KHTML
#include "functions.h"
#include "path.h"
#include "predicate.h"
#include "util.h"
#include "tokenizer.h"
#include "expression.h"
#include "variablereference.h"
#include "dom/dom_string.h"
#include "dom/dom_exception.h"
#include "dom/dom3_xpath.h"
#include "xml/dom_stringimpl.h"
#include "xml/dom3_xpathimpl.h"
#include <QList>
#include <QPair>
#include <QtDebug>
#include <stdio.h>
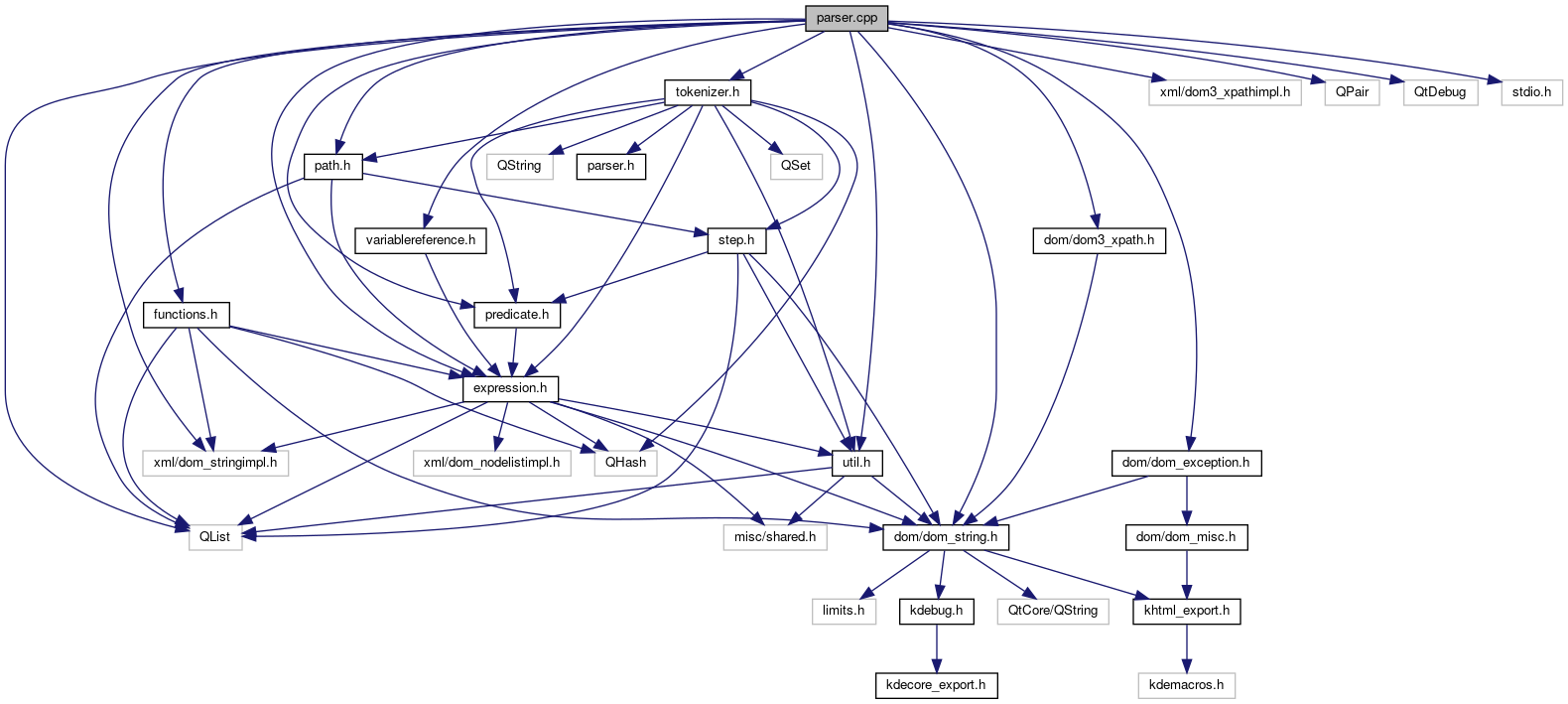
Go to the source code of this file.
