KDEUI
#include <kurllabel.h>
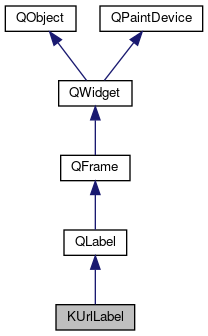
Public Slots | |
void | setAlternatePixmap (const QPixmap &pixmap) |
void | setFloatEnabled (bool do_float=true) |
virtual void | setFont (const QFont &font) |
void | setGlowEnabled (bool glow=true) |
void | setHighlightedColor (const QColor &highcolor) |
void | setHighlightedColor (const QString &highcolor) |
void | setSelectedColor (const QColor &color) |
void | setSelectedColor (const QString &color) |
void | setTipText (const QString &tip) |
void | setUnderline (bool on=true) |
void | setUrl (const QString &url) |
void | setUseCursor (bool on, QCursor *cursor=0L) |
void | setUseTips (bool on=true) |
Signals | |
void | enteredUrl (const QString &url) |
void | enteredUrl () |
void | leftClickedUrl (const QString &url) |
void | leftClickedUrl () |
void | leftUrl (const QString &url) |
void | leftUrl () |
void | middleClickedUrl (const QString &url) |
void | middleClickedUrl () |
void | rightClickedUrl (const QString &url) |
void | rightClickedUrl () |
Public Member Functions | |
KUrlLabel (QWidget *parent=0L) | |
KUrlLabel (const QString &url, const QString &text=QString(), QWidget *parent=0L) | |
virtual | ~KUrlLabel () |
const QPixmap * | alternatePixmap () const |
bool | isFloatEnabled () const |
bool | isGlowEnabled () const |
QString | tipText () const |
QString | url () const |
bool | useCursor () const |
bool | useTips () const |
![]() | |
QLabel (QWidget *parent, QFlags< Qt::WindowType > f) | |
QLabel (const QString &text, QWidget *parent, QFlags< Qt::WindowType > f) | |
QLabel (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
QLabel (const QString &text, QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
QLabel (QWidget *buddy, const QString &text, QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QLabel () | |
Qt::Alignment | alignment () const |
QWidget * | buddy () const |
void | clear () |
bool | hasScaledContents () const |
bool | hasSelectedText () const |
virtual int | heightForWidth (int w) const |
int | indent () const |
void | linkActivated (const QString &link) |
void | linkHovered (const QString &link) |
int | margin () const |
virtual QSize | minimumSizeHint () const |
QMovie * | movie () const |
bool | openExternalLinks () const |
const QPicture * | picture () const |
const QPixmap * | pixmap () const |
QString | selectedText () const |
int | selectionStart () const |
void | setAlignment (int alignment) |
void | setAlignment (QFlags< Qt::AlignmentFlag >) |
void | setBuddy (QWidget *buddy) |
void | setIndent (int) |
void | setMargin (int) |
void | setMovie (QMovie *movie) |
void | setNum (double num) |
void | setNum (int num) |
void | setOpenExternalLinks (bool open) |
void | setPicture (const QPicture &picture) |
void | setPixmap (const QPixmap &) |
void | setScaledContents (bool) |
void | setSelection (int start, int length) |
void | setText (const QString &) |
void | setTextFormat (Qt::TextFormat) |
void | setTextInteractionFlags (QFlags< Qt::TextInteractionFlag > flags) |
void | setWordWrap (bool on) |
virtual QSize | sizeHint () const |
QString | text () const |
Qt::TextFormat | textFormat () const |
Qt::TextInteractionFlags | textInteractionFlags () const |
bool | wordWrap () const |
![]() | |
QFrame (QWidget *parent, QFlags< Qt::WindowType > f) | |
QFrame (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QFrame () | |
QRect | frameRect () const |
Shadow | frameShadow () const |
Shape | frameShape () const |
int | frameStyle () const |
int | frameWidth () const |
int | lineWidth () const |
int | midLineWidth () const |
void | setFrameRect (const QRect &) |
void | setFrameShadow (Shadow) |
void | setFrameShape (Shape) |
void | setFrameStyle (int style) |
void | setLineWidth (int) |
void | setMidLineWidth (int) |
![]() | |
QWidget (QWidget *parent, QFlags< Qt::WindowType > f) | |
QWidget (QWidget *parent, const char *name, QFlags< Qt::WindowType > f) | |
~QWidget () | |
bool | acceptDrops () const |
QString | accessibleDescription () const |
QString | accessibleName () const |
QList< QAction * > | actions () const |
void | activateWindow () |
void | addAction (QAction *action) |
void | addActions (QList< QAction * > actions) |
void | adjustSize () |
bool | autoFillBackground () const |
Qt::BackgroundMode | backgroundMode () const |
QPoint | backgroundOffset () const |
BackgroundOrigin | backgroundOrigin () const |
QPalette::ColorRole | backgroundRole () const |
QSize | baseSize () const |
QString | caption () const |
QWidget * | childAt (int x, int y, bool includeThis) const |
QWidget * | childAt (const QPoint &p, bool includeThis) const |
QWidget * | childAt (int x, int y) const |
QWidget * | childAt (const QPoint &p) const |
