap< QString, QString > | entryMap () const |
bool | exists () const |
QStringList | groupList () const |
bool | hasDefault (const QString &key) const |
bool | hasDefault (const char *key) const |
bool | hasKey (const QString &key) const |
bool | hasKey (const char *key) const |
bool | isEntryImmutable (const QString &key) const |
bool | isEntryImmutable (const char *key) const |
bool | isImmutable () const |
bool | isValid () const |
QStringList | keyList () const |
void | markAsClean () |
QString | name () const |
KConfigGroup & | operator= (const KConfigGroup &) |
KConfigGroup | parent () const |
template<typename T > | |
T | readEntry (const QString &key, const T &aDefault) const |
template<typename T > | |
T | readEntry (const char *key, const T &aDefault) const |
QVariant | readEntry (const QString &key, const QVariant &aDefault) const |
QVariant | readEntry (const char *key, const QVariant &aDefault) const |
QString | readEntry (const QString &key, const QString &aDefault) const |
QString | readEntry (const char *key, const QString &aDefault) const |
QString | readEntry (const QString &key, const char *aDefault=0) const |
QString | readEntry (const char *key, const char *aDefault=0) const |
QVariantList | readEntry (const QString &key, const QVariantList &aDefault) const |
QVariantList | readEntry (const char *key, const QVariantList &aDefault) const |
QStringList | readEntry (const QString &key, const QStringList &aDefault) const |
QStringList | readEntry (const char *key, const QStringList &aDefault) const |
template<typename T > | |
QList< T > | readEntry (const QString &key, const QList< T > &aDefault) const |
template<typename T > | |
QList< T > | readEntry (const char *key, const QList< T > &aDefault) const |
QString | readEntryUntranslated (const QString &pKey, const QString &aDefault=QString()) const |
QString | readEntryUntranslated (const char *key, const QString &aDefault=QString()) const |
QString | readPathEntry (const QString &pKey, const QString &aDefault) const |
QString | readPathEntry (const char *key, const QString &aDefault) const |
QStringList | readPathEntry (const QString &pKey, const QStringList &aDefault) const |
QStringList | readPathEntry (const char *key, const QStringList &aDefault) const |
QStringList | readXdgListEntry (const QString &pKey, const QStringList &aDefault=QStringList()) const |
QStringList | readXdgListEntry (const char *pKey, const QStringList &aDefault=QStringList()) const |
void | reparent (KConfigBase *parent, WriteConfigFlags pFlags=Normal) |
void | revertToDefault (const QString &key) |
void | revertToDefault (const char *key) |
void | sync () |
void | writeEntry (const QString &key, const QVariant &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const char *key, const QVariant &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const QString &key, const QString &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const char *key, const QString &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const QString &key, const QByteArray &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const char *key, const QByteArray &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const QString &key, const char *value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const char *key, const char *value, WriteConfigFlags pFlags=Normal) |
template<typename T > | |
void | writeEntry (const char *key, const T &value, WriteConfigFlags pFlags=Normal) |
template<typename T > | |
void | writeEntry (const QString &key, const T &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const QString &key, const QStringList &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const char *key, const QStringList &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const QString &key, const QVariantList &value, WriteConfigFlags pFlags=Normal) |
void | writeEntry (const char *key, const QVariantList &value, WriteConfigFlags pFlags=Normal) |
template<typename T > | |
void | writeEntry (const QString &key, const QList< T > &value, WriteConfigFlags pFlags=Normal) |
template<typename T > | |
void | writeEntry (const char *key, const QList< T > &value, WriteConfigFlags pFlags=Normal) |
void | writePathEntry (const QString &pKey, const QString &path, WriteConfigFlags pFlags=Normal) |
void | writePathEntry (const char *pKey, const QString &path, WriteConfigFlags pFlags=Normal) |
void | writePathEntry (const QString &pKey, const QStringList &value, WriteConfigFlags pFlags=Normal) |
void | writePathEntry (const char *pKey, const QStringList &value, WriteConfigFlags pFlags=Normal) |
