KDEUI
#include <kglobalaccel.h>
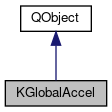
Public Types | |
enum | actionIdFields { ComponentUnique = 0, ActionUnique = 1, ComponentFriendly = 2, ActionFriendly = 3 } |
Public Member Functions | |
QList< QStringList > | allActionsForComponent (const QStringList &actionId) |
QList< QStringList > | allMainComponents () |
bool | isEnabled () const |
void | overrideMainComponentData (const KComponentData &componentData) |
void | setEnabled (bool enabled) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static void | activateGlobalShortcutContext (const QString &contextUnique, const QString &contextFriendly, const KComponentData &component=KGlobal::mainComponent()) |
static bool | cleanComponent (const QString &componentUnique) |
static QStringList | findActionNameSystemwide (const QKeySequence &seq) |
static QList< KGlobalShortcutInfo > | getGlobalShortcutsByKey (const QKeySequence &seq) |
static bool | isComponentActive (const QString &componentName) |
static bool | isGlobalShortcutAvailable (const QKeySequence &seq, const QString &component=QString()) |
static bool | promptStealShortcutSystemwide (QWidget *parent, const QList< KGlobalShortcutInfo > &shortcuts, const QKeySequence &seq) |
static bool | promptStealShortcutSystemwide (QWidget *parent, const QStringList &actionIdentifier, const QKeySequence &seq) |
static KGlobalAccel * | self () |
static void | stealShortcutSystemwide (const QKeySequence &seq) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Configurable global shortcut support.
KGlobalAccel allows you to have global accelerators that are independent of the focused window. Unlike regular shortcuts, the application's window does not need focus for them to be activated.
- See also
- KKeyChooser
- KKeyDialog
Definition at line 46 of file kglobalaccel.h.
Member Enumeration Documentation
Index for actionId QStringLists.
Enumerator | |
---|---|
ComponentUnique |
Components Unique Name (ID) |
ActionUnique |
Actions Unique Name(ID) |
ComponentFriendly |
Components Friendly Translated Name. |
ActionFriendly |
Actions Friendly Translated Name. |
Definition at line 55 of file kglobalaccel.h.
Member Function Documentation
|
static |
Set global shortcut context.
A global shortcut context allows an application to have different sets of global shortcuts and to switch between them. This is used by plasma to switch the active global shortcuts when switching between activities.
- Parameters
-
component the name of the component. KComponentData::componentName context the name of the context.
- Since
- 4.2
Definition at line 169 of file kglobalaccel.cpp.
QList< QStringList > KGlobalAccel::allActionsForComponent | ( | const QStringList & | actionId | ) |
QList< QStringList > KGlobalAccel::allMainComponents | ( | ) |
Return the unique and common names of all main components that have global shortcuts.
The action strings of the returned actionId stringlists will be empty.
Definition at line 496 of file kglobalaccel.cpp.
Clean the shortcuts for component componentUnique.
If the component is not active all global shortcut registrations are purged and the component is removed completely.
If the component is active all global shortcut registrations not in use will be purged. If there is no shortcut registration left the component is purged too.
If a purged component or shortcut is activated the next time it will reregister itself. All you probably will lose on wrong usage are the user's set shortcuts.
If you make sure your component is running and all global shortcuts it has are active this function can be used to clean up the registry.
Handle with care!
If the method return true
at least one shortcut was purged so handle all previously acquired information with care.
Definition at line 181 of file kglobalaccel.cpp.
|
static |
|
static |
Returns a list of global shortcuts registered for the shortcut .
If the list contains more that one entry it means the component that registered the shortcuts uses global shortcut contexts. All returned shortcuts belong to the same component.
- Since
- 4.2
Definition at line 520 of file kglobalaccel.cpp.
Check if component is active.
- Parameters
-
componentUnique the components unique identifier
- Returns
true
if active, if not
Definition at line 191 of file kglobalaccel.cpp.
bool KGlobalAccel::isEnabled | ( | ) | const |
|
static |
Check if the shortcut is available for the component
.
The component is only of interest if the current application uses global shortcut contexts. In that case a global shortcut by component
in an inactive global shortcut contexts does not block the seq
for us.
- Since
- 4.2
Definition at line 526 of file kglobalaccel.cpp.
void KGlobalAccel::overrideMainComponentData | ( | const KComponentData & | componentData | ) |
Set the KComponentData for which to manipulate shortcuts.
This is for exceptional situations, when you want to modify the shortcuts of another application as if they were yours. You cannot have your own working global shortcuts in a module/application using this special functionality. All global shortcuts of KActions will essentially be proxies. Be sure to set the default global shortcuts of the proxy KActions to the same as those on the receiving end. An example use case is the KControl Module for the window manager KWin, which has no own facility for users to change its global shortcuts.
- Parameters
-
componentData a KComponentData about the application for which you want to manipulate shortcuts.
Definition at line 223 of file kglobalaccel.cpp.
|
static |
Show a messagebox to inform the user that a global shorcut is already occupied, and ask to take it away from its current action(s).
This is GUI only, so nothing will be actually changed.
- See also
- stealShortcutSystemwide()
- Since
- 4.2
Definition at line 554 of file kglobalaccel.cpp.
|
static |
- See also
- promptStealShortcutSystemwide below
Definition at line 534 of file kglobalaccel.cpp.
|
static |
Returns (and creates if necessary) the singleton instance.
Definition at line 231 of file kglobalaccel.cpp.
void KGlobalAccel::setEnabled | ( | bool | enabled | ) |
|
static |
Take away the given shortcut from the named action it belongs to.
This applies to all actions with global shortcuts in any KDE application.
- See also
- promptStealShortcutSystemwide()
Definition at line 593 of file kglobalaccel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.