KDECore
#include <KUrl>
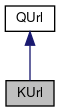
Classes | |
class | List |
Public Types | |
enum | AdjustPathOption { RemoveTrailingSlash, LeaveTrailingSlash, AddTrailingSlash } |
enum | CleanPathOption { SimplifyDirSeparators = 0x00, KeepDirSeparators = 0x01 } |
enum | DirectoryOption { ObeyTrailingSlash = 0x02, AppendTrailingSlash = 0x04, IgnoreTrailingSlash = 0x01 } |
enum | EncodedPathAndQueryOption { PermitEmptyPath =0x00, AvoidEmptyPath =0x01 } |
enum | EqualsOption { CompareWithoutTrailingSlash = 0x01, CompareWithoutFragment = 0x02, AllowEmptyPath = 0x04 } |
typedef QMap< QString, QString > | MetaDataMap |
enum | MimeDataFlags { DefaultMimeDataFlags = 0, NoTextExport = 1 } |
enum | QueryItemsOption { CaseInsensitiveKeys = 1 } |
Static Public Member Functions | |
static QString | decode_string (const QString &str) |
static QString | encode_string (const QString &str) |
static QString | encode_string_no_slash (const QString &str) |
static KUrl | fromMimeDataByteArray (const QByteArray &str) |
static KUrl | fromPath (const QString &text) |
static KUrl | fromPathOrUrl (const QString &text) |
static bool | isRelativeUrl (const QString &_url) |
static KUrl | join (const List &_list) |
static QString | relativePath (const QString &base_dir, const QString &path, bool *isParent=0) |
static QString | relativeUrl (const KUrl &base_url, const KUrl &url) |
static List | split (const QString &_url) |
static List | split (const KUrl &_url) |
![]() | |
void | decode (QString &url) |
void | encode (QString &url) |
QString | fromAce (const QByteArray &domain) |
QUrl | fromEncoded (const QByteArray &input) |
QUrl | fromEncoded (const QByteArray &input, ParsingMode parsingMode) |
QUrl | fromLocalFile (const QString &localFile) |
QString | fromPercentEncoding (const QByteArray &input) |
QString | fromPunycode (const QByteArray &pc) |
QUrl | fromUserInput (const QString &userInput) |
QStringList | idnWhitelist () |
bool | isRelativeUrl (const QString &url) |
void | setIdnWhitelist (const QStringList &list) |
QByteArray | toAce (const QString &domain) |
QByteArray | toPercentEncoding (const QString &input, const QByteArray &exclude, const QByteArray &include) |
QByteArray | toPunycode (const QString &uc) |
Related Functions | |
(Note that these are not member functions.) | |
bool | urlcmp (const QString &_url1, const QString &_url2) |
bool | urlcmp (const QString &_url1, const QString &_url2, const KUrl::EqualsOptions &options) |
Additional Inherited Members | |
![]() | |
typedef | FormattingOptions |
![]() | |
void | reset () |
Detailed Description
Represents and parses a URL.
A prototypical URL looks like:
KUrl handles escaping of URLs. This means that the specification of a full URL will differ from the corresponding string that would specify a local file or directory in file-operations like fopen. This is because an URL doesn't allow certain characters and escapes them. (e.g. '#'->"%23", space->"%20") (In a URL the hash-character '#' is used to specify a "reference", i.e. the position within a document).
The constructor KUrl(const QString&) expects a string properly escaped, or at least non-ambiguous. If you have the absolute path you should use KUrl::fromPath(const QString&).
If you have the URL of a local file or directory and need the absolute path, you would use toLocalFile().
This must also be considered when you have separated directory and file strings and need to put them together. While you can simply concatenate normal path strings, you must take care if the directory-part is already an escaped URL. (This might be needed if the user specifies a relative path, and your program supplies the rest from elsewhere.)
Wrong:
Instead you should use addPath(): Right:
Also consider that some URLs contain the password, but this shouldn't be visible. Your program should use prettyUrl() every time it displays a URL, whether in the GUI or in debug output or...
Note that prettyUrl() doesn't change the character escapes (like "%23"). Otherwise the URL would be invalid and the user wouldn't be able to use it in another context.
