KDEUI
#include <kcolordialog.h>
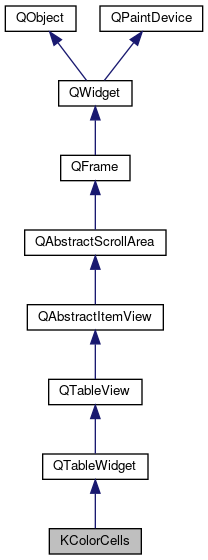
Signals | |
void | colorDoubleClicked (int index, const QColor &color) |
void | colorSelected (int index, const QColor &color) |
Public Member Functions | |
KColorCells (QWidget *parent, int rows, int columns) | |
~KColorCells () | |
bool | acceptDrags () const |
QColor | color (int index) const |
int | count () const |
int | selectedIndex () const |
void | setAcceptDrags (bool acceptDrags) |
void | setColor (int index, const QColor &col) |
void | setSelected (int index) |
void | setShading (bool shade) |
bool | shading () const |
![]() | |
QTableWidget (QWidget *parent) | |
QTableWidget (int rows, int columns, QWidget *parent) | |
~QTableWidget () | |
void | cellActivated (int row, int column) |
void | cellChanged (int row, int column) |
void | cellClicked (int row, int column) |
void | cellDoubleClicked (int row, int column) |
void | cellEntered (int row, int column) |
void | cellPressed (int row, int column) |
QWidget * | cellWidget (int row, int column) const |
void | clear () |
void | clearContents () |
void | closePersistentEditor (QTableWidgetItem *item) |
int | column (const QTableWidgetItem *item) const |
int | columnCount () const |
void | currentCellChanged (int currentRow, int currentColumn, int previousRow, int previousColumn) |
int | currentColumn () const |
QTableWidgetItem * | currentItem () const |
void | currentItemChanged (QTableWidgetItem *current, QTableWidgetItem *previous) |
int | currentRow () const |
void | editItem (QTableWidgetItem *item) |
QList< QTableWidgetItem * > | findItems (const QString &text, QFlags< Qt::MatchFlag > flags) const |
QTableWidgetItem * | horizontalHeaderItem (int column) const |
void | insertColumn (int column) |
void | insertRow (int row) |
bool | isItemSelected (const QTableWidgetItem *item) const |
QTableWidgetItem * | item (int row, int column) const |
void | itemActivated (QTableWidgetItem *item) |
QTableWidgetItem * | itemAt (const QPoint &point) const |
QTableWidgetItem * | itemAt (int ax, int ay) const |
void | itemChanged (QTableWidgetItem *item) |
void | itemClicked (QTableWidgetItem *item) |
void | itemDoubleClicked (QTableWidgetItem *item) |
void | itemEntered (QTableWidgetItem *item) |
void | itemPressed (QTableWidgetItem *item) |
const QTableWidgetItem * | itemPrototype () const |
void | itemSelectionChanged () |
void | openPersistentEditor (QTableWidgetItem *item) |
void | removeCellWidget (int row, int column) |
void | removeColumn (int column) |
void | removeRow (int row) |
int | row (const QTableWidgetItem *item) const |
int | rowCount () const |
void | scrollToItem (const QTableWidgetItem *item, QAbstractItemView::ScrollHint hint) |
QList< QTableWidgetItem * > | selectedItems () |
QList< QTableWidgetSelectionRange > | selectedRanges () const |
void | setCellWidget (int row, int column, QWidget *widget) |
void | setColumnCount (int columns) |
void | setCurrentCell (int row, int column) |
void | setCurrentCell (int row, int column, QFlags< QItemSelectionModel::SelectionFlag > command) |
void | setCurrentItem (QTableWidgetItem *item) |
void | setCurrentItem (QTableWidgetItem *item, QFlags< QItemSelectionModel::SelectionFlag > command) |
void | setHorizontalHeaderItem (int column, QTableWidgetItem *item) |
void | setHorizontalHeaderLabels (const QStringList &labels) |
void | setItem (int row, int column, QTableWidgetItem *item) |
void | setItemPrototype (const QTableWidgetItem *item) |
void | setItemSelected (const QTableWidgetItem *item, bool select) |
void | setRangeSelected (const QTableWidgetSelectionRange &range, bool select) |
void | setRowCount (int rows) |
void | setVerticalHeaderItem (int row, QTableWidgetItem *item) |
void | setVerticalHeaderLabels (const QStringList &labels) |
