KDECore
#include <KSharedPtr>
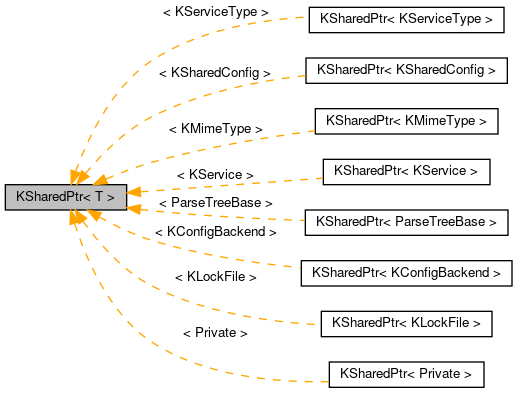
Public Member Functions | |
KSharedPtr () | |
KSharedPtr (T *p) | |
KSharedPtr (const KSharedPtr &o) | |
~KSharedPtr () | |
void | attach (T *p) |
void | clear () |
const T * | constData () const |
int | count () const |
T * | data () |
const T * | data () const |
bool | isNull () const |
bool | isUnique () const |
operator bool () const | |
bool | operator!= (const KSharedPtr &o) const |
bool | operator!= (const T *p) const |
const T & | operator* () const |
T & | operator* () |
const T * | operator-> () const |
T * | operator-> () |
bool | operator< (const KSharedPtr &o) const |
KSharedPtr< T > & | operator= (const KSharedPtr &o) |
KSharedPtr< T > & | operator= (T *p) |
bool | operator== (const KSharedPtr &o) const |
bool | operator== (const T *p) const |
Static Public Member Functions | |
template<class U > | |
static KSharedPtr< T > | dynamicCast (const KSharedPtr< U > &o) |
template<class U > | |
static KSharedPtr< T > | staticCast (const KSharedPtr< U > &o) |
Protected Attributes | |
T * | d |
Detailed Description
template<typename T>
class KSharedPtr< T >
Can be used to control the lifetime of an object that has derived QSharedData.
As long a someone holds a KSharedPtr on some QSharedData object it won't become deleted but is deleted once its reference count is 0. This struct emulates C++ pointers virtually perfectly. So just use it like a simple C++ pointer.
The difference with QSharedPointer is that QSharedPointer does the refcounting in the pointer, while KSharedPtr does the refcounting in the object. This allows to convert to a raw pointer temporarily and back to a KSharedPtr without deleting the object, if another reference exists. But it imposes a requirement on the object, which must inherit QSharedData.
The difference with using QSharedDataPointer is that QSharedDataPointer is a building block for implementing a value class with implicit sharing (like QString), whereas KSharedPtr provides refcounting to code that uses pointers.
Definition at line 38 of file kconfiggroup.h.
Constructor & Destructor Documentation
|
inline |
Creates a null pointer.
Definition at line 69 of file ksharedptr.h.
|
inlineexplicit |
|
inline |
|
inline |
Unreferences the object that this pointer points to.
If it was the last reference, the object will be deleted.
Definition at line 90 of file ksharedptr.h.
Member Function Documentation
Q_INLINE_TEMPLATE void KSharedPtr< T >::attach | ( | T * | p | ) |
Attach the given pointer to the current KSharedPtr.
If the previous shared pointer is not owned by any KSharedPtr, it is deleted.
Definition at line 209 of file ksharedptr.h.
Q_INLINE_TEMPLATE void KSharedPtr< T >::clear | ( | ) |
|
inline |
- Returns
- a const pointer to the shared object.
Definition at line 121 of file ksharedptr.h.
|
inline |
Returns the number of references.
- Returns
- the number of references
Definition at line 144 of file ksharedptr.h.
|
inline |
- Returns
- the pointer
Definition at line 111 of file ksharedptr.h.
|
inline |
- Returns
- the pointer
Definition at line 116 of file ksharedptr.h.
|
inlinestatic |
Convert KSharedPtr<U> to KSharedPtr<T>, using a dynamic_cast.
This will compile whenever T* and U* are compatible, i.e. T is a subclass of U or vice-versa. Example syntax: KSharedPtr<T> tPtr; KSharedPtr<U> uPtr = KSharedPtr<U>::dynamicCast( tPtr );
Since a dynamic_cast is used, if U derives from T, and tPtr isn't an instance of U, uPtr will be 0.
Definition at line 188 of file ksharedptr.h.
|
inline |
Test if the shared pointer is null.
- Returns
- true if the pointer is null, false otherwise.
- See also
- opertor (bool)
Definition at line 151 of file ksharedptr.h.
|
inline |
- Returns
- Whether this is the only shared pointer pointing to to the pointee, or whether it's shared among multiple shared pointers.
Definition at line 158 of file ksharedptr.h.
|
inline |
Test if the shared pointer is NOT null.
- Returns
- true if the shared pointer is NOT null, false otherwise.
- See also
- isNull
Definition at line 106 of file ksharedptr.h.
|
inline |
Definition at line 94 of file ksharedptr.h.
|
inline |
Definition at line 99 of file ksharedptr.h.
|
inline |
Definition at line 123 of file ksharedptr.h.
|
inline |
Definition at line 124 of file ksharedptr.h.
|
inline |
Definition at line 125 of file ksharedptr.h.
|
inline |
Definition at line 126 of file ksharedptr.h.
|
inline |
Definition at line 95 of file ksharedptr.h.
|
inline |
Definition at line 92 of file ksharedptr.h.
|
inline |
Definition at line 97 of file ksharedptr.h.
|
inline |
Definition at line 93 of file ksharedptr.h.
|
inline |
Definition at line 98 of file ksharedptr.h.
|
inlinestatic |
Convert KSharedPtr<U> to KSharedPtr<T>, using a static_cast.
This will compile whenever T* and U* are compatible, i.e. T is a subclass of U or vice-versa. Example syntax: KSharedPtr<T> tPtr; KSharedPtr<U> uPtr = KSharedPtr<U>::staticCast( tPtr );
Definition at line 173 of file ksharedptr.h.
Member Data Documentation
|
protected |
Definition at line 193 of file ksharedptr.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.