Member Typedef Documentation
Member Enumeration Documentation
option to be used in fileName and directory
Enumerator | |
---|---|
ObeyTrailingSlash |
This tells whether a trailing '/' should be ignored. If the flag is not set, for both If the flag is set, then everything behind the last '/'is considered to be the filename. So "hallo/torben" will be the path and the filename will be empty. |
AppendTrailingSlash |
tells whether the returned result should end with '/' or not. If the flag is set, '/' is added to the end of the path If the path is empty or just "/" then this flag has no effect. This option should only be used in directory(), it has no effect in fileName() |
IgnoreTrailingSlash |
Opposite of ObeyTrailingSlash (default) fileName("file:/foo/") and fileName("file:/foo") is "foo" in both cases. |
enum KUrl::EqualsOption |
Flags to be used in URL comparison functions like equals, or urlcmp.
Enumerator | |
---|---|
CompareWithoutTrailingSlash |
ignore trailing '/' characters. The paths "dir" and "dir/" are treated the same. Note however, that by default, the paths "" and "/" are not the same (For instance ftp://user@host redirects to ftp://user@host/home/user (on a linux server), while ftp://user@host/ is the root dir). This is also why path(RemoveTrailingSlash) for "/" returns "/" and not "". When dealing with web pages however, you should also set AllowEmptyPath so that no path and "/" are considered equal. |
CompareWithoutFragment |
disables comparison of HTML-style references. |
AllowEmptyPath |
Treat a URL with no path as equal to a URL with a path of "/", when CompareWithoutTrailingSlash is set. Example: KUrl::urlcmp("http://www.kde.org", "http://www.kde.org/", KUrl::CompareWithoutTrailingSlash | KUrl::AllowEmptyPath) returns true. This option is ignored if CompareWithoutTrailingSlash isn't set.
|
enum KUrl::MimeDataFlags |
Constructor & Destructor Documentation
KUrl::KUrl | ( | const QString & | urlOrPath | ) |
|
explicit |
|
explicit |
Constructor taking a QByteArray urlOrPath
, which is an encoded representation of the URL, exactly like the usual constructor.
This is useful when the URL, in its encoded form, is strictly ascii.
- Parameters
-
urlOrPath An encoded URL, or a path.
KUrl::KUrl | ( | const KUrl & | u | ) |
KUrl::KUrl | ( | const QUrl & | u | ) |
Constructor allowing relative URLs.
- Parameters
-
_baseurl The base url. _rel_url A relative or absolute URL. If this is an absolute URL then _baseurl
will be ignored. If this is a relative URL it will be combined with_baseurl
. Note that _rel_url should be encoded too, in any case. So do NOT pass a path here (use setPath or addPath instead).
Member Function Documentation
void KUrl::addPath | ( | const QString & | txt | ) |
Adds to the current path.
Assumes that the current path is a directory. txt
is appended to the current path. The function adds '/' if needed while concatenating. This means it does not matter whether the current path has a trailing '/' or not. If there is none, it becomes appended. If txt
has a leading '/' then this one is stripped.
- Parameters
-
txt The text to add. It is considered to be decoded.
Add an additional query item.
To replace an existing query item, the item should first be removed with removeQueryItem()
- Parameters
-
_item Name of item to add _value Value of item to add
void KUrl::adjustPath | ( | AdjustPathOption | trailing | ) |
Add or remove a trailing slash to/from the path.
If the URL has no path, then no '/' is added anyway. And on the other side: If the path is "/", then this character won't be stripped. Reason: "ftp://weis\@host" means something completely different than "ftp://weis\@host/". So adding or stripping the '/' would really alter the URL, while "ftp://host/path" and "ftp://host/path/" mean the same directory.
- Parameters
-
trailing RemoveTrailingSlash strips any trailing '/' and AddTrailingSlash adds a trailing '/' if there is none yet
Changes the directory by descending into the given directory.
It is assumed the current URL represents a directory. If dir
starts with a "/" the current URL will be "protocol://host/dir" otherwise _dir
will be appended to the path. _dir
can be ".." This function won't strip protocols. That means that when you are in file:///dir/dir2/my.tgz#tar:/ and you do cd("..") you will still be in file:///dir/dir2/my.tgz#tar:/
- Parameters
-
_dir the directory to change to
- Returns
- true if successful
void KUrl::cleanPath | ( | const CleanPathOption & | options = SimplifyDirSeparators | ) |
Resolves "." and ".." components in path.
Some servers seem not to like the removal of extra '/' even though it is against the specification in RFC 2396.
