KDEUI
#include <kpixmapcache.h>
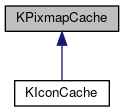
Public Types | |
enum | RemoveStrategy { RemoveOldest, RemoveSeldomUsed, RemoveLeastRecentlyUsed } |
Public Member Functions | |
KPixmapCache (const QString &name) | |
virtual | ~KPixmapCache () |
int | cacheLimit () const |
void | discard () |
virtual bool | find (const QString &key, QPixmap &pix) |
virtual void | insert (const QString &key, const QPixmap &pix) |
bool | isEnabled () const |
bool | isValid () const |
QPixmap | loadFromFile (const QString &filename) |
QPixmap | loadFromSvg (const QString &filename, const QSize &size=QSize()) |
void | removeEntries (int newsize=0) |
RemoveStrategy | removeEntryStrategy () const |
void | setCacheLimit (int kbytes) |
void | setRemoveEntryStrategy (RemoveStrategy strategy) |
void | setTimestamp (unsigned int time) |
void | setUseQPixmapCache (bool use) |
int | size () const |
unsigned int | timestamp () const |
bool | useQPixmapCache () const |
Static Public Member Functions | |
static void | deleteCache (const QString &name) |
Protected Member Functions | |
void | ensureInited () const |
virtual bool | loadCustomData (QDataStream &stream) |
virtual bool | loadCustomIndexHeader (QDataStream &stream) |
bool | recreateCacheFiles () |
void | setValid (bool valid) |
virtual bool | writeCustomData (QDataStream &stream) |
virtual void | writeCustomIndexHeader (QDataStream &stream) |
Detailed Description
General-purpose pixmap cache for KDE.
The pixmap cache can be used to store pixmaps which can later be loaded from the cache very quickly.
Its most common use is storing SVG images which might be expensive to render every time they are used. With the cache you can render each SVG only once and later use the stored version unless the SVG file or requested pixmap size changes.
KPixmapCache's API is similar to that of the QPixmapCache so if you're already using the latter then all you need to do is creating a KPixmapCache object (unlike QPixmapCache, KPixmapCache doesn't have many static methods) and calling insert() and find() method on that object:
The above example illustrates that you can also cache pixmaps created from some data. In case such data is updated, you might need to discard cache contents using discard() method:
As demonstrated, you can use cache's timestamp() method to see when the cache was created. If necessary, you can also change the timestamp using setTimestamp() method.
- Deprecated:
- KPixmapCache is susceptible to various non-trivial locking bugs and inefficiencies, and is supported for backward compatibility only (since it exposes a QDataStream API for subclasses). Users should port to KImageCache for a very close work-alike, or KSharedDataCache if they need more control.
- See also
- KImageCache, KSharedDataCache
Definition at line 85 of file kpixmapcache.h.
Member Enumeration Documentation
Describes which entries will be removed first during cache cleanup.
Enumerator | |
---|---|
RemoveOldest |
oldest entries are removed first. |
RemoveSeldomUsed |
least used entries are removed first. |
RemoveLeastRecentlyUsed |
least recently used entries are removed first. |
Definition at line 216 of file kpixmapcache.h.
Constructor & Destructor Documentation
|
explicit |
Constucts the pixmap cache object.
- Parameters
-
name unique name of the cache
Definition at line 983 of file kpixmapcache.cpp.
|
virtual |
Definition at line 996 of file kpixmapcache.cpp.
Member Function Documentation
int KPixmapCache::cacheLimit | ( | ) | const |
- Returns
- maximum size of the cache (in kilobytes). Default setting is 3 megabytes (1 megabyte = 2^20 bytes).
Definition at line 1122 of file kpixmapcache.cpp.
|
static |
Deletes a pixmap cache.
- Parameters
-
name unique name of the cache to be deleted
Definition at line 1217 of file kpixmapcache.cpp.
void KPixmapCache::discard | ( | ) |
Deletes all entries and reinitializes this cache.
NOTE: If useQPixmapCache is set to true then that cache must also be cleared. There is only one QPixmapCache for the entire process however so other KPixmapCaches and other QPixmapCache users may also be affected, leading to a temporary slowdown until the QPixmapCache is repopulated.
Definition at line 1226 of file kpixmapcache.cpp.
|
protected |
Makes sure that the cache is initialized correctly, including the loading of the cache index and data, and any shared memory attachments (for systems where that is enabled).
