KDEUI
#include <kwindowsystem.h>
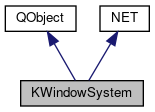
Signals | |
void | activeWindowChanged (WId id) |
void | compositingChanged (bool enabled) |
void | currentDesktopChanged (int desktop) |
void | desktopNamesChanged () |
void | numberOfDesktopsChanged (int num) |
void | showingDesktopChanged (bool showing) |
void | stackingOrderChanged () |
void | strutChanged () |
void | windowAdded (WId id) |
void | windowChanged (WId id, const unsigned long *properties) |
void | windowChanged (WId id, unsigned int properties) |
void | windowChanged (WId id) |
void | windowRemoved (WId id) |
void | workAreaChanged () |
Static Public Member Functions | |
static void | activateWindow (WId win, long time=0) |
static WId | activeWindow () |
static bool | allowedActionsSupported () |
static void | allowExternalProcessWindowActivation (int pid=-1) |
static void | clearState (WId win, unsigned long state) |
static bool | compositingActive () |
static QPoint | constrainViewportRelativePosition (const QPoint &pos) |
static int | currentDesktop () |
static void | demandAttention (WId win, bool set=true) |
static QString | desktopName (int desktop) |
static QPoint | desktopToViewport (int desktop, bool absolute) |
static void | doNotManage (const QString &title) |
static void | forceActiveWindow (WId win, long time=0) |
static WId | groupLeader (WId window) |
static bool | hasWId (WId id) |
static bool | icccmCompliantMappingState () |
static QPixmap | icon (WId win, int width=-1, int height=-1, bool scale=false) |
static QPixmap | icon (WId win, int width, int height, bool scale, int flags) |
static void | lowerWindow (WId win) |
static bool | mapViewport () |
static void | minimizeWindow (WId win, bool animation=true) |
static int | numberOfDesktops () |
static void | raiseWindow (WId win) |
static QString | readNameProperty (WId window, unsigned long atom) |
static KWindowSystem * | self () |
static void | setBlockingCompositing (WId window, bool active) |
static void | setCurrentDesktop (int desktop) |
static void | setDesktopName (int desktop, const QString &name) |
static void | setExtendedStrut (WId win, int left_width, int left_start, int left_end, int right_width, int right_start, int right_end, int top_width, int top_start, int top_end, int bottom_width, int bottom_start, int bottom_end) |
static void | setIcons (WId win, const QPixmap &icon, const QPixmap &miniIcon) |
static void | setMainWindow (QWidget *subwindow, WId mainwindow) |
static void | setOnAllDesktops (WId win, bool b) |
static void | setOnDesktop (WId win, int desktop) |
static void | setState (WId win, unsigned long state) |
static void | setStrut (WId win, int left, int right, int top, int bottom) |
static void | setType (WId win, NET::WindowType windowType) |
static void | setUserTime (WId win, long time) |
static bool | showingDesktop () |
static QList< WId > | stackingOrder () |
static WId | transientFor (WId window) |
static void | unminimizeWindow (WId win, bool animation=true) |
static int | viewportToDesktop (const QPoint &pos) |
static int | viewportWindowToDesktop (const QRect &r) |
static KWindowInfo | windowInfo (WId win, unsigned long properties, unsigned long properties2=0) |
static const QList< WId > & | windows () |
static QRect | workArea (int desktop=-1) |
static QRect | workArea (const QList< WId > &excludes, int desktop=-1) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static int | timestampCompare (unsigned long time1, unsigned long time2) |
static int | timestampDiff (unsigned long time1, unsigned long time2) |
static bool | typeMatchesMask (WindowType type, unsigned long mask) |
Protected Member Functions | |
virtual void | connectNotify (const char *signal) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Convenience access to certain properties and features of the window manager.
The class KWindowSystem provides information about the state of the window manager and allows asking the window manager to change them using a more high-level interface than the NETWinInfo/NETRootInfo lowlevel classes.