- Parameters
-
options use KeepDirSeparators if you don't want to remove consecutive occurrences of directory separator
The same as equals(), just with a less obvious name.
Compares this url with u
.
- Parameters
-
u the URL to compare this one with. ignore_trailing set to true to ignore trailing '/' characters.
- Returns
- True if both urls are the same. If at least one of the urls is invalid, false is returned.
- See also
- operator==. This function should be used if you want to ignore trailing '/' characters.
- Deprecated:
- Use equals() instead.
Decode %-style encoding and convert from local encoding to unicode.
Reverse of encode_string()
- Parameters
-
str String to decode (can be QString()).
- Deprecated:
- use QUrl::fromPercentEncoding(encodedURL) instead, but note that it takes a QByteArray and not a QString. Which makes sense since everything is 7 bit (ascii) when being percent-encoded.
QString KUrl::directory | ( | const DirectoryOptions & | options = IgnoreTrailingSlash | ) | const |
Returns the directory of the path.
- Parameters
-
options a set of DirectoryOption flags
- Returns
- The directory part of the current path. Everything between the last and the second last '/' is returned. For example
file:///hallo/torben/
would return "/hallo/torben/" whilefile:///hallo/torben
would return "hallo/". The returned string is decoded. QString() is returned when there is no path.
Convert unicoded string to local encoding and use %-style encoding for all common delimiters / non-ascii characters.
- Parameters
-
str String to encode (can be QString()).
- Returns
- the encoded string
- Deprecated:
- use QUrl::toPercentEncoding instead, but note that it returns a QByteArray and not a QString. Which makes sense since everything is 7 bit (ascii) after being percent-encoded.
Convert unicoded string to local encoding and use %-style encoding for all common delimiters / non-ascii characters as well as the slash '/'.
- Parameters
-
str String to encode
- Deprecated:
- use QUrl::toPercentEncoding(str,"/") instead, but note that it returns a QByteArray and not a QString. Which makes sense since everything is 7 bit (ascii) after being percent-encoded.
QString KUrl::encodedHtmlRef | ( | ) | const |
QString KUrl::encodedPathAndQuery | ( | AdjustPathOption | trailing = LeaveTrailingSlash , |
const EncodedPathAndQueryOptions & | options = PermitEmptyPath |
||
) | const |
Compares this url with u
.
- Parameters
-
u the URL to compare this one with. options a set of EqualsOption flags
- Returns
- True if both urls are the same. If at least one of the urls is invalid, false is returned.
- See also
- operator==. This function should be used if you want to set additional options, like ignoring trailing '/' characters.
QString KUrl::fileEncoding | ( | ) | const |
Returns encoding information from url, the content of the "charset" parameter.
- Returns
- An encoding suitable for QTextCodec::codecForName() or QString() if not encoding was specified.
QString KUrl::fileName | ( | const DirectoryOptions & | options = IgnoreTrailingSlash | ) | const |
|
static |
Creates a KUrl from a string, using the standard conventions for mime data (drag-n-drop or copy-n-paste).
Internally used by KUrl::List::fromMimeData, which is probably what you want to use instead.
- Deprecated:
- Since KDE4 you can pass both urls and paths to the KUrl constructors. Use KUrl(text) instead.
bool KUrl::hasHost | ( | ) | const |
bool KUrl::hasHTMLRef | ( | ) | const |
bool KUrl::hasPass | ( | ) | const |
bool KUrl::hasPath | ( | ) | const |
bool KUrl::hasRef | ( | ) | const |
Checks whether the URL has a reference/fragment part.
- Returns
- true if the URL has a reference part. In a URL like http://www.kde.org/kdebase.tar#tar:/README it would return true, too.
bool KUrl::hasSubUrl | ( | ) | const |
bool KUrl::hasUser | ( | ) | const |
QString KUrl::htmlRef | ( | ) | const |
bool KUrl::isLocalFile | ( | ) | const |
Checks whether the file is local.
- Returns
- true if the file is a plain local file (i.e. uses the file protocol and no hostname, or the local hostname). When isLocalFile returns true, you can use toLocalFile to read the file contents. Otherwise you need to use KIO (e.g. KIO::get).
Checks whether the given URL is parent of this URL.
For instance, ftp://host/dir/ is a parent of ftp://host/dir/subdir/subsubdir/.
- Returns
- true if this url is a parent of
u
(or the same URL asu
)
Convenience function.
