KHTML
#include <kjavaappletserver.h>
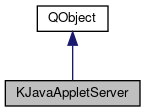
Public Member Functions | |
KJavaAppletServer () | |
~KJavaAppletServer () | |
QString | appletLabel () |
bool | callMember (QStringList &args, QStringList &ret_args) |
bool | createApplet (int contextId, int appletId, const QString &name, const QString &clazzName, const QString &baseURL, const QString &user, const QString &password, const QString &authname, const QString &codeBase, const QString &jarFile, QSize size, const QMap< QString, QString > ¶ms, const QString &windowTitle) |
void | createContext (int contextId, KJavaAppletContext *context) |
void | derefObject (QStringList &args) |
void | destroyApplet (int contextId, int appletId) |
void | destroyContext (int contextId) |
void | endWaitForReturnData () |
bool | getMember (QStringList &args, QStringList &ret_args) |
void | initApplet (int contextId, int appletId) |
KJavaProcess * | javaProcess () |
bool | putMember (QStringList &args) |
void | quit () |
void | removeDataJob (int loaderID) |
void | sendURLData (int loaderID, int code, const QByteArray &data) |
void | showConsole () |
void | startApplet (int contextId, int appletId) |
void | stopApplet (int contextId, int appletId) |
bool | usingKIO () |
void | waitForReturnData (JSStackFrame *) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static KJavaAppletServer * | allocateJavaServer () |
static void | freeJavaServer () |
static QString | getAppletLabel () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Slots | |
void | checkShutdown () |
void | killTimers () |
void | slotJavaRequest (const QByteArray &qb) |
void | timerEvent (QTimerEvent *) |
Protected Member Functions | |
void | setupJava (KJavaProcess *p) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
KJavaProcess * | process |
Additional Inherited Members | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Definition at line 42 of file kjavaappletserver.h.
Constructor & Destructor Documentation
KJavaAppletServer::KJavaAppletServer | ( | ) |
Create the applet server.
These shouldn't be used directly, use allocateJavaServer instead
Definition at line 135 of file kjavaappletserver.cpp.
KJavaAppletServer::~KJavaAppletServer | ( | ) |
Definition at line 155 of file kjavaappletserver.cpp.
Member Function Documentation
|
static |
A factory method that returns the default server.
This is the way this class is usually instantiated.
Definition at line 178 of file kjavaappletserver.cpp.
QString KJavaAppletServer::appletLabel | ( | ) |
Definition at line 173 of file kjavaappletserver.cpp.
bool KJavaAppletServer::callMember | ( | QStringList & | args, |
QStringList & | ret_args | ||
) |
Definition at line 763 of file kjavaappletserver.cpp.
|
protectedslot |
Definition at line 209 of file kjavaappletserver.cpp.
bool KJavaAppletServer::createApplet | ( | int | contextId, |
int | appletId, | ||
const QString & | name, | ||
const QString & | clazzName, | ||
const QString & | baseURL, | ||
const QString & | user, | ||
const QString & | password, | ||
const QString & | authname, | ||
const QString & | codeBase, | ||
const QString & | jarFile, | ||
QSize | size, | ||
const QMap< QString, QString > & | params, | ||
const QString & | windowTitle | ||
) |
Create an applet in the specified context with the specified id.
The applet name, class etc. are specified in the same way as in the HTML APPLET tag.
Definition at line 339 of file kjavaappletserver.cpp.
void KJavaAppletServer::createContext | ( | int | contextId, |
KJavaAppletContext * | context | ||
) |
Create an applet context with the specified id.
Definition at line 316 of file kjavaappletserver.cpp.
void KJavaAppletServer::derefObject | ( | QStringList & | args | ) |
Definition at line 773 of file kjavaappletserver.cpp.
void KJavaAppletServer::destroyApplet | ( | int | contextId, |
int | appletId | ||
) |
Destroy an applet in the specified context with the specified id.
Definition at line 407 of file kjavaappletserver.cpp.
void KJavaAppletServer::destroyContext | ( | int | contextId | ) |
Destroy the applet context with the specified id.
All the applets in the context will be destroyed as well.
Definition at line 328 of file kjavaappletserver.cpp.
void KJavaAppletServer::endWaitForReturnData | ( | ) |
Definition at line 716 of file kjavaappletserver.cpp.
|
static |
When you are done using your reference to the AppletServer, you must dereference it by calling freeJavaServer().
Definition at line 190 of file kjavaappletserver.cpp.
|
static |
This allows the KJavaAppletWidget to display some feedback in a QLabel while the applet is being loaded.
If the java process could not be started, an error message is displayed instead.
Definition at line 165 of file kjavaappletserver.cpp.
bool KJavaAppletServer::getMember | ( | QStringList & | args, |
QStringList & | ret_args | ||
) |
Definition at line 742 of file kjavaappletserver.cpp.
void KJavaAppletServer::initApplet | ( | int | contextId, |
int | appletId | ||
) |
This should be called by the KJavaAppletWidget.
Definition at line 397 of file kjavaappletserver.cpp.
|
inline |
Definition at line 135 of file kjavaappletserver.h.
|
protectedslot |
Definition at line 711 of file kjavaappletserver.cpp.
bool KJavaAppletServer::putMember | ( | QStringList & | args | ) |
Definition at line 752 of file kjavaappletserver.cpp.
void KJavaAppletServer::quit | ( | ) |
Shut down the KJAS server.
Definition at line 461 of file kjavaappletserver.cpp.
void KJavaAppletServer::removeDataJob | ( | int | loaderID | ) |
Removes KJavaDownloader from the list (deletes it too).
Definition at line 452 of file kjavaappletserver.cpp.
void KJavaAppletServer::sendURLData | ( | int | loaderID, |
int | code, | ||
const QByteArray & | data | ||
) |
Send data we got back from a KJavaDownloader back to the appropriate class loader.
Definition at line 443 of file kjavaappletserver.cpp.
|
protected |
Definition at line 218 of file kjavaappletserver.cpp.
void KJavaAppletServer::showConsole | ( | ) |
Show java console.
Definition at line 437 of file kjavaappletserver.cpp.
|
protectedslot |
Definition at line 469 of file kjavaappletserver.cpp.
void KJavaAppletServer::startApplet | ( | int | contextId, |
int | appletId | ||
) |
Start the specified applet.
Definition at line 417 of file kjavaappletserver.cpp.
void KJavaAppletServer::stopApplet | ( | int | contextId, |
int | appletId | ||
) |
Stop the specified applet.
Definition at line 427 of file kjavaappletserver.cpp.
|
protectedslot |
Definition at line 725 of file kjavaappletserver.cpp.
bool KJavaAppletServer::usingKIO | ( | ) |
Definition at line 777 of file kjavaappletserver.cpp.
void KJavaAppletServer::waitForReturnData | ( | JSStackFrame * | frame | ) |
Definition at line 730 of file kjavaappletserver.cpp.
Member Data Documentation
|
protected |
Definition at line 151 of file kjavaappletserver.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.