KDECore
#include <backgroundchecker.h>
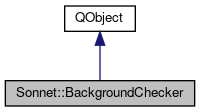
Public Slots | |
void | changeLanguage (const QString &lang) |
virtual void | continueChecking () |
void | replace (int start, const QString &oldText, const QString &newText) |
virtual void | start () |
virtual void | stop () |
Signals | |
void | done () |
void | misspelling (const QString &word, int start) |
Public Member Functions | |
BackgroundChecker (QObject *parent=0) | |
BackgroundChecker (const Speller &speller, QObject *parent=0) | |
~BackgroundChecker () | |
bool | addWordToPersonal (const QString &word) |
bool | checkWord (const QString &word) |
QString | currentContext () const |
void | restore (KConfig *config) |
void | setSpeller (const Speller &speller) |
void | setText (const QString &text) |
Speller | speller () const |
QStringList | suggest (const QString &word) const |
QString | text () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Slots | |
void | slotEngineDone () |
Protected Member Functions | |
virtual QString | fetchMoreText () |
virtual void | finishedCurrentFeed () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
BackgroundChecker is used to perform spell checking without blocking the application.
You can use it as is by calling the checkText function or subclass it and reimplement getMoreText function.
The misspelling signal is emitted whenever a misspelled word is found. The background checker stops right before emitting the signal. So the parent has to call continueChecking function to resume the checking.
done signal is emitted when whole text is spell checked.
Definition at line 54 of file backgroundchecker.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 40 of file backgroundchecker.cpp.
Definition at line 51 of file backgroundchecker.cpp.
BackgroundChecker::~BackgroundChecker | ( | ) |
Definition at line 63 of file backgroundchecker.cpp.
Member Function Documentation
Definition at line 120 of file backgroundchecker.cpp.
|
slot |
Definition at line 130 of file backgroundchecker.cpp.
Definition at line 115 of file backgroundchecker.cpp.
|
virtualslot |
After emitting misspelling signal the background checker stops.
The catcher is responsible for calling continueChecking function to resume checking.
Definition at line 135 of file backgroundchecker.cpp.
QString BackgroundChecker::currentContext | ( | ) | const |
Definition at line 159 of file backgroundchecker.cpp.
|
signal |
Emitted after the whole text has been spell checked.
|
protectedvirtual |
This function is called to get the text to spell check.
It will be called continuesly until it returns QString() in which case the done() signal is emitted. Note: the start parameter in mispelling() is not a combined position but a position in the last string returned by fetchMoreText. You need to store the state in the derivatives.
Definition at line 96 of file backgroundchecker.cpp.
|
protectedvirtual |
This function will be called whenever the background checker will be finished text which it got from fetchMoreText.
Definition at line 101 of file backgroundchecker.cpp.
|
signal |
Emitted whenever a misspelled word is found.
|
slot |
Definition at line 164 of file backgroundchecker.cpp.
void BackgroundChecker::restore | ( | KConfig * | config | ) |
Definition at line 68 of file backgroundchecker.cpp.
void BackgroundChecker::setSpeller | ( | const Speller & | speller | ) |
Definition at line 105 of file backgroundchecker.cpp.
void BackgroundChecker::setText | ( | const QString & | text | ) |
This method is used to spell check static text.
It automatically invokes start().
Use fetchMoreText() with start() to spell check a stream.
Definition at line 75 of file backgroundchecker.cpp.
|
protectedslot |
Definition at line 140 of file backgroundchecker.cpp.
Speller BackgroundChecker::speller | ( | ) | const |
Definition at line 110 of file backgroundchecker.cpp.
|
virtualslot |
Definition at line 82 of file backgroundchecker.cpp.
|
virtualslot |
Definition at line 91 of file backgroundchecker.cpp.
QStringList BackgroundChecker::suggest | ( | const QString & | word | ) | const |
Definition at line 125 of file backgroundchecker.cpp.
QString BackgroundChecker::text | ( | ) | const |
Definition at line 153 of file backgroundchecker.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:14 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.