void | writeXdgListEntry (const QString &pKey, const QStringList &value, WriteConfigFlags pFlags=Normal) |
void | writeXdgListEntry (const char *pKey, const QStringList &value, WriteConfigFlags pFlags=Normal) |
![]() | |
virtual | ~KConfigBase () |
void | deleteGroup (const QByteArray &group, WriteConfigFlags flags=Normal) |
void | deleteGroup (const QString &group, WriteConfigFlags flags=Normal) |
void | deleteGroup (const char *group, WriteConfigFlags flags=Normal) |
KConfigGroup | group (const QByteArray &group) |
KConfigGroup | group (const QString &group) |
KConfigGroup | group (const char *group) |
const KConfigGroup | group (const QByteArray &group) const |
const KConfigGroup | group (const QString &group) const |
const KConfigGroup | group (const char *group) const |
bool | hasGroup (const QString &group) const |
bool | hasGroup (const char *group) const |
bool | hasGroup (const QByteArray &group) const |
bool | isGroupImmutable (const QByteArray &aGroup) const |
bool | isGroupImmutable (const QString &aGroup) const |
bool | isGroupImmutable (const char *aGroup) const |
Protected Member Functions | |
void | deleteGroupImpl (const QByteArray &group, WriteConfigFlags flags) |
KConfigGroup | groupImpl (const QByteArray &b) |
const KConfigGroup | groupImpl (const QByteArray &b) const |
bool | hasGroupImpl (const QByteArray &group) const |
bool | isGroupImmutableImpl (const QByteArray &aGroup) const |
![]() | |
KConfigBase () | |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
enum | AccessMode { NoAccess, ReadOnly, ReadWrite } |
enum | WriteConfigFlag { Persistent = 0x01, Global = 0x02, Localized = 0x04, Normal =Persistent } |
Detailed Description
A class for one specific group in a KConfig object.
If you want to access the top-level entries of a KConfig object, which are not associated with any group, use an empty group name.
A KConfigGroup will be read-only if it is constructed from a const config object or from another read-only group.
Definition at line 53 of file kconfiggroup.h.
Constructor & Destructor Documentation
KConfigGroup::KConfigGroup | ( | ) |
KConfigGroup::KConfigGroup | ( | KConfigBase * | master, |
const QString & | group | ||
) |
Construct a config group corresponding to group
in master
.
This allows the creation of subgroups by passing another group as master
.
group
is the group name encoded in UTF-8.
Definition at line 467 of file kconfiggroup.cpp.
KConfigGroup::KConfigGroup | ( | KConfigBase * | master, |
const char * | group | ||
) |
Overload for KConfigGroup(KConfigBase*,const QString&)
Definition at line 472 of file kconfiggroup.cpp.
KConfigGroup::KConfigGroup | ( | const KConfigBase * | master, |
const QString & | group | ||
) |
Construct a read-only config group.
A read-only group will silently ignore any attempts to write to it.
This allows the creation of subgroups by passing an existing group as master
.
Definition at line 477 of file kconfiggroup.cpp.
KConfigGroup::KConfigGroup | ( | const KConfigBase * | master, |
const char * | group | ||
) |
Overload for KConfigGroup(const KConfigBase*,const QString&)
Definition at line 482 of file kconfiggroup.cpp.
KConfigGroup::KConfigGroup | ( | const KSharedConfigPtr & | master, |
const QString & | group | ||
) |
Overload for KConfigGroup(const KConfigBase*,const QString&)
Definition at line 487 of file kconfiggroup.cpp.
KConfigGroup::KConfigGroup | ( | const KSharedConfigPtr & | master, |
const char * | group | ||
) |
Overload for KConfigGroup(const KConfigBase*,const QString&)
Definition at line 492 of file kconfiggroup.cpp.
KConfigGroup::KConfigGroup | ( | const KConfigGroup & | rhs | ) |
Creates a read-only copy of a read-only group.
Definition at line 503 of file kconfiggroup.cpp.
KConfigGroup::~KConfigGroup | ( | ) |
Definition at line 508 of file kconfiggroup.cpp.
Member Function Documentation
|
virtual |
- Reimplemented from superclass.
Implements KConfigBase.
Definition at line 1188 of file kconfiggroup.cpp.
void KConfigGroup::changeGroup | ( | const QString & | group | ) |
Changes the group of the object.
- Deprecated:
- Create another KConfigGroup from the parent of this group instead.
Definition at line 564 of file kconfiggroup.cpp.
void KConfigGroup::changeGroup | ( | const char * | group | ) |
Overload for changeGroup(const QString&)
- Deprecated:
- Create another KConfigGroup from the parent of this group instead.
Definition at line 573 of file kconfiggroup.cpp.
KConfig * KConfigGroup::config | ( | ) |
Return the config object that this group belongs to.
