KDECore
#include <kdecore_export.h>
#include <QtCore/QChar>
#include <QtCore/QLatin1Char>
#include <QtCore/QStringList>
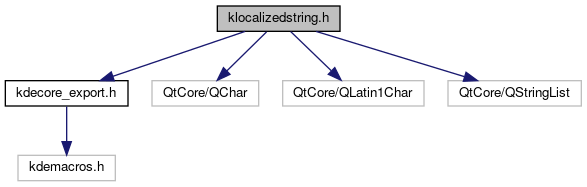

Go to the source code of this file.
Classes | |
class | I18nTypeCheck< T, s > |
class | I18nTypeCheck< char[s], s > |
class | KLocalizedString |
Macros | |
#define | I18N_ERR_MSG String_literal_as_second_argument_to_i18n___Perhaps_you_need_i18nc_or_i18np |
#define | I18N_NOOP(x) x |
#define | I18N_NOOP2(comment, x) x |
#define | I18N_NOOP2_NOSTRIP(ctxt, text) ctxt, text |
#define | STATIC_ASSERT_NOT_LITERAL_STRING(T) I18nTypeCheck<T, sizeof(T)>::I18N_ERR_MSG (); |
Functions | |
QString | i18n (const char *text) |
template<typename A1 > | |
QString | i18n (const char *text, const A1 &a1) |
template<typename A1 , typename A2 > | |
QString | i18n (const char *text, const A1 &a1, const A2 &a2) |
template<typename A1 , typename A2 , typename A3 > | |
QString | i18n (const char *text, const A1 &a1, const A2 &a2, const A3 &a3) |
template<typename A1 , typename A2 , typename A3 , typename A4 > | |
QString | i18n (const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 > | |
QString | i18n (const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 > | |
QString | i18n (const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 > | |
QString | i18n (const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 , typename A8 > | |
QString | i18n (const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7, const A8 &a8) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 , typename A8 , typename A9 > | |
QString | i18n (const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7, const A8 &a8, const A9 &a9) |
QString | i18nc (const char *ctxt, const char *text) |
template<typename A1 > | |
QString | i18nc (const char *ctxt, const char *text, const A1 &a1) |
template<typename A1 , typename A2 > | |
QString | i18nc (const char *ctxt, const char *text, const A1 &a1, const A2 &a2) |
template<typename A1 , typename A2 , typename A3 > | |
QString | i18nc (const char *ctxt, const char *text, const A1 &a1, const A2 &a2, const A3 &a3) |
template<typename A1 , typename A2 , typename A3 , typename A4 > | |
QString | i18nc (const char *ctxt, const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 > | |
QString | i18nc (const char *ctxt, const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 > | |
QString | i18nc (const char *ctxt, const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 > | |
QString | i18nc (const char *ctxt, const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 , typename A8 > | |
QString | i18nc (const char *ctxt, const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7, const A8 &a8) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 , typename A8 , typename A9 > | |
QString | i18nc (const char *ctxt, const char *text, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7, const A8 &a8, const A9 &a9) |
template<typename A1 > | |
QString | i18ncp (const char *ctxt, const char *sing, const char *plur, const A1 &a1) |
template<typename A1 , typename A2 > | |
QString | i18ncp (const char *ctxt, const char *sing, const char *plur, const A1 &a1, const A2 &a2) |
template<typename A1 , typename A2 , typename A3 > | |
QString | i18ncp (const char *ctxt, const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3) |
template<typename A1 , typename A2 , typename A3 , typename A4 > | |
QString | i18ncp (const char *ctxt, const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 > | |
QString | i18ncp (const char *ctxt, const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 > | |
QString | i18ncp (const char *ctxt, const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 > | |
QString | i18ncp (const char *ctxt, const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 , typename A8 > | |
QString | i18ncp (const char *ctxt, const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7, const A8 &a8) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 , typename A8 , typename A9 > | |
QString | i18ncp (const char *ctxt, const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7, const A8 &a8, const A9 &a9) |
template<typename A1 > | |
QString | i18np (const char *sing, const char *plur, const A1 &a1) |
template<typename A1 , typename A2 > | |
QString | i18np (const char *sing, const char *plur, const A1 &a1, const A2 &a2) |
template<typename A1 , typename A2 , typename A3 > | |
QString | i18np (const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3) |
template<typename A1 , typename A2 , typename A3 , typename A4 > | |
QString | i18np (const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 > | |
QString | i18np (const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 > | |
QString | i18np (const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 > | |
QString | i18np (const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 , typename A8 > | |
QString | i18np (const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7, const A8 &a8) |
template<typename A1 , typename A2 , typename A3 , typename A4 , typename A5 , typename A6 , typename A7 , typename A8 , typename A9 > | |
QString | i18np (const char *sing, const char *plur, const A1 &a1, const A2 &a2, const A3 &a3, const A4 &a4, const A5 &a5, const A6 &a6, const A7 &a7, const A8 &a8, const A9 &a9) |
KLocalizedString | ki18n (const char *msg) |
KLocalizedString | ki18nc (const char *ctxt, const char *msg) |
KLocalizedString | ki18ncp (const char *ctxt, const char *singular, const char *plural) |
KLocalizedString | ki18np (const char *singular, const char *plural) |
QString | tr2i18n (const char *message, const char *comment=0) |
Macro Definition Documentation
#define I18N_ERR_MSG String_literal_as_second_argument_to_i18n___Perhaps_you_need_i18nc_or_i18np |
Definition at line 615 of file klocalizedstring.h.
