KDECore
#include <kconfigdata.h>
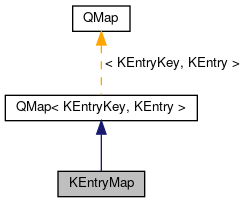
Public Types | |
enum | EntryOption { EntryDirty =1, EntryGlobal =2, EntryImmutable =4, EntryDeleted =8, EntryExpansion =16, EntryRawKey =32, EntryDefault =(SearchDefaults<<16), EntryLocalized =(SearchLocalized<<16) } |
enum | SearchFlag { SearchDefaults =1, SearchLocalized =2 } |
Public Member Functions | |
Iterator | findEntry (const QByteArray &group, const QByteArray &key=QByteArray(), SearchFlags flags=SearchFlags()) |
ConstIterator | findEntry (const QByteArray &group, const QByteArray &key=QByteArray(), SearchFlags flags=SearchFlags()) const |
Iterator | findExactEntry (const QByteArray &group, const QByteArray &key=QByteArray(), SearchFlags flags=SearchFlags()) |
QString | getEntry (const QByteArray &group, const QByteArray &key, const QString &defaultValue=QString(), SearchFlags flags=SearchFlags(), bool *expand=0) const |
bool | getEntryOption (const ConstIterator &it, EntryOption option) const |
bool | getEntryOption (const QByteArray &group, const QByteArray &key, SearchFlags flags, EntryOption option) const |
bool | hasEntry (const QByteArray &group, const QByteArray &key=QByteArray(), SearchFlags flags=SearchFlags()) const |
bool | revertEntry (const QByteArray &group, const QByteArray &key, SearchFlags flags=SearchFlags()) |
bool | setEntry (const QByteArray &group, const QByteArray &key, const QByteArray &value, EntryOptions options) |
void | setEntry (const QByteArray &group, const QByteArray &key, const QString &value, EntryOptions options) |
void | setEntryOption (Iterator it, EntryOption option, bool bf) |
void | setEntryOption (const QByteArray &group, const QByteArray &key, SearchFlags flags, EntryOption option, bool bf) |
![]() | |
QMap () | |
QMap (const QMap< Key, T > &other) | |
QMap (const std::map< Key, T > &other) | |
~QMap () | |
iterator | begin () |
const_iterator | begin () const |
void | clear () |
const_iterator | constBegin () const |
const_iterator | constEnd () const |
const_iterator | constFind (const Key &key) const |
bool | contains (const Key &key) const |
int | count () const |
int | count (const Key &key) const |
bool | empty () const |
iterator | end () |
const_iterator | end () const |
iterator | erase (iterator pos) |
void | erase (const Key &key) |
iterator | find (const Key &key) |
const_iterator | find (const Key &key) const |
iterator | insert (const Key &key, const T &value) |
iterator | insert (const Key &key, const T &value, bool overwrite) |
iterator | insertMulti (const Key &key, const T &value) |
bool | isEmpty () const |
const Key | key (const T &value) const |
const Key | key (const T &value, const Key &defaultKey) const |
QList< Key > | keys (const T &value) const |
QList< Key > | keys () const |
iterator | lowerBound (const Key &key) |
const_iterator | lowerBound (const Key &key) const |
bool | operator!= (const QMap< Key, T > &other) const |
QMap< Key, T > & | operator= (const QMap< Key, T > &other) |
bool | operator== (const QMap< Key, T > &other) const |
T & | operator[] (const Key &key) |
const T | operator[] (const Key &key) const |
int | remove (const Key &key) |
iterator | remove (iterator it) |
iterator | replace (const Key &key, const T &value) |
int | size () const |
void | swap (QMap< Key, T > &other) |
T | take (const Key &key) |
std::map< Key, T > | toStdMap () const |
QList< Key > | uniqueKeys () const |
QMap< Key, T > & | unite (const QMap< Key, T > &other) |
const_iterator | upperBound (const Key &key) const |
iterator | upperBound (const Key &key) |
const T | value (const Key &key, const T &defaultValue) const |
const T | value (const Key &key) const |
QList< T > | values () const |
QList< T > | values (const Key &key) const |
Additional Inherited Members | |
![]() | |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | key_type |
typedef | mapped_type |
typedef | size_type |
Detailed Description
type specifying a map of entries (key,value pairs). The keys are actually a key in a particular config file group together with the group name.
Definition at line 152 of file kconfigdata.h.
Member Enumeration Documentation
Enumerator | |
---|---|
EntryDirty | |
EntryGlobal | |
EntryImmutable | |
EntryDeleted | |
EntryExpansion | |
EntryRawKey | |
EntryDefault | |
EntryLocalized |
Definition at line 161 of file kconfigdata.h.
Enumerator | |
---|---|
SearchDefaults | |
SearchLocalized |
Definition at line 155 of file kconfigdata.h.
Member Function Documentation
Iterator KEntryMap::findEntry | ( | const QByteArray & | group, |
const QByteArray & | key = QByteArray() , |
||
SearchFlags | flags = SearchFlags() |
||
) |
ConstIterator KEntryMap::findEntry | ( | const QByteArray & | group, |
const QByteArray & | key = QByteArray() , |
||
SearchFlags | flags = SearchFlags() |
||
) | const |
QMap< KEntryKey, KEntry >::Iterator KEntryMap::findExactEntry | ( | const QByteArray & | group, |
const QByteArray & | key = QByteArray() , |
||
SearchFlags | flags = SearchFlags() |
||
) |
Definition at line 42 of file kconfigdata.cpp.
QString KEntryMap::getEntry | ( | const QByteArray & | group, |
const QByteArray & | key, | ||
const QString & | defaultValue = QString() , |
||
SearchFlags | flags = SearchFlags() , |
||
bool * | expand = 0 |
||
) | const |
Definition at line 209 of file kconfigdata.cpp.
bool KEntryMap::getEntryOption | ( | const ConstIterator & | it, |
EntryOption | option | ||
) | const |
|
inline |
Definition at line 203 of file kconfigdata.h.
bool KEntryMap::hasEntry | ( | const QByteArray & | group, |
const QByteArray & | key = QByteArray() , |
||
SearchFlags | flags = SearchFlags() |
||
) | const |
Definition at line 226 of file kconfigdata.cpp.
bool KEntryMap::revertEntry | ( | const QByteArray & | group, |
const QByteArray & | key, | ||
SearchFlags | flags = SearchFlags() |
||
) |
Definition at line 289 of file kconfigdata.cpp.
bool KEntryMap::setEntry | ( | const QByteArray & | group, |
const QByteArray & | key, | ||
const QByteArray & | value, | ||
EntryOptions | options | ||
) |
Returns true if the entry gets dirtied or false in other case.
|
inline |
Definition at line 188 of file kconfigdata.h.
void KEntryMap::setEntryOption | ( | Iterator | it, |
EntryOption | option, | ||
bool | bf | ||
) |
|
inline |
Definition at line 210 of file kconfigdata.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.