Returns whether '_url' is likely to be a "relative" URL instead of an "absolute" URL.
This is mostly meant for KUrl(url, relativeUrl).
If you are looking for the notion of "relative path" (foo) vs "absolute path" (/foo), use QUrl::isRelative() instead. Indeed, isRelativeUrl() returns true for the string "/foo" since it doesn't contain a protocol, while KUrl("/foo").isRelative() is false since the KUrl constructor turns it into file:///foo. The two methods basically test the same thing, but this one takes a string (which is faster) while the class method requires a QUrl/KUrl which gives a more expected result, given the "magic" in the KUrl constructor.
- Parameters
-
_url URL to examine
- Returns
- true when the URL is likely to be "relative", false otherwise.
KUrl::operator QVariant | ( | ) | const |
|
inline |
QString KUrl::pass | ( | ) | const |
QString KUrl::path | ( | AdjustPathOption | trailing = LeaveTrailingSlash | ) | const |
QString KUrl::pathOrUrl | ( | ) | const |
Return the URL as a string, which will be either the URL (as prettyUrl would return) or, when the URL is a local file without query or ref, the path.
Use this method, to display URLs to the user. You can give the result of pathOrUrl back to the KUrl constructor, it accepts both paths and urls.
- Returns
- the new KUrl
QString KUrl::pathOrUrl | ( | AdjustPathOption | trailing | ) | const |
void KUrl::populateMimeData | ( | QMimeData * | mimeData, |
const MetaDataMap & | metaData = MetaDataMap() , |
||
MimeDataFlags | flags = DefaultMimeDataFlags |
||
) | const |
Adds URL data into the given QMimeData.
By default, populateMimeData also exports the URL as plain text, for e.g. dropping onto a text editor. But in some cases this might not be wanted, e.g. if adding other mime data which provides better plain text data.
WARNING: do not call this method multiple times, use KUrl::List::populateMimeData instead.
- Parameters
-
mimeData the QMimeData instance used to drag or copy this URL metaData KIO metadata shipped in the mime data, which is used for instance to set a correct HTTP referrer (some websites require it for downloading e.g. an image) flags set NoTextExport to prevent setting plain/text data into mimeData
In such a case, setExportAsText( false ) should be called.
QString KUrl::prettyUrl | ( | AdjustPathOption | trailing = LeaveTrailingSlash | ) | const |
Returns the URL as string in human-friendly format.
Example:
- Parameters
-
trailing use to add or remove a trailing slash to/from the path. see adjustPath.
- Returns
- A human readable URL, with no non-necessary encodings/escaped characters. Password will not be shown.
- See also
- url()
QString KUrl::protocol | ( | ) | const |
Returns the protocol for the URL (i.e., file, http, etc.), lowercased.
- See also
- QUrl::scheme
QString KUrl::query | ( | ) | const |
Returns the value of a certain query item.
This does the same as QUrl::queryItemValue(), except that it decodes "+" into " " in the value.
- Parameters
-
item Item whose value we want
- Returns
- the value of the given query item name or QString() if the specified item does not exist.
Returns the list of query items as a map mapping keys to values.
This does the same as QUrl::queryItems(), except that it decodes "+" into " " in the value, supports CaseInsensitiveKeys, and returns a different data type.
- Parameters
-
options any of QueryItemsOption ored together.
- Returns
- the map of query items or the empty map if the url has no query items.
QString KUrl::ref | ( | ) | const |
|
static |
Convenience function.
Returns a relative path based on base_dir
that points to path
.
- Parameters
-
base_dir the base directory to derive from path the new target directory isParent A pointer to a boolean which, if provided, will be set to reflect whether path
hasbase_dir
is a parent dir.
Convenience function.
Returns a "relative URL" based on base_url
that points to url
.
If no "relative URL" can be created, e.g. because the protocol and/or hostname differ between base_url
and url
an absolute URL is returned. Note that if base_url
represents a directory, it should contain a trailing slash.
- Parameters
-
base_url the URL to derive from url new URL
- See also
- adjustPath()
void KUrl::setDirectory | ( | const QString & | dir | ) |
void KUrl::setEncodedPathAndQuery | ( | const QString & | _txt | ) |
void KUrl::setFileEncoding | ( | const QString & | encoding | ) |
void KUrl::setFileName | ( | const QString & | _txt | ) |
Sets the filename of the path.
