KDECore
#include <KTemporaryFile>
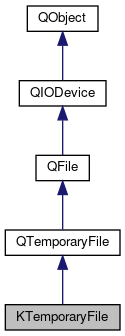
Public Member Functions | |
KTemporaryFile (const KComponentData &componentData=KGlobal::mainComponent()) | |
virtual | ~KTemporaryFile () |
void | setPrefix (const QString &prefix) |
void | setSuffix (const QString &suffix) |
![]() | |
QTemporaryFile () | |
QTemporaryFile (const QString &templateName) | |
QTemporaryFile (const QString &templateName, QObject *parent) | |
QTemporaryFile (QObject *parent) | |
~QTemporaryFile () | |
bool | autoRemove () const |
QString | fileName () const |
QString | fileTemplate () const |
bool | open () |
void | setAutoRemove (bool b) |
void | setFileTemplate (const QString &name) |
![]() | |
QFile (const QString &name) | |
QFile (QObject *parent) | |
QFile (const QString &name, QObject *parent) | |
~QFile () | |
virtual bool | atEnd () const |
virtual void | close () |
bool | copy (const QString &newName) |
FileError | error () const |
bool | exists () const |
QString | fileName () const |
bool | flush () |
int | handle () const |
virtual bool | isSequential () const |
bool | link (const QString &linkName) |
uchar * | map (qint64 offset, qint64 size, MemoryMapFlags flags) |
QString | name () const |
bool | open (QFlags< QIODevice::OpenModeFlag > aFlags, int fd) |
bool | open (FILE *fh, QFlags< QIODevice::OpenModeFlag > mode) |
bool | open (int fd, QFlags< QIODevice::OpenModeFlag > mode) |
bool | open (const RFile &f, QFlags< QIODevice::OpenModeFlag > mode, QFlags< QFile::FileHandleFlag > handleFlags) |
bool | open (FILE *fh, QFlags< QIODevice::OpenModeFlag > mode, QFlags< QFile::FileHandleFlag > handleFlags) |
bool | open (int fd, QFlags< QIODevice::OpenModeFlag > mode, QFlags< QFile::FileHandleFlag > handleFlags) |
bool | open (QFlags< QIODevice::OpenModeFlag > aFlags, FILE *f) |
Permissions | permissions () const |
virtual qint64 | pos () const |
QString | readLink () const |
bool | remove () |
bool | rename (const QString &newName) |
bool | resize (qint64 sz) |
virtual bool | seek (qint64 pos) |
void | setFileName (const QString &name) |
void | setName (const QString &name) |
bool | setPermissions (QFlags< QFile::Permission > permissions) |
virtual qint64 | size () const |
QString | symLinkTarget () const |
bool | unmap (uchar *address) |
void | unsetError () |
![]() | |
QIODevice () | |
QIODevice (QObject *parent) | |
virtual | ~QIODevice () |
void | aboutToClose () |
Offset | at () const |
bool | at (Offset offset) |
virtual qint64 | bytesAvailable () const |
virtual qint64 | bytesToWrite () const |
void | bytesWritten (qint64 bytes) |
virtual bool | canReadLine () const |
QString | errorString () const |
int | flags () const |
int | getch () |
bool | getChar (char *c) |
bool | isAsynchronous () const |
bool | isBuffered () const |
bool | isCombinedAccess () const |
bool | isDirectAccess () const |
bool | isInactive () const |
bool | isOpen () const |
bool | isRaw () const |
bool | isReadable () const |
bool | isSequentialAccess () const |
bool | isSynchronous () const |
bool | isTextModeEnabled () const |
bool | isTranslated () const |
bool | isWritable () const |
int | mode () const |
OpenMode | openMode () const |
qint64 | peek (char *data, qint64 maxSize) |
QByteArray | peek (qint64 maxSize) |
int | putch (int ch) |
bool | putChar (char c) |
qint64 | read (char *data, qint64 maxSize) |
QByteArray | read (qint64 maxSize) |
QByteArray | readAll () |
qint64 | readBlock (char *data, quint64 size) |
void | readChannelFinished () |
QByteArray | readLine (qint64 maxSize) |
qint64 | readLine (char *data, qint64 maxSize) |
void | readyRead () |
virtual bool | reset () |
void | resetStatus () |
void | setTextModeEnabled (bool enabled) |
int | state () const |
Status | status () const |
int | ungetch (int ch) |
void | ungetChar (char c) |
virtual bool | waitForBytesWritten (int msecs) |
virtual bool | waitForReadyRead (int msecs) |
qint64 | write (const char *data) |
qint64 | write (const char *data, qint64 maxSize) |
qint64 | write (const QByteArray &byteArray) |
qint64 | writeBlock (const QByteArray &data) |
qint64 | writeBlock (const char *data, quint64 size) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
QTemporaryFile * | createLocalFile (const QString &fileName) |
QTemporaryFile * | createLocalFile (QFile &file) |
![]() | |
bool | copy (const QString &fileName, const QString &newName) |
QString | decodeName (const QByteArray &localFileName) |
QString | decodeName (const char *localFileName) |
QByteArray | encodeName (const QString &fileName) |
bool | exists (const QString &fileName) |
bool | link (const QString &fileName, const QString &linkName) |
Permissions | permissions (const QString &fileName) |
QString | readLink (const QString &fileName) |