- Note
- Although this method is protected you should not use it from any subclasses.
Definition at line 1032 of file kpixmapcache.cpp.
Tries to load pixmap with the specified key from cache.
If the pixmap is found it is stored in pix, otherwise pix is unchanged.
- Returns
- true when pixmap was found and loaded from cache, false otherwise
Reimplemented in KIconCache.
Definition at line 1272 of file kpixmapcache.cpp.
Inserts the pixmap pix into the cache, associated with the key key.
Any existing pixmaps associated with key are overwritten.
Reimplemented in KIconCache.
Definition at line 1366 of file kpixmapcache.cpp.
bool KPixmapCache::isEnabled | ( | ) | const |
Cache will be disabled when e.g.
its data file cannot be created or read.
- Returns
- true when the cache is enabled.
Definition at line 1048 of file kpixmapcache.cpp.
bool KPixmapCache::isValid | ( | ) | const |
- Returns
- true when the cache is ready to be used. Not being valid usually means that some additional initialization has to be done before the cache can be used.
Definition at line 1054 of file kpixmapcache.cpp.
|
protectedvirtual |
Can be used by subclasses to load custom data from the stream.
This function will be called by KPixmapCache immediately following the image data for a single image being read from stream. (This function is called once for every single image).
- See also
- writeCustomData
- loadCustomIndexHeader
- Parameters
-
stream the QDataStream to read data from
- Returns
- true if custom data was successfully loaded, false otherwise. If false is returned then the cached item is assumed to be invalid and will not be available to find() or contains().
Reimplemented in KIconCache.
Definition at line 1361 of file kpixmapcache.cpp.
|
protectedvirtual |
Can be used by subclasses to load custom data from cache's header.
This function will be called by KPixmapCache immediately after the index header has been written out. (This function is called one time only for the entire cache).
- See also
- loadCustomData
- writeCustomIndexHeader
- Parameters
-
stream the QDataStream to read data from
- Returns
- true if custom index header data was successfully read, false otherwise. If false is returned then the cache is assumed to be invalid and further processing does not occur.
Reimplemented in KIconCache.
Definition at line 1039 of file kpixmapcache.cpp.
Loads a pixmap from given file, using the cache.
If the file does not exist on disk, an empty pixmap is returned, even if that file had previously been cached. In addition, if the file's modified-time is more recent than cache's timestamp(), the entire cache is discarded (to be regenerated). This behavior may change in a future KDE Platform release. If the cached data is current the pixmap is returned directly from the cache without any file loading.
- Note
- The mapping between filename and the actual key used internally is implementation-dependent and can change without warning. Use insert() manually if you need control of the key, otherwise consistently use this function.
- Parameters
-
filename The name of the pixmap to load, cache, and return.
- Returns
- The given pixmap, or an empty pixmap if the file was invalid or did not exist.
Definition at line 1447 of file kpixmapcache.cpp.
Same as loadFromFile(), but using an SVG file instead.
You may optionally pass in a size to control the size of the output pixmap.
- Note
- The returned pixmap is only cached for identical filenames and sizes. If you change the size in between calls to this function then the pixmap will have to be regenerated again.
- Parameters
-
filename The filename of the SVG file to load. size size of the pixmap where the SVG is render to. If not given then the SVG file's default size is used.
- Returns
- an empty pixmap if the file does not exist or was invalid, otherwise a pixmap of the desired size.
Definition at line 1473 of file kpixmapcache.cpp.
|
protected |
This function causes the cache files to be recreate by invalidating the cache.
Any shared memory mappings (if enabled) are dropped temporarily as well.
- Note
- The recreated cache will be initially empty, but with the same size limits and entry removal strategy (see removeEntryStrategy()).
If you use this in a subclass be prepared to handle writeCustomData() and writeCustomIndexHeader().
- Returns
- true if the cache was successfully recreated.
Definition at line 1156 of file kpixmapcache.cpp.
void KPixmapCache::removeEntries | ( | int | newsize = 0 | ) |
Removes some of the entries in the cache according to current removeEntryStrategy().
- Parameters
-
newsize wanted size of the cache, in bytes. If 0 is given then current cacheLimit() is used.
- Warning
- This currently works by copying some entries to a new cache and then replacing the old cache with the new one. Thus it might be slow and will temporarily use extra disk space.
