KDEUI
#include <kstringvalidator.h>
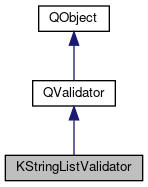
Public Member Functions | |
KStringListValidator (const QStringList &list=QStringList(), bool rejecting=true, bool fixupEnabled=false, QObject *parent=0) | |
~KStringListValidator () | |
virtual void | fixup (QString &input) const |
bool | isFixupEnabled () const |
bool | isRejecting () const |
void | setFixupEnabled (bool fixupEnabled) |
void | setRejecting (bool rejecting) |
void | setStringList (const QStringList &list) |
QStringList | stringList () const |
virtual State | validate (QString &input, int &pos) const |
![]() | |
QValidator (QObject *parent) | |
QValidator (QObject *parent, const char *name) | |
~QValidator () | |
QLocale | locale () const |
void | setLocale (const QLocale &locale) |
virtual State | validate (QString &input, int &pos) const =0 |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Properties | |
bool | fixupEnabled |
bool | rejecting |
QStringList | stringList |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
A QValidator to (dis)allow certain strings.
This validator allows you to accept only certain or to accept all but certain strings.
When used in rejecting mode, accepts only strings not in the stringlist. This mode is the default and comes in handy when asking the user for a name of some listed entity. Set the list of already used names to prevent the user from entering duplicate names.
When used in non-rejecting mode, accepts only strings that appear in the stringlist. Use with care! From a user's point of view this mode is hard to grasp.
This validator can also fix strings. In rejecting mode, a number will be appended to the string until it is Acceptable. E.g. if "foo" and "foo 1" are in the stringlist, then fixup will change "foo" to "foo 2", provided "foo 2" isn't in the list of forbidden strings.
In accepting mode, when the input starts with an Acceptable substring, truncates to the longest Acceptable string. When the input is the start of an Acceptable string, completes to the shortest Acceptable string.
NOTE: fixup isn't yet implemented.
Definition at line 60 of file kstringvalidator.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new string validator.
- Parameters
-
list The list of strings to (dis)allow. rejecting Selects the validator's mode (rejecting: true; accepting: false) fixupEnabled Selects whether to fix strings or not. parent Passed to lower level constructor.
Definition at line 35 of file kstringvalidator.cpp.
KStringListValidator::~KStringListValidator | ( | ) |
Destroys the string validator.
Definition at line 45 of file kstringvalidator.cpp.
Member Function Documentation
|
virtual |
Reimplemented from.
- See also
- QValidator.
Reimplemented from QValidator.
Definition at line 71 of file kstringvalidator.cpp.
bool KStringListValidator::isFixupEnabled | ( | ) | const |
Returns whether the fixup flag is set.
Definition at line 99 of file kstringvalidator.cpp.
bool KStringListValidator::isRejecting | ( | ) | const |
Returns whether the string validator is in rejecting mode.
Definition at line 89 of file kstringvalidator.cpp.
void KStringListValidator::setFixupEnabled | ( | bool | fixupEnabled | ) |
Sets the fixup flag.
If enabled, wrong input is corrected automatically.
Definition at line 94 of file kstringvalidator.cpp.
void KStringListValidator::setRejecting | ( | bool | rejecting | ) |
Sets whether the string validator is in rejecting mode or not.
If in rejecting mode, the strings from
- See also
- stringList are not allowed to appear in the validation string.
Definition at line 84 of file kstringvalidator.cpp.
void KStringListValidator::setStringList | ( | const QStringList & | list | ) |
Sets the.
- Parameters
-
list of string which is used as black or white list, depending on the rejecting mode (
- See also
- isRejecting()).
Definition at line 104 of file kstringvalidator.cpp.
QStringList KStringListValidator::stringList | ( | ) | const |
Returns the string list of the validator.
|
virtual |
Property Documentation
|
readwrite |
Definition at line 65 of file kstringvalidator.h.
|
readwrite |
Definition at line 64 of file kstringvalidator.h.
|
readwrite |
Definition at line 63 of file kstringvalidator.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.