#define I18N_NOOP | ( | x | ) | x |
I18N_NOOP marks a string to be translated without translating it.
Do not use this unless you know you need it. http://developer.kde.org/documentation/other/developer-faq.html#q2.11.2
Example usage where say_something() returns either "hello" or "goodbye":
Definition at line 51 of file klocalizedstring.h.
#define I18N_NOOP2 | ( | comment, | |
x | |||
) | x |
If the string is too ambiguous to be translated well to a non-english language, use this instead of I18N_NOOP to separate lookup string and english.
Example usage where say_something() returns either "hello" or "goodbye":
- Warning
- You need to call i18nc(context, stringVar) later on, not just i18n(stringVar).
- See also
- I18N_NOOP2_NOSTRIP
Definition at line 72 of file klocalizedstring.h.
#define I18N_NOOP2_NOSTRIP | ( | ctxt, | |
text | |||
) | ctxt, text |
- Since
- 4.3
Similar to I18N_NOOP2, except that neither of the two arguments is omitted. This is typically used when contexts need to differ between static entries, and only some of the entries need context:
Note that i18nc() will not have any problems with context being null, it will simply behave as ordinary i18n().
Definition at line 102 of file klocalizedstring.h.
#define STATIC_ASSERT_NOT_LITERAL_STRING | ( | T | ) | I18nTypeCheck<T, sizeof(T)>::I18N_ERR_MSG (); |
Definition at line 618 of file klocalizedstring.h.
Function Documentation
|
inline |
Returns a localized version of a string.
- Parameters
-
text string to be localized
- Returns
- localized string
Definition at line 630 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 1 argument.
- Parameters
-
text string to be localized a1 first argument
- Returns
- localized string
Definition at line 642 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 2 arguments.
- Parameters
-
text string to be localized a1 first argument a2 second argument
- Returns
- localized string
Definition at line 656 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 3 arguments.
- Parameters
-
text string to be localized a1 first argument a2 second argument a3 third argument
- Returns
- localized string
Definition at line 671 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 4 arguments.
- Parameters
-
text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument
- Returns
- localized string
Definition at line 687 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 5 arguments.
- Parameters
-
text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument
- Returns
- localized string
Definition at line 704 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 6 arguments.
- Parameters
-
text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument
- Returns
- localized string
Definition at line 722 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 7 arguments.
- Parameters
-
text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument
- Returns
- localized string
Definition at line 741 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 8 arguments.
- Parameters
-
text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument a8 eighth argument
- Returns
- localized string
Definition at line 761 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 9 arguments.
- Parameters
-
text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument a8 eighth argument a9 ninth argument
- Returns
- localized string
Definition at line 782 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string and a context.
- Parameters
-
ctxt context of the string text string to be localized
- Returns
- localized string
Definition at line 797 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 1 argument and a context.
- Parameters
-
ctxt context of the string text string to be localized a1 first argument
- Returns
- localized string
Definition at line 810 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 2 arguments and a context.
- Parameters
-
ctxt context of the string text string to be localized a1 first argument a2 second argument
- Returns
- localized string
Definition at line 824 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 3 arguments and a context.
- Parameters
-
ctxt context of the string text string to be localized a1 first argument a2 second argument a3 third argument
- Returns
- localized string
Definition at line 839 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 4 arguments and a context.
- Parameters
-
ctxt context of the string text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument
- Returns
- localized string
Definition at line 855 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 5 arguments and a context.
- Parameters
-
ctxt context of the string text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument
- Returns
- localized string
Definition at line 872 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 6 arguments and a context.
- Parameters
-
ctxt context of the string text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument
- Returns
- localized string
Definition at line 890 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 7 arguments and a context.
- Parameters
-
ctxt context of the string text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument
- Returns
- localized string
Definition at line 909 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 8 arguments and a context.
- Parameters
-
ctxt context of the string text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument a8 eighth argument
- Returns
- localized string
Definition at line 929 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 9 arguments and a context.
- Parameters
-
ctxt context of the string text string to be localized a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument a8 eighth argument a9 ninth argument
- Returns
- localized string
Definition at line 950 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 1 argument and a context using correct plural form.
