KHTML
#include <html_form.h>
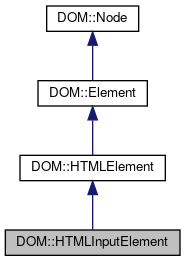
Protected Member Functions | |
HTMLInputElement (HTMLInputElementImpl *impl) | |
![]() | |
HTMLElement (HTMLElementImpl *impl) | |
void | assignOther (const Node &other, int elementId) |
![]() | |
Element (ElementImpl *_impl) | |
Detailed Description
Form control.
Note. Depending upon the environment the page is being viewed, the value property may be read-only for the file upload input type. For the "password" input type, the actual value returned may be masked to prevent unauthorized use. See the INPUT element definition in HTML 4.0.
Definition at line 349 of file html_form.h.
Constructor & Destructor Documentation
HTMLInputElement::HTMLInputElement | ( | ) |
Definition at line 299 of file html_form.cpp.
HTMLInputElement::HTMLInputElement | ( | const HTMLInputElement & | other | ) |
Definition at line 303 of file html_form.cpp.
|
inline |
Definition at line 354 of file html_form.h.
|
protected |
Definition at line 307 of file html_form.cpp.
HTMLInputElement::~HTMLInputElement | ( | ) |
Definition at line 323 of file html_form.cpp.
Member Function Documentation
DOMString HTMLInputElement::accept | ( | ) | const |
A comma-separated list of content types that a server processing this form will handle correctly.
See the accept attribute definition in HTML 4.0.
Definition at line 358 of file html_form.cpp.
DOMString HTMLInputElement::accessKey | ( | ) | const |
A single character access key to give access to the form control.
See the accesskey attribute definition in HTML 4.0.
Definition at line 369 of file html_form.cpp.
DOMString HTMLInputElement::align | ( | ) | const |
Aligns this object (vertically or horizontally) with respect to its surrounding text.
See the align attribute definition in HTML 4.0. This attribute is deprecated in HTML 4.0.
Definition at line 380 of file html_form.cpp.
DOMString HTMLInputElement::alt | ( | ) | const |
Alternate text for user agents not rendering the normal content of this element.
See the alt attribute definition in HTML 4.0.
Definition at line 391 of file html_form.cpp.
void HTMLInputElement::blur | ( | ) |
Removes keyboard focus from this element.
Definition at line 564 of file html_form.cpp.
bool HTMLInputElement::checked | ( | ) | const |
Describes whether a radio or check box is checked, when type
has the value "Radio" or "Checkbox".
The value is true if explicitly set. Represents the current state of the checkbox or radio button. See the checked attribute definition in HTML 4.0.
Definition at line 402 of file html_form.cpp.
void HTMLInputElement::click | ( | ) |
Simulate a mouse-click.
For INPUT
elements whose type
attribute has one of the following values: "Button", "Checkbox", "Radio", "Reset", or "Submit".
Definition at line 582 of file html_form.cpp.
bool HTMLInputElement::defaultChecked | ( | ) | const |
When type
has the value "Radio" or "Checkbox", stores the initial value of the checked
attribute.
Definition at line 341 of file html_form.cpp.
DOMString HTMLInputElement::defaultValue | ( | ) | const |
Stores the initial control value (i.e., the initial value of value
).
Definition at line 327 of file html_form.cpp.
bool HTMLInputElement::disabled | ( | ) | const |
The control is unavailable in this context.
See the disabled attribute definition in HTML 4.0.
Definition at line 426 of file html_form.cpp.
void HTMLInputElement::focus | ( | ) |
Gives keyboard focus to this element.
Definition at line 570 of file html_form.cpp.
HTMLFormElement HTMLInputElement::form | ( | ) | const |
KDE 4.0: remove.
Definition at line 353 of file html_form.cpp.
long HTMLInputElement::getSize | ( | ) | const |
Size information.
The precise meaning is specific to each type of field. See the size attribute definition in HTML 4.0.
Definition at line 493 of file html_form.cpp.
bool HTMLInputElement::indeterminate | ( | ) | const |
Describes whether a radio box is indeterminate.
Definition at line 414 of file html_form.cpp.
long HTMLInputElement::maxLength | ( | ) | const |
Maximum number of characters for text fields, when type
has the value "Text" or "Password".
See the maxlength attribute definition in HTML 4.0.
Definition at line 440 of file html_form.cpp.
DOMString HTMLInputElement::name | ( | ) | const |
Form control or object name when submitted with a form.
See the name attribute definition in HTML 4.0.
Definition at line 454 of file html_form.cpp.
HTMLInputElement & HTMLInputElement::operator= | ( | const HTMLInputElement & | other | ) |
Definition at line 317 of file html_form.cpp.
HTMLInputElement & HTMLInputElement::operator= | ( | const Node & | other | ) |
Definition at line 311 of file html_form.cpp.
bool HTMLInputElement::readOnly | ( | ) | const |
This control is read-only.
When type
has the value "text" or "password" only. See the readonly attribute definition in HTML 4.0.
Definition at line 465 of file html_form.cpp.
void HTMLInputElement::select | ( | ) |
Select the contents of the text area.
For INPUT
elements whose type
attribute has one of the following values: "Text", "File", or "Password".
