KDEUI
#include <kabstractwidgetjobtracker.h>
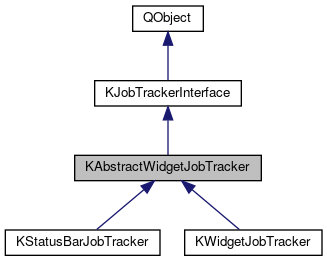
Public Slots | |
virtual void | registerJob (KJob *job) |
virtual void | unregisterJob (KJob *job) |
![]() | |
virtual void | registerJob (KJob *job) |
virtual void | unregisterJob (KJob *job) |
Signals | |
void | resume (KJob *job) |
void | stopped (KJob *job) |
void | suspend (KJob *job) |
Public Member Functions | |
KAbstractWidgetJobTracker (QWidget *parent=0) | |
virtual | ~KAbstractWidgetJobTracker () |
bool | autoDelete (KJob *job) const |
void | setAutoDelete (KJob *job, bool autoDelete) |
void | setStopOnClose (KJob *job, bool stopOnClose) |
bool | stopOnClose (KJob *job) const |
virtual QWidget * | widget (KJob *job)=0 |
![]() | |
KJobTrackerInterface (QObject *parent=0) | |
virtual | ~KJobTrackerInterface () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Slots | |
virtual void | finished (KJob *job) |
virtual void | slotClean (KJob *job) |
virtual void | slotResume (KJob *job) |
virtual void | slotStop (KJob *job) |
virtual void | slotSuspend (KJob *job) |
![]() | |
virtual void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1, const QPair< QString, QString > &field2) |
virtual void | finished (KJob *job) |
virtual void | infoMessage (KJob *job, const QString &plain, const QString &rich) |
virtual void | percent (KJob *job, unsigned long percent) |
virtual void | processedAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
virtual void | resumed (KJob *job) |
virtual void | speed (KJob *job, unsigned long value) |
virtual void | suspended (KJob *job) |
virtual void | totalAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
virtual void | warning (KJob *job, const QString &plain, const QString &rich) |
Protected Attributes | |
Private *const | d |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
The base class for widget based job trackers.
Definition at line 34 of file kabstractwidgetjobtracker.h.
Constructor & Destructor Documentation
KAbstractWidgetJobTracker::KAbstractWidgetJobTracker | ( | QWidget * | parent = 0 | ) |
Creates a new KAbstractWidgetJobTracker.
- Parameters
-
parent the parent of this object and of the widget displaying the job progresses
Definition at line 32 of file kabstractwidgetjobtracker.cpp.
|
virtual |
Destroys a KAbstractWidgetJobTracker.
Definition at line 38 of file kabstractwidgetjobtracker.cpp.
Member Function Documentation
Checks whether the dialog should be deleted or cleaned.
- Parameters
-
job the job's widget that will be auto-deleted
- Returns
- false if the dialog only calls slotClean, true if it will be deleted
- See also
- setAutoDelete()
Definition at line 68 of file kabstractwidgetjobtracker.cpp.
|
protectedvirtualslot |
Called when a job is finished, in any case.
It is used to notify that the job is terminated and that progress UI (if any) can be hidden.
- Parameters
-
job the job that emitted this signal
Definition at line 73 of file kabstractwidgetjobtracker.cpp.
|
virtualslot |
Register a new job in this tracker.
Note that job trackers inheriting from this class can have only one job registered at a time.
- Parameters
-
job the job to register
Reimplemented in KStatusBarJobTracker.
Definition at line 43 of file kabstractwidgetjobtracker.cpp.
|
signal |
Emitted when the user resumed the operation.
- Parameters
-
job The job that has been resumed
This controls whether the dialog should be deleted or only cleaned when the KJob is finished (or canceled).
If your dialog is an embedded widget and not a separate window, you should setAutoDelete(false) in the constructor of your custom dialog.
- Parameters
-
job the job's widget that is going to be auto-deleted autoDelete If false the dialog will only call method slotClean. If true the dialog will be deleted.
- See also
- autoDelete()
Definition at line 63 of file kabstractwidgetjobtracker.cpp.
This controls whether the job should be canceled if the dialog is closed.
- Parameters
-
job the job's widget that will be stopped when closing stopOnClose If true the job will be stopped if the dialog is closed, otherwise the job will continue even on close.
- See also
- stopOnClose()
Definition at line 53 of file kabstractwidgetjobtracker.cpp.
|
protectedvirtualslot |
This method is called when the widget should be cleaned (after job is finished).
redefine this for custom behavior.
- Parameters
-
job The job that is being cleaned
Definition at line 102 of file kabstractwidgetjobtracker.cpp.
|
protectedvirtualslot |
This method should be called for pause/resume Connect this to the progress widgets buttons etc.
- Parameters
-
job The job that is being resumed
Definition at line 94 of file kabstractwidgetjobtracker.cpp.
|
protectedvirtualslot |
This method should be called for correct cancellation of IO operation Connect this to the progress widgets buttons etc.
- Parameters
-
job The job that is being stopped
Definition at line 78 of file kabstractwidgetjobtracker.cpp.
|
protectedvirtualslot |
This method should be called for pause/resume Connect this to the progress widgets buttons etc.
- Parameters
-
job The job that is being suspended
Definition at line 86 of file kabstractwidgetjobtracker.cpp.
Checks whether the job will be killed when the dialog is closed.
- Parameters
-
job the job's widget that will be stopped when closing
- Returns
- true if the job is killed on close event, false otherwise.
- See also
- setStopOnClose()
Definition at line 58 of file kabstractwidgetjobtracker.cpp.
|
signal |
Emitted when the user aborted the operation.
- Parameters
-
job The job that has been stopped
|
signal |
Emitted when the user suspended the operation.
- Parameters
-
job The job that has been suspended
|
virtualslot |
Unregister a job from this tracker.
- Parameters
-
job the job to unregister
Reimplemented in KStatusBarJobTracker.
Definition at line 48 of file kabstractwidgetjobtracker.cpp.
The widget associated to this tracker.
- Parameters
-
job the job that is assigned the widget we want to return
- Returns
- the widget displaying the job progresses
Implemented in KStatusBarJobTracker, and KWidgetJobTracker.
Member Data Documentation
|
protected |
Definition at line 185 of file kabstractwidgetjobtracker.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:01 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.