KDECore
#include <KSortableList>
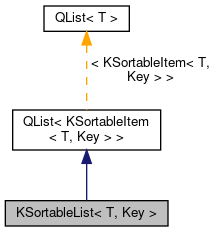
Public Member Functions | |
void | insert (Key i, const T &t) |
T & | operator[] (Key i) |
const T & | operator[] (Key i) const |
void | sort () |
![]() | |
QList () | |
QList (const QList< KSortableItem< T, Key > > &other) | |
QList (std::initializer_list< KSortableItem< T, Key > > args) | |
~QList () | |
void | append (const KSortableItem< T, Key > &value) |
void | append (const QList< KSortableItem< T, Key > > &value) |
const KSortableItem< T, Key > & | at (int i) const |
KSortableItem< T, Key > & | back () |
const KSortableItem< T, Key > & | back () const |
iterator | begin () |
const_iterator | begin () const |
void | clear () |
const_iterator | constBegin () const |
const_iterator | constEnd () const |
bool | contains (const KSortableItem< T, Key > &value) const |
int | count (const KSortableItem< T, Key > &value) const |
int | count () const |
bool | empty () const |
iterator | end () |
const_iterator | end () const |
bool | endsWith (const KSortableItem< T, Key > &value) const |
iterator | erase (iterator pos) |
iterator | erase (iterator begin, iterator end) |
iterator | find (iterator from, const KSortableItem< T, Key > &t) |
iterator | find (const KSortableItem< T, Key > &t) |
const_iterator | find (const KSortableItem< T, Key > &t) const |
const_iterator | find (const_iterator from, const KSortableItem< T, Key > &t) const |
int | findIndex (const KSortableItem< T, Key > &t) const |
KSortableItem< T, Key > & | first () |
const KSortableItem< T, Key > & | first () const |
KSortableItem< T, Key > & | front () |
const KSortableItem< T, Key > & | front () const |
int | indexOf (const KSortableItem< T, Key > &value, int from) const |
void | insert (int i, const KSortableItem< T, Key > &value) |
iterator | insert (iterator before, const KSortableItem< T, Key > &value) |
bool | isEmpty () const |
KSortableItem< T, Key > & | last () |
const KSortableItem< T, Key > & | last () const |
int | lastIndexOf (const KSortableItem< T, Key > &value, int from) const |
int | length () const |
QList< KSortableItem< T, Key > > | mid (int pos, int length) const |
void | move (int from, int to) |
bool | operator!= (const QList< KSortableItem< T, Key > > &other) const |
QList< KSortableItem< T, Key > > | operator+ (const QList< KSortableItem< T, Key > > &other) const |
QList< KSortableItem< T, Key > > & | operator+= (const QList< KSortableItem< T, Key > > &other) |
QList< KSortableItem< T, Key > > & | operator+= (const KSortableItem< T, Key > &value) |
QList< KSortableItem< T, Key > > & | operator<< (const KSortableItem< T, Key > &value) |
QList< KSortableItem< T, Key > > & | operator<< (const QList< KSortableItem< T, Key > > &other) |
QList< KSortableItem< T, Key > > & | operator= (const QList< KSortableItem< T, Key > > &other) |
bool | operator== (const QList< KSortableItem< T, Key > > &other) const |
KSortableItem< T, Key > & | operator[] (int i) |
const KSortableItem< T, Key > & | operator[] (int i) const |
void | pop_back () |
void | pop_front () |
void | prepend (const KSortableItem< T, Key > &value) |
void | push_back (const KSortableItem< T, Key > &value) |
void | push_front (const KSortableItem< T, Key > &value) |
iterator | remove (iterator pos) |
int | remove (const KSortableItem< T, Key > &t) |
int | removeAll (const KSortableItem< T, Key > &value) |
void | removeAt (int i) |
void | removeFirst () |
void | removeLast () |
bool | removeOne (const KSortableItem< T, Key > &value) |
void | replace (int i, const KSortableItem< T, Key > &value) |
void | reserve (int alloc) |
int | size () const |
bool | startsWith (const KSortableItem< T, Key > &value) const |
void | swap (int i, int j) |
void | swap (QList< KSortableItem< T, Key > > &other) |
KSortableItem< T, Key > | takeAt (int i) |
KSortableItem< T, Key > | takeFirst () |
KSortableItem< T, Key > | takeLast () |
QSet< KSortableItem< T, Key > > | toSet () const |
std::list< KSortableItem< T, Key > > | toStdList () const |
QVector< KSortableItem< T, Key > > | toVector () const |
KSortableItem< T, Key > | value (int i, const KSortableItem< T, Key > &defaultValue) const |
KSortableItem< T, Key > | value (int i) const |
Additional Inherited Members | |
![]() | |
QList< KSortableItem< T, Key > > | fromSet (const QSet< KSortableItem< T, Key > > &set) |
QList< KSortableItem< T, Key > > | fromStdList (const std::list< KSortableItem< T, Key > > &list) |
QList< KSortableItem< T, Key > > | fromVector (const QVector< KSortableItem< T, Key > > &vector) |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | pointer |
typedef | reference |
typedef | size_type |
typedef | value_type |
Detailed Description
template<typename T, typename Key = int>
class KSortableList< T, Key >
KSortableList is a QList which associates a key with each item in the list.
This key is used for sorting when calling sort().
This allows to temporarily calculate a key and use it for sorting, without having to store that key in the items, or calculate that key many times for the same item during sorting if that calculation is expensive.
Definition at line 144 of file ksortablelist.h.
Member Function Documentation
|
inline |
Insert a KSortableItem with the given values.
- Parameters
-
i the first value t the second value
Definition at line 152 of file ksortablelist.h.
|
inline |
Returns the first value of the KSortableItem at the given position.
- Returns
- the first value of the KSortableItem
Definition at line 161 of file ksortablelist.h.
|
inline |
Returns the first value of the KSortableItem at the given position.
- Returns
- the first value of the KSortableItem
Definition at line 169 of file ksortablelist.h.
|
inline |
Sorts the KSortableItems.
Definition at line 176 of file ksortablelist.h.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.