Definition at line 610 of file kconfiggroup.cpp.
const KConfig * KConfigGroup::config | ( | ) | const |
Return the config object that this group belongs to.
Definition at line 617 of file kconfiggroup.cpp.
void KConfigGroup::copyTo | ( | KConfigBase * | other, |
WriteConfigFlags | pFlags = Normal |
||
) | const |
Copies the entries in this group to another configuration object.
- Note
other
can be either another group or a different file.
- Parameters
-
other the configuration object to copy this group's entries to pFlags the flags to use when writing the entries to the other configuration object
- Since
- 4.1
Definition at line 1220 of file kconfiggroup.cpp.
Deletes the entry specified by pKey
in the current group.
This also hides system wide defaults.
- Parameters
-
pKey the key to delete pFlags the flags to use when deleting this entry
- See also
- deleteGroup(), readEntry(), writeEntry()
Definition at line 1112 of file kconfiggroup.cpp.
void KConfigGroup::deleteEntry | ( | const char * | pKey, |
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for deleteEntry(const QString&, WriteConfigFlags)
Definition at line 1104 of file kconfiggroup.cpp.
void KConfigGroup::deleteGroup | ( | WriteConfigFlags | pFlags = Normal | ) |
Delete all entries in the entire group.
- Parameters
-
pFlags flags passed to KConfig::deleteGroup
- See also
- deleteEntry()
Definition at line 555 of file kconfiggroup.cpp.
|
protectedvirtual |
Implements KConfigBase.
Definition at line 1202 of file kconfiggroup.cpp.
Returns a map (tree) of entries for all entries in this group.
Only the actual entry string is returned, none of the other internal data should be included.
- Returns
- a map of entries in this group, indexed by key
Definition at line 603 of file kconfiggroup.cpp.
bool KConfigGroup::exists | ( | ) | const |
Check whether the containing KConfig object acutally contains a group with this name.
Definition at line 588 of file kconfiggroup.cpp.
|
protectedvirtual |
Implements KConfigBase.
Definition at line 513 of file kconfiggroup.cpp.
|
protectedvirtual |
Implements KConfigBase.
Definition at line 525 of file kconfiggroup.cpp.
|
virtual |
- Reimplemented from superclass.
Implements KConfigBase.
Definition at line 1167 of file kconfiggroup.cpp.
Whether a default is specified for an entry in either the system wide configuration file or the global KDE config file.
If an application computes a default value at runtime for a certain entry, e.g. like:
then it may wish to make the following check before writing back changes:
This ensures that as long as the entry is not modified to differ from the computed default, the application will keep using the computed default and will follow changes the computed default makes over time.
- Parameters
-
key the key of the entry to check
- Returns
true
if the global or system settings files specify a default forkey
in this group,false
otherwise
Definition at line 1139 of file kconfiggroup.cpp.
bool KConfigGroup::hasDefault | ( | const char * | key | ) | const |
Overload for hasDefault(const QString&) const.
Definition at line 1130 of file kconfiggroup.cpp.
|
protectedvirtual |
Implements KConfigBase.
Definition at line 1195 of file kconfiggroup.cpp.
Checks whether the key has an entry in this group.
Use this to determine if a key is not specified for the current group (hasKey() returns false).
If this returns false
for a key, readEntry() (and its variants) will return the default value passed to them.
- Parameters
-
key the key to search for
- Returns
true
if the key is defined in this group by any of the configuration sources,false
otherwise
- See also
- readEntry()
Definition at line 1155 of file kconfiggroup.cpp.
bool KConfigGroup::hasKey | ( | const char * | key | ) | const |
Overload for hasKey(const QString&) const.
Definition at line 1144 of file kconfiggroup.cpp.
Checks if it is possible to change the given entry.
If isImmutable() returns true
, then this method will return true
for all inputs.
- Parameters
-
key the key to check
- Returns
false
if the key may be changed using this configuration group object,true
otherwise
Definition at line 632 of file kconfiggroup.cpp.
bool KConfigGroup::isEntryImmutable | ( | const char * | key | ) | const |
Overload for isEntryImmutable(const QString&) const.
Definition at line 624 of file kconfiggroup.cpp.
|
protectedvirtual |
Implements KConfigBase.
Definition at line 1210 of file kconfiggroup.cpp.
|
virtual |
Whether this group may be changed.
- Returns
false
if the group may be changed,true
otherwise
Implements KConfigBase.