Because of limitiations of the way Qt is implemented on Mac OSX, the WId's returned by methods in this class are not compatible with those expected by other Qt methods. So while it should be fine to pass WId's retrieved by for example calling the winId method on a QWidget to methods in this class the reverse is not true. You should never pass a WId obtained from this class to a Qt method accepting a WId parameter.
Class for interaction with the window manager.
Definition at line 55 of file kwindowsystem.h.
Member Enumeration Documentation
Masks specifying from which sources to read an icon.
They are tried from the best until an icon is found.
- NETWM from property from the window manager specification
- WMHints from WMHints property
- ClassHint load icon after getting name from the classhint
- XApp load the standard X icon (last fallback)
Enumerator | |
---|---|
NETWM |
read from property from the window manager specification |
WMHints |
read from WMHints property |
ClassHint |
load icon after getting name from the classhint |
XApp |
load the standard X icon (last fallback) |
Definition at line 268 of file kwindowsystem.h.
Member Function Documentation
|
static |
Requests that window win
is activated.
There are two ways how to activate a window, by calling activateWindow() and forceActiveWindow(). Generally, applications shouldn't make attempts to explicitly activate their windows, and instead let the user to activate them. In the special cases where this may be needed, applications should use activateWindow(). Window manager may consider whether this request wouldn't result in focus stealing, which would be obtrusive, and may refuse the request.
The usage of forceActiveWindow() is meant only for pagers and similar tools, which represent direct user actions related to window manipulation. Except for rare cases, this request will be always honored, and normal applications are forbidden to use it.
In case of problems, consult the KWin README in the kdebase package (kdebase/kwin/README), or ask on the kwin@ mailing list. kde. org
- Parameters
-
win the id of the window to make active time X server timestamp of the user activity that caused this request
Definition at line 355 of file kwindowsystem_mac.cpp.
|
static |
Returns the currently active window, or 0 if no window is active.
- Returns
- the window id of the active window, or 0 if no window is active
Definition at line 348 of file kwindowsystem_mac.cpp.
|
signal |
Hint that <Window> is active (= has focus) now.
- Parameters
-
id the id of the window that is active
|
static |
Returns true if the WM announces which actions it allows for windows.
Definition at line 597 of file kwindowsystem_mac.cpp.
|
static |
Allows a window from another process to raise and activate itself.
Depending on the window manager, the grant may only be temporary, or for a single activation, and it may require the current process to be the "foreground" one" (ie. the process with the input focus).
You should call this function before executing actions that may trigger the showing of a window or dialog in another process, e.g. a dbus signal or function call, or any other inter-process notification mechanism.
This is mostly used on Windows, where windows are not allowed to be raised and activated if their process is not the foreground one, but it may also apply to other window managers.
- Parameters
-
pid if specified, the grant only applies to windows belonging to the specific process. By default, a value of -1 means all processes.
Definition at line 622 of file kwindowsystem_mac.cpp.
|
static |
Clears the state of window win
from state
.
Possible values are or'ed combinations of NET::Modal, NET::Sticky, NET::MaxVert, NET::MaxHoriz, NET::Shaded, NET::SkipTaskbar, NET::SkipPager, NET::Hidden, NET::FullScreen, NET::KeepAbove, NET::KeepBelow, NET::StaysOnTop
- Parameters
-
win the id of the window state the flags that will be cleared
Definition at line 512 of file kwindowsystem_mac.cpp.
|
static |
Returns true if a compositing manager is running (i.e.
ARGB windows are supported, effects will be provided, etc.).
Definition at line 379 of file kwindowsystem_mac.cpp.
|
signal |
Compositing was enabled or disabled.
Note that this signal may be emitted before any compositing plugins have been initialized in the window manager.
If you need to check if a specific compositing plugin such as the blur effect is enabled, you should track that separately rather than test for it in a slot connected to this signal.
- Since
- 4.7.1
|
protectedvirtual |
Reimplemented from QObject.
Definition at line 616 of file kwindowsystem_mac.cpp.
- Since
- 4.0.1 Checks the relative difference used to move a window will still be inside valid desktop area.