In comparison to addPath() this function does not assume that the current path is a directory. This is only assumed if the current path ends with '/'.
Any reference is reset.
- Parameters
-
_txt The filename to be set. It is considered to be decoded. If the current path ends with '/' then _txt
int just appended, otherwise all text behind the last '/' in the current path is erased and_txt
is appended then. It does not matter whether_txt
starts with '/' or not.
void KUrl::setHTMLRef | ( | const QString & | _ref | ) |
void KUrl::setPass | ( | const QString & | pass | ) |
void KUrl::setPath | ( | const QString & | path | ) |
void KUrl::setProtocol | ( | const QString & | proto | ) |
void KUrl::setQuery | ( | const QString & | query | ) |
void KUrl::setRef | ( | const QString & | fragment | ) |
Sets the reference/fragment part (everything after '#').
If you have an encoded fragment already (as a QByteArray), you can call setEncodedFragment directly.
- Parameters
-
fragment the encoded reference (or QString() to remove it).
void KUrl::setUser | ( | const QString & | user | ) |
|
static |
Splits nested URLs like file:///home/weis/kde.tgz#gzip:/#tar:/kdebase A URL like http://www.kde.org#tar:/kde/README.hml#ref1 will be split in http://www.kde.org and tar:/kde/README.html::ref1.
That means in turn that "#ref1" is an HTML-style reference and not a new sub URL. Since HTML-style references mark a certain position in a document this reference is appended to every URL. The idea behind this is that browsers, for example, only look at the first URL while the rest is not of interest to them.
- Parameters
-
_url The URL that has to be split.
- Returns
- An empty list on error or the list of split URLs.
- See also
- hasSubUrl
|
static |
Splits nested URLs like file:///home/weis/kde.tgz#gzip:/#tar:/kdebase A URL like http://www.kde.org#tar:/kde/README.hml#ref1 will be split in http://www.kde.org and tar:/kde/README.html::ref1.
That means in turn that "#ref1" is an HTML-style reference and not a new sub URL. Since HTML-style references mark a certain position in a document this reference is appended to every URL. The idea behind this is that browsers, for example, only look at the first URL while the rest is not of interest to them.
- Returns
- An empty list on error or the list of split URLs.
- Parameters
-
_url The URL that has to be split.
- See also
- hasSubUrl
QString KUrl::toLocalFile | ( | AdjustPathOption | trailing = LeaveTrailingSlash | ) | const |
QString KUrl::toMimeDataString | ( | ) | const |
Returns the URL as a string, using the standard conventions for mime data (drag-n-drop or copy-n-paste).
Internally used by KUrl::List::fromMimeData, which is probably what you want to use instead.
KUrl KUrl::upUrl | ( | ) | const |
This function is useful to implement the "Up" button in a file manager for example.
cd() never strips a sub-protocol. That means that if you are in file:///home/x.tgz#gzip:/#tar:/ and hit the up button you expect to see file:///home. The algorithm tries to go up on the right-most URL. If that is not possible it strips the right most URL. It continues stripping URLs.
- Returns
- a URL that is a level higher
QString KUrl::url | ( | AdjustPathOption | trailing = LeaveTrailingSlash | ) | const |
Returns the URL as string, with all escape sequences intact, encoded in a given charset.
This is used in particular for encoding URLs in UTF-8 before using them in a drag and drop operation. Please note that the string returned by url() will include the password of the URL. If you want to show the URL to the user, use prettyUrl().
- Parameters
-
trailing use to add or remove a trailing slash to/from the path. See adjustPath
- Returns
- The complete URL, with all escape sequences intact, encoded in a given charset.
- See also
- prettyUrl()
QString KUrl::user | ( | ) | const |
Friends And Related Function Documentation
Compares URLs. They are parsed, split and compared. Two malformed URLs with the same string representation are nevertheless considered to be unequal. That means no malformed URL equals anything else.
- Deprecated:
- use KUrl(_url1).equals(KUrl(_url2)) instead.
|
related |
Compares URLs. They are parsed, split and compared. Two malformed URLs with the same string representation are nevertheless considered to be unequal. That means no malformed URL equals anything else.
- Parameters
-
_url1 A reference URL _url2 A URL that will be compared with the reference URL options a set of KUrl::EqualsOption flags
- Deprecated:
- use KUrl(_url1).equals(KUrl(_url2), options) instead.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.