KDEUI
#include <kruler.h>
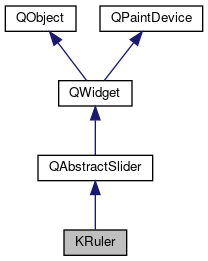
Public Types | |
enum | MetricStyle { Custom =0, Pixel, Inch, Millimetres, Centimetres, Metres } |
Public Slots | |
void | slotEndOffset (int) |
void | slotNewOffset (int) |
void | slotNewValue (int) |
Public Member Functions | |
KRuler (QWidget *parent=0) | |
KRuler (Qt::Orientation orient, QWidget *parent=0, Qt::WindowFlags f=0) | |
KRuler (Qt::Orientation orient, int widgetWidth, QWidget *parent=0, Qt::WindowFlags f=0) | |
~KRuler () | |
int | bigMarkDistance () const |
QString | endLabel () const |
int | endOffset () const |
int | length () const |
bool | lengthFixed () const |
int | littleMarkDistance () const |
int | maxValue () const |
int | mediumMarkDistance () const |
int | minValue () const |
int | offset () const |
double | pixelPerMark () const |
void | setBigMarkDistance (int) |
void | setEndLabel (const QString &) |
void | setFrameStyle (int) |
void | setLength (int) |
void | setLengthFixed (bool fix) |
void | setLittleMarkDistance (int) |
void | setMaxValue (int) |
void | setMediumMarkDistance (int) |
void | setMinValue (int) |
void | setOffset (int offset) |
void | setPixelPerMark (double rate) |
void | setRulerMetricStyle (KRuler::MetricStyle) |
void | setShowBigMarks (bool) |
void | setShowEndLabel (bool) |
void | setShowEndMarks (bool) |
void | setShowLittleMarks (bool) |
void | setShowMediumMarks (bool) |
void | setShowPointer (bool) |
void | setShowTinyMarks (bool) |
void | setTinyMarkDistance (int) |
bool | showBigMarks () const |
bool | showEndLabel () const |
bool | showEndMarks () const |
bool | showLittleMarks () const |
bool | showMediumMarks () const |
bool | showPointer () const |
bool | showTinyMarks () const |
void | slideDown (int count=1) |
void | slideUp (int count=1) |
int | tinyMarkDistance () const |
![]() | |
QAbstractSlider (QWidget *parent) | |
~QAbstractSlider () | |
void | actionTriggered (int action) |
void | addLine () |
void | addPage () |
bool | hasTracking () const |
bool | invertedAppearance () const |
bool | invertedControls () const |
bool | isSliderDown () const |
int | lineStep () const |
int | maximum () const |
int | maxValue () const |
int | minimum () const |
int | minValue () const |
Qt::Orientation | orientation () const |
int | pageStep () const |
void | rangeChanged (int min, int max) |
void | setInvertedAppearance (bool) |
void | setInvertedControls (bool) |
void | setLineStep (int v) |
void | setMaximum (int) |
void | setMaxValue (int v) |
void | setMinimum (int) |
void | setMinValue (int v) |
void | setOrientation (Qt::Orientation) |
void | setPageStep (int) |
void | setRange (int min, int max) |
void | setSingleStep (int) |
void | setSliderDown (bool) |
void | setSliderPosition (int) |
void | setSteps (int single, int page) |
void | setTracking (bool enable) |
void | setValue (int) |
int | singleStep () const |
void | sliderMoved (int value) |
int | sliderPosition () const |
void | sliderPressed () |
void | sliderReleased () |
void | subtractLine () |
void | subtractPage () |
void | triggerAction (SliderAction action) |
int | value () const |
void | valueChanged (int value) |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
virtual int | heightForWidth (int w) const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
virtual QSize | minimumSizeHint () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
virtual QSize | sizeHint () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Protected Member Functions | |
virtual void | paintEvent (QPaintEvent *) |
![]() | |
virtual void | changeEvent (QEvent *ev) |
virtual bool | event (QEvent *e) |
virtual void | keyPressEvent (QKeyEvent *ev) |
SliderAction | repeatAction () const |
void | setRepeatAction (SliderAction action, int thresholdTime, int repeatTime) |
virtual void | sliderChange (SliderChange change) |
virtual void | timerEvent (QTimerEvent *e) |
virtual void | wheelEvent (QWheelEvent *e) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | languageChange () |
virtual void | leaveEvent (QEvent *event) |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
![]() | |
QPaintDevice () | |
Additional Inherited Members | |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
![]() | |
typedef | RenderFlags |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
A ruler widget.
The vertical ruler looks similar to this:
There are tiny marks, little marks, medium marks, and big marks along the ruler.