QRect | childrenRect () const |
QRegion | childrenRegion () const |
void | clearFocus () |
void | clearMask () |
bool | close (bool alsoDelete) |
bool | close () |
QColorGroup | colorGroup () const |
void | constPolish () const |
QMargins | contentsMargins () const |
QRect | contentsRect () const |
Qt::ContextMenuPolicy | contextMenuPolicy () const |
QCursor | cursor () const |
void | customContextMenuRequested (const QPoint &pos) |
void | drawText (const QPoint &p, const QString &s) |
void | drawText (int x, int y, const QString &s) |
WId | effectiveWinId () const |
void | ensurePolished () const |
void | erase () |
void | erase (const QRect &rect) |
void | erase (const QRegion &rgn) |
void | erase (int x, int y, int w, int h) |
Qt::FocusPolicy | focusPolicy () const |
QWidget * | focusProxy () const |
QWidget * | focusWidget () const |
const QFont & | font () const |
QFontInfo | fontInfo () const |
QFontMetrics | fontMetrics () const |
QPalette::ColorRole | foregroundRole () const |
QRect | frameGeometry () const |
QSize | frameSize () const |
const QRect & | geometry () const |
void | getContentsMargins (int *left, int *top, int *right, int *bottom) const |
virtual HDC | getDC () const |
void | grabGesture (Qt::GestureType gesture, QFlags< Qt::GestureFlag > flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const |
QGraphicsProxyWidget * | graphicsProxyWidget () const |
bool | hasEditFocus () const |
bool | hasFocus () const |
bool | hasMouse () const |
bool | hasMouseTracking () const |
int | height () const |
void | hide () |
const QPixmap * | icon () const |
void | iconify () |
QString | iconText () const |
QInputContext * | inputContext () |
Qt::InputMethodHints | inputMethodHints () const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, QList< QAction * > actions) |
bool | isActiveWindow () const |
bool | isAncestorOf (const QWidget *child) const |
bool | isDesktop () const |
bool | isDialog () const |
bool | isEnabled () const |
bool | isEnabledTo (QWidget *ancestor) const |
bool | isEnabledToTLW () const |
bool | isFullScreen () const |
bool | isHidden () const |
bool | isInputMethodEnabled () const |
bool | isMaximized () const |
bool | isMinimized () const |
bool | isModal () const |
bool | isPopup () const |
bool | isShown () const |
bool | isTopLevel () const |
bool | isUpdatesEnabled () const |
bool | isVisible () const |
bool | isVisibleTo (QWidget *ancestor) const |
bool | isVisibleToTLW () const |
bool | isWindow () const |
bool | isWindowModified () const |
QLayout * | layout () const |
Qt::LayoutDirection | layoutDirection () const |
QLocale | locale () const |
void | lower () |
Qt::HANDLE | macCGHandle () const |
Qt::HANDLE | macQDHandle () const |
QPoint | mapFrom (QWidget *parent, const QPoint &pos) const |
QPoint | mapFromGlobal (const QPoint &pos) const |
QPoint | mapFromParent (const QPoint &pos) const |
QPoint | mapTo (QWidget *parent, const QPoint &pos) const |
QPoint | mapToGlobal (const QPoint &pos) const |
QPoint | mapToParent (const QPoint &pos) const |
QRegion | mask () const |
int | maximumHeight () const |
QSize | maximumSize () const |
int | maximumWidth () const |
int | minimumHeight () const |
QSize | minimumSize () const |
int | minimumWidth () const |
void | move (int x, int y) |
void | move (const QPoint &) |
QWidget * | nativeParentWidget () const |
QWidget * | nextInFocusChain () const |
QRect | normalGeometry () const |
void | overrideWindowFlags (QFlags< Qt::WindowType > flags) |
bool | ownCursor () const |
bool | ownFont () const |
bool | ownPalette () const |
virtual QPaintEngine * | paintEngine () const |
const QPalette & | palette () const |
QWidget * | parentWidget (bool sameWindow) const |
QWidget * | parentWidget () const |
QPlatformWindow * | platformWindow () const |
QPlatformWindowFormat | platformWindowFormat () const |
void | polish () |
QPoint | pos () const |
QWidget * | previousInFocusChain () const |
void | raise () |
void | recreate (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
QRect | rect () const |
virtual void | releaseDC (HDC hdc) const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, QFlags< QWidget::RenderFlag > renderFlags) |
void | repaint (int x, int y, int w, int h, bool b) |
void | repaint (const QRegion &rgn, bool b) |
void | repaint () |
void | repaint (int x, int y, int w, int h) |
void | repaint (const QRegion &rgn) |
void | repaint (bool b) |
void | repaint (const QRect &rect) |
void | repaint (const QRect &r, bool b) |
void | reparent (QWidget *parent, QFlags< Qt::WindowType > f, const QPoint &p, bool showIt) |
void | reparent (QWidget *parent, const QPoint &p, bool showIt) |
void | resize (int w, int h) |
