KDECore
#include <kdirwatch.h>
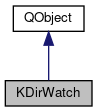
Public Types | |
enum | Method { FAM, INotify, DNotify, Stat, QFSWatch } |
enum | WatchMode { WatchDirOnly = 0, WatchFiles = 0x01, WatchSubDirs = 0x02 } |
Public Slots | |
void | setCreated (const QString &path) |
void | setDeleted (const QString &path) |
void | setDirty (const QString &path) |
Signals | |
void | created (const QString &path) |
void | deleted (const QString &path) |
void | dirty (const QString &path) |
Public Member Functions | |
KDirWatch (QObject *parent=0) | |
~KDirWatch () | |
void | addDir (const QString &path, WatchModes watchModes=WatchDirOnly) |
void | addFile (const QString &file) |
bool | contains (const QString &path) const |
QDateTime | ctime (const QString &path) const |
void | deleteQFSWatcher () |
Method | internalMethod () |
bool | isStopped () |
void | removeDir (const QString &path) |
void | removeFile (const QString &file) |
bool | restartDirScan (const QString &path) |
void | startScan (bool notify=false, bool skippedToo=false) |
bool | stopDirScan (const QString &path) |
void | stopScan () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static bool | exists () |
static KDirWatch * | self () |
static void | statistics () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Class for watching directory and file changes.
Watch directories and files for changes. The watched directories or files don't have to exist yet.
When a watched directory is changed, i.e. when files therein are created or deleted, KDirWatch will emit the signal dirty().
When a watched, but previously not existing directory gets created, KDirWatch will emit the signal created().
When a watched directory gets deleted, KDirWatch will emit the signal deleted(). The directory is still watched for new creation.
When a watched file is changed, i.e. attributes changed or written to, KDirWatch will emit the signal dirty().
Scanning of particular directories or files can be stopped temporarily and restarted. The whole class can be stopped and restarted. Directories and files can be added/removed from the list in any state.
The implementation uses the INOTIFY functionality on LINUX. Otherwise the FAM service is used, when available. As a last resort, a regular polling for change of modification times is done; the polling interval is a global config option: DirWatch/PollInterval and DirWatch/NFSPollInterval for NFS mounted directories. The choice of implementation can be adjusted by the user, with the key [DirWatch] PreferredMethod={Fam|Stat|QFSWatch|inotify}
- See also
- self()
Definition at line 64 of file kdirwatch.h.
Member Enumeration Documentation
enum KDirWatch::Method |
Enumerator | |
---|---|
FAM | |
INotify | |
DNotify | |
Stat | |
QFSWatch |
Definition at line 223 of file kdirwatch.h.
enum KDirWatch::WatchMode |
Available watch modes for directory monitoring.
Enumerator | |
---|---|
WatchDirOnly |
Watch just the specified directory. |
WatchFiles |
Watch also all files contained by the directory. |
WatchSubDirs |
Watch also all the subdirs contained by the directory. |
Definition at line 73 of file kdirwatch.h.
Constructor & Destructor Documentation
KDirWatch::KDirWatch | ( | QObject * | parent = 0 | ) |
Constructor.
Scanning begins immediately when a dir/file watch is added.
- Parameters
-
parent the parent of the QObject (or 0 for parent-less KDataTools)
Definition at line 1765 of file kdirwatch.cpp.
KDirWatch::~KDirWatch | ( | ) |
Member Function Documentation
void KDirWatch::addDir | ( | const QString & | path, |
WatchModes | watchModes = WatchDirOnly |
||
) |
Adds a directory to be watched.
The directory does not have to exist. When watchModes
is set to WatchDirOnly (the default), the signals dirty(), created(), deleted() can be emitted, all for the watched directory. When watchModes
is set to WatchFiles, all files in the watched directory are watched for changes, too. Thus, the signals dirty(), created(), deleted() can be emitted. When watchModes
is set to WatchSubDirs, all subdirs are watched using the same flags specified in watchModes
(symlinks aren't followed). If the path
points to a symlink to a directory, the target directory is watched instead. If you want to watch the link, use addFile()
.
- Parameters
-
path the path to watch watchModes watch modes
- See also
- KDirWatch::WatchMode
Definition at line 1796 of file kdirwatch.cpp.
void KDirWatch::addFile | ( | const QString & | file | ) |
Adds a file to be watched.
If it's a symlink to a directory, it watches the symlink itself.
- Parameters
-
file the file to watch
Definition at line 1801 of file kdirwatch.cpp.
Check if a directory is being watched by this KDirWatch instance.