To receive mouse clicks or mouse moves, the class has to be overloaded.
For performance reasons, the public methods don't call QWidget::repaint(). (Slots do, see documentation below.) All the changed settings will be painted once after leaving to the main event loop. For performance painting the slot methods should be used, they do a fast QWidget::repaint() call after changing the values. For setting multiple values like minValue(), maxValue(), offset() etc. using the public methods is recommended so the widget will be painted only once when entering the main event loop.

A ruler widget.
Member Enumeration Documentation
enum KRuler::MetricStyle |
Constructor & Destructor Documentation
|
explicit |
The style (or look) of the ruler.
Constructs a horizontal ruler.
Definition at line 113 of file kruler.cpp.
|
explicit |
Constructs a ruler with orientation orient
.
parent
and f
are passed to QFrame. The default look is a raised widget but may be changed with the inherited QFrame methods.
- Parameters
-
orient Orientation of the ruler. parent Will be handed over to QFrame. f Will be handed over to QFrame.
Definition at line 125 of file kruler.cpp.
KRuler::KRuler | ( | Qt::Orientation | orient, |
int | widgetWidth, | ||
QWidget * | parent = 0 , |
||
Qt::WindowFlags | f = 0 |
||
) |
Constructs a ruler with orientation orient
and initial width widgetWidth
.
The width sets the fixed width of the widget. This is useful if you want to draw the ruler bigger or smaller than the default size. Note: The size of the marks doesn't change. parent
and f
are passed to QFrame.
- Parameters
-
orient Orientation of the ruler. widgetWidth Fixed width of the widget. parent Will be handed over to QFrame. f Will be handed over to QFrame.
Definition at line 142 of file kruler.cpp.
KRuler::~KRuler | ( | ) |
Destructor.
Definition at line 185 of file kruler.cpp.
Member Function Documentation
int KRuler::bigMarkDistance | ( | ) | const |
Returns the distance between big marks.
QString KRuler::endLabel | ( | ) | const |
int KRuler::endOffset | ( | ) | const |
int KRuler::length | ( | ) | const |
bool KRuler::lengthFixed | ( | ) | const |
int KRuler::littleMarkDistance | ( | ) | const |
Returns the distance between little marks.
int KRuler::maxValue | ( | ) | const |
Returns the maximal value of the ruler pointer.
Definition at line 214 of file kruler.cpp.
int KRuler::mediumMarkDistance | ( | ) | const |
int KRuler::minValue | ( | ) | const |
Returns the minimal value of the ruler pointer.
Definition at line 200 of file kruler.cpp.
int KRuler::offset | ( | ) | const |
Returns the current ruler offset.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 629 of file kruler.cpp.
double KRuler::pixelPerMark | ( | ) | const |
Returns the number of pixels between two base marks.
void KRuler::setBigMarkDistance | ( | int | dist | ) |
Sets distance between big marks.
For English (inches) or metric styles it is twice the medium mark distance.
Definition at line 258 of file kruler.cpp.
void KRuler::setEndLabel | ( | const QString & | label | ) |
Sets the label this is drawn at the beginning of the visible part of the ruler to label
.
Definition at line 388 of file kruler.cpp.
void KRuler::setFrameStyle | ( | int | ) |
Definition at line 363 of file kruler.cpp.
void KRuler::setLength | ( | int | length | ) |
Sets the length of the ruler, i.e.
the difference between the begin mark and the end mark of the ruler.
when the length is not locked, it gets adjusted with the length of the widget.
Definition at line 496 of file kruler.cpp.
void KRuler::setLengthFixed | ( | bool | fix | ) |
Locks the length of the ruler, i.e.
the difference between the two end marks doesn't change when the widget is resized.
- Parameters
-
fix fixes the length, if true
Definition at line 522 of file kruler.cpp.
void KRuler::setLittleMarkDistance | ( | int | dist | ) |
Sets the distance between little marks.
The default value is 1 in the metric system and 2 in the English (inches) system.
Definition at line 232 of file kruler.cpp.
void KRuler::setMaxValue | ( | int | value | ) |
Sets the maximum value of the ruler pointer (default is 100).
This method calls update() so that the widget is painted after leaving to the main event loop.
Definition at line 206 of file kruler.cpp.
void KRuler::setMediumMarkDistance | ( | int | dist | ) |
Sets the distance between medium marks.
For English (inches) styles it defaults to twice the little mark distance. For metric styles it defaults to five times the little mark distance.
Definition at line 245 of file kruler.cpp.
void KRuler::setMinValue | ( | int | value | ) |
Sets the minimal value of the ruler pointer (default is 0).