Namespaces | |
khtml | |
khtml::XPath | |
Macros | |
#define | YY_(msgid) msgid |
#define | YY_LOCATION_PRINT(File, Loc) ((void) 0) |
#define | YY_REDUCE_PRINT(Rule) |
#define | YY_STACK_PRINT(Bottom, Top) |
#define | YY_SYMBOL_PRINT(Title, Type, Value, Location) |
#define | YYABORT goto yyabortlab |
#define | YYACCEPT goto yyacceptlab |
#define | YYBACKUP(Token, Value) |
#define | YYBISON 1 |
#define | YYBISON_VERSION "2.4.2" |
#define | yychar khtmlxpathyychar |
#define | yyclearin (yychar = YYEMPTY) |
#define | YYCOPY(To, From, Count) |
#define | yydebug khtmlxpathyydebug |
#define | YYDEBUG 1 |
#define | YYDPRINTF(Args) |
#define | YYEMPTY (-2) |
#define | YYEOF 0 |
#define | YYERRCODE 256 |
#define | yyerrok (yyerrstatus = 0) |
#define | yyerror khtmlxpathyyerror |
#define | YYERROR goto yyerrorlab |
#define | YYERROR_VERBOSE 0 |
#define | YYFAIL goto yyerrlab |
#define | YYFINAL 43 |
#define | YYFPRINTF fprintf |
#define | YYFREE free |
#define | YYID(n) (n) |
#define | YYINITDEPTH 200 |
#define | YYLAST 115 |
#define | yylex khtmlxpathyylex |
#define | YYLEX yylex () |
#define | YYLLOC_DEFAULT(Current, Rhs, N) |
#define | YYLSP_NEEDED 0 |
#define | yylval khtmlxpathyylval |
#define | YYMALLOC malloc |
#define | YYMAXDEPTH 10000 |
#define | YYMAXUTOK 275 |
#define | yynerrs khtmlxpathyynerrs |
#define | YYNNTS 26 |
#define | YYNRULES 60 |
#define | YYNSTATES 90 |
#define | YYNTOKENS 30 |
#define | YYPACT_NINF -45 |
#define | yyparse khtmlxpathyyparse |
#define | YYPOPSTACK(N) (yyvsp -= (N), yyssp -= (N)) |
#define | YYPULL 1 |
#define | YYPURE 0 |
#define | YYPUSH 0 |
#define | YYRECOVERING() (!!yyerrstatus) |
#define | YYRHSLOC(Rhs, K) ((Rhs)[K]) |
#define | YYSIZE_MAXIMUM ((YYSIZE_T) -1) |
#define | YYSIZE_T unsigned int |
#define | YYSKELETON_NAME "yacc.c" |
#define | YYSTACK_ALLOC YYMALLOC |
#define | YYSTACK_ALLOC_MAXIMUM YYSIZE_MAXIMUM |
#define | YYSTACK_BYTES(N) |
#define | YYSTACK_FREE YYFREE |
#define | YYSTACK_GAP_MAXIMUM (sizeof (union yyalloc) - 1) |
#define | YYSTACK_RELOCATE(Stack_alloc, Stack) |
#define | YYTABLE_NINF -1 |
#define | YYTERROR 1 |
#define | YYTOKEN_TABLE 0 |
#define | YYTOKENTYPE |
#define | YYTRANSLATE(YYX) ((unsigned int) (YYX) <= YYMAXUTOK ? yytranslate[YYX] : YYUNDEFTOK) |
#define | YYUNDEFTOK 2 |
#define | YYUSE(e) ((void) (e)) |
Typedefs | |
typedef short int | yytype_int16 |
typedef short int | yytype_int8 |
typedef unsigned short int | yytype_uint16 |
typedef unsigned char | yytype_uint8 |
Enumerations | |
enum | yytokentype { EQOP = 258, RELOP = 259, MULOP = 260, MINUS = 261, PLUS = 262, AND = 263, OR = 264, AXISNAME = 265, NODETYPE = 266, PI = 267, FUNCTIONNAME = 268, LITERAL = 269, VARIABLEREFERENCE = 270, NUMBER = 271, DOTDOT = 272, SLASHSLASH = 273, NAMETEST = 274, ERROR = 275, EQOP = 258, RELOP = 259, MULOP = 260, MINUS = 261, PLUS = 262, AND = 263, OR = 264, AXISNAME = 265, NODETYPE = 266, PI = 267, FUNCTIONNAME = 268, LITERAL = 269, VARIABLEREFERENCE = 270, NUMBER = 271, DOTDOT = 272, SLASHSLASH = 273, NAMETEST = 274, ERROR = 275 } |
