KIO
#include <kfileitemdelegate.h>
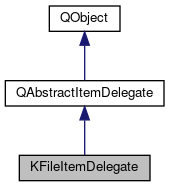
Public Types | |
enum | Information { NoInformation, Size, Permissions, OctalPermissions, Owner, OwnerAndGroup, CreationTime, ModificationTime, AccessTime, MimeType, FriendlyMimeType, LinkDest, LocalPathOrUrl, Comment } |
typedef QList< Information > | InformationList |
Public Slots | |
bool | helpEvent (QHelpEvent *event, QAbstractItemView *view, const QStyleOptionViewItem &option, const QModelIndex &index) |
QRegion | shape (const QStyleOptionViewItem &option, const QModelIndex &index) |
Public Member Functions | |
KFileItemDelegate (QObject *parent=0) | |
virtual | ~KFileItemDelegate () |
virtual QWidget * | createEditor (QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const |
virtual bool | editorEvent (QEvent *event, QAbstractItemModel *model, const QStyleOptionViewItem &option, const QModelIndex &index) |
virtual bool | eventFilter (QObject *object, QEvent *event) |
QRect | iconRect (const QStyleOptionViewItem &option, const QModelIndex &index) const |
bool | jobTransfersVisible () const |
QSize | maximumSize () const |
virtual void | paint (QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const |
virtual void | setEditorData (QWidget *editor, const QModelIndex &index) const |
void | setJobTransfersVisible (bool jobTransfersVisible) |
void | setMaximumSize (const QSize &size) |
virtual void | setModelData (QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const |
void | setShadowBlur (qreal radius) |
void | setShadowColor (const QColor &color) |
void | setShadowOffset (const QPointF &offset) |
void | setShowInformation (const InformationList &list) |
void | setShowInformation (Information information) |
void | setShowToolTipWhenElided (bool showToolTip) |
void | setWrapMode (QTextOption::WrapMode wrapMode) |
qreal | shadowBlur () const |
QColor | shadowColor () const |
QPointF | shadowOffset () const |
InformationList | showInformation () const |
bool | showToolTipWhenElided () const |
virtual QSize | sizeHint (const QStyleOptionViewItem &option, const QModelIndex &index) const |
virtual void | updateEditorGeometry (QWidget *editor, const QStyleOptionViewItem &option, const QModelIndex &index) const |
QTextOption::WrapMode | wrapMode () const |
![]() | |
QAbstractItemDelegate (QObject *parent) | |
virtual | ~QAbstractItemDelegate () |
void | closeEditor (QWidget *editor, QAbstractItemDelegate::EndEditHint hint) |
void | commitData (QWidget *editor) |
bool | helpEvent (QHelpEvent *event, QAbstractItemView *view, const QStyleOptionViewItem &option, const QModelIndex &index) |
virtual void | paint (QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const =0 |
virtual QSize | sizeHint (const QStyleOptionViewItem &option, const QModelIndex &index) const =0 |
void | sizeHintChanged (const QModelIndex &index) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Properties | |
InformationList | information |
bool | jobTransfersVisible |
QSize | maximumSize |
qreal | shadowBlur |
QColor | shadowColor |
QPointF | shadowOffset |
bool | showToolTipWhenElided |
![]() | |
objectName | |
Additional Inherited Members | |
![]() | |
QString | elidedText (const QFontMetrics &fontMetrics, int width, Qt::TextElideMode mode, const QString &text) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Detailed Description
KFileItemDelegate is intended to be used to provide a KDE file system view, when using one of the standard item views in Qt with KDirModel.
While primarily intended to be used with KDirModel, it uses Qt::DecorationRole and Qt::DisplayRole for the icons and text labels, just like QItemDelegate, and can thus be used with any standard model.
When used with KDirModel however, KFileItemDelegate can change the way the display and/or decoration roles are drawn, based on properties of the file items. For example, if the file item is a symbolic link, it will use an italic font to draw the file name.
KFileItemDelegate also supports showing additional information about the file items below the icon labels.
Which information should be shown, if any, is controlled by the information property, which is a list that can be set by calling setShowInformation(), and read by calling showInformation(). By default this list is empty.
To use KFileItemDelegate, instantiate an object from the delegate, and call setItemDelegate() in one of the standard item views in Qt:
Definition at line 67 of file kfileitemdelegate.h.
Member Typedef Documentation
Definition at line 179 of file kfileitemdelegate.h.
Member Enumeration Documentation
This enum defines the additional information that can be displayed below item labels in icon views.
The information will only be shown for indexes for which the model provides a valid value for KDirModel::FileItemRole, and only when there's sufficient vertical space to display at least one line of the information, along with the display label.
For the number of items to be shown for folders, the model must provide a valid value for KDirMode::ChildCountRole, in addition to KDirModel::FileItemRole.
