KDECore
#include <KProcess>
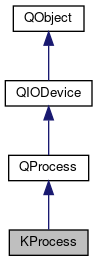
Public Types | |
enum | OutputChannelMode { SeparateChannels = QProcess::SeparateChannels, MergedChannels = QProcess::MergedChannels, ForwardedChannels = QProcess::ForwardedChannels, OnlyStdoutChannel, OnlyStderrChannel } |
Public Member Functions | |
KProcess (QObject *parent=0) | |
virtual | ~KProcess () |
void | clearEnvironment () |
void | clearProgram () |
int | execute (int msecs=-1) |
KProcess & | operator<< (const QString &arg) |
KProcess & | operator<< (const QStringList &args) |
OutputChannelMode | outputChannelMode () const |
int | pid () const |
QStringList | program () const |
void | setEnv (const QString &name, const QString &value, bool overwrite=true) |
void | setNextOpenMode (QIODevice::OpenMode mode) |
void | setOutputChannelMode (OutputChannelMode mode) |
void | setProgram (const QString &exe, const QStringList &args=QStringList()) |
void | setProgram (const QStringList &argv) |
void | setShellCommand (const QString &cmd) |
void | start () |
int | startDetached () |
void | unsetEnv (const QString &name) |
![]() | |
QProcess (QObject *parent) | |
virtual | ~QProcess () |
virtual bool | atEnd () const |
virtual qint64 | bytesAvailable () const |
virtual qint64 | bytesToWrite () const |
virtual bool | canReadLine () const |
virtual void | close () |
void | closeReadChannel (ProcessChannel channel) |
void | closeWriteChannel () |
QStringList | environment () const |
void | error (QProcess::ProcessError error) |
QProcess::ProcessError | error () const |
int | exitCode () const |
QProcess::ExitStatus | exitStatus () const |
void | finished (int exitCode, QProcess::ExitStatus exitStatus) |
void | finished (int exitCode) |
virtual bool | isSequential () const |
void | kill () |
QString | nativeArguments () const |
Q_PID | pid () const |
ProcessChannelMode | processChannelMode () const |
QProcessEnvironment | processEnvironment () const |
QByteArray | readAllStandardError () |
QByteArray | readAllStandardOutput () |
ProcessChannel | readChannel () const |
ProcessChannelMode | readChannelMode () const |
void | readyReadStandardError () |
void | readyReadStandardOutput () |
void | setEnvironment (const QStringList &environment) |
void | setNativeArguments (const QString &arguments) |
void | setProcessChannelMode (ProcessChannelMode mode) |
void | setProcessEnvironment (const QProcessEnvironment &environment) |
void | setReadChannel (ProcessChannel channel) |
void | setReadChannelMode (ProcessChannelMode mode) |
void | setStandardErrorFile (const QString &fileName, QFlags< QIODevice::OpenModeFlag > mode) |
void | setStandardInputFile (const QString &fileName) |
void | setStandardOutputFile (const QString &fileName, QFlags< QIODevice::OpenModeFlag > mode) |
void | setStandardOutputProcess (QProcess *destination) |
void | setWorkingDirectory (const QString &dir) |
void | start (const QString &program, const QStringList &arguments, QFlags< QIODevice::OpenModeFlag > mode) |
void | start (const QString &program, QFlags< QIODevice::OpenModeFlag > mode) |
void | started () |
QProcess::ProcessState | state () const |
void | stateChanged (QProcess::ProcessState newState) |
void | terminate () |
virtual bool | waitForBytesWritten (int msecs) |
bool | waitForFinished (int msecs) |
virtual bool | waitForReadyRead (int msecs) |
bool | waitForStarted (int msecs) |
QString | workingDirectory () const |
![]() | |
QIODevice () | |
QIODevice (QObject *parent) | |
virtual | ~QIODevice () |
void | aboutToClose () |
Offset | at () const |
bool | at (Offset offset) |
void | bytesWritten (qint64 bytes) |
QString | errorString () const |
int | flags () const |
int | getch () |
bool | getChar (char *c) |
bool | isAsynchronous () const |
bool | isBuffered () const |
bool | isCombinedAccess () const |
bool | isDirectAccess () const |
bool | isInactive () const |
bool | isOpen () const |
bool | isRaw () const |
bool | isReadable () const |
bool | isSequentialAccess () const |
bool | isSynchronous () const |
bool | isTextModeEnabled () const |
bool | isTranslated () const |
bool | isWritable () const |
int | mode () const |
virtual bool | open (QFlags< QIODevice::OpenModeFlag > mode) |
OpenMode | openMode () const |
qint64 | peek (char *data, qint64 maxSize) |
QByteArray | peek (qint64 maxSize) |
virtual qint64 | pos () const |
int | putch (int ch) |
bool | putChar (char c) |
qint64 | read (char *data, qint64 maxSize) |
QByteArray | read (qint64 maxSize) |
QByteArray | readAll () |
qint64 | readBlock (char *data, quint64 size) |
void | readChannelFinished () |
QByteArray | readLine (qint64 maxSize) |
qint64 | readLine (char *data, qint64 maxSize) |
void | readyRead () |
virtual bool | reset () |
void | resetStatus () |
virtual bool | seek (qint64 pos) |
void | setTextModeEnabled (bool enabled) |
virtual qint64 | size () const |