void | resize (const QSize &) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setActiveWindow () |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundColor (const QColor &color) |
void | setBackgroundMode (Qt::BackgroundMode widgetBackground, Qt::BackgroundMode paletteBackground) |
void | setBackgroundOrigin (BackgroundOrigin background) |
void | setBackgroundPixmap (const QPixmap &pixmap) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setCaption (const QString &c) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContentsMargins (const QMargins &margins) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setEraseColor (const QColor &color) |
void | setErasePixmap (const QPixmap &pixmap) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus (Qt::FocusReason reason) |
void | setFocus () |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setFont (const QFont &f, bool b) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (int x, int y, int w, int h) |
void | setGeometry (const QRect &) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setIcon (const QPixmap &i) |
void | setIconText (const QString &it) |
void | setInputContext (QInputContext *context) |
void | setInputMethodEnabled (bool enabled) |
void | setInputMethodHints (QFlags< Qt::InputMethodHint > hints) |
void | setKeyCompression (bool b) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setPalette (const QPalette &p, bool b) |
void | setPaletteBackgroundColor (const QColor &color) |
void | setPaletteBackgroundPixmap (const QPixmap &pixmap) |
void | setPaletteForegroundColor (const QColor &color) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, QFlags< Qt::WindowType > f) |
void | setPlatformWindow (QPlatformWindow *window) |
void | setPlatformWindowFormat (const QPlatformWindowFormat &format) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setShown (bool shown) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy::Policy hor, QSizePolicy::Policy ver, bool hfw) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setSizePolicy (QSizePolicy) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
QStyle * | setStyle (const QString &style) |
void | setStyleSheet (const QString &styleSheet) |
void | setToolTip (const QString &) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlags (QFlags< Qt::WindowType > type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (QFlags< Qt::WindowState > windowState) |
void | setWindowSurface (QWindowSurface *surface) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const |
QSize | sizeIncrement () const |
QSizePolicy | sizePolicy () const |
void | stackUnder (QWidget *w) |
QString | statusTip () const |
QStyle * | style () const |
QString | styleSheet () const |
bool | testAttribute (Qt::WidgetAttribute attribute) const |
QString | toolTip () const |
QWidget * | topLevelWidget () const |
bool | underMouse () const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetFont () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | unsetPalette () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | update () |
void | updateGeometry () |
bool | updatesEnabled () const |
QRect | visibleRect () const |
QRegion | visibleRegion () const |
QString | whatsThis () const |
int | width () const |
QWidget * | window () const |
QString | windowFilePath () const |
Qt::WindowFlags | windowFlags () const |
QIcon | windowIcon () const |
QString | windowIconText () const |
Qt::WindowModality | windowModality () const |
qreal | windowOpacity () const |
QString | windowRole () const |
Qt::WindowStates | windowState () const |
QWindowSurface * | windowSurface () const |
QString | windowTitle () const |
Qt::WindowType | windowType () const |
WId | winId () const |
int | x () const |
const QX11Info & | x11Info () const |
Qt::HANDLE | x11PictureHandle () const |
int | y () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
virtual | ~QPaintDevice () |
int | colorCount () const |
int | depth () const |
int | height () const |
int | heightMM () const |
int | logicalDpiX () const |
int | logicalDpiY () const |
int | numColors () const |
virtual QPaintEngine * | paintEngine () const =0 |
bool | paintingActive () const |
int | physicalDpiX () const |
int | physicalDpiY () const |
int | width () const |
int | widthMM () const |
int | x11Cells () const |
Qt::HANDLE | x11Colormap () const |
bool | x11DefaultColormap () const |
bool | x11DefaultVisual () const |
int | x11Depth () const |
Display * | x11Display () const |
int | x11Screen () const |
void * | x11Visual () const |
Protected Member Functions | |
virtual void | enterEvent (QEvent *) |
virtual bool | event (QEvent *) |
virtual void | leaveEvent (QEvent *) |