Note that KFileItemDelegate will not call KFileItem::determineMimeType() if KFileItem::isMimeTypeKnown() returns false, so if you want to display mime types you should use a KMimeTypeResolver with the model and the view, to ensure that mime types are resolved. If the mime type isn't known, "Unknown" will be displayed until the mime type has been successfully resolved.
Definition at line 162 of file kfileitemdelegate.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a new KFileItemDelegate.
- Parameters
-
parent The parent object for the delegate.
Definition at line 910 of file kfileitemdelegate.cpp.
|
virtual |
Destroys the item delegate.
Definition at line 935 of file kfileitemdelegate.cpp.
Member Function Documentation
|
virtual |
Reimplemented from QAbstractItemDelegate.
Reimplemented from QAbstractItemDelegate.
Definition at line 1439 of file kfileitemdelegate.cpp.
|
virtual |
Reimplemented from QAbstractItemDelegate.
Reimplemented from QAbstractItemDelegate.
Definition at line 1455 of file kfileitemdelegate.cpp.
Reimplemented from QAbstractItemDelegate.
Reimplemented from QObject.
Definition at line 1630 of file kfileitemdelegate.cpp.
|
slot |
Reimplemented from QAbstractItemDelegate.
Definition at line 1546 of file kfileitemdelegate.cpp.
QRect KFileItemDelegate::iconRect | ( | const QStyleOptionViewItem & | option, |
const QModelIndex & | index | ||
) | const |
Returns the rectangle of the icon that is aligned inside the decoration rectangle.
- Since
- 4.4
Definition at line 1099 of file kfileitemdelegate.cpp.
bool KFileItemDelegate::jobTransfersVisible | ( | ) | const |
Returns whether or not the displaying of job transfers is enabled.
- See also
- setJobTransfersVisible()
- Since
- 4.5
QSize KFileItemDelegate::maximumSize | ( | ) | const |
|
virtual |
Paints the item indicated by index
, using painter
.
The item will be drawn in the rectangle specified by option.rect. The correct size for that rectangle can be obtained by calling sizeHint().
This function will use the following data values if the model provides them for the item, in place of the values in option:
- Qt::FontRole The font that should be used for the display role.
- Qt::AlignmentRole The alignment of the display role.
- Qt::ForegroundRole The text color for the display role.
- Qt::BackgroundRole The background color for the item.
This function is reimplemented from QAbstractItemDelegate.
- Parameters
-
painter The painter with which to draw the item. option The style options that should be used when painting the item. index The index to the item that should be painted.
Definition at line 1245 of file kfileitemdelegate.cpp.
|
virtual |
Reimplemented from QAbstractItemDelegate.
Reimplemented from QAbstractItemDelegate.
Definition at line 1467 of file kfileitemdelegate.cpp.
void KFileItemDelegate::setJobTransfersVisible | ( | bool | jobTransfersVisible | ) |
Enable/Disable the displaying of an animated overlay that is shown for any destination urls (in the view).
When enabled, the animations (if any) will be drawn automatically.
Only the files/folders that are visible and have jobs associated with them will display the animation. You would likely not want this enabled if you perform some kind of custom painting that takes up a whole item, and will just make this(and what you paint) look funky.
Default is disabled.
Note: The model (KDirModel) needs to have it's method called with the same value, when you make the call to this method.
- Since
- 4.5
Definition at line 1107 of file kfileitemdelegate.cpp.
void KFileItemDelegate::setMaximumSize | ( | const QSize & | size | ) |
Sets the maximum size for KFileItemDelegate::sizeHint().
- See also
- maximumSize()
- Since
- 4.1
Definition at line 1064 of file kfileitemdelegate.cpp.
|
virtual |
Reimplemented from QAbstractItemDelegate.
Reimplemented from QAbstractItemDelegate.
Definition at line 1498 of file kfileitemdelegate.cpp.
void KFileItemDelegate::setShadowBlur | ( | qreal | radius | ) |
Sets the blur radius for the text shadow.
- See also
- shadowBlur()
Definition at line 1052 of file kfileitemdelegate.cpp.
void KFileItemDelegate::setShadowColor | ( | const QColor & | color | ) |
Sets the color used for drawing the text shadow.
To enable text shadows, set the shadow color to a non-transparent color. To disable text shadows, set the color to Qt::transparent.
- See also
- shadowColor()
Definition at line 1028 of file kfileitemdelegate.cpp.
void KFileItemDelegate::setShadowOffset | ( | const QPointF & | offset | ) |
Sets the horizontal and vertical offset for the text shadow.
- See also
- shadowOffset()
Definition at line 1040 of file kfileitemdelegate.cpp.
void KFileItemDelegate::setShowInformation | ( | const InformationList & | list | ) |
Sets the list of information lines that are shown below the icon label in list views.
You will typically construct the list like this:
The information lines will be displayed in the list order. The delegate will first draw the item label, and then as many information lines as will fit in the available space.