int | state () const |
Status | status () const |
int | ungetch (int ch) |
void | ungetChar (char c) |
qint64 | write (const char *data) |
qint64 | write (const char *data, qint64 maxSize) |
qint64 | write (const QByteArray &byteArray) |
qint64 | writeBlock (const QByteArray &data) |
qint64 | writeBlock (const char *data, quint64 size) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static int | execute (const QString &exe, const QStringList &args=QStringList(), int msecs=-1) |
static int | execute (const QStringList &argv, int msecs=-1) |
static int | startDetached (const QString &exe, const QStringList &args=QStringList()) |
static int | startDetached (const QStringList &argv) |
![]() | |
int | execute (const QString &program, const QStringList &arguments) |
int | execute (const QString &program) |
bool | startDetached (const QString &program) |
bool | startDetached (const QString &program, const QStringList &arguments) |
bool | startDetached (const QString &program, const QStringList &arguments, const QString &workingDirectory, qint64 *pid) |
QStringList | systemEnvironment () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
KProcess (KProcessPrivate *d, QObject *parent) | |
![]() | |
virtual qint64 | readData (char *data, qint64 maxlen) |
void | setProcessState (ProcessState state) |
virtual void | setupChildProcess () |
virtual qint64 | writeData (const char *data, qint64 len) |
![]() | |
virtual qint64 | readData (char *data, qint64 maxSize)=0 |
virtual qint64 | readLineData (char *data, qint64 maxSize) |
void | setErrorString (const QString &str) |
void | setOpenMode (QFlags< QIODevice::OpenModeFlag > openMode) |
virtual qint64 | writeData (const char *data, qint64 maxSize)=0 |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
KProcessPrivate *const | d_ptr |
Additional Inherited Members | |
![]() | |
typedef | Offset |
typedef | OpenMode |
typedef | Status |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Child process invocation, monitoring and control.
This class extends QProcess by some useful functionality, overrides some defaults with saner values and wraps parts of the API into a more accessible one. This is the preferred way of spawning child processes in KDE; don't use QProcess directly.
Definition at line 44 of file kprocess.h.
Member Enumeration Documentation
Modes in which the output channels can be opened.
Enumerator | |
---|---|
SeparateChannels |
Standard output and standard error are handled by KProcess as separate channels. |
MergedChannels |
Standard output and standard error are handled by KProcess as one channel. |
ForwardedChannels |
Both standard output and standard error are forwarded to the parent process' respective channel. |
OnlyStdoutChannel |
Only standard output is handled; standard error is forwarded. |
OnlyStderrChannel |
Only standard error is handled; standard output is forwarded. |
Definition at line 54 of file kprocess.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
Definition at line 106 of file kprocess.cpp.
|
virtual |
Destructor.
Definition at line 122 of file kprocess.cpp.
|
protected |
Definition at line 114 of file kprocess.cpp.
Member Function Documentation
void KProcess::clearEnvironment | ( | ) |
Empties the process' environment.
Note that LD_LIBRARY_PATH/DYLD_LIBRARY_PATH is automatically added on *NIX.
This function must be called before starting the process.
Definition at line 164 of file kprocess.cpp.
void KProcess::clearProgram | ( | ) |
Clear the program and command line argument list.
Definition at line 254 of file kprocess.cpp.
int KProcess::execute | ( | int | msecs = -1 | ) |
Start the process, wait for it to finish, and return the exit code.
This method is roughly equivalent to the sequence: start(); waitForFinished(msecs); return exitCode();
Unlike the other execute() variants this method is not static, so the process can be parametrized properly and talked to.
- Parameters
-
msecs time to wait for process to exit before killing it
- Returns
- -2 if the process could not be started, -1 if it crashed, otherwise its exit code
Definition at line 347 of file kprocess.cpp.
|
static |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
exe the program to execute args the command line arguments for the program, one per list element msecs time to wait for process to exit before killing it
- Returns
- -2 if the process could not be started, -1 if it crashed, otherwise its exit code
Definition at line 359 of file kprocess.cpp.
|
static |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
argv the program to execute and the command line arguments for the program, one per list element msecs time to wait for process to exit before killing it
- Returns
- -2 if the process could not be started, -1 if it crashed, otherwise its exit code
Definition at line 367 of file kprocess.cpp.