void | sortItems (int column, Qt::SortOrder order) |
QTableWidgetItem * | takeHorizontalHeaderItem (int column) |
QTableWidgetItem * | takeItem (int row, int column) |
QTableWidgetItem * | takeVerticalHeaderItem (int row) |
QTableWidgetItem * | verticalHeaderItem (int row) const |
int | visualColumn (int logicalColumn) const |
QRect | visualItemRect (const QTableWidgetItem *item) const |
int | visualRow (int logicalRow) const |
![]() | |
QTableView (QWidget *parent) | |
~QTableView () | |
void | clearSpans () |
int | columnAt (int x) const |
int | columnSpan (int row, int column) const |
int | columnViewportPosition (int column) const |
int | columnWidth (int column) const |
Qt::PenStyle | gridStyle () const |
void | hideColumn (int column) |
void | hideRow (int row) |
QHeaderView * | horizontalHeader () const |
virtual QModelIndex | indexAt (const QPoint &pos) const |
bool | isColumnHidden (int column) const |
bool | isCornerButtonEnabled () const |
bool | isRowHidden (int row) const |
bool | isSortingEnabled () const |
void | resizeColumnsToContents () |
void | resizeColumnToContents (int column) |
void | resizeRowsToContents () |
void | resizeRowToContents (int row) |
int | rowAt (int y) const |
int | rowHeight (int row) const |
int | rowSpan (int row, int column) const |
int | rowViewportPosition (int row) const |
void | selectColumn (int column) |
void | selectRow (int row) |
void | setColumnHidden (int column, bool hide) |
void | setColumnWidth (int column, int width) |
void | setCornerButtonEnabled (bool enable) |
void | setGridStyle (Qt::PenStyle style) |
void | setHorizontalHeader (QHeaderView *header) |
virtual void | setModel (QAbstractItemModel *model) |
virtual void | setRootIndex (const QModelIndex &index) |
void | setRowHeight (int row, int height) |
void | setRowHidden (int row, bool hide) |
virtual void | setSelectionModel (QItemSelectionModel *selectionModel) |
void | setShowGrid (bool show) |
void | setSortingEnabled (bool enable) |
void | setSpan (int row, int column, int rowSpanCount, int columnSpanCount) |
void | setVerticalHeader (QHeaderView *header) |
void | setWordWrap (bool on) |
void | showColumn (int column) |
bool | showGrid () const |
void | showRow (int row) |
void | sortByColumn (int column) |
void | sortByColumn (int column, Qt::SortOrder order) |
QHeaderView * | verticalHeader () const |
bool | wordWrap () const |
![]() | |
QAbstractItemView (QWidget *parent) | |
~QAbstractItemView () | |
void | activated (const QModelIndex &index) |
bool | alternatingRowColors () const |
int | autoScrollMargin () const |
void | clearSelection () |
void | clicked (const QModelIndex &index) |
void | closePersistentEditor (const QModelIndex &index) |
QModelIndex | currentIndex () const |
Qt::DropAction | defaultDropAction () const |
void | doubleClicked (const QModelIndex &index) |
DragDropMode | dragDropMode () const |
bool | dragDropOverwriteMode () const |
bool | dragEnabled () const |
void | edit (const QModelIndex &index) |
EditTriggers | editTriggers () const |
void | entered (const QModelIndex &index) |
bool | hasAutoScroll () const |
ScrollMode | horizontalScrollMode () const |
QSize | iconSize () const |
virtual QModelIndex | indexAt (const QPoint &point) const =0 |
QWidget * | indexWidget (const QModelIndex &index) const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
QAbstractItemDelegate * | itemDelegate () const |
QAbstractItemDelegate * | itemDelegate (const QModelIndex &index) const |
QAbstractItemDelegate * | itemDelegateForColumn (int column) const |
QAbstractItemDelegate * | itemDelegateForRow (int row) const |
virtual void | keyboardSearch (const QString &search) |
QAbstractItemModel * | model () const |
void | openPersistentEditor (const QModelIndex &index) |
void | pressed (const QModelIndex &index) |
