KDECore
#include <KSaveFile>
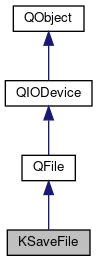
Public Member Functions | |
KSaveFile () | |
KSaveFile (const QString &filename, const KComponentData &componentData=KGlobal::mainComponent()) | |
virtual | ~KSaveFile () |
void | abort () |
bool | directWriteFallback () const |
QFile::FileError | error () const |
QString | errorString () const |
QString | fileName () const |
bool | finalize () |
virtual bool | open (OpenMode flags=QIODevice::ReadWrite) |
void | setDirectWriteFallback (bool enabled) |
void | setFileName (const QString &filename) |
![]() | |
QFile (const QString &name) | |
QFile (QObject *parent) | |
QFile (const QString &name, QObject *parent) | |
~QFile () | |
virtual bool | atEnd () const |
virtual void | close () |
bool | copy (const QString &newName) |
FileError | error () const |
bool | exists () const |
QString | fileName () const |
bool | flush () |
int | handle () const |
virtual bool | isSequential () const |
bool | link (const QString &linkName) |
uchar * | map (qint64 offset, qint64 size, MemoryMapFlags flags) |
QString | name () const |
bool | open (QFlags< QIODevice::OpenModeFlag > aFlags, int fd) |
bool | open (FILE *fh, QFlags< QIODevice::OpenModeFlag > mode) |
bool | open (int fd, QFlags< QIODevice::OpenModeFlag > mode) |
bool | open (const RFile &f, QFlags< QIODevice::OpenModeFlag > mode, QFlags< QFile::FileHandleFlag > handleFlags) |
bool | open (FILE *fh, QFlags< QIODevice::OpenModeFlag > mode, QFlags< QFile::FileHandleFlag > handleFlags) |
bool | open (int fd, QFlags< QIODevice::OpenModeFlag > mode, QFlags< QFile::FileHandleFlag > handleFlags) |
bool | open (QFlags< QIODevice::OpenModeFlag > aFlags, FILE *f) |
virtual bool | open (QFlags< QIODevice::OpenModeFlag > mode) |
Permissions | permissions () const |
virtual qint64 | pos () const |
QString | readLink () const |
bool | remove () |
bool | rename (const QString &newName) |
bool | resize (qint64 sz) |
virtual bool | seek (qint64 pos) |
void | setFileName (const QString &name) |
void | setName (const QString &name) |
bool | setPermissions (QFlags< QFile::Permission > permissions) |
virtual qint64 | size () const |
QString | symLinkTarget () const |
bool | unmap (uchar *address) |
void | unsetError () |
![]() | |
QIODevice () | |
QIODevice (QObject *parent) | |
virtual | ~QIODevice () |
void | aboutToClose () |
Offset | at () const |
bool | at (Offset offset) |
virtual qint64 | bytesAvailable () const |
virtual qint64 | bytesToWrite () const |
void | bytesWritten (qint64 bytes) |
virtual bool | canReadLine () const |
QString | errorString () const |
int | flags () const |
int | getch () |
bool | getChar (char *c) |
bool | isAsynchronous () const |
bool | isBuffered () const |
bool | isCombinedAccess () const |
bool | isDirectAccess () const |
bool | isInactive () const |
bool | isOpen () const |
bool | isRaw () const |
bool | isReadable () const |
bool | isSequentialAccess () const |
bool | isSynchronous () const |
bool | isTextModeEnabled () const |
bool | isTranslated () const |
bool | isWritable () const |
int | mode () const |
OpenMode | openMode () const |
qint64 | peek (char *data, qint64 maxSize) |
QByteArray | peek (qint64 maxSize) |
int | putch (int ch) |
bool | putChar (char c) |
qint64 | read (char *data, qint64 maxSize) |
QByteArray | read (qint64 maxSize) |
QByteArray | readAll () |
qint64 | readBlock (char *data, quint64 size) |
void | readChannelFinished () |
QByteArray | readLine (qint64 maxSize) |
qint64 | readLine (char *data, qint64 maxSize) |
void | readyRead () |
virtual bool | reset () |
void | resetStatus () |
void | setTextModeEnabled (bool enabled) |
int | state () const |
Status | status () const |
int | ungetch (int ch) |
void | ungetChar (char c) |
virtual bool | waitForBytesWritten (int msecs) |
virtual bool | waitForReadyRead (int msecs) |
qint64 | write (const char *data) |
qint64 | write (const char *data, qint64 maxSize) |
qint64 | write (const QByteArray &byteArray) |
qint64 | writeBlock (const QByteArray &data) |
qint64 | writeBlock (const char *data, quint64 size) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static bool | backupFile (const QString &filename, const QString &backupDir=QString()) |
static bool | numberedBackupFile (const QString &filename, const QString &backupDir=QString(), const QString &backupExtension=QString::fromLatin1("~"), const uint maxBackups=10) |
static bool | rcsBackupFile (const QString &filename, const QString &backupDir=QString(), const QString &backupMessage=QString()) |
static bool | simpleBackupFile (const QString &filename, const QString &backupDir=QString(), const QString &backupExtension=QLatin1String("~")) |
![]() | |
