KHTML
#include <dom2_events.h>
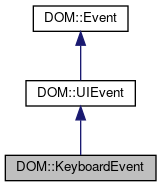
Public Types | |
enum | KeyLocation { DOM_KEY_LOCATION_STANDARD = 0x00, DOM_KEY_LOCATION_LEFT = 0x01, DOM_KEY_LOCATION_RIGHT = 0x02, DOM_KEY_LOCATION_NUMPAD = 0x03 } |
![]() | |
enum | PhaseType { CAPTURING_PHASE = 1, AT_TARGET = 2, BUBBLING_PHASE = 3 } |
Public Member Functions | |
KeyboardEvent () | |
KeyboardEvent (const KeyboardEvent &other) | |
KeyboardEvent (const Event &other) | |
virtual | ~KeyboardEvent () |
bool | altKey () const |
bool | ctrlKey () const |
bool | getModifierState (DOMString keyIdentifierArg) const |
void | initKeyboardEvent (DOMString typeArg, bool canBubbleArg, bool cancelableArg, AbstractView viewArg, DOMString keyIdentifierArg, unsigned long keyLocationArg, DOMString modifiersList) |
DOMString | keyIdentifier () const |
unsigned long | keyLocation () const |
bool | metaKey () const |
KeyboardEvent & | operator= (const KeyboardEvent &other) |
KeyboardEvent & | operator= (const Event &other) |
bool | shiftKey () const |
![]() | |
UIEvent () | |
UIEvent (const UIEvent &other) | |
UIEvent (const Event &other) | |
virtual | ~UIEvent () |
int | charCode () const |
long | detail () const |
void | initUIEvent (const DOMString &typeArg, bool canBubbleArg, bool cancelableArg, const AbstractView &viewArg, long detailArg) |
int | keyCode () const |
int | layerX () const |
int | layerY () const |
UIEvent & | operator= (const UIEvent &other) |
UIEvent & | operator= (const Event &other) |
int | pageX () const |
int | pageY () const |
AbstractView | view () const |
int | which () const |
![]() | |
Event () | |
Event (const Event &other) | |
Event (EventImpl *i) | |
virtual | ~Event () |
bool | bubbles () const |
bool | cancelable () const |
Node | currentTarget () const |
unsigned short | eventPhase () const |
EventImpl * | handle () const |
void | initEvent (const DOMString &eventTypeArg, bool canBubbleArg, bool cancelableArg) |
bool | isNull () const |
Event & | operator= (const Event &other) |
void | preventDefault () |
void | stopPropagation () |
Node | target () const |
DOMTimeStamp | timeStamp () const |
DOMString | type () const |
Additional Inherited Members | |
![]() | |
UIEvent (UIEventImpl *impl) | |
![]() | |
EventImpl * | impl |
Detailed Description
Introduced in DOM Level 3.
DOM::KeyboardEvent The KeyboardEvent interface provides specific contextual information associated with keyboard devices. Each keyboard event references a key using an identifier. Keyboard events are commonly directed at the element that has the focus.
The KeyboardEvent interface provides convenient attributes for some common modifiers keys: KeyboardEvent.ctrlKey, KeyboardEvent.shiftKey, KeyboardEvent.altKey, KeyboardEvent.metaKey. These attributes are equivalent to use the method KeyboardEvent.getModifierState(keyIdentifierArg) with "Control", "Shift", "Alt", or "Meta" respectively.
To create an instance of the KeyboardEvent interface, use the DocumentEvent.createEvent("KeyboardEvent") method call.
Definition at line 630 of file dom2_events.h.
Member Enumeration Documentation
Definition at line 639 of file dom2_events.h.
Constructor & Destructor Documentation
KeyboardEvent::KeyboardEvent | ( | ) |
Definition at line 536 of file dom2_events.cpp.
KeyboardEvent::KeyboardEvent | ( | const KeyboardEvent & | other | ) |
Definition at line 540 of file dom2_events.cpp.
KeyboardEvent::KeyboardEvent | ( | const Event & | other | ) |
Definition at line 544 of file dom2_events.cpp.
|
virtual |
Definition at line 567 of file dom2_events.cpp.
Member Function Documentation
bool KeyboardEvent::altKey | ( | ) | const |
altKey of type boolean, readonly
true if the alt (Alt) key modifier is activated.
Definition at line 591 of file dom2_events.cpp.
bool KeyboardEvent::ctrlKey | ( | ) | const |
ctrlKey of type boolean, readonly
true if the control (Ctrl) key modifier is activated.
Definition at line 581 of file dom2_events.cpp.
getModifierState
This methods queries the state of a modifier using a key identifier
Parameters:
keyIdentifierArg of type DOMString A modifier key identifier. Supported modifier keys are "Alt", "Control", "Meta", "Shift".
Return Value boolean true if it is modifier key and the modifier is activated, false otherwise.
Definition at line 601 of file dom2_events.cpp.
void KeyboardEvent::initKeyboardEvent | ( | DOMString | typeArg, |
bool | canBubbleArg, | ||
bool | cancelableArg, | ||
AbstractView | viewArg, | ||
DOMString | keyIdentifierArg, | ||
unsigned long | keyLocationArg, | ||
DOMString | modifiersList | ||
) |
initKeyboardEvent
The initKeyboardEvent method is used to initialize the value of a KeyboardEvent object and has the same behavior as UIEvent.initUIEvent(). The value of UIEvent.detail remains undefined.
Parameters: typeArg of type DOMString Specifies the event type. canBubbleArg of type boolean Specifies whether or not the event can bubble. cancelableArg of type boolean Specifies whether or not the event's default action can be prevent. viewArg of type views::AbstractView Specifies the TextEvent's AbstractView. keyIdentifierArg of type DOMString Specifies KeyboardEvent.keyIdentifier. keyLocationArg of type unsigned long Specifies KeyboardEvent.keyLocation. modifiersList of type DOMString A white space separated list of modifier key identifiers to be activated on this object.
Definition at line 606 of file dom2_events.cpp.
DOMString KeyboardEvent::keyIdentifier | ( | ) | const |
keyIdentifier of type DOMString, readonly
keyIdentifier holds the identifier of the key. The key identifiers are defined in Appendix A.2 "Key identifiers set" (http://www.w3.org/TR/DOM-Level-3-Events/keyset.html#KeySet-Set)
Definition at line 571 of file dom2_events.cpp.
unsigned long KeyboardEvent::keyLocation | ( | ) | const |
keyLocation of type unsigned long, readonly
The keyLocation attribute contains an indication of the location of they key on the device. See the KeyLocation enum for possible values
Definition at line 576 of file dom2_events.cpp.
bool KeyboardEvent::metaKey | ( | ) | const |
metaKey of type boolean, readonly
true if the meta (Meta) key modifier is activated.
Definition at line 596 of file dom2_events.cpp.
KeyboardEvent & KeyboardEvent::operator= | ( | const KeyboardEvent & | other | ) |
Definition at line 549 of file dom2_events.cpp.
KeyboardEvent & KeyboardEvent::operator= | ( | const Event & | other | ) |
Definition at line 555 of file dom2_events.cpp.
bool KeyboardEvent::shiftKey | ( | ) | const |
shiftKey of type boolean, readonly
true if the shift (Shift) key modifier is activated.
Definition at line 586 of file dom2_events.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.