KDEUI
#include <highlighter.h>
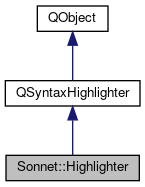
Public Slots | |
void | slotAutoDetection () |
void | slotRehighlight () |
Signals | |
void | activeChanged (const QString &description) |
QT_MOC_COMPAT void | newSuggestions (const QString &originalWord, const QStringList &suggestions) |
Public Member Functions | |
Highlighter (QTextEdit *textEdit, const QString &configFile=QString(), const QColor &col=QColor()) | |
~Highlighter () | |
void | addWordToDictionary (const QString &word) |
bool | automatic () const |
bool | checkerEnabledByDefault () const |
QString | currentLanguage () const |
void | ignoreWord (const QString &word) |
bool | isActive () const |
bool | isWordMisspelled (const QString &word) |
void | setActive (bool active) |
void | setAutomatic (bool automatic) |
void | setCurrentLanguage (const QString &lang) |
void | setMisspelledColor (const QColor &color) |
bool | spellCheckerFound () const |
QStringList | suggestionsForWord (const QString &word, int max=10) |
![]() | |
QSyntaxHighlighter (QObject *parent) | |
QSyntaxHighlighter (QTextDocument *parent) | |
QSyntaxHighlighter (QTextEdit *parent) | |
virtual | ~QSyntaxHighlighter () |
QTextDocument * | document () const |
void | rehighlight () |
void | rehighlightBlock (const QTextBlock &block) |
void | setDocument (QTextDocument *doc) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static QStringList | personalWords () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
bool | eventFilter (QObject *o, QEvent *e) |
virtual void | highlightBlock (const QString &text) |
bool | intraWordEditing () const |
void | setIntraWordEditing (bool editing) |
virtual void | setMisspelled (int start, int count) |
virtual void | unsetMisspelled (int start, int count) |
![]() | |
QTextBlock | currentBlock () const |
int | currentBlockState () const |
QTextBlockUserData * | currentBlockUserData () const |
QTextCharFormat | format (int position) const |
virtual void | highlightBlock (const QString &text)=0 |
int | previousBlockState () const |
void | setCurrentBlockState (int newState) |
void | setCurrentBlockUserData (QTextBlockUserData *data) |
void | setFormat (int start, int count, const QFont &font) |
void | setFormat (int start, int count, const QTextCharFormat &format) |
void | setFormat (int start, int count, const QColor &color) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | customEvent (QEvent *event) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
The Sonnet Highlighter.
Definition at line 34 of file highlighter.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 76 of file highlighter.cpp.
Sonnet::Highlighter::~Highlighter | ( | ) |
Definition at line 134 of file highlighter.cpp.
Member Function Documentation
|
signal |
Emitted when as-you-type spell checking is enabled or disabled.
- Parameters
-
description is a i18n description of the new state, with an optional reason
void Sonnet::Highlighter::addWordToDictionary | ( | const QString & | word | ) |
Adds the given word permanently to the dictionary.
It will never be marked as misspelled again, even after restarting the application.
- Parameters
-
word the word which will be added to the dictionary
- Since
- 4.1
Definition at line 413 of file highlighter.cpp.
bool Sonnet::Highlighter::automatic | ( | ) | const |
Definition at line 196 of file highlighter.cpp.
bool Sonnet::Highlighter::checkerEnabledByDefault | ( | ) | const |
Return true if checker is enabled by default.
- Since
- 4.5
Definition at line 443 of file highlighter.cpp.
QString Sonnet::Highlighter::currentLanguage | ( | ) | const |
Definition at line 295 of file highlighter.cpp.
Reimplemented from QObject.
Definition at line 338 of file highlighter.cpp.
|
protectedvirtual |
Definition at line 273 of file highlighter.cpp.
void Sonnet::Highlighter::ignoreWord | ( | const QString & | word | ) |
Ignores the given word.
This word will not be marked misspelled for this session. It will again be marked as misspelled when creating new highlighters.
- Parameters
-
word the word which will be ignored
- Since
- 4.1
Definition at line 418 of file highlighter.cpp.
|
protected |
Definition at line 201 of file highlighter.cpp.
bool Sonnet::Highlighter::isActive | ( | ) | const |
Returns the state of spell checking.
- Returns
- true if spell checking is active
- See also
- setActive()
Definition at line 268 of file highlighter.cpp.
Checks if a given word is marked as misspelled by the highlighter.
- Parameters
-
word the word to be checked
- Returns
- true if the given word is misspelled.
- Since
- 4.1
Definition at line 433 of file highlighter.cpp.
|
signal |
- Parameters
-
originalWord missspelled word suggestions list of word which can replace missspelled word
- Deprecated:
- use isWordMisspelled() and suggestionsForWord() instead.
|
static |
Definition at line 180 of file highlighter.cpp.
void Sonnet::Highlighter::setActive | ( | bool | active | ) |
Enable/Disable spell checking.
If active
is true then spell checking is enabled; otherwise it is disabled. Note that you have to disable automatic (de)activation with setAutomatic() before you change the state of spell checking if you want to persistently enable/disable spell checking.
- Parameters
-
active if true, then spell checking is enabled
- See also
- isActive(), setAutomatic()
Definition at line 254 of file highlighter.cpp.
void Sonnet::Highlighter::setAutomatic | ( | bool | automatic | ) |
Definition at line 212 of file highlighter.cpp.
void Sonnet::Highlighter::setCurrentLanguage | ( | const QString & | lang | ) |
Definition at line 300 of file highlighter.cpp.
|
protected |
Definition at line 206 of file highlighter.cpp.
|
protectedvirtual |
Definition at line 324 of file highlighter.cpp.
void Sonnet::Highlighter::setMisspelledColor | ( | const QColor & | color | ) |
Sets the color in which the highlighter underlines misspelled words.
- Since
- 4.2
Definition at line 438 of file highlighter.cpp.
|
slot |
Definition at line 222 of file highlighter.cpp.
|
slot |
Definition at line 161 of file highlighter.cpp.
bool Sonnet::Highlighter::spellCheckerFound | ( | ) | const |
Definition at line 139 of file highlighter.cpp.
QStringList Sonnet::Highlighter::suggestionsForWord | ( | const QString & | word, |
int | max = 10 |
||
) |
Returns a list of suggested replacements for the given misspelled word.
If the word is not misspelled, the list will be empty.
- Parameters
-
word the misspelled word max at most this many suggestions will be returned. If this is -1, as many suggestions as the spell backend supports will be returned.
- Returns
- a list of suggested replacements for the word
- Since
- 4.1
Definition at line 423 of file highlighter.cpp.
|
protectedvirtual |
Definition at line 333 of file highlighter.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.