KHTML
#include <css_value.h>
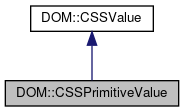
Public Types | |
enum | UnitTypes { CSS_UNKNOWN = 0, CSS_NUMBER = 1, CSS_PERCENTAGE = 2, CSS_EMS = 3, CSS_EXS = 4, CSS_CHS = 5, CSS_REMS = 6, CSS_PX = 7, CSS_CM = 8, CSS_MM = 9, CSS_IN = 10, CSS_PT = 11, CSS_PC = 12, CSS_DEG = 13, CSS_RAD = 14, CSS_GRAD = 15, CSS_MS = 16, CSS_S = 17, CSS_HZ = 18, CSS_KHZ = 19, CSS_DIMENSION = 20, CSS_STRING = 21, CSS_URI = 22, CSS_IDENT = 23, CSS_ATTR = 24, CSS_COUNTER = 25, CSS_RECT = 26, CSS_RGBCOLOR = 27, CSS_DPI = 28, CSS_DPCM = 29, CSS_PAIR = 100, CSS_HTML_RELATIVE = 255 } |
![]() | |
enum | UnitTypes { CSS_INHERIT = 0, CSS_PRIMITIVE_VALUE = 1, CSS_VALUE_LIST = 2, CSS_CUSTOM = 3, CSS_INITIAL = 4, CSS_SVG_VALUE = 1001 } |
Public Member Functions | |
CSSPrimitiveValue () | |
CSSPrimitiveValue (const CSSPrimitiveValue &other) | |
CSSPrimitiveValue (const CSSValue &other) | |
CSSPrimitiveValue (CSSPrimitiveValueImpl *impl) | |
~CSSPrimitiveValue () | |
Counter | getCounterValue () const |
float | getFloatValue (unsigned short unitType) const |
Rect | getRectValue () const |
RGBColor | getRGBColorValue () const |
DOM::DOMString | getStringValue () const |
CSSPrimitiveValue & | operator= (const CSSPrimitiveValue &other) |
CSSPrimitiveValue & | operator= (const CSSValue &other) |
unsigned short | primitiveType () const |
void | setFloatValue (unsigned short unitType, float floatValue) |
void | setStringValue (unsigned short stringType, const DOM::DOMString &stringValue) |
![]() | |
CSSValue () | |
CSSValue (const CSSValue &other) | |
CSSValue (CSSValueImpl *impl) | |
~CSSValue () | |
DOM::DOMString | cssText () const |
unsigned short | cssValueType () const |
CSSValueImpl * | handle () const |
bool | isCSSPrimitiveValue () const |
bool | isCSSValueList () const |
bool | isNull () const |
CSSValue & | operator= (const CSSValue &other) |
void | setCssText (const DOM::DOMString &) |
Additional Inherited Members | |
![]() | |
CSSValueImpl * | impl |
Detailed Description
The CSSPrimitiveValue
interface represents a single CSS value .
This interface may be used to determine the value of a specific style property currently set in a block or to set a specific style properties explicitly within the block. An instance of this interface can be obtained from the getPropertyCSSValue
method of the CSSStyleDeclaration
interface.
Definition at line 372 of file css_value.h.
Member Enumeration Documentation
An integer indicating which type of unit applies to the value.
Definition at line 389 of file css_value.h.
Constructor & Destructor Documentation
DOM::CSSPrimitiveValue::CSSPrimitiveValue | ( | ) |
Definition at line 278 of file css_value.cpp.
DOM::CSSPrimitiveValue::CSSPrimitiveValue | ( | const CSSPrimitiveValue & | other | ) |
Definition at line 282 of file css_value.cpp.
DOM::CSSPrimitiveValue::CSSPrimitiveValue | ( | const CSSValue & | other | ) |
Definition at line 286 of file css_value.cpp.
DOM::CSSPrimitiveValue::CSSPrimitiveValue | ( | CSSPrimitiveValueImpl * | impl | ) |
Definition at line 292 of file css_value.cpp.
DOM::CSSPrimitiveValue::~CSSPrimitiveValue | ( | ) |
Definition at line 321 of file css_value.cpp.
Member Function Documentation
Counter DOM::CSSPrimitiveValue::getCounterValue | ( | ) | const |
This method is used to get the Counter value.
If this CSS value doesn't contain a counter value, a DOMException
is raised. Modification to the corresponding style property can be achieved using the Counter
interface.
- Returns
- The Counter value.
- Exceptions
-
DOMException INVALID_ACCESS_ERR: Raises if the CSS value doesn't contain a Counter value.
Definition at line 369 of file css_value.cpp.
float DOM::CSSPrimitiveValue::getFloatValue | ( | unsigned short | unitType | ) | const |
This method is used to get a float value in a specified unit.