- Parameters
-
list A list of information items that should be shown
Definition at line 1007 of file kfileitemdelegate.cpp.
void KFileItemDelegate::setShowInformation | ( | Information | information | ) |
Sets a single information line that is shown below the icon label in list views.
This is a convenience function for when you only want to show a single line of information.
- Parameters
-
information The information that should be shown
Definition at line 1013 of file kfileitemdelegate.cpp.
void KFileItemDelegate::setShowToolTipWhenElided | ( | bool | showToolTip | ) |
Sets whether a tooltip should be shown if the display role is elided containing the full display role information.
- Note
- The tooltip will only be shown if the Qt::ToolTipRole differs from Qt::DisplayRole, or if they match, showToolTipWhenElided flag is set and the display role information is elided.
- See also
- showToolTipWhenElided()
- Since
- 4.2
Definition at line 1076 of file kfileitemdelegate.cpp.
void KFileItemDelegate::setWrapMode | ( | QTextOption::WrapMode | wrapMode | ) |
When the contents text needs to be wrapped, wrapMode
strategy will be followed.
- Since
- 4.4
Definition at line 1088 of file kfileitemdelegate.cpp.
qreal KFileItemDelegate::shadowBlur | ( | ) | const |
Returns the blur radius for the text shadow.
- See also
- setShadowBlur()
QColor KFileItemDelegate::shadowColor | ( | ) | const |
Returns the color used for the text shadow.
- See also
- setShadowColor()
QPointF KFileItemDelegate::shadowOffset | ( | ) | const |
Returns the offset used for the text shadow.
- See also
- setShadowOffset()
|
slot |
Returns the shape of the item as a region.
The returned region can be used for precise hit testing of the item.
Definition at line 1587 of file kfileitemdelegate.cpp.
KFileItemDelegate::InformationList KFileItemDelegate::showInformation | ( | ) | const |
Returns the file item information that should be shown below item labels in list views.
Definition at line 1022 of file kfileitemdelegate.cpp.
bool KFileItemDelegate::showToolTipWhenElided | ( | ) | const |
Returns whether a tooltip should be shown if the display role is elided containing the full display role information.
- Note
- The tooltip will only be shown if the Qt::ToolTipRole differs from Qt::DisplayRole, or if they match, showToolTipWhenElided flag is set and the display role information is elided.
- See also
- setShowToolTipWhenElided()
- Since
- 4.2
|
virtual |
Returns the nominal size for the item referred to by index
, given the provided options.
If the model provides a valid Qt::FontRole and/or Qt::AlignmentRole for the item, those will be used instead of the ones specified in the style options.
This function is reimplemented from QAbstractItemDelegate.
- Parameters
-
option The style options that should be used when painting the item. index The index to the item for which to return the size hint.
Definition at line 941 of file kfileitemdelegate.cpp.
|
virtual |
Reimplemented from QAbstractItemDelegate.
Reimplemented from QAbstractItemDelegate.
Definition at line 1507 of file kfileitemdelegate.cpp.
QTextOption::WrapMode KFileItemDelegate::wrapMode | ( | ) | const |
Returns the wrapping strategy followed to show text when it needs wrapping.
- Since
- 4.4
Definition at line 1094 of file kfileitemdelegate.cpp.
Property Documentation
|
readwrite |
This property holds which additional information (if any) should be shown below items in icon views.
Access functions:
- void setShownformation(InformationList information)
- InformationList showInformation() const
Definition at line 79 of file kfileitemdelegate.h.
|
readwrite |
This property determines if there are KIO jobs on a destination URL visible, then they will have a small animation overlay displayed on them.
- Since
- 4.5
Definition at line 135 of file kfileitemdelegate.h.
|
readwrite |
This property holds the maximum size that can be returned by KFileItemDelegate::sizeHint().
If the maximum size is empty, it will be ignored.
Definition at line 119 of file kfileitemdelegate.h.
|
readwrite |
This property holds the blur radius for the text shadow.
The default value for this property is 2.
Access functions:
- void setShadowBlur(qreal radius)
- qreal shadowBlur() const
Definition at line 112 of file kfileitemdelegate.h.
|
readwrite |
This property holds the color used for the text shadow.
The alpha value in the color determines the opacity of the shadow. Shadows are only rendered when the alpha value is non-zero. The default value for this property is Qt::transparent.
Access functions:
Definition at line 92 of file kfileitemdelegate.h.
|
readwrite |
This property holds the horizontal and vertical offset for the text shadow.
The default value for this property is (1, 1).
Access functions:
Definition at line 102 of file kfileitemdelegate.h.
|
readwrite |
This property determines whether a tooltip will be shown by the delegate if the display role is elided.
This tooltip will contain the full display role information. The tooltip will only be shown if the Qt::ToolTipRole differs from Qt::DisplayRole, or if they match, showToolTipWhenElided flag is set and the display role information is elided.
Definition at line 128 of file kfileitemdelegate.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.