Definition at line 1258 of file kpixmapcache.cpp.
KPixmapCache::RemoveStrategy KPixmapCache::removeEntryStrategy | ( | ) | const |
- Returns
- current entry removal strategy. Default is RemoveLeastRecentlyUsed.
Definition at line 1146 of file kpixmapcache.cpp.
void KPixmapCache::setCacheLimit | ( | int | kbytes | ) |
Sets the maximum size of the cache (in kilobytes).
If cache gets bigger than the limit then some entries are removed (according to removeEntryStrategy()).
Setting the cache limit to 0 disables caching (as all entries will get immediately removed).
Note that the cleanup might not be done immediately, so the cache might temporarily (for a few seconds) grow bigger than the limit.
Definition at line 1127 of file kpixmapcache.cpp.
void KPixmapCache::setRemoveEntryStrategy | ( | KPixmapCache::RemoveStrategy | strategy | ) |
Sets the removeEntryStrategy used when removing entries.
Definition at line 1151 of file kpixmapcache.cpp.
void KPixmapCache::setTimestamp | ( | unsigned int | time | ) |
Sets the timestamp of app-specific cache.
It's saved in the cache file and can later be retrieved using the timestamp() method. By default the timestamp is set to the cache creation time.
Definition at line 1072 of file kpixmapcache.cpp.
void KPixmapCache::setUseQPixmapCache | ( | bool | use | ) |
Sets whether QPixmapCache (memory caching) should be used in addition to disk cache.
QPixmapCache is used by default.
- Note
- On most systems KPixmapCache can use shared-memory to share cached pixmaps with other applications attached to the same shared pixmap, which means additional memory caching is unnecessary and actually wasteful of memory.
- Warning
- QPixmapCache is shared among the entire process and therefore can cause strange interactions with other instances of KPixmapCache. This may be fixed in the future and should be not relied upon.
Definition at line 1112 of file kpixmapcache.cpp.
|
protected |
Sets whether this cache is valid or not.
(The cache must be enabled in addition for isValid() to return true.
- See also
- isEnabled(),
- setEnabled()).
Most cache functions do not work if the cache is not valid. KPixmapCache assumes the cache is valid as long as its cache files were able to be created (see recreateCacheFiles()) even if the cache is not enabled.
Can be used by subclasses to indicate that cache needs some additional initialization before it can be used (note that KPixmapCache will not handle actually performing this extra initialization).
Definition at line 1060 of file kpixmapcache.cpp.
int KPixmapCache::size | ( | ) | const |
- Returns
- approximate size of the cache, in kilobytes (1 kilobyte == 1024 bytes)
Definition at line 1103 of file kpixmapcache.cpp.
unsigned int KPixmapCache::timestamp | ( | ) | const |
- Note
- KPixmapCache does not ever change the timestamp, so the application must set the timestamp if it to be used.
- Returns
- Timestamp of the cache, set using the setTimestamp() method.
Definition at line 1066 of file kpixmapcache.cpp.
bool KPixmapCache::useQPixmapCache | ( | ) | const |
Whether QPixmapCache should be used to cache pixmaps in memory in addition to caching them on the disk.
NOTE: The design of QPixmapCache means that the entries stored in the cache are shared throughout the entire process, and not just in this particular KPixmapCache. KPixmapCache makes an effort to ensure that entries from other KPixmapCaches do not inadvertently spill over into this one, but is not entirely successful (see discard())
Definition at line 1117 of file kpixmapcache.cpp.
|
protectedvirtual |
Can be used by subclasses to write custom data into the stream.
This function will be called by KPixmapCache immediately after the image data for a single image has been written to stream. (This function is called once for every single image).
- See also
- loadCustomData
- writeCustomIndexHeader
- Parameters
-
stream the QDataStream to write data to
Reimplemented in KIconCache.
Definition at line 1429 of file kpixmapcache.cpp.
|
protectedvirtual |
Can be used by subclasses to write custom data into cache's header.
This function will be called by KPixmapCache immediately following the index header has being loaded. (This function is called one time only for the entire cache).
- See also
- writeCustomData
- loadCustomIndexHeader
- Parameters
-
stream the QDataStream to write data to
Reimplemented in KIconCache.
Definition at line 1044 of file kpixmapcache.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.