bool | copy (const QString &fileName, const QString &newName) |
QString | decodeName (const QByteArray &localFileName) |
QString | decodeName (const char *localFileName) |
QByteArray | encodeName (const QString &fileName) |
bool | exists (const QString &fileName) |
bool | link (const QString &fileName, const QString &linkName) |
Permissions | permissions (const QString &fileName) |
QString | readLink (const QString &fileName) |
bool | remove (const QString &fileName) |
bool | rename (const QString &oldName, const QString &newName) |
bool | resize (const QString &fileName, qint64 sz) |
void | setDecodingFunction (DecoderFn function) |
void | setEncodingFunction (EncoderFn function) |
bool | setPermissions (const QString &fileName, QFlags< QFile::Permission > permissions) |
QString | symLinkTarget (const QString &fileName) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | DecoderFn |
typedef | EncoderFn |
typedef | FileHandleFlags |
typedef | Permissions |
typedef | PermissionSpec |
![]() | |
typedef | Offset |
typedef | OpenMode |
typedef | Status |
![]() | |
virtual qint64 | readData (char *data, qint64 len) |
virtual qint64 | readLineData (char *data, qint64 maxlen) |
virtual qint64 | writeData (const char *data, qint64 len) |
![]() | |
virtual qint64 | readData (char *data, qint64 maxSize)=0 |
void | setErrorString (const QString &str) |
void | setOpenMode (QFlags< QIODevice::OpenModeFlag > openMode) |
virtual qint64 | writeData (const char *data, qint64 maxSize)=0 |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Class to allow for atomic file I/O, as well as utility functions.
The KSaveFile class has been made to write out changes to an existing file atomically. This means that either ALL changes will be written to the file, or NO changes have been written, and the original file (if any) has been unchanged. This is useful if you have lots of time-consuming processing to perform during which an interruption could occur, or if any error in the file structure will cause the entire file to be corrupt.
When you create a KSaveFile for a given file, a temporary file is instead created and all your I/O occurs in the save file. Once you call finalize() the temporary file is renamed to the target file, so that all your changes happen at once. If abort() is called then the temporary file is removed and the target file is untouched. KSaveFile derives from QFile so you can use it just as you would a normal QFile.
This class also includes several static utility functions available that can help ensure data integrity. See the individual functions for details.
Here is a quick example of how to use KSaveFile:
First we create the KSaveFile and open it.
At this point the file "/lib/foo/bar.dat" has not been altered in any way. Now, let's write out some data to the file.
Even after writing this data, the target file "/lib/foo/bar.dat" still has not been altered in any way. Now that we are done writing our data, we can write out all the changes that we have made by calling finalize().
If a user interruption or error occurred while we were writing out our changes, we would instead call abort() to cancel all the I/O without affecting the target file.
- See also
- QFile
Definition at line 96 of file ksavefile.h.
Constructor & Destructor Documentation
KSaveFile::KSaveFile | ( | ) |
Default constructor.
Definition at line 62 of file ksavefile.cpp.
|
explicit |
Creates a new KSaveFile and sets the target file to filename
.
- Parameters
-
filename the path of the file componentData unused
Definition at line 68 of file ksavefile.cpp.
|
virtual |
Destructor.
- Note
- If the file has been opened but not yet finalized, the destructor will call finalize(). If you do not want the target file to be affected you need to call abort() before destroying the object.
Definition at line 74 of file ksavefile.cpp.
Member Function Documentation
void KSaveFile::abort | ( | ) |
Discard changes without affecting the target file.
This will discard all changes that have been made to this file. The target file will not be altered in any way.
Definition at line 204 of file ksavefile.cpp.
|
static |
Static method to create a backup file before saving.
If empty (the default), the backup will be in the same directory as filename
. The backup type (simple, rcs, or numbered), extension string, and maximum number of backup files are read from the user's global configuration. Use simpleBackupFile() or numberedBackupFile() to force one of these specific backup styles. You can use this method even if you don't use KSaveFile.
- Parameters
-
filename the file to backup backupDir optional directory where to save the backup file in.
- Returns
- true if successful, or false if an error has occurred.