Definition at line 1116 of file kwindowsystem_x11.cpp.
|
static |
Returns the current virtual desktop.
- Returns
- the current virtual desktop
Definition at line 384 of file kwindowsystem_mac.cpp.
|
signal |
Switched to another virtual desktop.
- Parameters
-
desktop the number of the new desktop
|
static |
When application finishes some operation and wants to notify the user about it, it can call demandAttention().
Instead of activating the window, which could be obtrusive, the window will be marked specially as demanding user's attention. See also explanation in description of activateWindow().
Note that it's usually better to use KNotifyClient.
Definition at line 373 of file kwindowsystem_mac.cpp.
|
static |
Returns the name of the specified desktop.
- Parameters
-
desktop the number of the desktop
- Returns
- the name of the desktop
Definition at line 561 of file kwindowsystem_mac.cpp.
|
signal |
Desktops have been renamed.
Returns topleft corner of the viewport area for the given mapped virtual desktop.
Definition at line 1089 of file kwindowsystem_x11.cpp.
|
static |
Informs kwin via dbus to not manage a window with the specified title
.
Useful for swallowing legacy applications, for example java applets.
- Parameters
-
title the title of the window
Definition at line 609 of file kwindowsystem_mac.cpp.
|
static |
Sets window win
to be the active window.
Note that this should be called only in special cases, applications shouldn't force themselves or other windows to be the active window. Generally, this call should used only by pagers and similar tools. See the explanation in description of activateWindow().
- Parameters
-
win the id of the window to make active time X server timestamp of the user activity that caused this request
Definition at line 366 of file kwindowsystem_mac.cpp.
|
static |
Returns the leader window for the group the given window is in, if any.
- Parameters
-
window the id of the window
Definition at line 622 of file kwindowsystem_x11.cpp.
|
static |
Test to see if id
still managed at present.
- Parameters
-
id the window id to test
- Returns
- true if the window id is still managed
Definition at line 324 of file kwindowsystem_mac.cpp.
|
static |
Returns true if the WM uses IconicState also for windows on inactive virtual desktops.
Definition at line 542 of file kwindowsystem_mac.cpp.
Returns an icon for window win
.
If width
and height
are specified, the best icon for the requested size is returned.
If scale
is true, the icon is smooth-scaled to have exactly the requested size.
- Parameters
-
win the id of the window width the desired width, or -1 height the desired height, or -1 scale if true the icon will be scaled to the desired size. Otherwise the icon will not be modified.
- Returns
- the icon of the window
Definition at line 418 of file kwindowsystem_mac.cpp.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.Overloaded variant that allows specifying from which sources the icon should be read.
You should usually prefer the simpler variant which tries all possibilities to get an icon.
- Parameters
-
win the id of the window width the desired width, or -1 height the desired height, or -1 scale if true the icon will be scaled to the desired size. Otherwise the icon will not be modified. flags OR-ed flags from the IconSource enum
Definition at line 461 of file kwindowsystem_mac.cpp.
|
static |
Lowers the given window.
This call is only for pagers and similar tools that represent direct user actions. Applications should not use it, they should keep using QWidget::lower() or XLowerWindow() if necessary.
Definition at line 536 of file kwindowsystem_mac.cpp.
|
static |
Returns true if viewports are mapped to virtual desktops.
Definition at line 1042 of file kwindowsystem_x11.cpp.
|
static |
Iconifies a window.
Compatible to XIconifyWindow but has an additional parameter animation
.
- Parameters
-
win the id of the window animation true to show an animation
- See also
- deIconifyWindow()
Definition at line 518 of file kwindowsystem_mac.cpp.
|
static |
Returns the number of virtual desktops.
- Returns
- the number of virtual desktops
Definition at line 389 of file kwindowsystem_mac.cpp.
|
signal |
The number of desktops changed.
- Parameters
-
num the new number of desktops
|
static |
Raises the given window.
This call is only for pagers and similar tools that represent direct user actions. Applications should not use it, they should keep using QWidget::raise() or XRaiseWindow() if necessary.