- Parameters
-
path the directory to check
- Returns
- true if the directory is being watched
Definition at line 1879 of file kdirwatch.cpp.
|
signal |
Emitted when a file or directory is created.
- Parameters
-
path the path of the file or directory
Returns the time the directory/file was last changed.
- Parameters
-
path the file to check
- Returns
- the date of the last modification
Definition at line 1809 of file kdirwatch.cpp.
|
signal |
Emitted when a file or directory is deleted.
The object is still watched for new creation.
- Parameters
-
path the path of the file or directory
void KDirWatch::deleteQFSWatcher | ( | ) |
Definition at line 1893 of file kdirwatch.cpp.
|
signal |
Emitted when a watched object is changed.
For a directory this signal is emitted when files therein are created or deleted. For a file this signal is emitted when its size or attributes change.
When you watch a directory, changes in the size or attributes of contained files may or may not trigger this signal to be emitted depending on which backend is used by KDirWatch.
The new ctime is set before the signal is emitted.
- Parameters
-
path the path of the file or directory
|
static |
Returns true if there is an instance of KDirWatch.
- Returns
- true if there is an instance of KDirWatch.
- See also
- KDirWatch::self()
Definition at line 1755 of file kdirwatch.cpp.
KDirWatch::Method KDirWatch::internalMethod | ( | ) |
Returns the preferred internal method to watch for changes.
Definition at line 1927 of file kdirwatch.cpp.
bool KDirWatch::isStopped | ( | ) |
Is scanning stopped? After creation of a KDirWatch instance, this is false.
- Returns
- true when scanning stopped
Definition at line 1865 of file kdirwatch.cpp.
void KDirWatch::removeDir | ( | const QString & | path | ) |
Removes a directory from the list of scanned directories.
If specified path is not in the list this does nothing.
- Parameters
-
path the path of the dir to be removed from the list
Definition at line 1819 of file kdirwatch.cpp.
void KDirWatch::removeFile | ( | const QString & | file | ) |
Removes a file from the list of watched files.
If specified path is not in the list this does nothing.
- Parameters
-
file the file to be removed from the list
Definition at line 1828 of file kdirwatch.cpp.
Restarts scanning for specified path.
It doesn't notify about the changes (by emitting a signal). The ctime value is reset.
Call it when you are finished with big operations on that path, and when you have refreshed that path.
- Parameters
-
path the path to restart scanning
- Returns
- true if the
path
is being watched, otherwise false
- See also
- stopDirScanning()
Definition at line 1846 of file kdirwatch.cpp.
|
static |
The KDirWatch instance usually globally used in an application.
It is automatically deleted when the application exits.
However, you can create an arbitrary number of KDirWatch instances aside from this one - for those you have to take care of memory management.
This function returns an instance of KDirWatch. If there is none, it will be created.
- Returns
- a KDirWatch instance
Definition at line 1749 of file kdirwatch.cpp.
|
slot |
Emits created().
- Parameters
-
path the path of the file or directory
Definition at line 1909 of file kdirwatch.cpp.
|
slot |
Emits deleted().
- Parameters
-
path the path of the file or directory
Definition at line 1921 of file kdirwatch.cpp.
|
slot |
Emits dirty().
- Parameters
-
path the path of the file or directory
Definition at line 1915 of file kdirwatch.cpp.
Starts scanning of all dirs in list.
- Parameters
-
notify If true, all changed directories (since stopScan() call) will be notified for refresh. If notify is false, all ctimes will be reset (except those who are stopped, but only if skippedToo
is false) and changed dirs won't be notified. You can start scanning even if the list is empty. First call should be called withfalse
or else all directories in list will be notified.skippedToo if true, the skipped directoris (scanning of which was stopped with stopDirScan() ) will be reset and notified for change. Otherwise, stopped directories will continue to be unnotified.
Definition at line 1870 of file kdirwatch.cpp.
|
static |
Dump statistic information about the KDirWatch::self() instance.
This checks for consistency, too.
Definition at line 1899 of file kdirwatch.cpp.
Stops scanning the specified path.
The path
is not deleted from the internal list, it is just skipped. Call this function when you perform an huge operation on this directory (copy/move big files or many files). When finished, call restartDirScan(path).
- Parameters
-
path the path to skip
- Returns
- true if the
path
is being watched, otherwise false
- See also
- restartDirScanning()
Definition at line 1837 of file kdirwatch.cpp.
void KDirWatch::stopScan | ( | ) |
Stops scanning of all directories in internal list.
The timer is stopped, but the list is not cleared.
Definition at line 1857 of file kdirwatch.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.