Definition at line 285 of file ksavefile.cpp.
bool KSaveFile::directWriteFallback | ( | ) | const |
Returns true if the fallback solution for saving files in read-only directories is enabled.
- Since
- 4.10.3
Definition at line 280 of file ksavefile.cpp.
QFile::FileError KSaveFile::error | ( | ) | const |
Returns the last error that occurred.
Use this function to check for errors.
- Returns
- The last error that occurred, or QFile::NoError.
Definition at line 181 of file ksavefile.cpp.
QString KSaveFile::errorString | ( | ) | const |
Returns a human-readable description of the last error.
Use this function to get a human-readable description of the last error that occurred.
- Returns
- A string describing the last error that occurred.
Definition at line 190 of file ksavefile.cpp.
QString KSaveFile::fileName | ( | ) | const |
Returns the name of the target file.
This function returns the name of the target file, or an empty QString if it has not yet been set.
- Returns
- The name of the target file.
Definition at line 199 of file ksavefile.cpp.
bool KSaveFile::finalize | ( | ) |
Finalize changes to the file.
This will commit all the changes that have been made to the file.
- Returns
- true if successful, or false if an error has occurred.
Definition at line 219 of file ksavefile.cpp.
|
static |
Static method to create a backup file for a given filename.
This function creates a series of numbered backup files from the given filename.
The backup file names will be of the form: <name>.<number><extension> for instance
chat.3.log
The new backup file will be have the backup number 1. Each existing backup file will have its number incremented by 1. Any backup files with numbers greater than the maximum number permitted (maxBackups
) will be removed. You can use this method even if you don't use KSaveFile.
- Parameters
-
filename the file to backup backupDir optional directory where to save the backup file in. If empty (the default), the backup will be in the same directory as filename
.backupExtension the extension to append to filename
, which is "~" by default. Do not use an extension containing digits.maxBackups the maximum number of backup files permitted. For best performance a small number (10) is recommended.
- Returns
- true if successful, or false if an error has occurred.
Definition at line 388 of file ksavefile.cpp.
Open the save file.
This function will open the save file by creating a temporary file to write to. It will also check to ensure that there are sufficient permissions to write to the target file.
- Parameters
-
flags Sets the QIODevice::OpenMode. It should contain the write flag, otherwise you have a save file you cannot save to.
- Returns
- true if successful, or false if an error has occurred.
Definition at line 81 of file ksavefile.cpp.
|
static |
Static method to create an rcs backup file for a given filename.
This function creates a rcs-formatted backup file from the given filename.
The backup file names will be of the form: <name>,v for instance
photo.jpg,v
The new backup file will be in RCS format. Each existing backup file will be committed as a new revision. You can use this method even if you don't use KSaveFile.
- Parameters
-
filename the file to backup backupDir optional directory where to save the backup file in. If empty (the default), the backup will be in the same directory as filename
.backupMessage is the RCS commit message for this revision.
- Returns
- true if successful, or false if an error has occurred.
Definition at line 321 of file ksavefile.cpp.
void KSaveFile::setDirectWriteFallback | ( | bool | enabled | ) |
Allows writing over the existing file if necessary.
QSaveFile creates a temporary file in the same directory as the final file and atomically renames it. However this is not possible if the directory permissions do not allow creating new files. In order to preserve atomicity guarantees, open() fails when it cannot create the temporary file.
In order to allow users to edit files with write permissions in a directory with restricted permissions, call setDirectWriteFallback() with enabled set to true, and the following calls to open() will fallback to opening the existing file directly and writing into it, without the use of a temporary file. This does not have atomicity guarantees, i.e. an application crash or for instance a power failure could lead to a partially-written file on disk. It also means cancelWriting() has no effect, in such a case.
Typically, to save documents edited by the user, call setDirectWriteFallback(true), and to save application internal files (configuration files, data files, ...), keep the default setting which ensures atomicity.
- Since
- 4.10.3
Definition at line 275 of file ksavefile.cpp.
void KSaveFile::setFileName | ( | const QString & | filename | ) |
Set the target filename for the save file.
You must use this to set the filename of the target file if you do not use the contructor that does so.
- Parameters
-
filename Name of the target file.
Definition at line 166 of file ksavefile.cpp.
|
static |
Static method to create a backup file for a given filename.
This function creates a backup file from the given filename. You can use this method even if you don't use KSaveFile.
- Parameters
-
filename the file to backup backupDir optional directory where to save the backup file in. If empty (the default), the backup will be in the same directory as filename
.backupExtension the extension to append to filename
, "~" by default.
- Returns
- true if successful, or false if an error has occurred.
Definition at line 305 of file ksavefile.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.