virtual void | mouseReleaseEvent (QMouseEvent *) |
![]() | |
virtual void | changeEvent (QEvent *ev) |
virtual void | contextMenuEvent (QContextMenuEvent *ev) |
virtual void | focusInEvent (QFocusEvent *ev) |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *ev) |
virtual void | keyPressEvent (QKeyEvent *ev) |
virtual void | mouseMoveEvent (QMouseEvent *ev) |
virtual void | mousePressEvent (QMouseEvent *ev) |
virtual void | paintEvent (QPaintEvent *) |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
bool | focusNextChild () |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | languageChange () |
virtual bool | macEvent (EventHandlerCallRef caller, EventRef event) |
virtual int | metric (PaintDeviceMetric m) const |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | qwsEvent (QWSEvent *event) |
void | resetInputContext () |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus () |
virtual void | wheelEvent (QWheelEvent *event) |
virtual bool | winEvent (MSG *message, long *result) |
virtual bool | x11Event (XEvent *event) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QPaintDevice () | |
Additional Inherited Members | |
![]() | |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
QWidgetMapper * | wmapper () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
int | x11AppCells (int screen) |
Qt::HANDLE | x11AppColormap (int screen) |
bool | x11AppDefaultColormap (int screen) |
bool | x11AppDefaultVisual (int screen) |
int | x11AppDepth (int screen) |
Display * | x11AppDisplay () |
int | x11AppDpiX (int screen) |
int | x11AppDpiY (int screen) |
Qt::HANDLE | x11AppRootWindow (int screen) |
int | x11AppScreen () |
void * | x11AppVisual (int screen) |
void | x11SetAppDpiX (int dpi, int screen) |
void | x11SetAppDpiY (int dpi, int screen) |
![]() | |
typedef | RenderFlags |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
A drop-in replacement for QLabel that displays hyperlinks.
KUrlLabel is a drop-in replacement for QLabel that handles text in a fashion similar to how an HTML widget handles hyperlinks. The text can be underlined (or not) and set to different colors. It can also "glow" (cycle colors) when the mouse passes over it.
KUrlLabel also provides signals for several events, including the mouse leaving and entering the text area and all forms of mouse clicking.
By default KUrlLabel accepts focus. When focused, standard focus rectangle is displayed as in HTML widget. Pressing Enter key accepts the focused label.
A typical usage would be something like so:
In this example, the text "My homepage" would be displayed as blue, underlined text. When the mouse passed over it, it would "glow" red. When the user clicks on the text, the signal leftClickedUrl() would be emitted with "http://www.home.com/~me" as its argument.
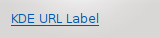
Definition at line 69 of file kurllabel.h.
Constructor & Destructor Documentation
|
explicit |
Default constructor.
Use setUrl() and setText() or QListView::setPixmap() to set the resp. properties.
Definition at line 97 of file kurllabel.cpp.
|
explicit |
Convenience constructor.
- Parameters
-
url is the URL emitted when the label is clicked. text is the displayed string. If it's equal to QString() the url
will be used instead.parent Passed to lower level constructor
parent
and name
are passed to QLabel, which in turn passes them further down
Definition at line 88 of file kurllabel.cpp.
|
virtual |
Destructs the label.
Definition at line 106 of file kurllabel.cpp.
Member Function Documentation
const QPixmap* KUrlLabel::alternatePixmap | ( | ) | const |
- Returns
- the alternate pixmap (may be 0L if none was set).
|
signal |
Emitted when the mouse has passed over the label.
- Parameters
-
url The URL for this label.
|
signal |
Emitted when the mouse has passed over the label.
|
protectedvirtual |
Overridden for internal reasons; the API remains unaffected.
Reimplemented from QWidget.
Definition at line 272 of file kurllabel.cpp.
Catch parent palette changes.
Use parentWidget() unless you are a toplevel widget, then try qAapp
Reimplemented from QLabel.
Definition at line 312 of file kurllabel.cpp.
bool KUrlLabel::isFloatEnabled | ( | ) | const |
This feature is very similar to the "glow" feature in that the color of the label switches to the selected color when the cursor passes over it.
In addition, underlining is turned on for as long as the mouse is overhead. Note that if "glow" and underlining are both already turned on, this feature will have no visible effect.
Definition at line 257 of file kurllabel.cpp.
bool KUrlLabel::isGlowEnabled | ( | ) | const |
When this is on, the text will switch to the selected color whenever the mouse passes over it.