If this CSS value doesn't contain a float value or can't be converted into the specified unit, a DOMException
is raised.
- Parameters
-
unitType A unit code to get the float value. The unit code can only be a float unit type (e.g. CSS_NUMBER
,CSS_PERCENTAGE
,CSS_EMS
,CSS_EXS
,CSS_PX
,CSS_PX
,CSS_CM
,CSS_MM
,CSS_IN
,CSS_PT
,CSS_PC
,CSS_DEG
,CSS_RAD
,CSS_GRAD
,CSS_MS
,CSS_S
,CSS_HZ
,CSS_KHZ
,CSS_DIMENSION
).
- Returns
- The float value in the specified unit.
- Exceptions
-
DOMException INVALID_ACCESS_ERR: Raises if the CSS value doesn't contain a float value or if the float value can't be converted into the specified unit.
Definition at line 342 of file css_value.cpp.
Rect DOM::CSSPrimitiveValue::getRectValue | ( | ) | const |
This method is used to get the Rect value.
If this CSS value doesn't contain a rect value, a DOMException
is raised. Modification to the corresponding style property can be achieved using the Rect
interface.
- Returns
- The Rect value.
- Exceptions
-
DOMException INVALID_ACCESS_ERR: Raises if the CSS value doesn't contain a Rect value.
Definition at line 375 of file css_value.cpp.
RGBColor DOM::CSSPrimitiveValue::getRGBColorValue | ( | ) | const |
This method is used to get the RGB color.
If this CSS value doesn't contain a RGB color value, a DOMException
is raised. Modification to the corresponding style property can be achieved using the RGBColor
interface.
- Returns
- the RGB color value.
- Exceptions
-
DOMException INVALID_ACCESS_ERR: Raises if the attached property can't return a RGB color value.
Definition at line 381 of file css_value.cpp.
DOMString DOM::CSSPrimitiveValue::getStringValue | ( | ) | const |
This method is used to get the string value in a specified unit.
If the CSS value doesn't contain a string value, a DOMException
is raised.
- Returns
- The string value in the current unit. The current
valueType
can only be a string unit type (e.g.CSS_URI
,CSS_IDENT
andCSS_ATTR
).
- Exceptions
-
DOMException INVALID_ACCESS_ERR: Raises if the CSS value doesn't contain a string value.
Definition at line 363 of file css_value.cpp.
CSSPrimitiveValue & DOM::CSSPrimitiveValue::operator= | ( | const CSSPrimitiveValue & | other | ) |
Definition at line 296 of file css_value.cpp.
CSSPrimitiveValue & DOM::CSSPrimitiveValue::operator= | ( | const CSSValue & | other | ) |
Definition at line 306 of file css_value.cpp.
unsigned short DOM::CSSPrimitiveValue::primitiveType | ( | ) | const |
The type of the value as defined by the constants specified above.
Definition at line 325 of file css_value.cpp.
void DOM::CSSPrimitiveValue::setFloatValue | ( | unsigned short | unitType, |
float | floatValue | ||
) |
A method to set the float value with a specified unit.
If the property attached with this value can not accept the specified unit or the float value, the value will be unchanged and a DOMException
will be raised.
- Parameters
-
unitType A unit code as defined above. The unit code can only be a float unit type (e.g. NUMBER
,PERCENTAGE
,CSS_EMS
,CSS_EXS
,CSS_PX
,CSS_PX
,CSS_CM
,CSS_MM
,CSS_IN
,CSS_PT
,CSS_PC
,CSS_DEG
,CSS_RAD
,CSS_GRAD
,CSS_MS
,CSS_S
,CSS_HZ
,CSS_KHZ
,CSS_DIMENSION
).floatValue The new float value.
- Returns
- Exceptions
-
DOMException INVALID_ACCESS_ERR: Raises if the attached property doesn't support the float value or the unit type.
NO_MODIFICATION_ALLOWED_ERR: Raised if this property is readonly.
Definition at line 331 of file css_value.cpp.
void DOM::CSSPrimitiveValue::setStringValue | ( | unsigned short | stringType, |
const DOM::DOMString & | stringValue | ||
) |
A method to set the string value with a specified unit.
If the property attached to this value can't accept the specified unit or the string value, the value will be unchanged and a DOMException
will be raised.
- Parameters
-
stringType A string code as defined above. The string code can only be a string unit type (e.g. CSS_URI
,CSS_IDENT
,CSS_INHERIT
andCSS_ATTR
).stringValue The new string value. If the stringType
is equal toCSS_INHERIT
, thestringValue
should beinherit
.
- Returns
- Exceptions
-
DOMException INVALID_ACCESS_ERR: Raises if the CSS value doesn't contain a string value or if the string value can't be converted into the specified unit.
NO_MODIFICATION_ALLOWED_ERR: Raised if this property is readonly.
Definition at line 351 of file css_value.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.