Definition at line 576 of file html_form.cpp.
long HTMLInputElement::selectionEnd | ( | ) |
Returns the character offset of end of selection, or if none, the cursor position.
This operation is only supported if the type of this element is text; otherwise -1 is returned. NOTE: this method is not part of the DOM, but a Mozilla extension
Definition at line 595 of file html_form.cpp.
long HTMLInputElement::selectionStart | ( | ) |
Returns the character offset of beginning of selection, or if none, the cursor position.
This operation is only supported if the type of this element is text; otherwise -1 is returned. NOTE: this method is not part of the DOM, but a Mozilla extension
Definition at line 588 of file html_form.cpp.
void HTMLInputElement::setAccept | ( | const DOMString & | value | ) |
see accept
Definition at line 364 of file html_form.cpp.
void HTMLInputElement::setAccessKey | ( | const DOMString & | value | ) |
see accessKey
Definition at line 375 of file html_form.cpp.
void HTMLInputElement::setAlign | ( | const DOMString & | value | ) |
see align
Definition at line 386 of file html_form.cpp.
void HTMLInputElement::setAlt | ( | const DOMString & | value | ) |
see alt
Definition at line 397 of file html_form.cpp.
void HTMLInputElement::setChecked | ( | bool | _checked | ) |
see checked
Definition at line 408 of file html_form.cpp.
void HTMLInputElement::setDefaultChecked | ( | bool | _defaultChecked | ) |
see defaultChecked
Definition at line 347 of file html_form.cpp.
void HTMLInputElement::setDefaultValue | ( | const DOMString & | value | ) |
see defaultValue
Definition at line 336 of file html_form.cpp.
void HTMLInputElement::setDisabled | ( | bool | _disabled | ) |
see disabled
Definition at line 432 of file html_form.cpp.
void HTMLInputElement::setIndeterminate | ( | bool | _indeterminate | ) |
see indeterminate
Definition at line 420 of file html_form.cpp.
void HTMLInputElement::setMaxLength | ( | long | _maxLength | ) |
see maxLength
Definition at line 446 of file html_form.cpp.
void HTMLInputElement::setName | ( | const DOMString & | value | ) |
see name
Definition at line 460 of file html_form.cpp.
void HTMLInputElement::setReadOnly | ( | bool | _readOnly | ) |
see readOnly
Definition at line 471 of file html_form.cpp.
void HTMLInputElement::setSelectionEnd | ( | long | offset | ) |
Move the end of the selection (and the cursor) to the given offset in text This call has no effect if the type of this input element isn't text NOTE: this method is not part of the DOM, but a Mozilla extension.
Definition at line 608 of file html_form.cpp.
void HTMLInputElement::setSelectionRange | ( | long | start, |
long | end | ||
) |
Makes the position span from start to end, and positions the cursor after the selection.
This call has no effect if the type of this input element isn't text or if it is not rendered. NOTE: this method is not part of the DOM, but a Mozilla extension
Definition at line 614 of file html_form.cpp.
void HTMLInputElement::setSelectionStart | ( | long | offset | ) |
Move the beginning of the selection to the given offset in text This call has no effect if the type of this input element isn't text NOTE: this method is not part of the DOM, but a Mozilla extension.
Definition at line 602 of file html_form.cpp.
void HTMLInputElement::setSize | ( | const DOMString & | value | ) |
Definition at line 487 of file html_form.cpp.
void HTMLInputElement::setSize | ( | long | value | ) |
see getSize
Definition at line 499 of file html_form.cpp.
void HTMLInputElement::setSrc | ( | const DOMString & | value | ) |
see src
Definition at line 511 of file html_form.cpp.
void HTMLInputElement::setTabIndex | ( | long | _tabIndex | ) |
see tabIndex
Definition at line 522 of file html_form.cpp.
void HTMLInputElement::setType | ( | const DOMString & | _type | ) |
see type
Definition at line 534 of file html_form.cpp.
void HTMLInputElement::setUseMap | ( | const DOMString & | value | ) |
see useMap
Definition at line 546 of file html_form.cpp.
void HTMLInputElement::setValue | ( | const DOMString & | value | ) |
see value
Definition at line 557 of file html_form.cpp.
DOMString HTMLInputElement::size | ( | ) | const |
Definition at line 479 of file html_form.cpp.
DOMString HTMLInputElement::src | ( | ) | const |
When the type
attribute has the value "Image", this attribute specifies the location of the image to be used to decorate the graphical submit button.
See the src attribute definition in HTML 4.0.
Definition at line 504 of file html_form.cpp.
long HTMLInputElement::tabIndex | ( | ) | const |
Index that represents the element's position in the tabbing order.
See the tabindex attribute definition in HTML 4.0.
Definition at line 516 of file html_form.cpp.
DOMString HTMLInputElement::type | ( | ) | const |
The type of control created.
See the type attribute definition in HTML 4.0.
Definition at line 528 of file html_form.cpp.
DOMString HTMLInputElement::useMap | ( | ) | const |
Use client-side image map.
See the usemap attribute definition in HTML 4.0.
Definition at line 540 of file html_form.cpp.
DOMString HTMLInputElement::value | ( | ) | const |
The current form control value.
Used for radio buttons and check boxes. See the value attribute definition in HTML 4.0.
Definition at line 551 of file html_form.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.