Definition at line 252 of file kurllabel.cpp.
|
protectedvirtual |
Overridden for internal reasons; the API remains unaffected.
Reimplemented from QWidget.
Definition at line 296 of file kurllabel.cpp.
|
signal |
Emitted when the user clicked the left mouse button on this label.
- Parameters
-
url The URL for this label.
|
signal |
Emitted when the user clicked the left mouse button on this label.
|
signal |
Emitted when the mouse is no longer over the label.
- Parameters
-
url The URL for this label.
|
signal |
Emitted when the mouse is no longer over the label.
|
signal |
Emitted when the user clicked the middle mouse button on this label.
- Parameters
-
url The URL for this label.
|
signal |
Emitted when the user clicked the left mouse button on this label.
|
protectedvirtual |
Overridden for internal reasons; the API remains unaffected.
Reimplemented from QLabel.
Definition at line 111 of file kurllabel.cpp.
|
signal |
Emitted when the user clicked the right mouse button on this label.
- Parameters
-
url The URL for this label.
|
signal |
Emitted when the user clicked the right mouse button on this label.
|
slot |
Sets the "alt" pixmap.
This pixmap will be displayed when the cursor passes over the label. The effect is similar to the trick done with 'onMouseOver' in javascript.
- See also
- alternatePixmap()
Definition at line 262 of file kurllabel.cpp.
|
slot |
Turns on or off the "float" feature.
This feature is very similar to the "glow" feature in that the color of the label switches to the selected color when the cursor passes over it. In addition, underlining is turned on for as long as the mouse is overhead. Note that if "glow" and underlining are both already turned on, this feature will have no visible effect. By default, it is false
.
Definition at line 247 of file kurllabel.cpp.
|
virtualslot |
Overridden for internal reasons; the API remains unaffected.
Definition at line 139 of file kurllabel.cpp.
|
slot |
Turns on or off the "glow" feature.
When this is on, the text will switch to the selected color whenever the mouse passes over it. By default, it is true
.
Definition at line 242 of file kurllabel.cpp.
|
slot |
Sets the highlight color.
This is the default foreground color (non-selected). By default, it is blue
.
Definition at line 216 of file kurllabel.cpp.
|
slot |
This is an overloaded version for convenience.
- See also
- setHighlightedColor()
Definition at line 224 of file kurllabel.cpp.
|
slot |
Sets the selected color.
This is the color the text will change to when either a mouse passes over it and "glow" mode is on or when it is selected (clicked). By default, it is red
.
Definition at line 229 of file kurllabel.cpp.
|
slot |
This is an overloaded version for convenience.
- See also
- setSelectedColor()
Definition at line 237 of file kurllabel.cpp.
|
slot |
Specifies what text to display when tooltips are turned on.
If this is not used, the tip will default to the URL.
- See also
- setUseTips()
Definition at line 199 of file kurllabel.cpp.
|
slot |
Turns on or off the underlining.
When this is on, the text will be underlined. By default, it is true
.
Definition at line 147 of file kurllabel.cpp.
|
slot |
Turns the custom cursor feature on or off.
When this is on, the cursor will change to a custom cursor (default is a "pointing hand") whenever the cursor passes over the label. By default, it is on.
- Parameters
-
on whether a custom cursor should be displayed. cursor is the custom cursor. 0L
indicates the default "hand cursor".
Definition at line 169 of file kurllabel.cpp.
|
slot |
Turns on or off the tool tip feature.
When this is on, the URL will be displayed as a tooltip whenever the mouse passes passes over it. By default, it is false
.
Definition at line 189 of file kurllabel.cpp.
QString KUrlLabel::tipText | ( | ) | const |
Returns the current tooltip text.
QString KUrlLabel::url | ( | ) | const |
Returns the URL.
bool KUrlLabel::useCursor | ( | ) | const |
- Returns
- true if the cursor will change while over the URL.
- See also
- setUseCursor ()
bool KUrlLabel::useTips | ( | ) | const |
- Returns
- true if a tooltip will be displayed.
- See also
- setTipText()
Property Documentation
|
readwrite |
Definition at line 74 of file kurllabel.h.
|
readwrite |
Definition at line 76 of file kurllabel.h.
|
readwrite |
Definition at line 75 of file kurllabel.h.
|
readwrite |
Definition at line 73 of file kurllabel.h.
|
readwrite |
Definition at line 72 of file kurllabel.h.
|
readwrite |
Definition at line 78 of file kurllabel.h.
|
readwrite |
Definition at line 77 of file kurllabel.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.