Definition at line 530 of file kwindowsystem_mac.cpp.
|
static |
Function that reads and returns the contents of the given text property (WM_NAME, WM_ICON_NAME,...).
Definition at line 602 of file kwindowsystem_mac.cpp.
|
static |
Access to the singleton instance.
Useful mainly for connecting to signals.
Definition at line 308 of file kwindowsystem_mac.cpp.
|
static |
Sets whether the client wishes to block compositing (for better performance)
- Since
- 4.7
Definition at line 627 of file kwindowsystem_mac.cpp.
|
static |
Convenience function to set the current desktop to desktop
.
See NETRootInfo.
- Parameters
-
desktop the number of the new desktop
Definition at line 394 of file kwindowsystem_mac.cpp.
|
static |
Sets the name of the specified desktop.
- Parameters
-
desktop the number of the desktop name the new name for the desktop
Definition at line 566 of file kwindowsystem_mac.cpp.
|
static |
Sets the strut of window win
to to
left
width ranging from left_start
to left_end
on the left edge, and simiarly for the other edges.
For not reserving a strut, pass 0 as the width. E.g. to reserve 10x10 square in the topleft corner, use e.g. setExtendedStrut( w, 10, 0, 10, 0, 0, 0, 0, 0, 0, 0, 0, 0 ).
- Parameters
-
win the id of the window left_width width of the strut at the left edge left_start starting y coordinate of the strut at the left edge left_end ending y coordinate of the strut at the left edge right_width width of the strut at the right edge right_start starting y coordinate of the strut at the right edge right_end ending y coordinate of the strut at the right edge top_width width of the strut at the top edge top_start starting x coordinate of the strut at the top edge top_end ending x coordinate of the strut at the top edge bottom_width width of the strut at the bottom edge bottom_start starting x coordinate of the strut at the bottom edge bottom_end ending x coordinate of the strut at the bottom edge
Definition at line 583 of file kwindowsystem_mac.cpp.
Sets an icon
and a miniIcon
on window win
.
- Parameters
-
win the id of the window icon the new icon miniIcon the new mini icon
Definition at line 467 of file kwindowsystem_mac.cpp.
|
static |
Sets the parent window of subwindow
to be mainwindow
.
This overrides the parent set the usual way as the QWidget parent, but only for the window manager - e.g. stacking order and window grouping will be affected, but features like automatic deletion of children when the parent is deleted are unaffected and normally use the QWidget parent.
This function should be used before a dialog is shown for a window that belongs to another application.
Definition at line 412 of file kwindowsystem_mac.cpp.
|
static |
Sets window win
to be present on all virtual desktops if is
true.
Otherwise the window lives only on one single desktop.
- Parameters
-
win the id of the window b true to show the window on all desktops, false otherwise
Definition at line 400 of file kwindowsystem_mac.cpp.
|
static |
Moves window win
to desktop desktop
.
- Parameters
-
win the id of the window desktop the number of the new desktop
Definition at line 406 of file kwindowsystem_mac.cpp.
|
static |
Sets the state of window win
to state
.
Possible values are or'ed combinations of NET::Modal, NET::Sticky, NET::MaxVert, NET::MaxHoriz, NET::Shaded, NET::SkipTaskbar, NET::SkipPager, NET::Hidden, NET::FullScreen, NET::KeepAbove, NET::KeepBelow, NET::StaysOnTop
- Parameters
-
win the id of the window state the new flags that will be set
Definition at line 506 of file kwindowsystem_mac.cpp.
|
static |
Convenience function for setExtendedStrut() that automatically makes struts as wide/high as the screen width/height.
Sets the strut of window win
to left
, right
, top
, bottom
.
- Parameters
-
win the id of the window left the left strut right the right strut top the top strut bottom the bottom strut
Definition at line 591 of file kwindowsystem_mac.cpp.
|
static |
Sets the type of window win
to windowType
.