Definition at line 1160 of file kconfiggroup.cpp.
bool KConfigGroup::isValid | ( | ) | const |
Whether the group is valid.
A group is invalid if it was constructed without arguments.
You should not call any functions on an invalid group.
- Returns
true
if the group is valid,false
if it is invalid.
Definition at line 445 of file kconfiggroup.cpp.
QStringList KConfigGroup::keyList | ( | ) | const |
Returns a list of keys this group contains.
Definition at line 1174 of file kconfiggroup.cpp.
|
virtual |
- Reimplemented from superclass.
Implements KConfigBase.
Definition at line 1181 of file kconfiggroup.cpp.
QString KConfigGroup::name | ( | ) | const |
The name of this group.
The root group is named "<default>".
Definition at line 581 of file kconfiggroup.cpp.
KConfigGroup & KConfigGroup::operator= | ( | const KConfigGroup & | rhs | ) |
Definition at line 497 of file kconfiggroup.cpp.
KConfigGroup KConfigGroup::parent | ( | ) | const |
Returns the group that this group belongs to.
- Returns
- the parent group, or an invalid group if this is a top-level group
- Since
- 4.1
Definition at line 538 of file kconfiggroup.cpp.
|
inline |
Reads the value of an entry specified by pKey
in the current group.
This template method makes it possible to write QString foo = readEntry("...", QString("default")); and the same with all other types supported by QVariant.
The return type of the method is simply the same as the type of the default value.
- Note
- readEntry("...", Qt::white) will not compile because Qt::white is an enum. You must turn it into readEntry("...", QColor(Qt::white)).
- Only the following QVariant types are allowed : String, StringList, List, Font, Point, Rect, Size, Color, Int, UInt, Bool, Double, LongLong, ULongLong, DateTime and Date.
- Parameters
-
key The key to search for aDefault A default value returned if the key was not found
- Returns
- The value for this key, or
aDefault
.
- See also
- writeEntry(), deleteEntry(), hasKey()
Definition at line 248 of file kconfiggroup.h.
|
inline |
Overload for readEntry(const QString&, const T&) const.
Definition at line 252 of file kconfiggroup.h.
Reads the value of an entry specified by key
in the current group.
- Parameters
-
key the key to search for aDefault a default value returned if the key was not found
- Returns
- the value for this key, or
aDefault
if the key was not found
- See also
- writeEntry(), deleteEntry(), hasKey()
Definition at line 716 of file kconfiggroup.cpp.
Overload for readEntry(const QString&, const QVariant&)
Definition at line 701 of file kconfiggroup.cpp.
Reads the string value of an entry specified by key
in the current group.
If you want to read a path, please use readPathEntry().
- Parameters
-
key the key to search for aDefault a default value returned if the key was not found
- Returns
- the value for this key, or
aDefault
if the key was not found
- See also
- readPathEntry(), writeEntry(), deleteEntry(), hasKey()
Definition at line 680 of file kconfiggroup.cpp.
Overload for readEntry(const QString&, const QString&)
Definition at line 662 of file kconfiggroup.cpp.
Overload for readEntry(const QString&, const QString&)
Definition at line 657 of file kconfiggroup.cpp.
QString KConfigGroup::readEntry | ( | const char * | key, |
const char * | aDefault = 0 |
||
) | const |
Overload for readEntry(const QString&, const QString&)
Definition at line 652 of file kconfiggroup.cpp.
QVariantList KConfigGroup::readEntry | ( | const QString & | key, |
const QVariantList & | aDefault | ||
) | const |
Overload for readEntry(const QString&, const QStringList&)
- Warning
- This function doesn't convert the items returned to any type. It's actually a list of QVariant::String's. If you want the items converted to a specific type use readEntry(const char*, const QList<T>&) const
Definition at line 736 of file kconfiggroup.cpp.
QVariantList KConfigGroup::readEntry | ( | const char * | key, |
const QVariantList & | aDefault | ||
) | const |
Overload for readEntry(const QString&, const QVariantList&)
Definition at line 721 of file kconfiggroup.cpp.
QStringList KConfigGroup::readEntry | ( | const QString & | key, |
const QStringList & | aDefault | ||
) | const |
Reads a list of strings from the config object.
- Parameters
-
key The key to search for aDefault The default value to use if the key does not exist
- Returns
- The list, or
aDefault
ifkey
does not exist
- See also
- readXdgListEntry(), writeEntry(), deleteEntry(), hasKey()
Definition at line 696 of file kconfiggroup.cpp.