virtual void | reset () |
QModelIndex | rootIndex () const |
virtual void | scrollTo (const QModelIndex &index, ScrollHint hint)=0 |
void | scrollToBottom () |
void | scrollToTop () |
virtual void | selectAll () |
QAbstractItemView::SelectionBehavior | selectionBehavior () const |
QAbstractItemView::SelectionMode | selectionMode () const |
QItemSelectionModel * | selectionModel () const |
void | setAlternatingRowColors (bool enable) |
void | setAutoScroll (bool enable) |
void | setAutoScrollMargin (int margin) |
void | setCurrentIndex (const QModelIndex &index) |
void | setDefaultDropAction (Qt::DropAction dropAction) |
void | setDragDropMode (DragDropMode behavior) |
void | setDragDropOverwriteMode (bool overwrite) |
void | setDragEnabled (bool enable) |
void | setDropIndicatorShown (bool enable) |
void | setEditTriggers (QFlags< QAbstractItemView::EditTrigger > triggers) |
void | setHorizontalScrollMode (ScrollMode mode) |
void | setIconSize (const QSize &size) |
void | setIndexWidget (const QModelIndex &index, QWidget *widget) |
void | setItemDelegate (QAbstractItemDelegate *delegate) |
void | setItemDelegateForColumn (int column, QAbstractItemDelegate *delegate) |
void | setItemDelegateForRow (int row, QAbstractItemDelegate *delegate) |
void | setSelectionBehavior (QAbstractItemView::SelectionBehavior behavior) |
void | setSelectionMode (QAbstractItemView::SelectionMode mode) |
void | setTabKeyNavigation (bool enable) |
void | setTextElideMode (Qt::TextElideMode mode) |
void | setVerticalScrollMode (ScrollMode mode) |
bool | showDropIndicator () const |
QSize | sizeHintForIndex (const QModelIndex &index) const |
bool | tabKeyNavigation () const |
Qt::TextElideMode | textElideMode () const |
void | update (const QModelIndex &index) |
ScrollMode | verticalScrollMode () const |
void | viewportEntered () |
virtual QRect | visualRect (const QModelIndex &index) const =0 |
![]() | |
QAbstractScrollArea (QWidget *parent) | |
~QAbstractScrollArea () | |
void | addScrollBarWidget (QWidget *widget, QFlags< Qt::AlignmentFlag > alignment) |
QWidget * | cornerWidget () const |
QScrollBar * | horizontalScrollBar () const |
Qt::ScrollBarPolicy | horizontalScrollBarPolicy () const |
QSize | maximumViewportSize () const |
virtual QSize | minimumSizeHint () const |
QWidgetList | scrollBarWidgets (QFlags< Qt::AlignmentFlag > alignment) |
void | setCornerWidget (QWidget *widget) |
void | setHorizontalScrollBar (QScrollBar *scrollBar) |
void | setHorizontalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setVerticalScrollBar (QScrollBar *scrollBar) |
void | setVerticalScrollBarPolicy (Qt::ScrollBarPolicy) |
void | setViewport (QWidget *widget) |
virtual QSize | sizeHint () const |
QScrollBar * | verticalScrollBar () const |
Qt::ScrollBarPolicy | verticalScrollBarPolicy () const |
QWidget * | viewport () const |
![]() | |
QFrame (QWidget *parent, QFlags< Qt::WindowType > f) | |
QFrame (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QFrame () | |
QRect | frameRect () const |
Shadow | frameShadow () const |
Shape | frameShape () const |
int | frameStyle () const |
int | frameWidth () const |
int | lineWidth () const |
int | midLineWidth () const |
void | setFrameRect (const QRect &) |
void | setFrameShadow (Shadow) |
void | setFrameShape (Shape) |
void | setFrameStyle (int style) |
void | setLineWidth (int) |
void | setMidLineWidth (int) |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Protected Member Functions | |
virtual void | dragEnterEvent (QDragEnterEvent *) |
virtual void | dragMoveEvent (QDragMoveEvent *) |
virtual void | dropEvent (QDropEvent *) |
virtual void | mouseDoubleClickEvent (QMouseEvent *) |
virtual void | mouseMoveEvent (QMouseEvent *) |
virtual void | mousePressEvent (QMouseEvent *) |
virtual void | mouseReleaseEvent (QMouseEvent *) |