- Parameters
-
ctxt context of the string sing string to be localized in singular plur string to be localized in plural a1 first argument
- Returns
- localized string
Definition at line 1123 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 2 arguments and a context using correct plural form.
- Parameters
-
ctxt context of the string sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument
- Returns
- localized string
Definition at line 1138 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 3 arguments and a context using correct plural form.
- Parameters
-
ctxt context of the string sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument
- Returns
- localized string
Definition at line 1154 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 4 arguments and a context using correct plural form.
- Parameters
-
ctxt context of the string sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument
- Returns
- localized string
Definition at line 1171 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 5 arguments and a context using correct plural form.
- Parameters
-
ctxt context of the string sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument
- Returns
- localized string
Definition at line 1189 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 6 arguments and a context using correct plural form.
- Parameters
-
ctxt context of the string sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument
- Returns
- localized string
Definition at line 1208 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 7 arguments and a context using correct plural form.
- Parameters
-
ctxt context of the string sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument
- Returns
- localized string
Definition at line 1228 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 8 arguments and a context using correct plural form.
- Parameters
-
ctxt context of the string sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument a8 eighth argument
- Returns
- localized string
Definition at line 1249 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 9 arguments and a context using correct plural form.
- Parameters
-
ctxt context of the string sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument a8 eighth argument a9 ninth argument
- Returns
- localized string
Definition at line 1271 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 1 argument using correct plural form.
- Parameters
-
sing string to be localized in singular plur string to be localized in plural a1 first argument
- Returns
- localized string
Definition at line 966 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 2 arguments using correct plural form.
- Parameters
-
sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument
- Returns
- localized string
Definition at line 980 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 3 arguments using correct plural form.
- Parameters
-
sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument
- Returns
- localized string
Definition at line 995 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 4 arguments using correct plural form.
- Parameters
-
sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument
- Returns
- localized string
Definition at line 1011 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 5 arguments using correct plural form.
- Parameters
-
sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument
- Returns
- localized string
Definition at line 1028 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 6 arguments using correct plural form.
- Parameters
-
sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument
- Returns
- localized string
Definition at line 1046 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 7 arguments using correct plural form.
- Parameters
-
sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument
- Returns
- localized string
Definition at line 1065 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 8 arguments using correct plural form.
- Parameters
-
sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument a8 eighth argument
- Returns
- localized string
Definition at line 1085 of file klocalizedstring.h.
|
inline |
Returns a localized version of a string with 9 arguments using correct plural form.
- Parameters
-
sing string to be localized in singular plur string to be localized in plural a1 first argument a2 second argument a3 third argument a4 fourth argument a5 fifth argument a6 sixth argument a7 seventh argument a8 eighth argument a9 ninth argument
- Returns
- localized string
Definition at line 1106 of file klocalizedstring.h.
KLocalizedString ki18n | ( | const char * | msg | ) |
Creates localized string from a given message.
Normaly you should use i18n() templates instead, as you need real KLocalizedString object only in special cases. All text arguments must be UTF-8 encoded and must not be empty or NULL.
- Parameters
-
msg message text
- Returns
- created KLocalizedString
Definition at line 924 of file klocalizedstring.cpp.
KLocalizedString ki18nc | ( | const char * | ctxt, |
const char * | msg | ||
) |
Creates localized string from a given message, with added context.
Context is only for disambiguation purposes (both for lookup and for translators), it is not part of the message. Normaly you should use i18nc() templates instead, as you need real KLocalizedString object only in special cases. All text arguments must be UTF-8 encoded and must not be empty or NULL.
- Parameters
-
ctxt context text msg message text
- Returns
- created KLocalizedString
Definition at line 929 of file klocalizedstring.cpp.
KLocalizedString ki18ncp | ( | const char * | ctxt, |
const char * | singular, | ||
const char * | plural | ||
) |
Creates localized string from a given plural and singular form, with added context.
Context is only for disambiguation purposes (both for lookup and for translators), it is not part of the message. Normaly you should use i18ncp() templates instead, as you need real KLocalizedString object only in special cases. All text arguments must be UTF-8 encoded and must not be empty or NULL.
- Parameters
-
ctxt context text singular message text in singular plural message text in plural
- Returns
- created KLocalizedString
Definition at line 939 of file klocalizedstring.cpp.
KLocalizedString ki18np | ( | const char * | singular, |
const char * | plural | ||
) |
Creates localized string from a given plural and singular form.
Normaly you should use i18np() templates instead, as you need real KLocalizedString object only in special cases. All text arguments must be UTF-8 encoded and must not be empty or NULL.
- Parameters
-
singular message text in singular plural message text in plural
- Returns
- created KLocalizedString
Definition at line 934 of file klocalizedstring.cpp.
|
inline |
Qt's uic generated translation calls go through numerous indirections unnecessary in our case.
So we use uic -tr tr2i18n to redirect them to our i18n API.
Definition at line 602 of file klocalizedstring.h.
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.