KDEUI
#include <kiconeffect.h>
Public Types | |
enum | Effects { NoEffect, ToGray, Colorize, ToGamma, DeSaturate, ToMonochrome, LastEffect } |
Public Member Functions | |
KIconEffect () | |
~KIconEffect () | |
QImage | apply (const QImage &src, int group, int state) const |
QImage | apply (const QImage &src, int effect, float value, const QColor &rgb, bool trans) const |
QImage | apply (const QImage &src, int effect, float value, const QColor &rgb, const QColor &rgb2, bool trans) const |
QPixmap | apply (const QPixmap &src, int group, int state) const |
QPixmap | apply (const QPixmap &src, int effect, float value, const QColor &rgb, bool trans) const |
QPixmap | apply (const QPixmap &src, int effect, float value, const QColor &rgb, const QColor &rgb2, bool trans) const |
QImage | doublePixels (const QImage &src) const |
QString | fingerprint (int group, int state) const |
bool | hasEffect (int group, int state) const |
void | init () |
Static Public Member Functions | |
static void | colorize (QImage &image, const QColor &col, float value) |
static void | deSaturate (QImage &image, float value) |
static void | overlay (QImage &src, QImage &overlay) |
static void | semiTransparent (QImage &image) |
static void | semiTransparent (QPixmap &pixmap) |
static void | toGamma (QImage &image, float value) |
static void | toGray (QImage &image, float value) |
static void | toMonochrome (QImage &image, const QColor &black, const QColor &white, float value) |
Detailed Description
Applies effects to icons.
This class applies effects to icons depending on their state and group. For example, it can be used to make all disabled icons in a toolbar gray.
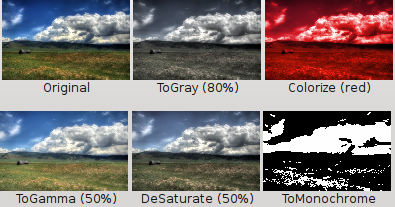
- See also
- KIcon
Definition at line 47 of file kiconeffect.h.
Member Enumeration Documentation
enum KIconEffect::Effects |
This is the enumeration of all possible icon effects.
Note that 'LastEffect' is no valid icon effect but only used internally to check for invalid icon effects.
- NoEffect: Don't apply any icon effect
- ToGray: Tints the icon gray
- Colorize: Tints the icon with an other color
- ToGamma: Change the gamma value of the icon
- DeSaturate: Reduce the saturation of the icon
- ToMonochrome: Produces a monochrome icon
Enumerator | |
---|---|
NoEffect | |
ToGray | |
Colorize | |
ToGamma | |
DeSaturate | |
ToMonochrome | |
LastEffect |
Definition at line 72 of file kiconeffect.h.
Constructor & Destructor Documentation
KIconEffect::KIconEffect | ( | ) |
Create a new KIconEffect.
You will most likely never have to use this to create a new KIconEffect yourself, as you can use the KIconEffect provided by the global KIconLoader (which itself is accessible by KIconLoader::global()) through its iconEffect() function.
Definition at line 64 of file kiconeffect.cpp.
KIconEffect::~KIconEffect | ( | ) |
Definition at line 70 of file kiconeffect.cpp.
Member Function Documentation
Applies an effect to an image.
The effect to apply depends on the group
and state
parameters, and is configured by the user.
- Parameters
-
src The image. group The group for the icon, see KIconLoader::Group state The icon's state, see KIconLoader::States
- Returns
- An image with the effect applied.
Definition at line 196 of file kiconeffect.cpp.
QImage KIconEffect::apply | ( | const QImage & | src, |
int | effect, | ||
float | value, | ||
const QColor & | rgb, | ||
bool | trans | ||
) | const |
Applies an effect to an image.
- Parameters
-
src The image. effect The effect to apply, one of KIconEffect::Effects. value Strength of the effect. 0 <= value
<= 1.rgb Color parameter for effects that need one. trans Add Transparency if trans = true.