This method calls update() so that the widget is painted after leaving to the main event loop.
Definition at line 192 of file kruler.cpp.
void KRuler::setOffset | ( | int | offset | ) |
Sets the ruler slide offset.
This is like slideup() or slidedown() with an absolute offset from the start of the ruler.
- Parameters
-
offset Number of pixel to move the ruler up or left from the beginning
Definition at line 534 of file kruler.cpp.
void KRuler::setPixelPerMark | ( | double | rate | ) |
Sets the number of pixels between two base marks.
Calling this method stretches or shrinks your ruler.
For pixel display ( MetricStyle) the value is 10.0 marks per pixel ;-) For English (inches) it is 9.0, and for centimetres ~2.835 -> 3.0 . If you want to magnify your part of display, you have to adjust the mark distance here
. Notice: The double type is only supported to give the possibility of having some double values. It should be used with care. Using values below 10.0 shows visible jumps of markpositions (e.g. 2.345). Using whole numbers is highly recommended. To use int
values use setPixelPerMark((int)your_int_value); default: 1 mark per 10 pixels
Definition at line 485 of file kruler.cpp.
void KRuler::setRulerMetricStyle | ( | KRuler::MetricStyle | style | ) |
Sets up the necessary tasks for the provided styles.
A convenience method.
Definition at line 408 of file kruler.cpp.
void KRuler::setShowBigMarks | ( | bool | show | ) |
Shows/hides big marks.
Definition at line 316 of file kruler.cpp.
void KRuler::setShowEndLabel | ( | bool | show | ) |
Show/hide number values of the little marks.
Default is false
. Show/hide number values of the medium marks.
Default is false
. Show/hide number values of the big marks.
Default is false
. Show/hide number values of the end marks.
Default is false
.
Definition at line 371 of file kruler.cpp.
void KRuler::setShowEndMarks | ( | bool | show | ) |
Shows/hides end marks.
Definition at line 332 of file kruler.cpp.
void KRuler::setShowLittleMarks | ( | bool | show | ) |
Shows/hides little marks.
Definition at line 286 of file kruler.cpp.
void KRuler::setShowMediumMarks | ( | bool | show | ) |
Shows/hides medium marks.
Definition at line 301 of file kruler.cpp.
void KRuler::setShowPointer | ( | bool | show | ) |
Shows/hides the pointer.
Definition at line 347 of file kruler.cpp.
void KRuler::setShowTinyMarks | ( | bool | show | ) |
Shows/hides tiny marks.
Definition at line 271 of file kruler.cpp.
void KRuler::setTinyMarkDistance | ( | int | dist | ) |
Sets the distance between tiny marks.
This is mostly used in the English system (inches) with distance of 1.
Definition at line 219 of file kruler.cpp.
bool KRuler::showBigMarks | ( | ) | const |
bool KRuler::showEndLabel | ( | ) | const |
bool KRuler::showEndMarks | ( | ) | const |
Definition at line 341 of file kruler.cpp.
bool KRuler::showLittleMarks | ( | ) | const |
bool KRuler::showMediumMarks | ( | ) | const |
bool KRuler::showPointer | ( | ) | const |
bool KRuler::showTinyMarks | ( | ) | const |
void KRuler::slideDown | ( | int | count = 1 | ) |
Sets the number of pixels by which the ruler may slide down or right.
The number of pixels moved is realive to the previous position. The Method makes sense for updating a ruler, which is working with a scrollbar.
This doesn't affect the position of the ruler pointer. Only the visible part of the ruler is moved.
- Parameters
-
count Number of pixel moving up or left relative to the previous position
Definition at line 566 of file kruler.cpp.
void KRuler::slideUp | ( | int | count = 1 | ) |
Sets the number of pixels by which the ruler may slide up or left.
The number of pixels moved is realive to the previous position. The Method makes sense for updating a ruler, which is working with a scrollbar.
This doesn't affect the position of the ruler pointer. Only the visible part of the ruler is moved.
- Parameters
-
count Number of pixel moving up or left relative to the previous position
Definition at line 557 of file kruler.cpp.
|
slot |
Definition at line 613 of file kruler.cpp.
|
slot |
Sets the ruler marks to a new position.
The pointer is NOT updated. QWidget::repaint() is called afterwards.
Definition at line 602 of file kruler.cpp.
|
slot |
Sets the pointer to a new position.
The offset is NOT updated. QWidget::repaint() is called afterwards.
Definition at line 576 of file kruler.cpp.
int KRuler::tinyMarkDistance | ( | ) | const |
Returns the distance between tiny marks.
Property Documentation
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.