Functions | |
for (;yybottom<=yytop;yybottom++) | |
for (yyi=0;yyi< yynrhs;yyi++) | |
if (!yymsg) yymsg | |
Expression * | khtml::XPath::khtmlParseXPathStatement (const DOMString &statement, int &ec) |
Expression * | khtmlParseXPathStatement (const DOM::DOMString &statement, int &ec) |
void | khtml::XPath::khtmlxpathyyerror (const char *str) |
switch (yytype) | |
static void | yy_reduce_print (yyvsp, yyrule) YYSTYPE *yyvsp |
static void | yy_stack_print (yybottom, yytop) yytype_int16 *yybottom |
static void | yy_symbol_print (yyoutput, yytype, yyvaluep) FILE *yyoutput |
YY_SYMBOL_PRINT (yymsg, yytype, yyvaluep, yylocationp) | |
static void | yy_symbol_value_print (yyoutput, yytype, yyvaluep) FILE *yyoutput |
static void | yydestruct (yymsg, yytype, yyvaluep) const char *yymsg |
else | YYFPRINTF (yyoutput,"nterm %s (", yytname[yytype]) |
YYFPRINTF (yyoutput,")") | |
YYFPRINTF (stderr,"\n") | |
YYFPRINTF (stderr,"Reducing stack by rule %d (line %lu):\n", yyrule-1, yylno) | |
int | yyparse () |
YYUSE (yyoutput) | |
Variables | |
static Expression * | _topExpr |
static int | xpathParseException |
int | yychar |
static const yytype_int8 | yycheck [] |
int | yydebug |
static const yytype_uint8 | yydefact [] |
static const yytype_int8 | yydefgoto [] |
int | yyi |
unsigned long int | yylno = yyrline[yyrule] |
YYSTYPE | yylval |
int | yynerrs |
static const yytype_int8 | yypact [] |
static const yytype_int8 | yypgoto [] |
static const yytype_uint8 | yyprhs [] |
static const yytype_uint8 | yyr1 [] |
static const yytype_uint8 | yyr2 [] |
static const yytype_int8 | yyrhs [] |
static const yytype_uint16 | yyrline [] |
int | yyrule |
static const yytype_uint8 | yystos [] |
static const yytype_uint8 | yytable [] |
static const char *const | yytname [] |
yytype_int16 * | yytop |
static const yytype_uint8 | yytranslate [] |
int | yytype |
YYSTYPE const *const | yyvaluep |
Macro Definition Documentation
#define YY_ | ( | msgid | ) | msgid |
Definition at line 261 of file parser.cpp.
#define YY_LOCATION_PRINT | ( | File, | |
Loc | |||
) | ((void) 0) |
Definition at line 759 of file parser.cpp.
#define YY_REDUCE_PRINT | ( | Rule | ) |
Definition at line 919 of file parser.cpp.
#define YY_STACK_PRINT | ( | Bottom, | |
Top | |||
) |
Definition at line 881 of file parser.cpp.
Definition at line 786 of file parser.cpp.
#define YYABORT goto yyabortlab |
Definition at line 679 of file parser.cpp.
#define YYACCEPT goto yyacceptlab |
Definition at line 678 of file parser.cpp.
Definition at line 700 of file parser.cpp.
#define YYBISON 1 |
Definition at line 45 of file parser.cpp.
#define YYBISON_VERSION "2.4.2" |
Definition at line 48 of file parser.cpp.
#define yychar khtmlxpathyychar |
Definition at line 70 of file parser.cpp.
Definition at line 674 of file parser.cpp.