QStringList KConfigGroup::readEntry | ( | const char * | key, |
const QStringList & | aDefault | ||
) | const |
Overload for readEntry(const QString&, const QStringList&)
Definition at line 685 of file kconfiggroup.cpp.
|
inline |
Reads a list of values from the config object.
- Parameters
-
key the key to search for aDefault the default value to use if the key does not exist
- Returns
- the list, or
aDefault
ifkey
does not exist
- See also
- readXdgListEntry(), writeEntry(), deleteEntry(), hasKey()
Definition at line 323 of file kconfiggroup.h.
|
inline |
Overload for readEntry(const QString&, const QList<T>&)
Definition at line 327 of file kconfiggroup.h.
QString KConfigGroup::readEntryUntranslated | ( | const QString & | pKey, |
const QString & | aDefault = QString() |
||
) | const |
Reads an untranslated string entry.
You should not normally need to use this.
- Parameters
-
pKey the key to search for aDefault a default value returned if the key was not found
- Returns
- the value for this key, or
aDefault
if the key does not exist
Definition at line 637 of file kconfiggroup.cpp.
QString KConfigGroup::readEntryUntranslated | ( | const char * | key, |
const QString & | aDefault = QString() |
||
) | const |
Overload for readEntryUntranslated(const QString&, const QString&)
Definition at line 642 of file kconfiggroup.cpp.
Reads a path.
Read the value of an entry specified by pKey
in the current group and interpret it as a path. This means, dollar expansion is activated for this value, so that e.g. $HOME gets expanded.
- Parameters
-
pKey The key to search for. aDefault A default value returned if the key was not found.
- Returns
- The value for this key. Can be QString() if
aDefault
is null.
Definition at line 780 of file kconfiggroup.cpp.
Overload for readPathEntry(const QString&, const QString&)
Definition at line 785 of file kconfiggroup.cpp.
QStringList KConfigGroup::readPathEntry | ( | const QString & | pKey, |
const QStringList & | aDefault | ||
) | const |
Reads a list of paths.
Read the value of an entry specified by pKey
in the current group and interpret it as a list of paths. This means, dollar expansion is activated for this value, so that e.g. $HOME gets expanded.
- Parameters
-
pKey the key to search for aDefault a default value returned if the key was not found
- Returns
- the list, or
aDefault
if the key does not exist
Definition at line 799 of file kconfiggroup.cpp.
QStringList KConfigGroup::readPathEntry | ( | const char * | key, |
const QStringList & | aDefault | ||
) | const |
Overload for readPathEntry(const QString&, const QStringList&)
Definition at line 804 of file kconfiggroup.cpp.
QStringList KConfigGroup::readXdgListEntry | ( | const QString & | pKey, |
const QStringList & | aDefault = QStringList() |
||
) | const |
Reads a list of strings from the config object, following XDG desktop entry spec separator semantics.
- Parameters
-
pKey the key to search for aDefault the default value to use if the key does not exist
- Returns
- the list, or
aDefault
ifpKey
does not exist
Definition at line 741 of file kconfiggroup.cpp.
QStringList KConfigGroup::readXdgListEntry | ( | const char * | pKey, |
const QStringList & | aDefault = QStringList() |
||
) | const |
Overload for readXdgListEntry(const QString&, const QStringList&)
Definition at line 746 of file kconfiggroup.cpp.
void KConfigGroup::reparent | ( | KConfigBase * | parent, |
WriteConfigFlags | pFlags = Normal |
||
) |
Changes the configuration object that this group belongs to.
- Note
other
can be another group, the top-level KConfig object or a different KConfig object entirely.
If parent
is already the parent of this group, this method will have no effect.
- Parameters
-
parent the config object to place this group under pFlags the flags to use in determining which storage source to write the data to
- Since
- 4.1
Definition at line 1235 of file kconfiggroup.cpp.
void KConfigGroup::revertToDefault | ( | const QString & | key | ) |
Reverts an entry to the default settings.
Reverts the entry with key key
in the current group in the application specific config file to either the system wide (default) value or the value specified in the global KDE config file.
To revert entries in the global KDE config file, the global KDE config file should be opened explicitly in a separate config object.
- Note
- This is not the same as deleting the key, as instead the global setting will be copied to the configuration file that this object manipulates.
- Parameters
-
key The key of the entry to revert.