int | positionToCell (const QPoint &pos, bool ignoreBorders=false) const |
virtual void | resizeEvent (QResizeEvent *event) |
virtual int | sizeHintForColumn (int column) const |
virtual int | sizeHintForRow (int column) const |
![]() | |
virtual bool | dropMimeData (int row, int column, const QMimeData *data, Qt::DropAction action) |
virtual bool | event (QEvent *e) |
QModelIndex | indexFromItem (QTableWidgetItem *item) const |
QTableWidgetItem * | itemFromIndex (const QModelIndex &index) const |
QList< QTableWidgetItem * > | items (const QMimeData *data) const |
virtual QMimeData * | mimeData (const QList< QTableWidgetItem * > items) const |
virtual QStringList | mimeTypes () const |
virtual Qt::DropActions | supportedDropActions () const |
![]() | |
void | columnCountChanged (int oldCount, int newCount) |
void | columnMoved (int column, int oldIndex, int newIndex) |
void | columnResized (int column, int oldWidth, int newWidth) |
virtual void | currentChanged (const QModelIndex ¤t, const QModelIndex &previous) |
virtual int | horizontalOffset () const |
virtual bool | isIndexHidden (const QModelIndex &index) const |
virtual QModelIndex | moveCursor (CursorAction cursorAction, QFlags< Qt::KeyboardModifier > modifiers) |
virtual void | paintEvent (QPaintEvent *event) |
void | rowCountChanged (int oldCount, int newCount) |
void | rowMoved (int row, int oldIndex, int newIndex) |
void | rowResized (int row, int oldHeight, int newHeight) |
virtual QModelIndexList | selectedIndexes () const |
virtual void | selectionChanged (const QItemSelection &selected, const QItemSelection &deselected) |
virtual void | setSelection (const QRect &rect, QFlags< QItemSelectionModel::SelectionFlag > flags) |
virtual void | timerEvent (QTimerEvent *event) |
virtual void | updateGeometries () |
virtual int | verticalOffset () const |
virtual QStyleOptionViewItem | viewOptions () const |
![]() | |
virtual void | closeEditor (QWidget *editor, QAbstractItemDelegate::EndEditHint hint) |
virtual void | commitData (QWidget *editor) |
virtual void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight) |
QPoint | dirtyRegionOffset () const |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
DropIndicatorPosition | dropIndicatorPosition () const |
virtual bool | edit (const QModelIndex &index, EditTrigger trigger, QEvent *event) |
virtual void | editorDestroyed (QObject *editor) |
void | executeDelayedItemsLayout () |
virtual void | focusInEvent (QFocusEvent *event) |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
virtual int | horizontalOffset () const =0 |
int | horizontalStepsPerItem () const |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual bool | isIndexHidden (const QModelIndex &index) const =0 |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual QModelIndex | moveCursor (CursorAction cursorAction, QFlags< Qt::KeyboardModifier > modifiers)=0 |
virtual void | rowsAboutToBeRemoved (const QModelIndex &parent, int start, int end) |
virtual void | rowsInserted (const QModelIndex &parent, int start, int end) |
void | scheduleDelayedItemsLayout () |
void | scrollDirtyRegion (int dx, int dy) |
virtual QItemSelectionModel::SelectionFlags | selectionCommand (const QModelIndex &index, const QEvent *event) const |
void | setDirtyRegion (const QRegion ®ion) |
void | setHorizontalStepsPerItem (int steps) |
virtual void | setSelection (const QRect &rect, QFlags< QItemSelectionModel::SelectionFlag > flags)=0 |
void | setState (State state) |
void | setVerticalStepsPerItem (int steps) |
virtual void | startDrag (QFlags< Qt::DropAction > supportedActions) |
State | state () const |
virtual int | verticalOffset () const =0 |
int | verticalStepsPerItem () const |
virtual bool | viewportEvent (QEvent *event) |