#define YYCOPY | ( | To, | |
From, | |||
Count | |||
) |
#define yydebug khtmlxpathyydebug |
Definition at line 71 of file parser.cpp.
#define YYDEBUG 1 |
Definition at line 107 of file parser.cpp.
#define YYDPRINTF | ( | Args | ) |
Definition at line 780 of file parser.cpp.
#define YYEMPTY (-2) |
Definition at line 675 of file parser.cpp.
#define YYEOF 0 |
Definition at line 676 of file parser.cpp.
#define YYERRCODE 256 |
Definition at line 719 of file parser.cpp.
#define yyerrok (yyerrstatus = 0) |
Definition at line 673 of file parser.cpp.
#define yyerror khtmlxpathyyerror |
Definition at line 68 of file parser.cpp.
#define YYERROR goto yyerrorlab |
Definition at line 680 of file parser.cpp.
#define YYERROR_VERBOSE 0 |
Definition at line 129 of file parser.cpp.
#define YYFAIL goto yyerrlab |
Definition at line 690 of file parser.cpp.
#define YYFINAL 43 |
Definition at line 417 of file parser.cpp.
#define YYFPRINTF fprintf |
Definition at line 777 of file parser.cpp.
#define YYFREE free |
Definition at line 350 of file parser.cpp.
#define YYID | ( | n | ) | (n) |
Definition at line 274 of file parser.cpp.
#define YYINITDEPTH 200 |
Definition at line 938 of file parser.cpp.
#define YYLAST 115 |
Definition at line 419 of file parser.cpp.
#define yylex khtmlxpathyylex |
Definition at line 67 of file parser.cpp.
#define YYLEX yylex () |
Definition at line 769 of file parser.cpp.
#define YYLLOC_DEFAULT | ( | Current, | |
Rhs, | |||
N | |||
) |
Definition at line 728 of file parser.cpp.
#define YYLSP_NEEDED 0 |
Definition at line 63 of file parser.cpp.
#define yylval khtmlxpathyylval |
Definition at line 69 of file parser.cpp.
#define YYMALLOC malloc |
Definition at line 343 of file parser.cpp.
#define YYMAXDEPTH 10000 |
Definition at line 949 of file parser.cpp.
#define YYMAXUTOK 275 |
Definition at line 432 of file parser.cpp.
#define yynerrs khtmlxpathyynerrs |
Definition at line 72 of file parser.cpp.
#define YYNNTS 26 |
Definition at line 424 of file parser.cpp.
#define YYNRULES 60 |
Definition at line 426 of file parser.cpp.
#define YYNSTATES 90 |
Definition at line 428 of file parser.cpp.
#define YYNTOKENS 30 |
Definition at line 422 of file parser.cpp.
#define YYPACT_NINF -45 |
Definition at line 599 of file parser.cpp.
int yyparse khtmlxpathyyparse |
Definition at line 66 of file parser.cpp.
#define YYPOPSTACK | ( | N | ) | (yyvsp -= (N), yyssp -= (N)) |
#define YYPULL 1 |
Definition at line 60 of file parser.cpp.
#define YYPURE 0 |
Definition at line 54 of file parser.cpp.
#define YYPUSH 0 |
Definition at line 57 of file parser.cpp.
#define YYRECOVERING | ( | ) | (!!yyerrstatus) |
Definition at line 698 of file parser.cpp.
#define YYRHSLOC | ( | Rhs, | |
K | |||
) | ((Rhs)[K]) |
Definition at line 726 of file parser.cpp.
#define YYSIZE_MAXIMUM ((YYSIZE_T) -1) |
Definition at line 251 of file parser.cpp.
#define YYSIZE_T unsigned int |
Definition at line 247 of file parser.cpp.
#define YYSKELETON_NAME "yacc.c" |
Definition at line 51 of file parser.cpp.
#define YYSTACK_ALLOC YYMALLOC |
Definition at line 329 of file parser.cpp.
#define YYSTACK_ALLOC_MAXIMUM YYSIZE_MAXIMUM |
Definition at line 332 of file parser.cpp.
#define YYSTACK_BYTES | ( | N | ) |
Definition at line 376 of file parser.cpp.