Definition at line 1125 of file kconfiggroup.cpp.
void KConfigGroup::revertToDefault | ( | const char * | key | ) |
Overload for revertToDefault(const QString&)
Definition at line 1117 of file kconfiggroup.cpp.
|
virtual |
- Reimplemented from superclass.
Syncs the parent config.
Implements KConfigBase.
Definition at line 595 of file kconfiggroup.cpp.
void KConfigGroup::writeEntry | ( | const QString & | key, |
const QVariant & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Writes a value to the configuration object.
- Parameters
-
key the key to write to value the value to write pFlags the flags to use when writing this entry
- See also
- readEntry(), writeXdgListEntry(), deleteEntry()
Definition at line 1037 of file kconfiggroup.cpp.
void KConfigGroup::writeEntry | ( | const char * | key, |
const QVariant & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 891 of file kconfiggroup.cpp.
void KConfigGroup::writeEntry | ( | const QString & | key, |
const QString & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 823 of file kconfiggroup.cpp.
void KConfigGroup::writeEntry | ( | const char * | key, |
const QString & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 815 of file kconfiggroup.cpp.
void KConfigGroup::writeEntry | ( | const QString & | key, |
const QByteArray & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 850 of file kconfiggroup.cpp.
void KConfigGroup::writeEntry | ( | const char * | key, |
const QByteArray & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 841 of file kconfiggroup.cpp.
void KConfigGroup::writeEntry | ( | const QString & | key, |
const char * | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 828 of file kconfiggroup.cpp.
void KConfigGroup::writeEntry | ( | const char * | key, |
const char * | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 836 of file kconfiggroup.cpp.
|
inline |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 425 of file kconfiggroup.h.
|
inline |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 429 of file kconfiggroup.h.
void KConfigGroup::writeEntry | ( | const QString & | key, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 869 of file kconfiggroup.cpp.
void KConfigGroup::writeEntry | ( | const char * | key, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 856 of file kconfiggroup.cpp.
void KConfigGroup::writeEntry | ( | const QString & | key, |
const QVariantList & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 1042 of file kconfiggroup.cpp.
void KConfigGroup::writeEntry | ( | const char * | key, |
const QVariantList & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 874 of file kconfiggroup.cpp.
|
inline |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 448 of file kconfiggroup.h.
|
inline |
Overload for writeEntry(const QString&, const QVariant&, WriteConfigFlags)
Definition at line 452 of file kconfiggroup.h.
void KConfigGroup::writePathEntry | ( | const QString & | pKey, |
const QString & | path, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Writes a file path to the configuration.
If the path is located under $HOME, the user's home directory is replaced with $HOME in the persistent storage. The path should therefore be read back with readPathEntry()
- Parameters
-
pKey the key to write to path the path to write pFlags the flags to use when writing this entry
- See also
- writeEntry(), readPathEntry()
Definition at line 1074 of file kconfiggroup.cpp.
void KConfigGroup::writePathEntry | ( | const char * | pKey, |
const QString & | path, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writePathEntry(const QString&, const QString&, WriteConfigFlags)
Definition at line 1079 of file kconfiggroup.cpp.
void KConfigGroup::writePathEntry | ( | const QString & | pKey, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Writes a list of paths to the configuration.
If any of the paths are located under $HOME, the user's home directory is replaced with $HOME in the persistent storage. The paths should therefore be read back with readPathEntry()
- Parameters
-
pKey the key to write to value the list to write pFlags the flags to use when writing this entry
- See also
- writeEntry(), readPathEntry()
Definition at line 1087 of file kconfiggroup.cpp.
void KConfigGroup::writePathEntry | ( | const char * | pKey, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writePathEntry(const QString&, const QStringList&, WriteConfigFlags)
Definition at line 1092 of file kconfiggroup.cpp.
void KConfigGroup::writeXdgListEntry | ( | const QString & | pKey, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Writes a list of strings to the config object, following XDG desktop entry spec separator semantics.
- Parameters
-
pKey the key to write to value the list to write pFlags the flags to use when writing this entry
- See also
- writeEntry(), readXdgListEntry()
Definition at line 1047 of file kconfiggroup.cpp.
void KConfigGroup::writeXdgListEntry | ( | const char * | pKey, |
const QStringList & | value, | ||
WriteConfigFlags | pFlags = Normal |
||
) |
Overload for writeXdgListEntry(const QString&, const QStringList&, WriteConfigFlags)
Definition at line 1052 of file kconfiggroup.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.