KDEUI
#include <klinkitemselectionmodel.h>
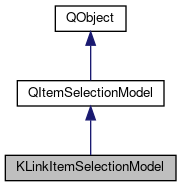
Public Member Functions | |
KLinkItemSelectionModel (QAbstractItemModel *targetModel, QItemSelectionModel *linkedItemSelectionModel, QObject *parent=0) | |
~KLinkItemSelectionModel () | |
void | select (const QModelIndex &index, QItemSelectionModel::SelectionFlags command) |
void | select (const QItemSelection &selection, QItemSelectionModel::SelectionFlags command) |
![]() | |
QItemSelectionModel (QAbstractItemModel *model) | |
QItemSelectionModel (QAbstractItemModel *model, QObject *parent) | |
virtual | ~QItemSelectionModel () |
virtual void | clear () |
void | clearSelection () |
bool | columnIntersectsSelection (int column, const QModelIndex &parent) const |
void | currentChanged (const QModelIndex ¤t, const QModelIndex &previous) |
void | currentColumnChanged (const QModelIndex ¤t, const QModelIndex &previous) |
QModelIndex | currentIndex () const |
void | currentRowChanged (const QModelIndex ¤t, const QModelIndex &previous) |
bool | hasSelection () const |
bool | isColumnSelected (int column, const QModelIndex &parent) const |
bool | isRowSelected (int row, const QModelIndex &parent) const |
bool | isSelected (const QModelIndex &index) const |
const QAbstractItemModel * | model () const |
virtual void | reset () |
bool | rowIntersectsSelection (int row, const QModelIndex &parent) const |
virtual void | select (const QItemSelection &selection, QFlags< QItemSelectionModel::SelectionFlag > command) |
virtual void | select (const QModelIndex &index, QFlags< QItemSelectionModel::SelectionFlag > command) |
QModelIndexList | selectedColumns (int row) const |
QModelIndexList | selectedIndexes () const |
QModelIndexList | selectedRows (int column) const |
const QItemSelection | selection () const |
void | selectionChanged (const QItemSelection &selected, const QItemSelection &deselected) |
void | setCurrentIndex (const QModelIndex &index, QFlags< QItemSelectionModel::SelectionFlag > command) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Attributes | |
KLinkItemSelectionModelPrivate *const | d_ptr |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | SelectionFlags |
![]() | |
void | emitSelectionChanged (const QItemSelection &newSelection, const QItemSelection &oldSelection) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Makes it possible to share a selection in multiple views which do not have the same source model.
Although multiple views can share the same QItemSelectionModel, the views then need to have the same source model.
If there is a proxy model between the model and one of the views, or different proxy models in each, this class makes it possible to share the selection between the views.
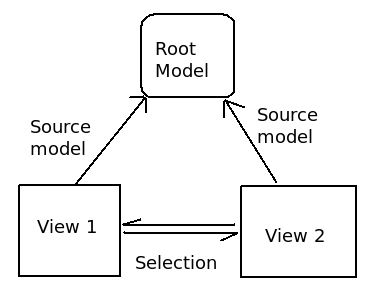
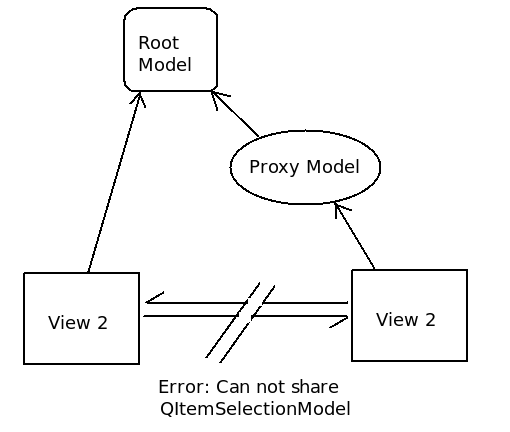
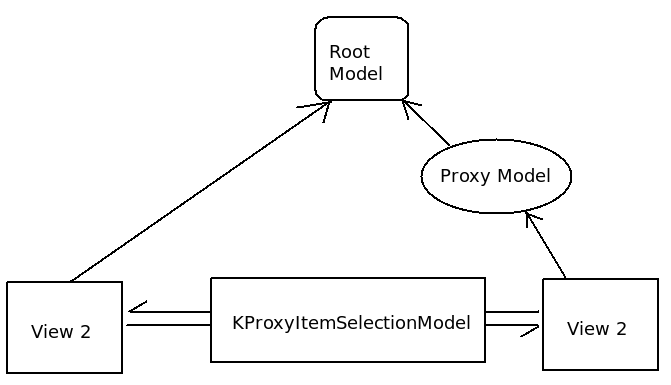
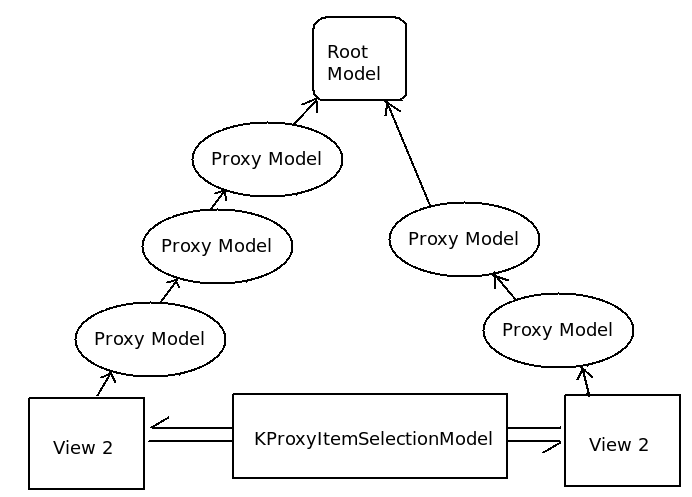
See also kdelibs/kdeui/tests/proxymodeltestapp/proxyitemselectionwidget.cpp.
- Since
- 4.5
Definition at line 95 of file klinkitemselectionmodel.h.
Constructor & Destructor Documentation
KLinkItemSelectionModel::KLinkItemSelectionModel | ( | QAbstractItemModel * | targetModel, |
QItemSelectionModel * | linkedItemSelectionModel, | ||
QObject * | parent = 0 |
||
) |
Constructor.
Definition at line 68 of file klinkitemselectionmodel.cpp.
KLinkItemSelectionModel::~KLinkItemSelectionModel | ( | ) |
Definition at line 77 of file klinkitemselectionmodel.cpp.
Member Function Documentation
void KLinkItemSelectionModel::select | ( | const QModelIndex & | index, |
QItemSelectionModel::SelectionFlags | command | ||
) |
Definition at line 82 of file klinkitemselectionmodel.cpp.
void KLinkItemSelectionModel::select | ( | const QItemSelection & | selection, |
QItemSelectionModel::SelectionFlags | command | ||
) |
Definition at line 134 of file klinkitemselectionmodel.cpp.
Member Data Documentation
|
protected |
Definition at line 108 of file klinkitemselectionmodel.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.