#define YYSTACK_FREE YYFREE |
Definition at line 330 of file parser.cpp.
#define YYSTACK_GAP_MAXIMUM (sizeof (union yyalloc) - 1) |
Definition at line 372 of file parser.cpp.
#define YYSTACK_RELOCATE | ( | Stack_alloc, | |
Stack | |||
) |
Definition at line 403 of file parser.cpp.
#define YYTABLE_NINF -1 |
Definition at line 625 of file parser.cpp.
#define YYTERROR 1 |
Definition at line 718 of file parser.cpp.
#define YYTOKEN_TABLE 0 |
Definition at line 134 of file parser.cpp.
#define YYTOKENTYPE |
Definition at line 140 of file parser.cpp.
#define YYTRANSLATE | ( | YYX | ) | ((unsigned int) (YYX) <= YYMAXUTOK ? yytranslate[YYX] : YYUNDEFTOK) |
Definition at line 434 of file parser.cpp.
#define YYUNDEFTOK 2 |
Definition at line 431 of file parser.cpp.
#define YYUSE | ( | e | ) | ((void) (e)) |
Definition at line 267 of file parser.cpp.
Typedef Documentation
typedef short int yytype_int16 |
Definition at line 234 of file parser.cpp.
typedef short int yytype_int8 |
Definition at line 222 of file parser.cpp.
typedef unsigned short int yytype_uint16 |
Definition at line 228 of file parser.cpp.
typedef unsigned char yytype_uint8 |
Definition at line 213 of file parser.cpp.
Enumeration Type Documentation
enum yytokentype |
Definition at line 143 of file parser.cpp.
Function Documentation
for | ( | ;yybottom<=yytop;yybottom++ | ) |
Definition at line 873 of file parser.cpp.
for | ( | ) |
Definition at line 909 of file parser.cpp.
if | ( | ! | yymsg | ) |
Expression* khtmlParseXPathStatement | ( | const DOM::DOMString & | statement, |
int & | ec | ||
) |
Definition at line 2170 of file parser.cpp.
switch | ( | yytype | ) |
Definition at line 823 of file parser.cpp.
|
static |
|
static |
else YYFPRINTF | ( | yyoutput | ) |
YYFPRINTF | ( | yyoutput | , |
" | |||
) |
YYFPRINTF | ( | stderr | , |
"\n" | |||
) |
int yyparse | ( | ) |
Definition at line 1244 of file parser.cpp.
YYUSE | ( | yyoutput | ) |
Variable Documentation
|
static |
Definition at line 111 of file parser.cpp.
|
static |
Definition at line 112 of file parser.cpp.
int yychar |
Definition at line 1213 of file parser.cpp.
|
static |
Definition at line 642 of file parser.cpp.
int yydebug |
Definition at line 927 of file parser.cpp.
|
static |
Definition at line 576 of file parser.cpp.
|
static |
Definition at line 590 of file parser.cpp.
int yyi |
Definition at line 902 of file parser.cpp.
Definition at line 905 of file parser.cpp.
YYSTYPE yylval |
Definition at line 1216 of file parser.cpp.
int yynerrs |
Definition at line 1219 of file parser.cpp.
|
static |
Definition at line 600 of file parser.cpp.
|
static |
Definition at line 614 of file parser.cpp.
|
static |
Definition at line 473 of file parser.cpp.
|
static |
Definition at line 550 of file parser.cpp.
|
static |
Definition at line 562 of file parser.cpp.
|
static |
Definition at line 485 of file parser.cpp.
|
static |
Definition at line 508 of file parser.cpp.
int yyrule |
Definition at line 900 of file parser.cpp.
|
static |
Definition at line 660 of file parser.cpp.
|
static |
Definition at line 626 of file parser.cpp.
|
static |
Definition at line 523 of file parser.cpp.
yytype_int16* yytop |
Definition at line 869 of file parser.cpp.
|
static |
Definition at line 438 of file parser.cpp.
int yytype |
Definition at line 811 of file parser.cpp.
YYSTYPE * yyvaluep |
Definition at line 812 of file parser.cpp.
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.