virtual QRegion | visualRegionForSelection (const QItemSelection &selection) const =0 |
![]() | |
virtual void | contextMenuEvent (QContextMenuEvent *e) |
virtual void | scrollContentsBy (int dx, int dy) |
void | setupViewport (QWidget *viewport) |
void | setViewportMargins (const QMargins &margins) |
void | setViewportMargins (int left, int top, int right, int bottom) |
virtual void | wheelEvent (QWheelEvent *e) |
![]() | |
virtual void | changeEvent (QEvent *ev) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | enterEvent (QEvent *event) |
bool | focusNextChild () |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
![]() | |
QPaintDevice () | |
Additional Inherited Members | |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
![]() | |
typedef | EditTriggers |
![]() | |
typedef | RenderFlags |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
A table of editable color cells.
Definition at line 40 of file kcolordialog.h.
Constructor & Destructor Documentation
KColorCells::KColorCells | ( | QWidget * | parent, |
int | rows, | ||
int | columns | ||
) |
Constructs a new table of color cells, consisting of rows
* columns
colors.
- Parameters
-
parent The parent of the new widget rows The number of rows in the table columns The number of columns in the table
Definition at line 182 of file kcolordialog.cpp.
KColorCells::~KColorCells | ( | ) |
Definition at line 211 of file kcolordialog.cpp.
Member Function Documentation
bool KColorCells::acceptDrags | ( | ) | const |
QColor KColorCells::color | ( | int | index | ) | const |
Returns the color at a given index in the table.
Definition at line 216 of file kcolordialog.cpp.
|
signal |
Emitted when a color in the table is double-clicked.
|
signal |
Emitted when a color is selected in the table.
int KColorCells::count | ( | ) | const |
Returns the total number of color cells in the table.
Definition at line 226 of file kcolordialog.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 396 of file kcolordialog.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 406 of file kcolordialog.cpp.
|
protectedvirtual |
Reimplemented from QTableWidget.
Definition at line 415 of file kcolordialog.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 458 of file kcolordialog.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 372 of file kcolordialog.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 336 of file kcolordialog.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 428 of file kcolordialog.cpp.
Definition at line 345 of file kcolordialog.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 311 of file kcolordialog.cpp.
int KColorCells::selectedIndex | ( | ) | const |
Returns the index of the cell which is currently selected.
Definition at line 258 of file kcolordialog.cpp.
void KColorCells::setAcceptDrags | ( | bool | acceptDrags | ) |
Definition at line 241 of file kcolordialog.cpp.
void KColorCells::setColor | ( | int | index, |
const QColor & | col | ||
) |
Sets the color in the given index in the table.
Definition at line 263 of file kcolordialog.cpp.
void KColorCells::setSelected | ( | int | index | ) |
Sets the currently selected cell to index
.
Definition at line 251 of file kcolordialog.cpp.
void KColorCells::setShading | ( | bool | shade | ) |
Definition at line 231 of file kcolordialog.cpp.
bool KColorCells::shading | ( | ) | const |
|
protectedvirtual |
Reimplemented from QTableView.
Definition at line 326 of file kcolordialog.cpp.
|
protectedvirtual |
Reimplemented from QTableView.
Definition at line 331 of file kcolordialog.cpp.
Property Documentation
|
readwrite |
Definition at line 43 of file kcolordialog.h.
|
readwrite |
Definition at line 44 of file kcolordialog.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:01 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.