bool | remove (const QString &fileName) |
bool | rename (const QString &oldName, const QString &newName) |
bool | resize (const QString &fileName, qint64 sz) |
void | setDecodingFunction (DecoderFn function) |
void | setEncodingFunction (EncoderFn function) |
bool | setPermissions (const QString &fileName, QFlags< QFile::Permission > permissions) |
QString | symLinkTarget (const QString &fileName) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | DecoderFn |
typedef | EncoderFn |
typedef | FileHandleFlags |
typedef | Permissions |
typedef | PermissionSpec |
![]() | |
typedef | Offset |
typedef | OpenMode |
typedef | Status |
![]() | |
virtual bool | open (QFlags< QIODevice::OpenModeFlag > flags) |
![]() | |
virtual qint64 | readData (char *data, qint64 len) |
virtual qint64 | readLineData (char *data, qint64 maxlen) |
virtual qint64 | writeData (const char *data, qint64 len) |
![]() | |
virtual qint64 | readData (char *data, qint64 maxSize)=0 |
void | setErrorString (const QString &str) |
void | setOpenMode (QFlags< QIODevice::OpenModeFlag > openMode) |
virtual qint64 | writeData (const char *data, qint64 maxSize)=0 |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
A QTemporaryFile that will save in the KDE temp directory.
This class derives from QTemporaryFile and makes sure that your temporary files go in the temporary directory defined by KDE. (This is retrieved by using KStandardDirs to locate the "tmp" resource.) In general, whenever you would use a QTemporaryFile() use a KTemporaryFile() instead.
By default the filename will start with your application's instance name, followed by six random characters and an extension of ".tmp". You can use setPrefix() and setSuffix() to change the beginning and ending of the random name, as well as change the directory if you wish (read the descriptions of these functions for more information). For complex specifications, you may be better off calling QTemporaryFile::setFileTemplate() directly.
For example, let's make a new temporary file:
This temporary file will currently be stored in the default KDE temporary directory and have an extension of ".tmp". Now, let's change the directory:
Now the temporary file will be stored in "/var/lib/foodata" instead of the default KDE temporary directory, with an extension of ".tmp". It's important to remember the leading and trailing slashes to properly define the path! Next, let's change the suffix to a particular extension:
Now the temporary file will be stored in "/var/lib/foodata" and have an extension of ".pdf" instead of ".tmp".
Once you are done determining the name of the file, call open() to create the file.
If open() is unable to create the file it will return false. If the call to open() returns true you are ready to use your temporary file. If you don't want the file removed automatically when the KTemporaryFile object is destroyed, you need to call setAutoRemove(false), but make sure you have a good reason for leaving your temp files around.
- See also
- QTemporaryFile
Definition at line 92 of file ktemporaryfile.h.
Constructor & Destructor Documentation
|
explicit |
Construct a new KTemporaryFile.
The file will be stored in the temporary directory configured in KDE. The default prefix is the value of the default KDE temporary directory, plus your application's instance name. The default suffix is ".tmp".
- Parameters
-
componentData The KComponentData to use for the name of the file and to look up the directory.
Definition at line 43 of file ktemporaryfile.cpp.
|
virtual |
Destructor.
Definition at line 50 of file ktemporaryfile.cpp.
Member Function Documentation
void KTemporaryFile::setPrefix | ( | const QString & | prefix | ) |
Sets a prefix to use when creating the file.
This function sets a prefix to use when creating the file. The random part of the filename will come after this prefix. The prefix can also change or modify the target directory. If prefix
is an absolute path it will override the default temporary directory. If prefix
is a relative directory it will be relative to the default temporary location. To set a relative directory for the current working directory you should use QTemporaryFile::setFileTemplate() directly.
- Parameters
-
prefix The prefix to use when creating the file. Remember to end the prefix with a '/' if you are designating a directory.
Definition at line 55 of file ktemporaryfile.cpp.
void KTemporaryFile::setSuffix | ( | const QString & | suffix | ) |
Sets a suffix to use when creating the file.
Sets a suffix to use when creating the file. The random part of the filename will come before this suffix.
- Parameters
-
suffix The suffix to use when creating the file.
Definition at line 72 of file ktemporaryfile.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.