KDEUI
#include <kwidgetitemdelegate.h>
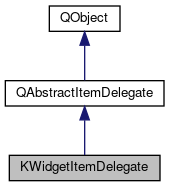
Public Member Functions | |
KWidgetItemDelegate (QAbstractItemView *itemView, QObject *parent=0) | |
virtual | ~KWidgetItemDelegate () |
QPersistentModelIndex | focusedIndex () const |
QAbstractItemView * | itemView () const |
![]() | |
QAbstractItemDelegate (QObject *parent) | |
virtual | ~QAbstractItemDelegate () |
void | closeEditor (QWidget *editor, QAbstractItemDelegate::EndEditHint hint) |
void | commitData (QWidget *editor) |
virtual QWidget * | createEditor (QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const |
virtual bool | editorEvent (QEvent *event, QAbstractItemModel *model, const QStyleOptionViewItem &option, const QModelIndex &index) |
bool | helpEvent (QHelpEvent *event, QAbstractItemView *view, const QStyleOptionViewItem &option, const QModelIndex &index) |
virtual void | paint (QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const =0 |
virtual void | setEditorData (QWidget *editor, const QModelIndex &index) const |
virtual void | setModelData (QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const |
virtual QSize | sizeHint (const QStyleOptionViewItem &option, const QModelIndex &index) const =0 |
void | sizeHintChanged (const QModelIndex &index) |
virtual void | updateEditorGeometry (QWidget *editor, const QStyleOptionViewItem &option, const QModelIndex &index) const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
QList< QEvent::Type > | blockedEventTypes (QWidget *widget) const |
virtual QList< QWidget * > | createItemWidgets () const =0 |
void | paintWidgets (QPainter *painter, const QStyleOptionViewItem &option, const QPersistentModelIndex &index) const |
void | setBlockedEventTypes (QWidget *widget, QList< QEvent::Type > types) const |
virtual void | updateItemWidgets (const QList< QWidget * > widgets, const QStyleOptionViewItem &option, const QPersistentModelIndex &index) const =0 |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QString | elidedText (const QFontMetrics &fontMetrics, int width, Qt::TextElideMode mode, const QString &text) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This class allows to create item delegates embedding simple widgets to interact with items.
For instance you can add push buttons, line edits, etc. to your delegate and use them to modify the state of your model.
- Since
- 4.1
Definition at line 49 of file kwidgetitemdelegate.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new ItemDelegate to be used with a given itemview.
Private class that helps to provide binary compatibility between releases.
- Parameters
-
itemView the item view the new delegate will monitor parent the parent of this delegate
Definition at line 182 of file kwidgetitemdelegate.cpp.
|
virtual |
Destroys an ItemDelegate.
Definition at line 204 of file kwidgetitemdelegate.cpp.
Member Function Documentation
|
protected |
Retrieves the list of blocked event types for the given widget.
- Parameters
-
widget the specified widget.
- Returns
- the list of blocked event types, can be empty if no events are blocked.
Definition at line 308 of file kwidgetitemdelegate.cpp.
Creates the list of widgets needed for an item.
- Note
- No initialization of the widgets is supposed to happen here. The widgets will be initialized based on needs for a given item.
- If you want to connect some widget signals to any slot, you should do it here.
- If you want to know the index for which you are creating widgets, it is available as a QModelIndex Q_PROPERTY called "goya:creatingWidgetForIndex". Ensure to add Q_DECLARE_METATYPE(QModelIndex) before your method definition to tell QVariant about QModelIndex.
- Returns
- the list of newly created widgets which will be used to interact with an item.
- See also
- updateItemWidgets()
QPersistentModelIndex KWidgetItemDelegate::focusedIndex | ( | ) | const |
Retrieves the currently focused index.
An invalid index if none is focused.
- Returns
- the current focused index, or QPersistentModelIndex() if none is focused.
Definition at line 214 of file kwidgetitemdelegate.cpp.
QAbstractItemView * KWidgetItemDelegate::itemView | ( | ) | const |
Retrieves the item view this delegate is monitoring.
- Returns
- the item view this delegate is monitoring
Definition at line 209 of file kwidgetitemdelegate.cpp.
|
protected |
Paint the widgets of the item.
This method is meant to be used in the paint() method of your item delegate implementation.
- Parameters
-
painter the painter the widgets will be painted on. option the current set of style options for the view. index the model index of the item currently painted.
- Warning
- since 4.2 this method is not longer needed to be called. All widgets will kept updated without the need of calling paintWidgets() in your paint() event. For the widgets of a certain index to be updated your model has to emit dataChanged() on the indexes that want to be updated.
Definition at line 226 of file kwidgetitemdelegate.cpp.
|
protected |
Sets the list of event types
that a widget
will block.
Blocked events are not passed to the view. This way you can prevent an item from being selected when a button is clicked for instance.
- Parameters
-
widget the widget which must block events types the list of event types the widget must block
Definition at line 303 of file kwidgetitemdelegate.cpp.
|
protectedpure virtual |
Updates a list of widgets for its use inside of the delegate (painting or event handling).
- Note
- All the positioning and sizing should be done in item coordinates.
- Warning
- Do not make widget connections in here, since this method will be called very regularly.
- Parameters
-
widgets the widgets to update option the current set of style options for the view. index the model index of the item currently manipulated.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.