- Parameters
-
win the id of the window windowType the type of the window (see NET::WindowType)
Definition at line 473 of file kwindowsystem_mac.cpp.
|
static |
Sets user timestamp time
on window win
.
The timestamp is expressed as XServer time. If a window is shown with user timestamp older than the time of the last user action, it won't be activated after being shown. The most common case is the special value 0 which means not to activate the window after being shown.
Definition at line 577 of file kwindowsystem_mac.cpp.
|
static |
Returns the state of showing the desktop.
Definition at line 572 of file kwindowsystem_mac.cpp.
|
signal |
The state of showing the desktop has changed.
|
static |
Returns the list of all toplevel windows currently managed by the window manager in the current stacking order (from lower to higher).
May be useful for pagers.
- Returns
- the list of all toplevel windows in stacking order
Definition at line 340 of file kwindowsystem_mac.cpp.
|
signal |
Emitted when the stacking order of the window changed.
The new order can be obtained with stackingOrder().
|
signal |
Something changed with the struts, may or may not have changed the work area.
Usually just using the workAreaChanged() signal is sufficient.
|
static |
Returns the WM_TRANSIENT_FOR property for the given window, i.e.
the mainwindow for this window.
- Parameters
-
window the id of the window
Definition at line 603 of file kwindowsystem_x11.cpp.
|
static |
DeIconifies a window.
Compatible to XMapWindow but has an additional parameter animation
.
- Parameters
-
win the id of the window animation true to show an animation
- See also
- iconifyWindow()
Definition at line 524 of file kwindowsystem_mac.cpp.
|
static |
Returns mapped virtual desktop for the given position in the viewport.
Definition at line 1059 of file kwindowsystem_x11.cpp.
|
static |
Returns mapped virtual desktop for the given window geometry.
Definition at line 1072 of file kwindowsystem_x11.cpp.
|
signal |
A window has been added.
- Parameters
-
id the id of the window
|
signal |
The window changed.
The properties parameter contains the NET properties that were modified (see netwm_def.h). First element are NET::Property values, second element are NET::Property2 values (i.e. the format is the same like for the NETWinInfo class constructor).
- Parameters
-
id the id of the window properties the properties that were modified
|
signal |
- Deprecated:
- The window changed.
The unsigned int parameter contains the NET properties that were modified (see netwm_def.h).
- Parameters
-
id the id of the window properties the properties that were modified
|
signal |
The window changed somehow.
- Parameters
-
id the id of the window
|
static |
Returns information about window win
.
It is recommended to check whether the returned info is valid by calling the valid() method.
- Parameters
-
win the id of the window properties all properties that should be retrieved (see NET::Property enum for details). Unlisted properties cause related information to be invalid in the returned data, but make this function faster when not all data is needed. properties2 additional properties (see NET::Property2 enum)
- Returns
- the window information
Definition at line 330 of file kwindowsystem_mac.cpp.
|
signal |
A window has been removed.
- Parameters
-
id the id of the window that has been removed
|
static |
Returns the list of all toplevel windows currently managed by the window manager in the order of creation.
Please do not rely on indexes of this list: Whenever you enter Qt's event loop in your application, it may happen that entries are removed or added. Your module should perhaps work on a copy of this list and verify a window with hasWId() before any operations.
Iteration over this list can be done easily with
- Returns
- the list of all toplevel windows
Definition at line 318 of file kwindowsystem_mac.cpp.
|
static |
Returns the workarea for the specified desktop, or the current work area if no desktop has been specified.
- Parameters
-
desktop the number of the desktop to check, -1 for the current desktop
- Returns
- the size and position of the desktop
Definition at line 547 of file kwindowsystem_mac.cpp.
Returns the workarea for the specified desktop, or the current work area if no desktop has been specified.
Excludes struts of clients in the exclude List.
- Parameters
-
excludes the list of clients whose struts will be excluded desktop the number of the desktop to check, -1 for the current desktop
- Returns
- the size and position of the desktop
Definition at line 554 of file kwindowsystem_mac.cpp.
|
signal |
The workarea has changed.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.