Append an element to the command line argument list for this process.
If no executable is set yet, it will be set instead.
For example, doing an "ls -l /usr/local/bin" can be achieved by:
This function must be called before starting the process, obviously.
- Parameters
-
arg the argument to add
- Returns
- a reference to this KProcess
Definition at line 232 of file kprocess.cpp.
KProcess & KProcess::operator<< | ( | const QStringList & | args | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
args the arguments to add
- Returns
- a reference to this KProcess
Definition at line 243 of file kprocess.cpp.
KProcess::OutputChannelMode KProcess::outputChannelMode | ( | ) | const |
Query how the output channels of the child process are handled.
- Returns
- the output channel handling mode
Definition at line 148 of file kprocess.cpp.
int KProcess::pid | ( | ) | const |
Obtain the process' ID as known to the system.
Unlike with QProcess::pid(), this is a real PID also on Windows.
This function can be called only while the process is running. It cannot be applied to detached processes.
- Returns
- the process ID
Definition at line 401 of file kprocess.cpp.
QStringList KProcess::program | ( | ) | const |
Obtain the currently set program and arguments.
- Returns
- a list, the first element being the program, the remaining ones being command line arguments to the program.
Definition at line 331 of file kprocess.cpp.
Adds the variable name
to the process' environment.
This function must be called before starting the process.
- Parameters
-
name the name of the environment variable value the new value for the environment variable overwrite if false
and the environment variable is already set, the old value will be preserved
Definition at line 169 of file kprocess.cpp.
void KProcess::setNextOpenMode | ( | QIODevice::OpenMode | mode | ) |
Set the QIODevice open mode the process will be opened in.
This function must be called before starting the process, obviously.
- Parameters
-
mode the open mode. Note that this mode is automatically "reduced" according to the channel modes and redirections. The default is QIODevice::ReadWrite.
Definition at line 155 of file kprocess.cpp.
void KProcess::setOutputChannelMode | ( | OutputChannelMode | mode | ) |
Set how to handle the output channels of the child process.
The default is ForwardedChannels, which is unlike in QProcess. Do not request more than you actually handle, as this output is simply lost otherwise.
This function must be called before starting the process.
- Parameters
-
mode the output channel handling mode
Definition at line 127 of file kprocess.cpp.
void KProcess::setProgram | ( | const QString & | exe, |
const QStringList & | args = QStringList() |
||
) |
Set the program and the command line arguments.
This function must be called before starting the process, obviously.
- Parameters
-
exe the program to execute args the command line arguments for the program, one per list element
Definition at line 209 of file kprocess.cpp.
void KProcess::setProgram | ( | const QStringList & | argv | ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
argv the program to execute and the command line arguments for the program, one per list element
Definition at line 220 of file kprocess.cpp.
void KProcess::setShellCommand | ( | const QString & | cmd | ) |
Set a command to execute through a shell (a POSIX sh on *NIX and cmd.exe on Windows).
Using this for anything but user-supplied commands is usually a bad idea, as the command's syntax depends on the platform. Redirections including pipes, etc. are better handled by the respective functions provided by QProcess.
If KProcess determines that the command does not really need a shell, it will trasparently execute it without one for performance reasons.
This function must be called before starting the process, obviously.
- Parameters
-
cmd the command to execute through a shell. The caller must make sure that all filenames etc. are properly quoted when passed as argument. Failure to do so often results in serious security holes. See KShell::quoteArg().
Definition at line 265 of file kprocess.cpp.
void KProcess::start | ( | ) |
Start the process.
- See also
- QProcess::start(const QString &, const QStringList &, OpenMode)
Definition at line 340 of file kprocess.cpp.
int KProcess::startDetached | ( | ) |
Start the process and detach from it.
See QProcess::startDetached() for details.
Unlike the other startDetached() variants this method is not static, so the process can be parametrized properly.
- Note
- Currently, only the setProgram()/setShellCommand() and setWorkingDirectory() parametrizations are supported.
The KProcess object may be re-used immediately after calling this function.
- Returns
- the PID of the started process or 0 on error
Definition at line 374 of file kprocess.cpp.
|
static |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
exe the program to start args the command line arguments for the program, one per list element
- Returns
- the PID of the started process or 0 on error
Definition at line 385 of file kprocess.cpp.
|
static |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
argv the program to start and the command line arguments for the program, one per list element
- Returns
- the PID of the started process or 0 on error
Definition at line 394 of file kprocess.cpp.
void KProcess::unsetEnv | ( | const QString & | name | ) |
Removes the variable name
from the process' environment.
This function must be called before starting the process.
- Parameters
-
name the name of the environment variable
Definition at line 190 of file kprocess.cpp.
Member Data Documentation
|
protected |
Definition at line 327 of file kprocess.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.