- Returns
- An image with the effect applied.
Definition at line 212 of file kiconeffect.cpp.
QImage KIconEffect::apply | ( | const QImage & | src, |
int | effect, | ||
float | value, | ||
const QColor & | rgb, | ||
const QColor & | rgb2, | ||
bool | trans | ||
) | const |
Definition at line 219 of file kiconeffect.cpp.
Applies an effect to a pixmap.
- Parameters
-
src The pixmap. group The group for the icon, see KIconLoader::Group state The icon's state, see KIconLoader::States
- Returns
- A pixmap with the effect applied.
Definition at line 257 of file kiconeffect.cpp.
QPixmap KIconEffect::apply | ( | const QPixmap & | src, |
int | effect, | ||
float | value, | ||
const QColor & | rgb, | ||
bool | trans | ||
) | const |
Applies an effect to a pixmap.
- Parameters
-
src The pixmap. effect The effect to apply, one of KIconEffect::Effects. value Strength of the effect. 0 <= value
<= 1.rgb Color parameter for effects that need one. trans Add Transparency if trans = true.
- Returns
- A pixmap with the effect applied.
Definition at line 273 of file kiconeffect.cpp.
QPixmap KIconEffect::apply | ( | const QPixmap & | src, |
int | effect, | ||
float | value, | ||
const QColor & | rgb, | ||
const QColor & | rgb2, | ||
bool | trans | ||
) | const |
Definition at line 280 of file kiconeffect.cpp.
Colorizes an image with a specific color.
- Parameters
-
image The image col The color with which the image
is tintedvalue Strength of the effect. 0 <= value
<= 1
Definition at line 389 of file kiconeffect.cpp.
|
static |
Desaturates an image.
- Parameters
-
image The image value Strength of the effect. 0 <= value
<= 1
Definition at line 487 of file kiconeffect.cpp.
Returns an image twice as large, consisting of 2x2 pixels.
- Parameters
-
src the image.
- Returns
- the scaled image.
Definition at line 652 of file kiconeffect.cpp.
QString KIconEffect::fingerprint | ( | int | group, |
int | state | ||
) | const |
Returns a fingerprint for the effect by encoding the given group
and state
into a QString.
This is useful for caching.
- Parameters
-
group the group, see KIconLoader::Group state the state, see KIconLoader::States
- Returns
- the fingerprint of the given
group+
state
Definition at line 162 of file kiconeffect.cpp.
bool KIconEffect::hasEffect | ( | int | group, |
int | state | ||
) | const |
Tests whether an effect has been configured for the given icon group.
- Parameters
-
group the group to check, see KIconLoader::Group state the state to check, see KIconLoader::States
- Returns
- true if an effect is configured for the given
group
instate
, otherwise false.
Definition at line 152 of file kiconeffect.cpp.
void KIconEffect::init | ( | ) |
Rereads configuration.
Definition at line 75 of file kiconeffect.cpp.
Overlays an image with an other image.
- Parameters
-
src The image overlay The image to overlay src
with
Definition at line 701 of file kiconeffect.cpp.
|
static |
Renders an image semi-transparent.
- Parameters
-
image The image
Definition at line 527 of file kiconeffect.cpp.
|
static |
Renders a pixmap semi-transparent.
- Parameters
-
pixmap The pixmap
Definition at line 622 of file kiconeffect.cpp.
|
static |
Changes the gamma value of an image.
- Parameters
-
image The image value Strength of the effect. 0 <= value
<= 1
Definition at line 508 of file kiconeffect.cpp.
|
static |
Tints an image gray.
- Parameters
-
image The image value Strength of the effect. 0 <= value
<= 1
Definition at line 359 of file kiconeffect.cpp.
|
static |
Produces a monochrome icon with a given foreground and background color.
- Parameters
-
image The image white The color with which the white parts of image
are paintedblack The color with which the black parts of image
are paintedvalue Strength of the effect. 0 <= value
<= 1
Definition at line 427 of file kiconeffect.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.